In C++, you can parallelize a `for` loop using the Standard Library's `std::async` or OpenMP to improve performance by distributing iterations across multiple threads.
Here's a simple example using OpenMP:
#include <omp.h>
#include <iostream>
int main() {
const int N = 1000;
int sum = 0;
#pragma omp parallel for reduction(+:sum)
for (int i = 0; i < N; ++i) {
sum += i;
}
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Understanding Parallel Programming in C++
What is Parallel Programming?
Parallel programming is a method used to enhance computational efficiency by dividing tasks into smaller sub-tasks that can be executed simultaneously. By doing so, it leverages multiple processing units, leading to faster execution times and effective resource utilization.
Benefits of parallel programming include increased speed and efficiency, reduction in execution time for extensive computational tasks, and enhanced scalability for applications dealing with large datasets or requiring complex calculations.
Why Use Parallel Loops?
When you have a task that can be divided into independent operations, using parallel loops can substantially cut down processing time. This is especially beneficial in applications like data analysis, image processing, simulations, and any scenario where large datasets are manipulated.
Real-world applications include:
- Scientific simulations, where multiple calculations are performed concurrently.
- Data processing, where actions on elements of large datasets can be carried out in parallel to optimize speed.
- Machine learning, where model training can be accelerated through parallel computation.
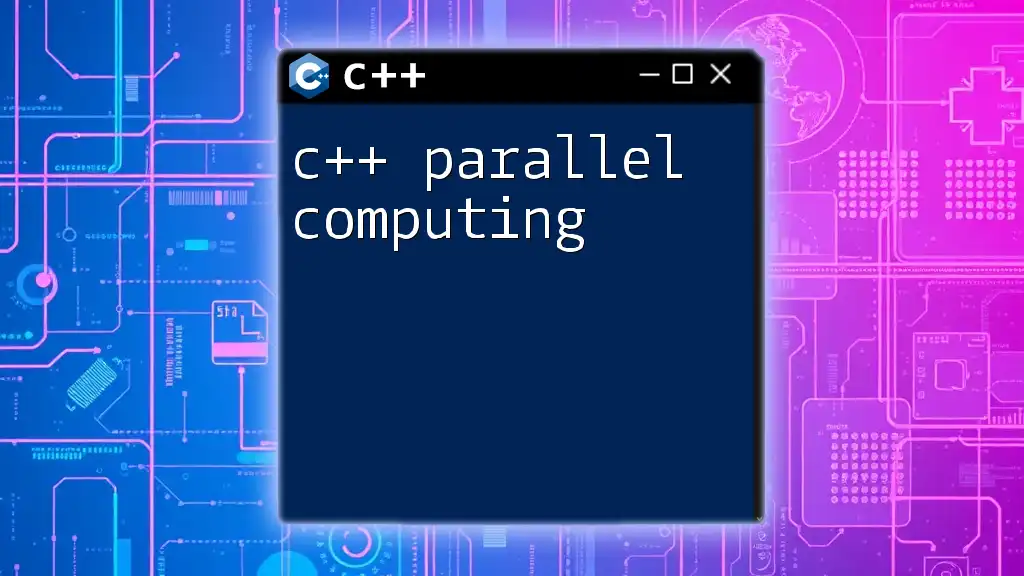
C++ Parallel Features
C++ Standard Library and Thread Support
C++ introduced a rich set of parallel programming features primarily through its Standard Library starting with C++11. Important components include `<thread>`, `<mutex>`, and `<future>` which facilitate multithreading and asynchronous programming models.
The capability introduced in recent C++ standards allows developers to write efficient multi-threaded applications that maximize the potential of modern multi-core processors.
Introduction to Parallel Algorithms
C++17 took usability a step further with the introduction of parallel algorithms found in the `<execution>` header. This allows you to write algorithms that can automatically optimize for parallel execution, applying the same syntax as traditional algorithms. This makes it easier for developers to take advantage of concurrency without deep knowledge of thread management.
