C++ parallel computing allows programmers to execute multiple operations simultaneously, enhancing performance by utilizing multi-core processors effectively.
Here’s a simple code snippet that demonstrates parallel computing using the OpenMP library:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel for
for (int i = 0; i < 10; i++) {
std::cout << "Thread " << omp_get_thread_num() << " processes index " << i << std::endl;
}
return 0;
}
What is Parallel Computing?
Parallel computing is a computational model that enables multiple processes or threads to execute simultaneously. This approach significantly enhances performance, particularly for complex problems that require substantial computational power. The primary benefits of parallel computing include increased speed, improved resource utilization, and enhanced execution of heavy tasks. By distributing workloads across multiple processors, parallel computing drastically reduces the time required to complete computations.
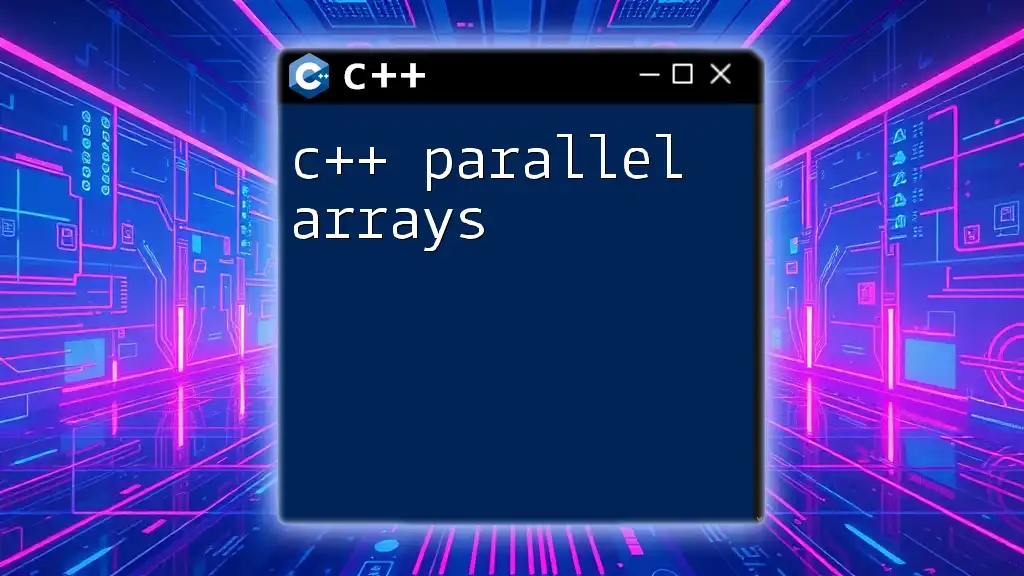
Why C++ for Parallel Computing?
C++ is a widely-used programming language known for its efficiency and performance. It gives developers fine-grained control over system resources, making it an excellent choice for implementing parallel computing techniques. C++ also offers an array of libraries and tools that facilitate parallel programming. This combination of performance and capabilities makes C++ a strong contender for developers tackling parallel computing challenges.
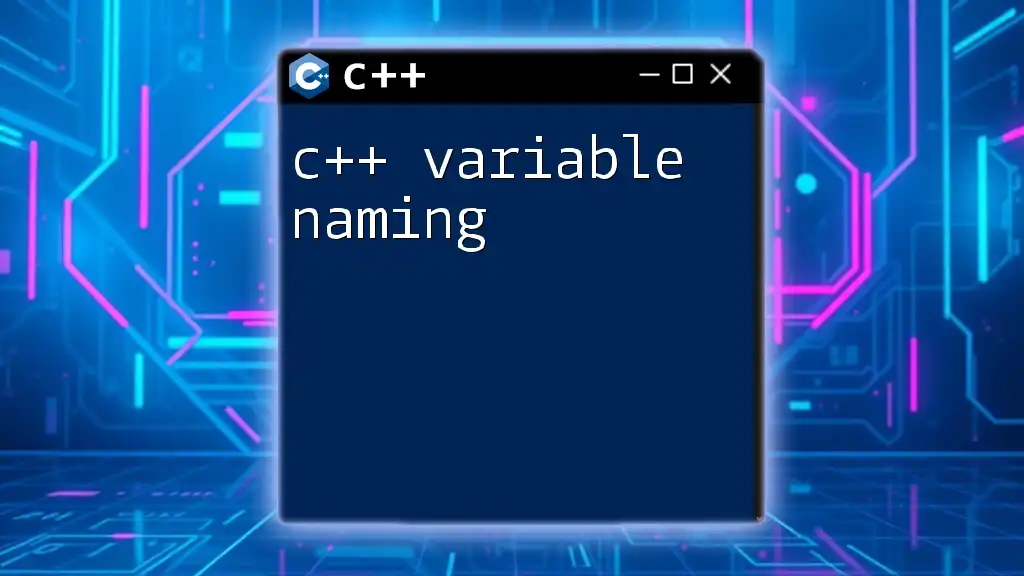
Key Concepts of Parallel Computing
Understanding Threads and Processes
Threads and processes are foundational elements of parallel computing.
