The enhanced for loop in C++ simplifies iterating over elements in a collection, allowing you to access each element directly without using an index.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding the Enhanced For Loop
What is the Enhanced For Loop?
The enhanced for loop, also known as the range-based for loop, is a specific type of loop introduced in C++11. It simplifies the process of iterating over collections such as arrays, vectors, and other STL containers. Unlike traditional for loops, which require explicit index management, the enhanced for loop provides a more readable and streamlined syntax, allowing you to focus on the operations being performed on each element rather than the mechanics of tracking the index.
The basic syntax of the enhanced for loop is as follows:
for (auto element : collection) {
// code to be executed
}
Key Features of the Enhanced For Loop
A few key features make the enhanced for loop particularly useful:
- Simplified Syntax: The syntax is concise and reduces boilerplate code, enhancing code readability.
- Automatic Handling of Iterators: The loop automatically manages iterators for you, eliminating potential errors related to iterator usage.
- Increased Readability: With fewer lines of code and clear intent, the enhanced for loop improves the maintainability of your code.
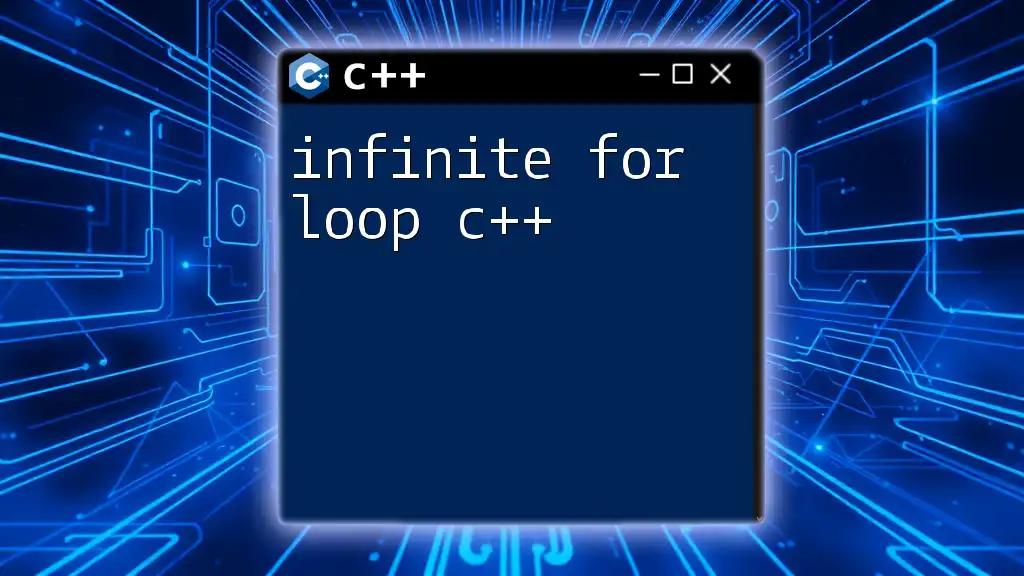
Syntax and Structure
Basic Syntax
The enhanced for loop consists of three primary components:
- Element: Represents the individual item from the collection that you will process.
- Collection: This is the range of items over which you want to iterate.
- Loop Body: The block of code that operates on each element.
Here's the structure repeated for clarity:
for (auto element : collection) {
// code to be executed
}
Using the `auto` Keyword
Using the `auto` keyword in the enhanced for loop facilitates type deduction, allowing the compiler to automatically determine the type of the element being iterated. This increases flexibility and reduces the need for explicit type declarations, leading to cleaner and more maintainable code. For instance:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
In this example, `num` is automatically deduced to be of type `int`, making the code succinct yet effective.
