An infinite `for` loop in C++ continues to execute indefinitely until an external condition or event interrupts it, typically structured with the syntax `for(;;)` or `for(1; 1; 1)`.
Here’s a simple example:
#include <iostream>
int main() {
for (;;) {
std::cout << "This loop will run forever!" << std::endl;
}
return 0; // This line will never be reached
}
What is an Infinite For Loop in C++?
Understanding the Basic Concept
An infinite loop is a loop that runs indefinitely, either due to a specific condition that never becomes false or through the absence of such a condition. In C++, this typically arises when the termination condition in the loop structure is omitted, leading the program to continue executing the loop's body incessantly. Understanding infinite loops is crucial for effective programming, as they can lead to resource exhaustion if not controlled properly.
Real-World Use Cases
Infinite loops have valid use cases in various programming scenarios. For instance, they are often used in:
- Server applications: These programs need to handle requests continuously, thus requiring them to remain in a waiting state.
- Game loops: Many game engines utilize infinite loops to consistently refresh the game state and render graphics.
- Background processes: In embedded systems, loops may run indefinitely to monitor sensors or control actuators.
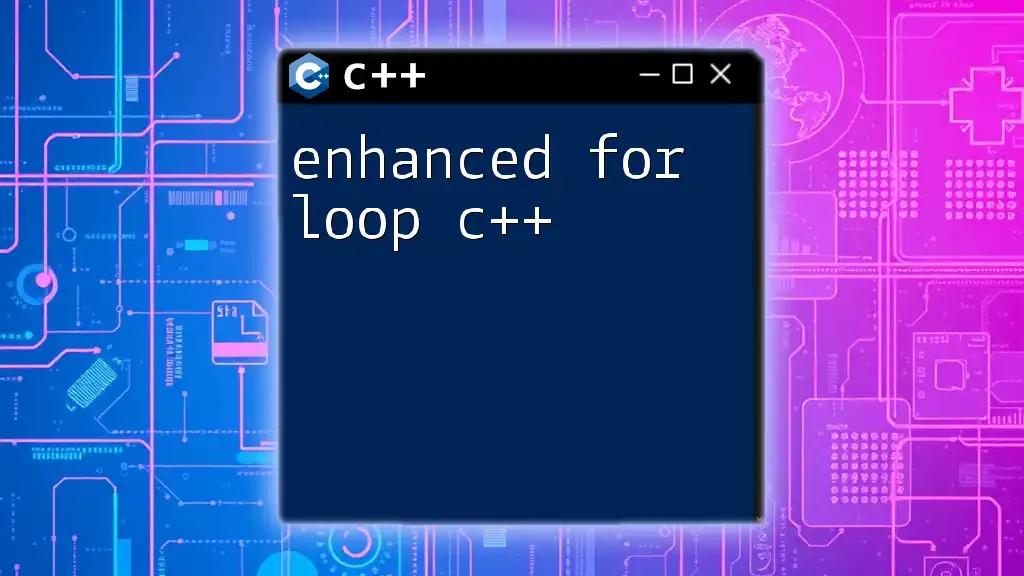
How to Create an Infinite For Loop in C++
Syntax of a For Loop
A typical for loop in C++ is structured as follows:
for (initialization; condition; increment/decrement) {
// Code to execute
}
This structure facilitates iteration through a set of operations. However, by manipulating its components, one can create an infinite loop.
Transforming For Loop into an Infinite Loop
An infinite for loop can be generated by leaving the condition section empty, effectively making it always evaluate to true, as shown below:
for (;;) {
// Code to execute indefinitely
}
In this example, because there are no conditions to check, the loop will run forever, executing the code contained within its block repeatedly.
