The C++ range for loop provides a simplified syntax for iterating over elements in a container, such as an array or vector, without needing to manage iterators or indices manually.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a C++ Range Based For Loop?
The C++ range for loop, also known as the range based for loop, is a powerful feature introduced in C++11. It allows developers to iterate over all elements in a collection, such as arrays, vectors, or sets, in a more concise and readable manner.
One of the key advantages of using the range based for loop is that it eliminates the potential for common errors that can occur with traditional for loops, such as off-by-one mistakes or accessing invalid indices. It provides clearer, more maintainable code, enhancing overall readability.
The syntax is notably simpler compared to traditional for loops, making it an excellent choice for quick iterations without sacrificing clarity.
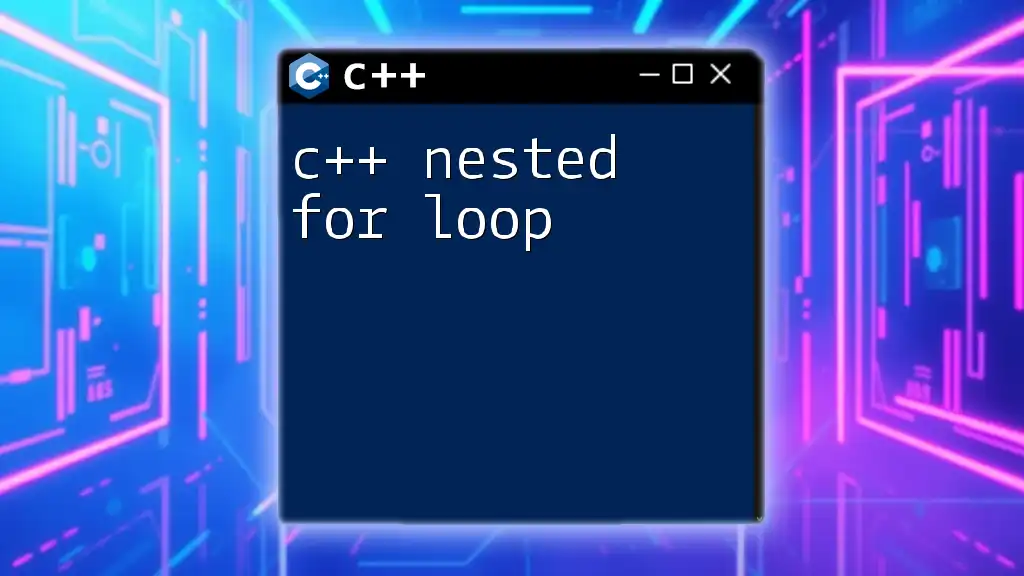
Syntax of C++ Range Based For Loop
The basic syntax of a C++ range for loop is as follows:
for (declaration : collection) {
// body of the loop
}
Explanation of Each Part of the Syntax
-
declaration: This is where you define the type and name of the loop variable, which will take on the values from the collection during each iteration.
-
collection: This represents the iterable container, such as an array, vector, or any other C++ container that supports iteration.
Example: Basic Syntax
Here’s a simple example demonstrating the basic syntax of the range based for loop:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto number : numbers) {
std::cout << number << " ";
}
Output: `1 2 3 4 5`
This example shows how to use a range for loop to iterate over a vector of integers, automatically determining the type of `number` using `auto`.