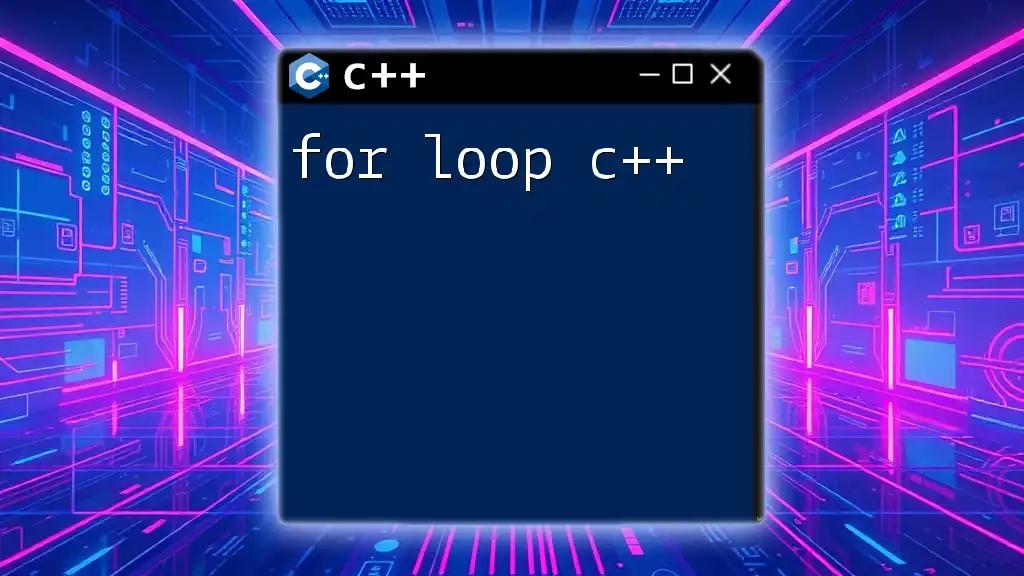
Using Enhanced For Loop with Different Data Structures
Arrays
The enhanced for loop works seamlessly with arrays, allowing you to iterate through each element effortlessly. Here's how you can utilize it:
int arr[] = {1, 2, 3, 4, 5};
for (auto num : arr) {
std::cout << num << " ";
}
In this example, `num` takes on the value of each element in `arr` one by one, making it easy to access and manipulate element values.
Vectors
When working with the C++ Standard Library's `std::vector`, the enhanced for loop provides a straightforward approach to iterate over elements:
std::vector<std::string> names = {"Alice", "Bob", "Charlie"};
for (auto name : names) {
std::cout << name << std::endl;
}
Each iteration assigns the current vector element to `name`, allowing you to perform operations just as easily.
Other Containers (Lists, Maps, Sets)
The enhanced for loop can also be utilized with other STL containers like `std::list`, `std::map`, and `std::set`. Here's a quick look at each:
For sets:
std::set<int> numbers = {1, 2, 3};
for (auto num : numbers) {
std::cout << num << " ";
}
For maps:
std::map<std::string, int> ages = {{"Alice", 30}, {"Bob", 25}};
for (const auto& pair : ages) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
In these cases, the enhanced for loop abstracts away the complexity of iterators, making it easier to focus on the task at hand.
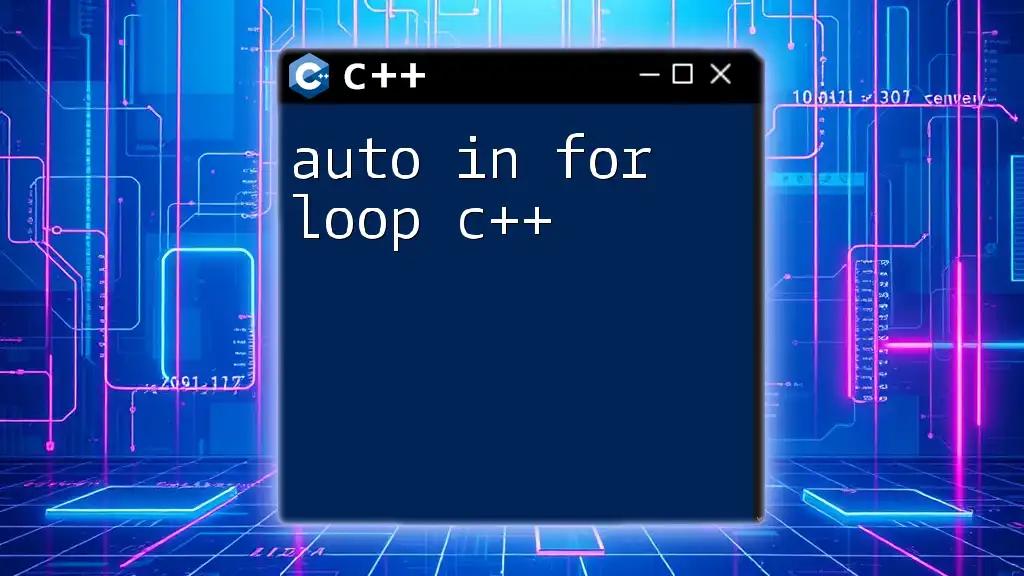
Advantages of Using Enhanced For Loop
Improved Readability
One of the prominent advantages of the enhanced for loop is its readability. The absence of manual index management means that the code is not only shorter but also clearer in intent. When others read your code, they can instantly understand the looping process without deciphering index calculations.