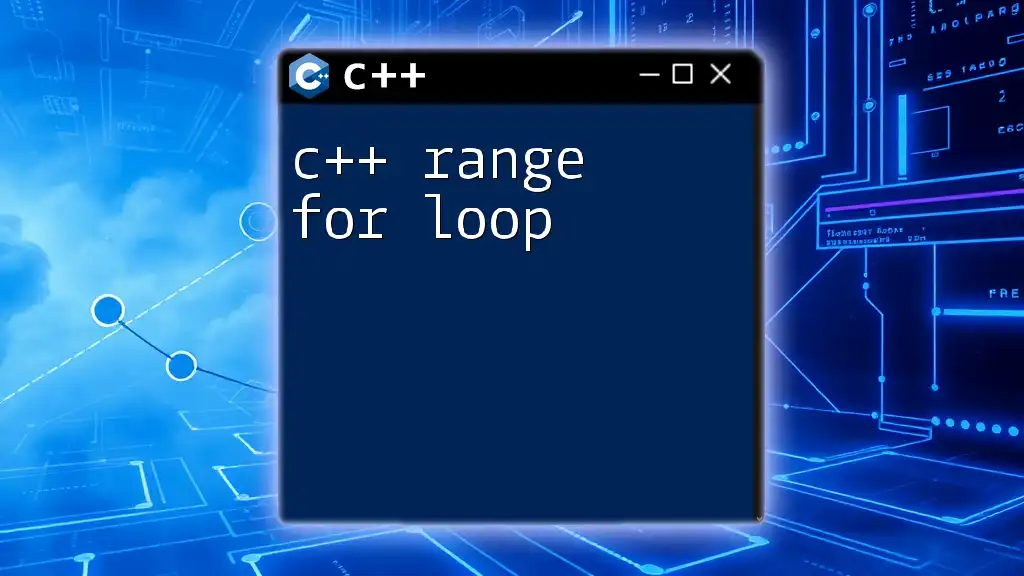
How to Implement a Parallel For Loop in C++
Syntax and Basic Structure
A parallel for loop can be structured similarly to a standard for loop, with the distinction that it allows multiple iterations to run simultaneously.
Here's a simple example using C++'s parallel capabilities:
#include <algorithm>
#include <execution>
#include <vector>
std::vector<int> data = {1, 2, 3, 4, 5};
std::for_each(std::execution::par, data.begin(), data.end(), [](int &n) {
n *= 2; // Example operation
});
In this code snippet, `std::for_each` is employed with `std::execution::par`, indicating that the operation on `data` should be executed in parallel.
Parallelizing a Simple For Loop
You can parallelize a basic initialization loop, as shown below:
#include <vector>
#include <iostream>
#include <algorithm>
#include <execution>
int main() {
std::vector<int> data(100);
for (size_t i = 0; i < data.size(); ++i) {
data[i] = i; // Simple initialization
}
std::for_each(std::execution::par, data.begin(), data.end(), [](int &n) {
n *= 2; // Parallel operation
});
for (const auto &val : data) {
std::cout << val << " ";
}
return 0;
}
In this example, initialization of `data` takes place in a traditional manner, but the operation where each element is doubled runs in parallel, enhancing performance considerably.
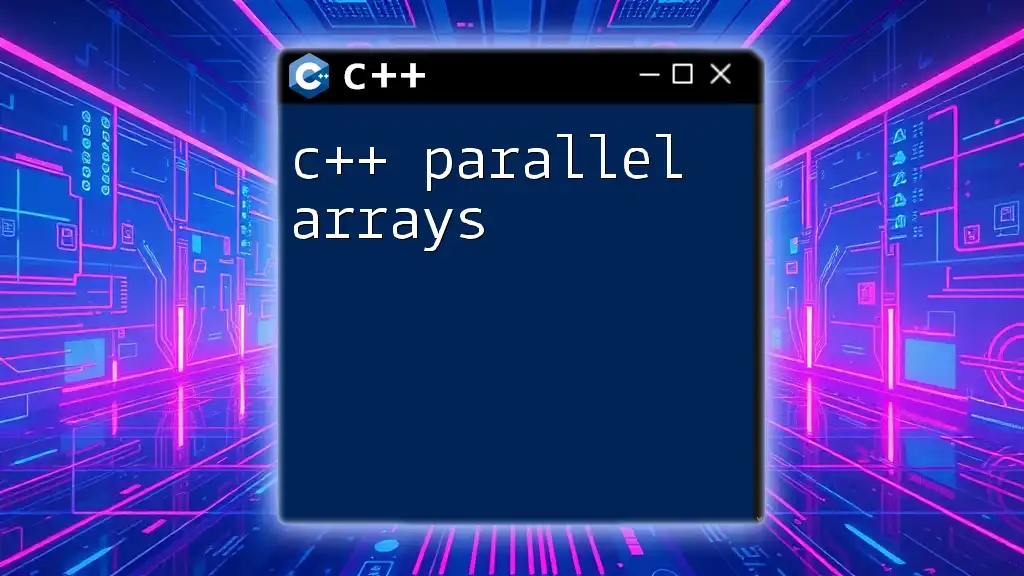
Common Mistakes and Optimizations
Mistakes to Avoid in Parallel Loops
When using parallel loops, developers must be cautious of race conditions. This occurs when multiple threads access shared data and attempt to modify it concurrently, leading to unpredictable outcomes.
It is essential to ensure thread safety by:
- Using appropriate synchronization mechanisms (like mutexes).