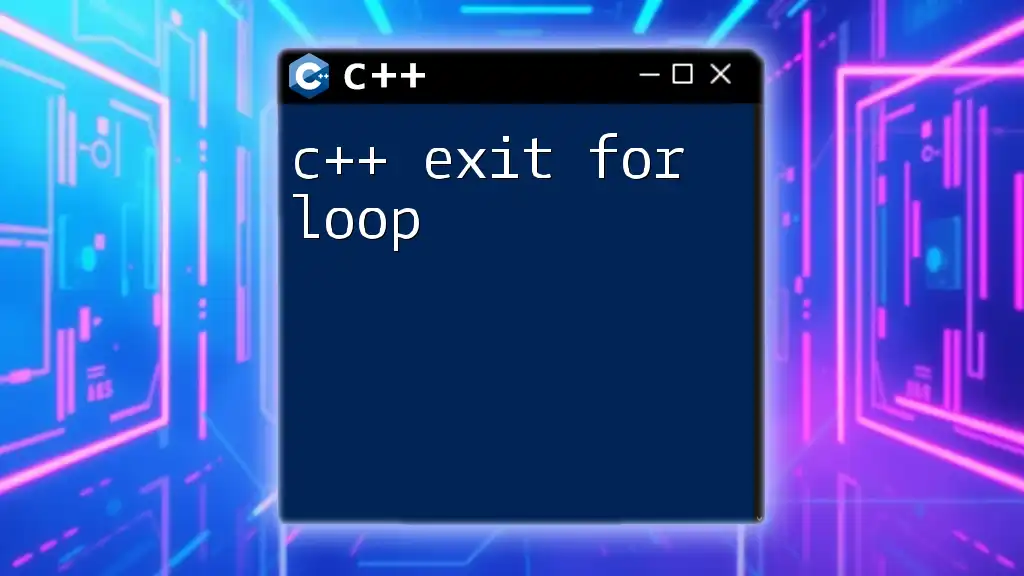
How to Use C++ Range Based For Loop
Iterating Over Arrays
Iterating over C++ arrays using a range based for loop is straightforward. Here’s how you can do it:
int arr[] = {10, 20, 30, 40};
for (auto element : arr) {
std::cout << element << " ";
}
In this code, `element` takes each value from the array `arr` in turn, allowing access to each element without needing to manage an index variable.
Iterating Over Vectors
Vectors are one of the most common collections in C++. The range based for loop simplifies iterations over vectors significantly:
std::vector<std::string> fruits = {"Apple", "Banana", "Cherry"};
for (auto fruit : fruits) {
std::cout << fruit << " ";
}
This example clearly shows how easy it is to access each string in the vector `fruits`.
Iterating Over Maps
Iterating over maps can be a little more complicated due to their key-value structure. However, the range based for loop simplifies this as follows:
std::map<std::string, int> fruitCount = {{"Apple", 5}, {"Banana", 3}};
for (auto const& item : fruitCount) {
std::cout << item.first << ": " << item.second << " ";
}
In this example, `item.first` accesses the key, while `item.second` accesses the value, effectively allowing you to iterate through the map’s contents.
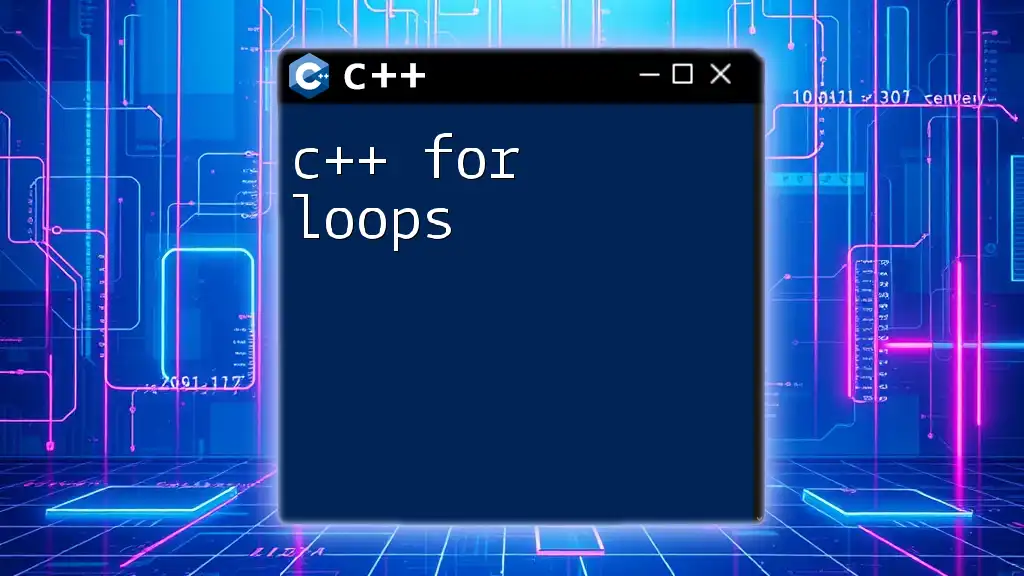
Important Concepts Related to C++ Range Based For Loop
Using `auto` in Range Based For Loop
The `auto` keyword in C++ is a powerful feature that allows the compiler to automatically deduce the type of a variable. This can simplify your code even further, especially when working with complex data types.