Avoiding Off-by-One Errors
Traditional loops often lead to off-by-one errors, where a loop might accidentally skip the first element or exceed the last element when iterating. The enhanced for loop eliminates this risk by directly accessing each element without the need for an explicit index. For example:
for (int i = 0; i <= size; ++i) { // This can lead to out-of-bounds access
// do something
}
This can often lead to frustrating bugs. Contrast this with the enhanced for loop, which handles this case safely:
for (auto item : collection) {
// process each item safely
}
No Need for Manual Iterators
The enhanced for loop abstracts away the need for manually managing iterators, which can be especially cumbersome. In a traditional loop, you may need to specify the beginning and end of a collection and manage an iterator explicitly. With the enhanced for loop, you can focus directly on the elements being processed, leading to a cleaner design.

Limitations of Enhanced For Loop
Cannot Modify Elements
While the enhanced for loop provides great benefits in terms of readability, one limitation is that it cannot modify elements directly. You can, however, use references to achieve modifications. For example:
for (auto& num : arr) {
num *= 2; // This modifies the original array elements
}
By using `auto&`, you ensure that `num` refers to the actual element of `arr`, allowing you to modify its value.
Compatibility with Non-Container Types
The enhanced for loop excels with STL containers but does not work with types that do not have a defined iterable interface. For instance, attempting to use an enhanced loop with basic data types or custom types without iterator support would result in compilation errors.
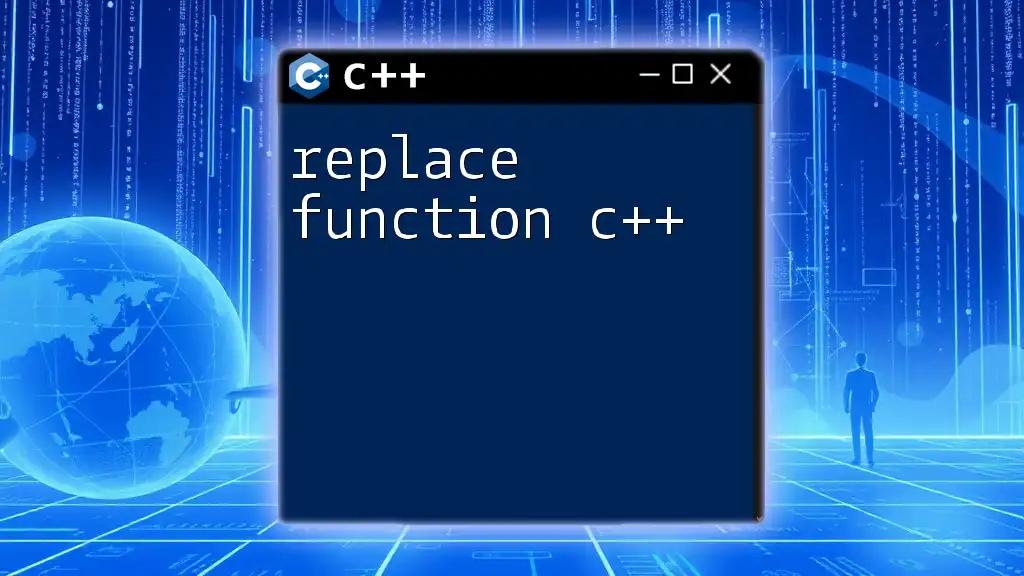
Conclusion
The enhanced for loop in C++ is an invaluable tool for any programmer, providing a clean and intuitive approach to iterating over collections. By reducing complexity, minimizing errors, and enhancing code readability, it sets a standard for clearer programming.
As you practice and implement the enhanced for loop, you'll find it integrates seamlessly into your coding style. I encourage you to explore further into the STL and continue refining your understanding of C++, enriching your skills as a developer.