- Threads are lightweight processes that share the same memory space but execute instructions independently. They are less resource-intensive, making them ideal for tasks that require frequent context switching.
- Processes, on the other hand, execute in separate memory spaces. They are more isolated from each other and are generally heavier than threads.
Why use threads in C++? Since threads consume fewer resources, they enable developers to maximize the number of concurrent executions in parallel computing tasks.
Parallelism vs. Concurrency
- Parallelism refers specifically to performing multiple computations simultaneously. This means running multiple threads or processes at the exact same time, maximizing hardware utilization.
- Concurrency focuses on managing multiple computations at once but not necessarily executing them simultaneously. Concurrency allows for interleaving of tasks, providing the illusion of parallelism.
Choosing between parallelism and concurrency depends on the problem at hand and the desired efficiency.
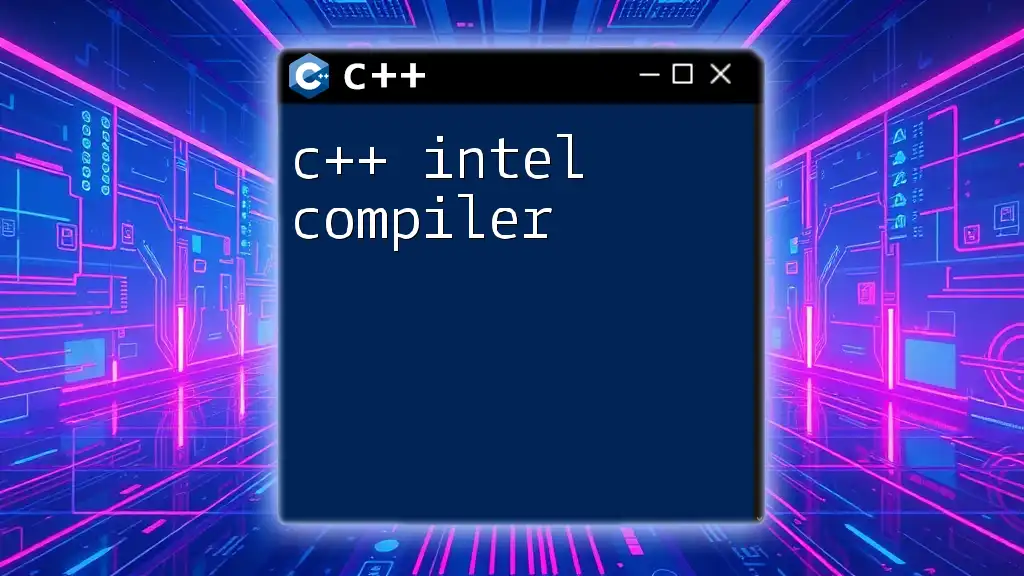
C++ Parallel Programming Constructs
The Standard Thread Library
C++ provides comprehensive support for threading via the Standard Template Library (STL). The library includes the `<thread>` header, allowing developers to create and manage threads easily.
To illustrate basic thread usage in C++, consider the following example:
#include <iostream>
#include <thread>
void function1() {
std::cout << "Thread Function 1\n";
}
int main() {
std::thread t1(function1);
t1.join(); // Wait for thread t1 to finish
return 0;
}
In this example, we defined a simple thread that executes a function printing a message. The `join()` method ensures that the main thread waits for `t1` to complete, preventing premature termination.
Thread Synchronization Mechanisms
As threads work independently, synchronization becomes essential to avoid data inconsistencies. C++ offers several mechanisms for synchronization, with mutexes being among the most commonly used.
A mutex (short for 'mutual exclusion') ensures that only one thread accesses a resource at a given time. Here’s how to implement a mutex in C++:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Mutex declaration
void printTask(int id) {
mtx.lock(); // Lock mutex to prevent race condition
std::cout << "Task ID: " << id << "\n";
mtx.unlock(); // Unlock mutex
}
In this example, the mutex protects the shared output, ensuring consistent thread behavior.
Condition variables are another synchronization mechanism available in C++. They are particularly useful in scenarios like producer-consumer problems, where threads must wait for certain conditions to be met before proceeding.