Here’s an example:
for (auto num : {1, 2, 3.5, 4.2}) {
std::cout << num << " ";
}
In this case, `num` will take on the type of each item in the initializer list, demonstrating the flexibility that `auto` provides.
Const References in C++ Range Based For Loop
To optimize performance, particularly when iterating over large collections, it’s often beneficial to use const references. This prevents unnecessary copying of objects. Here’s an example:
std::vector<int> nums = {1, 2, 3, 4};
for (const auto& num : nums) {
std::cout << num << " ";
}
By using `const auto&`, the loop variable `num` will refer to each element in `nums` without copying it, resulting in more efficient code execution.
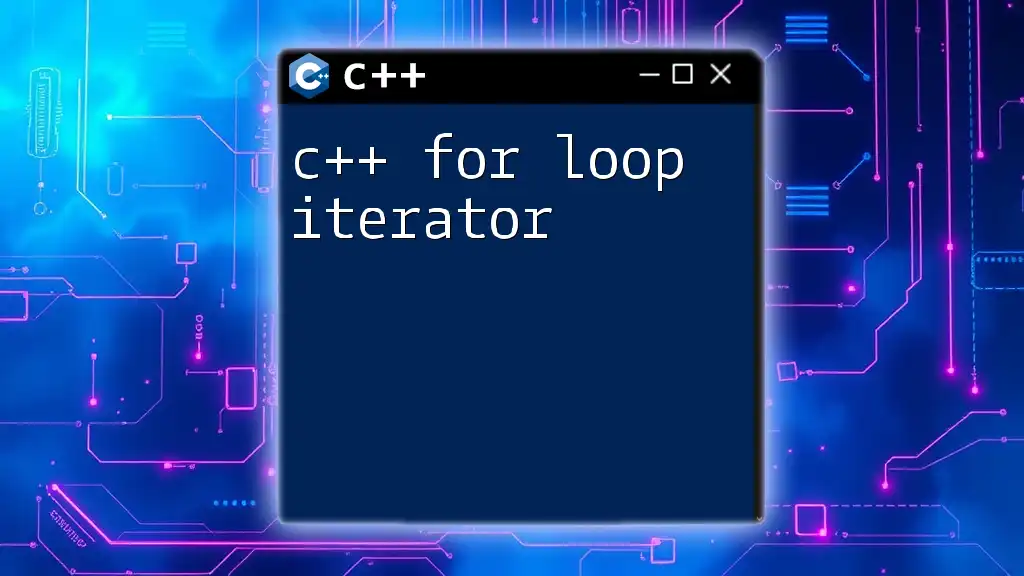
Common Mistakes to Avoid
While using a C++ range for loop can simplify your code, there are still common pitfalls to watch out for:
-
Modifying elements during iteration: Changing the contents of the collection while iterating over it can lead to unexpected behavior or runtime errors.
-
Failing to use `const`: Omitting `const` can result in unintended modifications to the elements of a collection when you only intend to read them.
-
Incorrect variable types: Always ensure that the declaration of your loop variable matches the type of elements held in your collection.
