To exit a loop in C++, you can use the `break` statement, which immediately terminates the nearest enclosing loop.
Here's a simple example:
#include <iostream>
int main() {
for (int i = 0; i < 10; ++i) {
if (i == 5) {
break; // Exit the loop when i is 5
}
std::cout << i << std::endl;
}
return 0;
}
Understanding the `for` Loop Structure
The `for` loop in C++ is a fundamental control structure used for iterating over a sequence of values. The basic syntax of a `for` loop consists of three key parts: initialization, condition, and increment/decrement. Here’s a breakdown of the syntax:
for (initialization; condition; increment/decrement) {
// Loop body
}
Example of a Simple `for` Loop
To illustrate, let’s take a look at a simple `for` loop that prints numbers from 0 to 9:
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
In this example:
- Initialization (`int i = 0`): sets the starting point of the loop.
- Condition (`i < 10`): determines when the loop stops executing.
- Increment (`i++`): increments the counter at the end of each iteration.
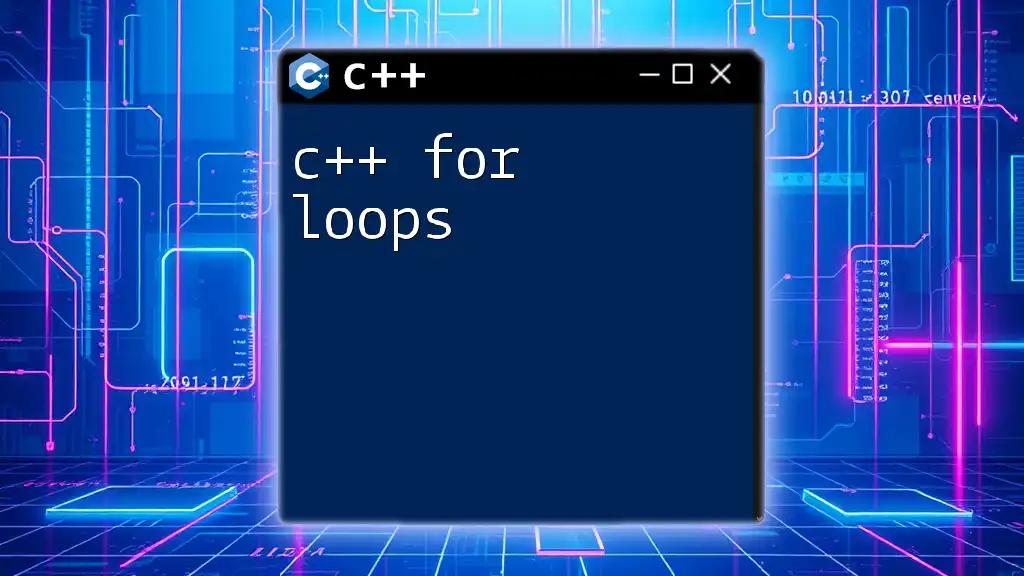
How to Exit a `for` Loop
Exiting a `for` loop effectively is essential to control program flow. The most common way to exit a loop prematurely is by using the `break` statement.
The `break` Statement
The `break` statement immediately terminates the loop in which it resides. It can be especially useful when a condition is met.
Example of Using `break` to Exit a Loop
Here’s a practical example of implementing `break` within a loop:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exits the loop when i is equal to 5
}
std::cout << i << std::endl;
}
Explanation of Example: In this code snippet, the loop will output the numbers 0 to 4. When `i` equals 5, the `break` statement is executed, causing the loop to terminate, demonstrating an effective method to exit a loop based on a specific condition.