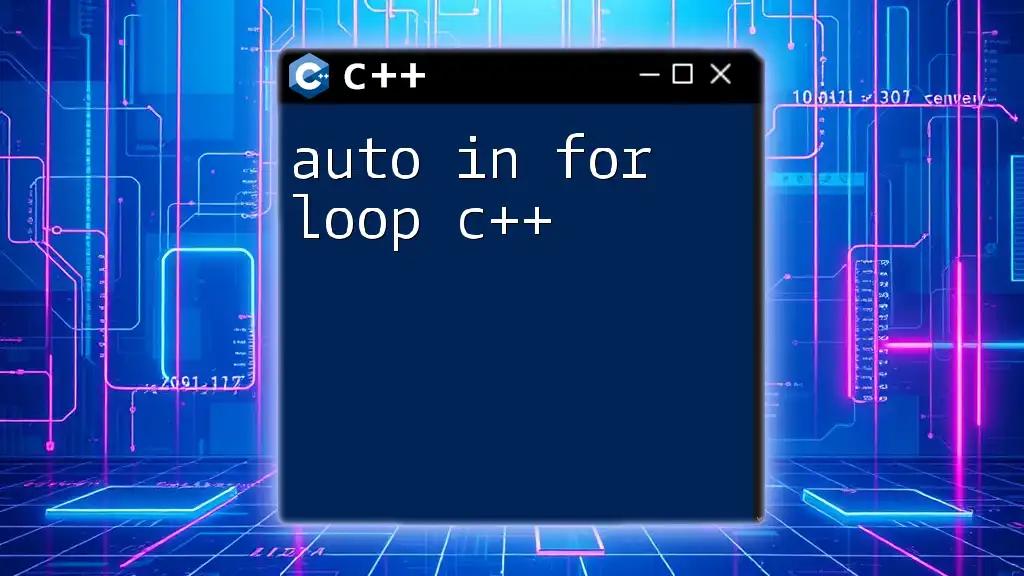
Identifying an Infinite Loop
Characteristics of an Infinite Loop in C++
Infinite loops often exhibit specific traits, including:
- Continuous execution: The code within the loop runs without any termination point.
- Resource consumption: An infinite loop can cause strain on the CPU, leading to high resource usage.
- Lack of observable changes: If the program behaves abnormally without yielding control, it might indicate a loop is running infinitely.
Debugging Infinite Loops
To effectively debug an infinite loop, programmers should:
- Use debugging tools: Modern IDEs provide built-in tools that allow stepping through code line by line to monitor execution.
- Introduce breakpoints: Setting breakpoints within the loop can help isolate the issue.
- Implement logging: Adding logs to track loop progress can assist in identifying where the expected exit condition might fail.
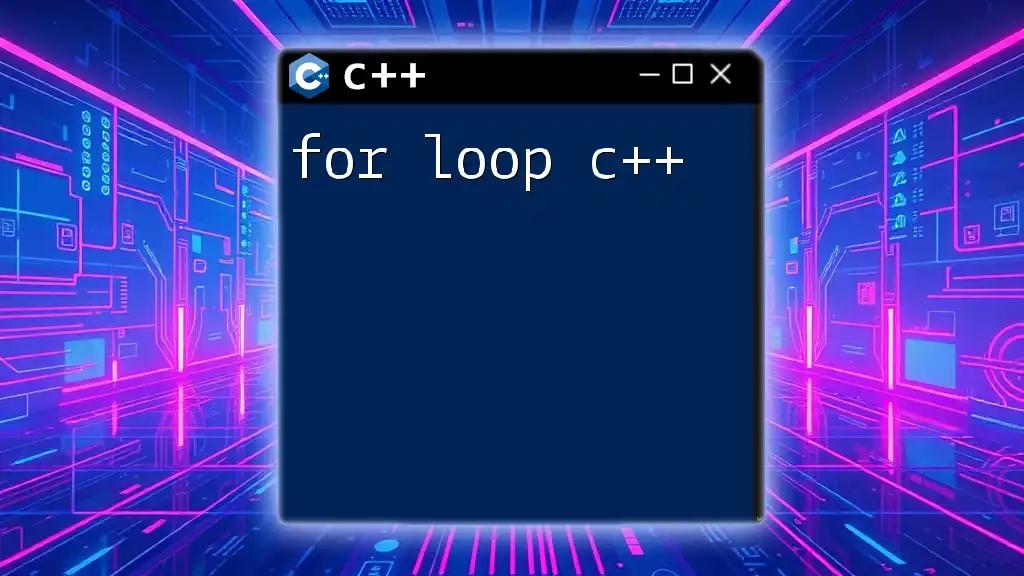
Best Practices for Using Infinite Loops
When to Use Infinite Loops
While infinite loops can be powerful, they should only be utilized when appropriate. Ideal scenarios include:
- Situations where tasks must be performed repeatedly without a predetermined endpoint, such as listening for user input or handling network connections.