- Avoiding shared states where possible.
Tips for Optimizing Performance
To maximize the benefits of adopting C++ parallelization, consider:
- Utilizing data structures that afford concurrency, such as concurrent queues or thread-safe collections.
- Carefully determining task granularity. Dividing work into fine-grained tasks may lead to more overhead, so finding the sweet spot is crucial.
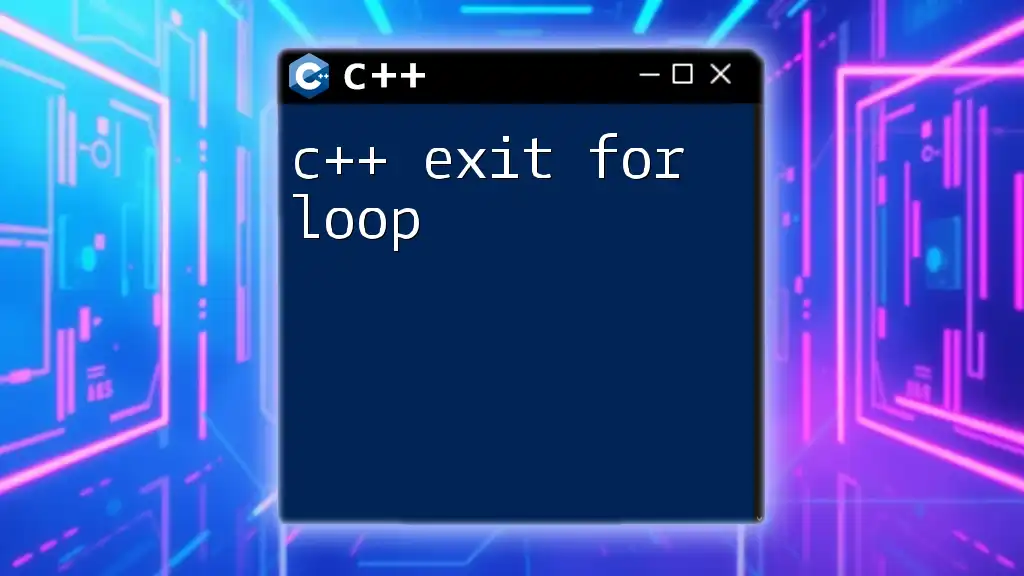
Advanced Parallel Loop Techniques
Work Distribution Strategies
Efficient parallelization often involves clever work distribution strategies. These may include:
- Chunking tasks into smaller pieces which are then distributed across available threads.
- Task spawning, where smaller tasks are dynamically created and assigned to threads based on availability.
Load balancing is a key consideration; distributing workloads evenly can significantly improve performance by preventing some threads from becoming a bottleneck.
Combining Parallel Loops and Other Features
Another powerful aspect of C++ parallel programming is the ability to combine parallel loops with asynchronous features such as futures and promises. This can help manage tasks that need to wait for results from other computations to proceed, offering a higher level of control over execution flow.
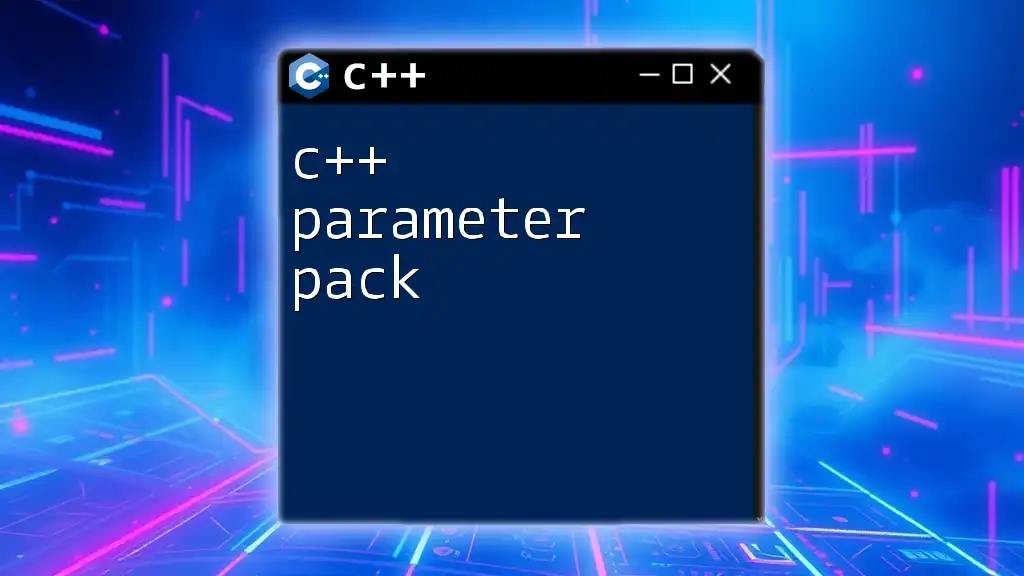
Conclusion
Utilizing C++ parallelization techniques like the parallel for loop can dramatically improve the efficiency of your applications. As you explore this programming style, you’ll find a wealth of opportunities to optimize workloads and enhance performance. Embracing these techniques will not only make your code faster but also prepare you for the challenges of modern programming with heavy computational requirements.