C++ Parallel Algorithms
With the arrival of C++17, the introduction of parallel algorithms has streamlined the way developers implement parallel processing. The `<algorithm>` and `<execution>` headers allow for easy parallelization of algorithms.
For example, consider using `std::sort` in a parallel manner:
#include <vector>
#include <algorithm>
#include <execution>
int main() {
std::vector<int> vec = {4, 2, 5, 1, 3};
std::sort(std::execution::par, vec.begin(), vec.end()); // Parallel sort
return 0;
}
In this snippet, we utilize the `std::execution::par` to sort the vector in parallel, significantly enhancing performance on large datasets.
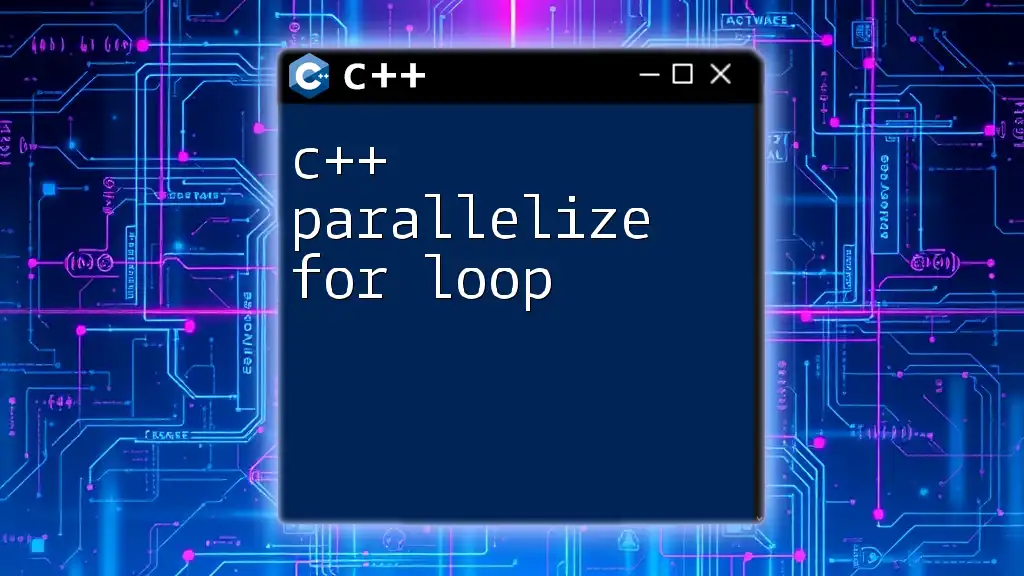
Advanced Parallel Processing Techniques
Task-Based Parallelism
A task-based parallelism model breaks a workload into smaller tasks that can be executed independently. This model efficiently distributes tasks across available processing units, optimizing resource usage.
Thread pools play a crucial role in task-based architectures. They consist of a number of worker threads that execute tasks from a shared queue. An example of using `std::async` for creating a simple task that executes asynchronously might look like this:
#include <iostream>
#include <future>
int calculate() {
return 42; // Example calculation
}
int main() {
std::future<int> result = std::async(calculate);
std::cout << "Result: " << result.get() << "\n"; // Wait and get result
return 0;
}
In this case, `std::async` creates a task that runs `calculate()` in the background, allowing the main program to continue executing without waiting for the task to finish.
OpenMP for Parallel Processing in C++
OpenMP (Open Multi-Processing) is an API that enables developers to write parallel programs using a straightforward directive-based approach. It abstracts much of the complexity typically associated with thread management.
As an example, consider the following program that demonstrates the fork-join model using OpenMP:
#include <omp.h>
#include <iostream>
int main() {
#pragma omp parallel
{
std::cout << "Hello from thread " << omp_get_thread_num() << "\n";
}
return 0;
}
With OpenMP, the `#pragma omp parallel` directive creates a team of threads that execute the enclosed block, showcasing parallel processing effortlessly.