Alternative Methods to Exit a `for` Loop
Besides `break`, there are other methods to exit a `for` loop.
The `return` Statement
The `return` statement can also be used for exiting a loop when it is placed within a function. When `return` is executed, the function terminates, which in turn stops any loops contained within it.
Example of Using `return` Within a Function
Here’s an example:
void loopUntilFive() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
return; // Exits the function and thus the loop
}
std::cout << i << std::endl;
}
}
Explanation: In this scenario, the function `loopUntilFive` prints numbers from 0 to 4. When `i` reaches 5, the `return` statement is executed, immediately terminating both the function and the loop. This method can be beneficial when you want to exit not just the loop, but the function entirely.
Using Flags for Loop Control
Another technique to manage control flow in a loop is through the use of flags. A flag is a variable that can be set to indicate whether certain conditions have been met.
Example of Flag-Based Exit
Here’s how you could apply a flag in a loop:
bool exitLoop = false;
for (int i = 0; i < 10 && !exitLoop; i++) {
if (i == 5) {
exitLoop = true; // Sets flag to exit the loop
}
std::cout << i << std::endl;
}
Explanation of Flags: In this example, the loop will execute as long as `exitLoop` is `false`. When `i` equals 5, `exitLoop` is set to `true`, causing the loop to exit the next time the condition is checked. This approach is particularly useful when you have more complex conditions for exiting the loop.

Common Pitfalls of Exiting a `for` Loop
Infinite Loops
One of the most common pitfalls associated with loops, including `for` loops, is the occurrence of infinite loops. An infinite loop happens when the loop's exit condition never becomes true. This can lead to your program freezing or crashing.
To avoid infinite loops:
- Ensure that the loop's increment or decrement is properly defined and executed.
- Carefully design the exit condition to guarantee it will eventually be met.
Overuse of `break`
While the `break` statement is a powerful tool for exiting loops, overusing it can lead to code that is hard to read and maintain. It can obscure the flow of control and make it difficult for others (or yourself later) to understand the logic behind the loop.
To maintain clear and readable code, use `break` judiciously and consider alternative structures when possible, like condition checks at the beginning of the loop.

Conclusion
In summary, understanding how to effectively c++ exit for loop operations is crucial for managing flow control in your programs. Using statements like `break` and `return`, as well as flag variables, can significantly enhance your ability to manage loop execution.
Experimenting with these techniques in your own code can provide valuable insights and improve your proficiency with C++. As you practice, you will develop a deeper understanding of loop behavior, enabling you to write cleaner, more efficient C++ programs.
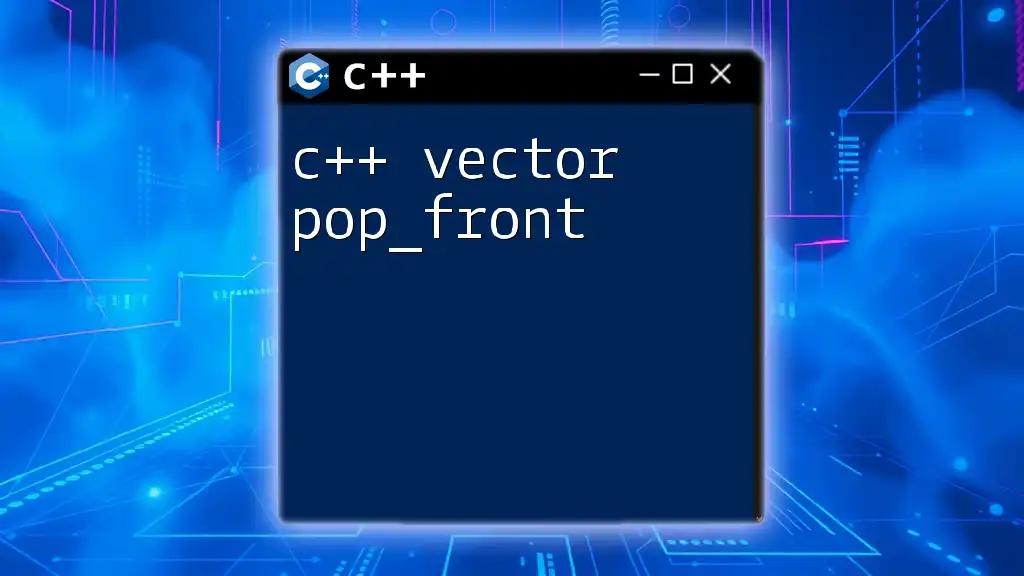
Additional Resources
To further delve into the nuances of C++ looping constructs, consider exploring:
- Comprehensive C++ documentation for advanced techniques.
- Online platforms and communities where you can engage with other developers for real-world advice and examples.