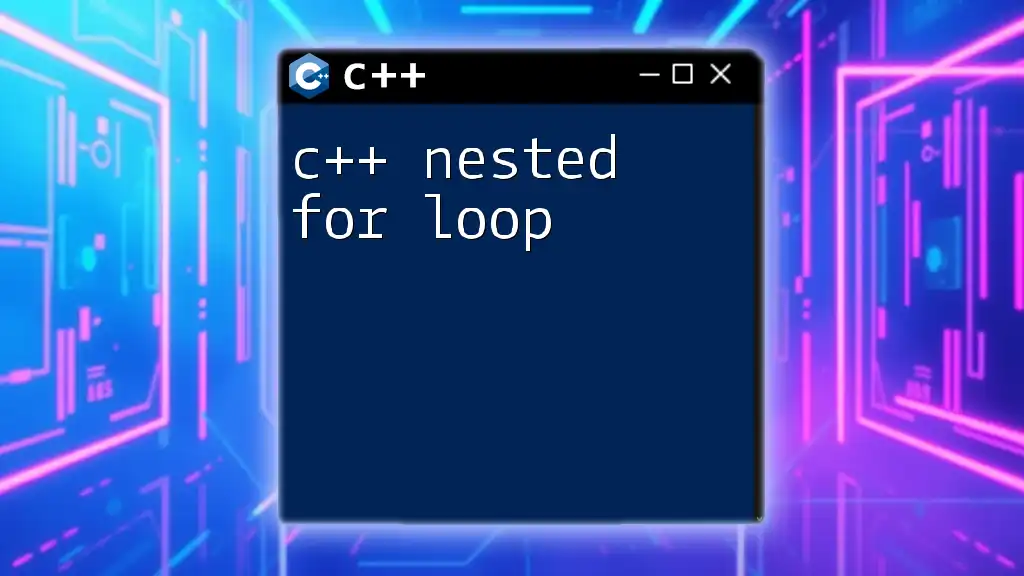
Additional Resources
Consider delving into recommended books, online courses, and official documentation available for C++ parallel programming. These resources can provide deeper insights and enhance your understanding of advanced techniques.
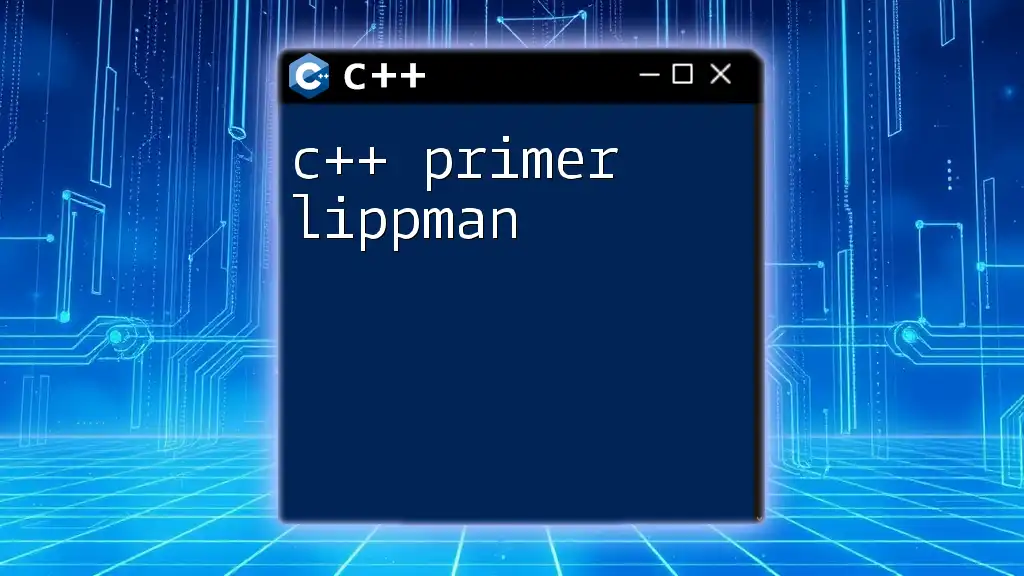
Call to Action
Have you experimented with parallel loops in your C++ projects? Share your experiences in the comments below! If there are other topics you’re interested in learning about, let us know for future content.