- Cases where you want the program to remain active until a shutdown command is received.
Enhancing Control within Infinite Loops
To ensure that infinite loops can be exited gracefully, it is essential to implement control conditions. Utilizing a break statement allows for a clean exit from the loop under certain circumstances. Here’s an example:
for (;;) {
// Input logic to break out of the loop
if (userInput == "exit") {
break;
}
}
In this example, the loop continues indefinitely until the user inputs "exit," prompting the `break` statement to terminate the loop.
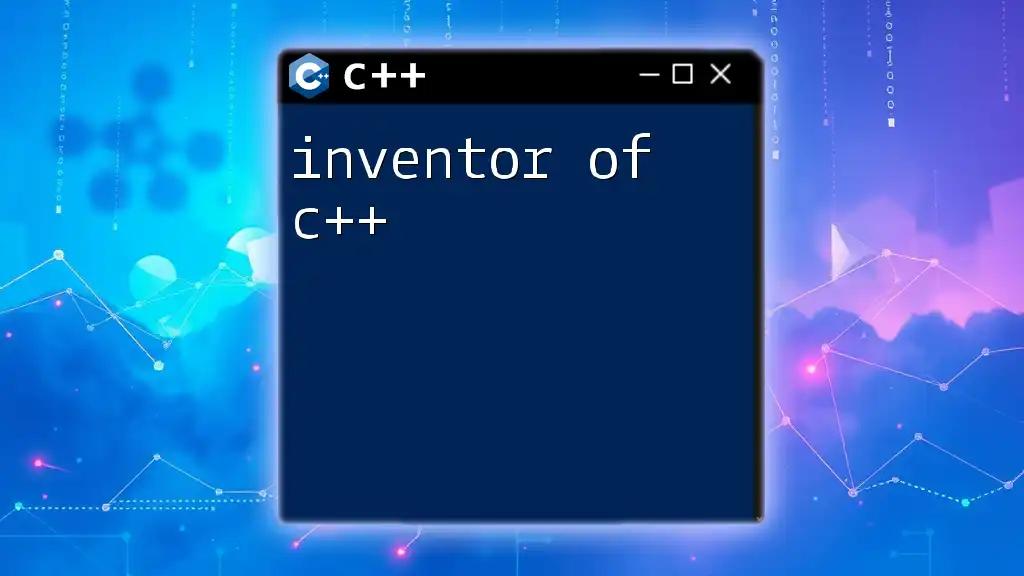
Performance Considerations
Resource Management in Infinite Loops
Infinite loops can impose a significant load on system resources. It is vital to manage CPU and memory usage effectively to avoid performance degradation. Regularly monitor resource allocation and utilize techniques like sleeping to mitigate excessive CPU utilization. For example:
for (;;) {
std::cout << "Running... \n";
// Optional sleep to prevent CPU hogging
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
In the code above, the inclusion of a sleep statement allows the CPU to handle other processes, preventing the infinite loop from consuming all available resources.
Monitoring Infinite Loops
Consider employing tools like profilers or performance monitoring software to keep an eye on the resources consumed by your infinite loops. Regular monitoring can help catch issues before they escalate into major problems.

Common Mistakes When Implementing Infinite Loops
Overlooking Exit Conditions
A common mistake developers make is overlooking or failing to implement exit conditions. An infinite loop without an exit point can lead to unresponsive applications or even crashes. Make sure to consistently evaluate whether your loops require a condition to exit based on application logic.
The Risk of Infinite Recursion
Infinite loops can sometimes be confused with infinite recursion, where a function calls itself without a termination condition. This can lead to a stack overflow. To avoid this, always ensure that recursive calls have well-defined base cases.

Conclusion
In conclusion, the infinite for loop in C++ serves as a powerful tool in programming when utilized correctly. By understanding its structure, real-world applications, and best practices, you can leverage infinite loops without falling into common pitfalls. Effective management and debugging strategies will empower you to maintain robust and high-performing C++ applications that fully utilize the advantages of infinite loops. For further growth in mastering C++ concepts, consider exploring additional resources available from dedicated learning platforms that specialize in programming education.