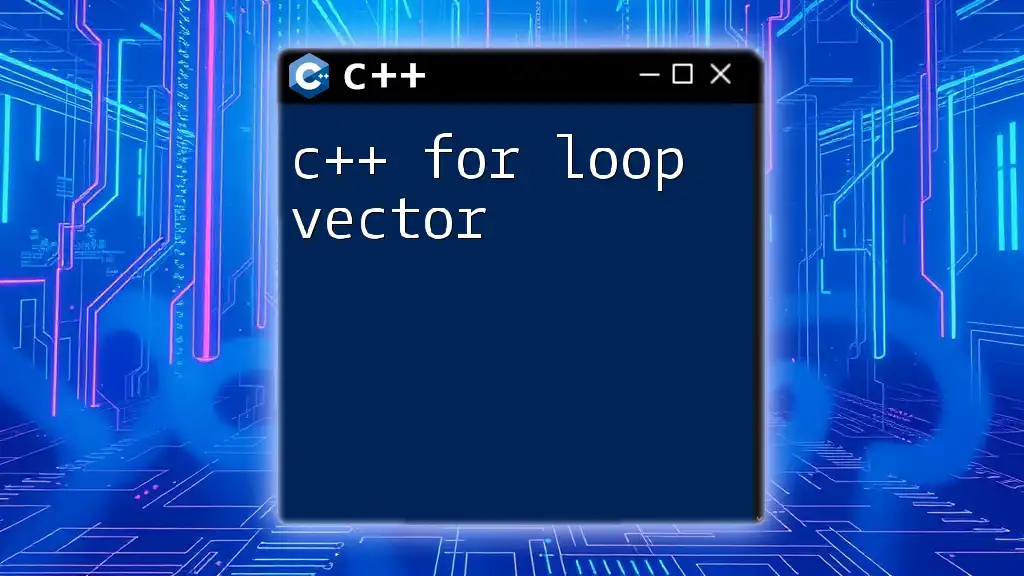
Performance Considerations
When it comes to performance, the C++ range based for loop can be more efficient than a traditional for loop, particularly because it can avoid the overhead of multiple function calls and the need for index tracking.
Additionally, using move semantics with range based for loops allows for efficient transfers of ownership, reducing copying overhead when working with temporary objects.
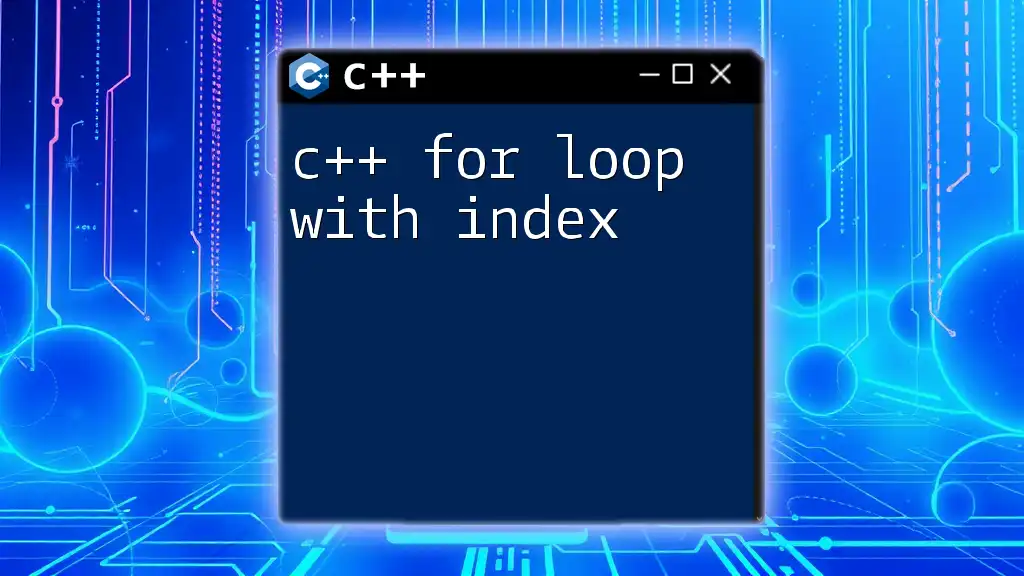
Conclusion
In summary, the C++ range for loop is a valuable tool for simplifying iterations over collections in a clear and concise manner. By leveraging its advantages, such as improved readability and reduced errors, you can write clean and maintainable code with ease.
Take the time to integrate C++ range based for loops into your programming practices and elevate the quality of your code. Explore more advanced features and enhance your skills through dedicated courses and practical applications.