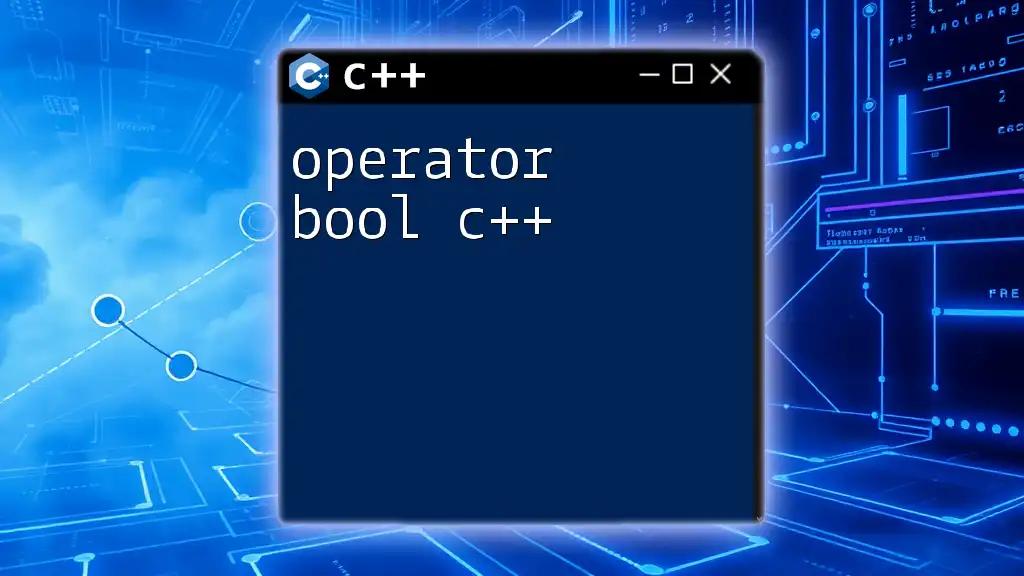
Additional Resources
For those looking to deepen their knowledge of C++, consider the following resources:
- The official C++ documentation for comprehensive details on STL and other features.
- Recommended books such as "Effective Modern C++" by Scott Meyers or "C++ Primer" by Lippman, Lajoie, and Moo.
- Online courses that cover C++ fundamentals and advanced topics, which often include practical applications of the enhanced for loop.
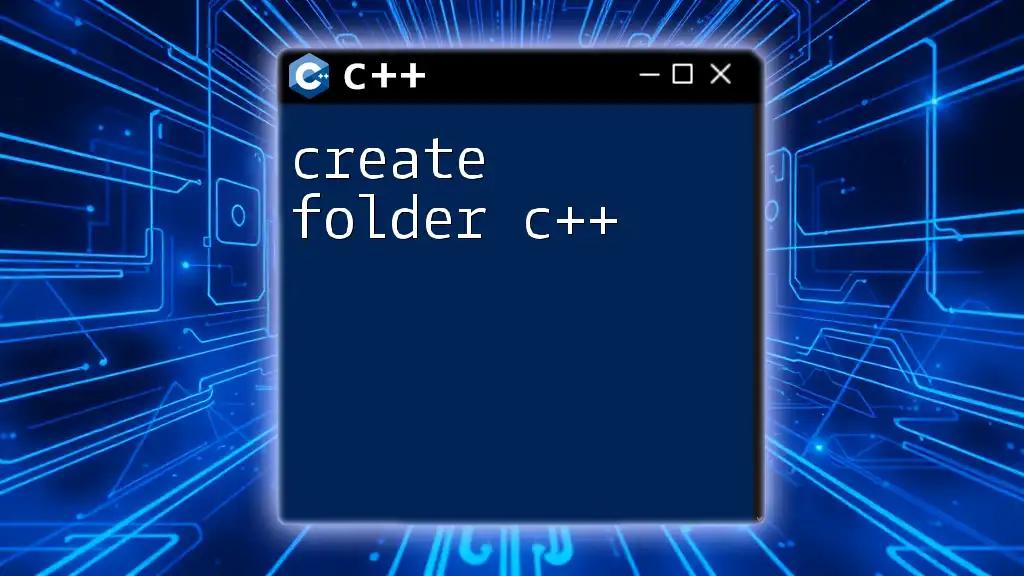
Call to Action
I invite you to share your experiences with the enhanced for loop, ask questions, or provide feedback in the comments below. Feel free to spread the word and share this guide with fellow programmers eager to enhance their C++ skills!