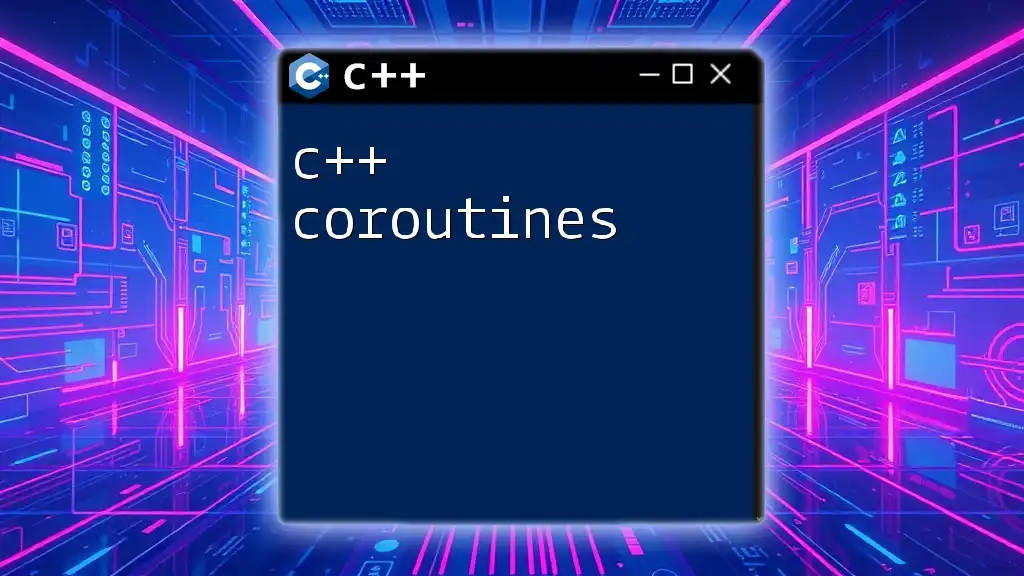
Challenges in C++ Parallel Programming
Debugging and Testing Concurrent Programs
Parallel programming introduces unique challenges, particularly concerning debugging. Common issues include race conditions, deadlocks, and resource starvation. Race conditions arise when multiple threads access shared resources concurrently, potentially causing inconsistencies. Deadlocks occur when threads wait indefinitely for resources held by each other.
To effectively navigate these challenges, developers can utilize tools like Valgrind, especially the Helgrind tool, which helps detect race conditions.
Performance Considerations
Measuring the performance of parallel programs is critical for identifying bottlenecks. Techniques like profiling and benchmarking can provide insights into the efficiency of a parallel solution.
To enhance performance, it’s vital to minimize overhead costs associated with thread management, synchronization, and communication. Careful design of algorithms, selection of data structures, and thoughtful partitioning of tasks can lead to significant performance improvements in parallel computing applications.
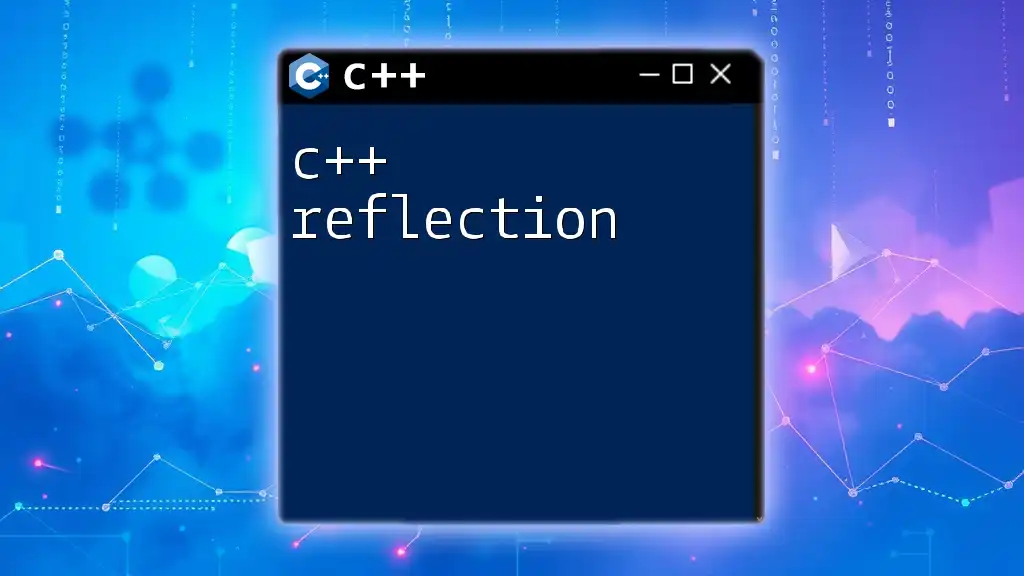
Conclusion
C++ parallel computing offers a wealth of opportunities for developers seeking to leverage multiple processing units to enhance performance. With a rich tapestry of resources, techniques, and libraries available, C++ stands out as a powerful language for implementing parallel computing models.
By experimenting with the constructs, libraries, and approaches outlined in this guide, developers can unlock the full potential of C++ parallel computing and push the boundaries of what is possible in software performance.