The invention of C++ by Bjarne Stroustrup in the early 1980s revolutionized programming by introducing object-oriented features to the C programming language, enhancing code reusability and efficiency.
Here’s a simple code snippet in C++ that demonstrates a basic class and object:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
Overview of C++
C++ is a powerful programming language that has shaped the way we approach software development today. It stands out for its combination of low-level manipulation capabilities inherited from C and the object-oriented principles that allow developers to create complex systems with ease. From systems programming to game development, C++ plays a vital role across various fields, making its invention a landmark event in the history of computing.
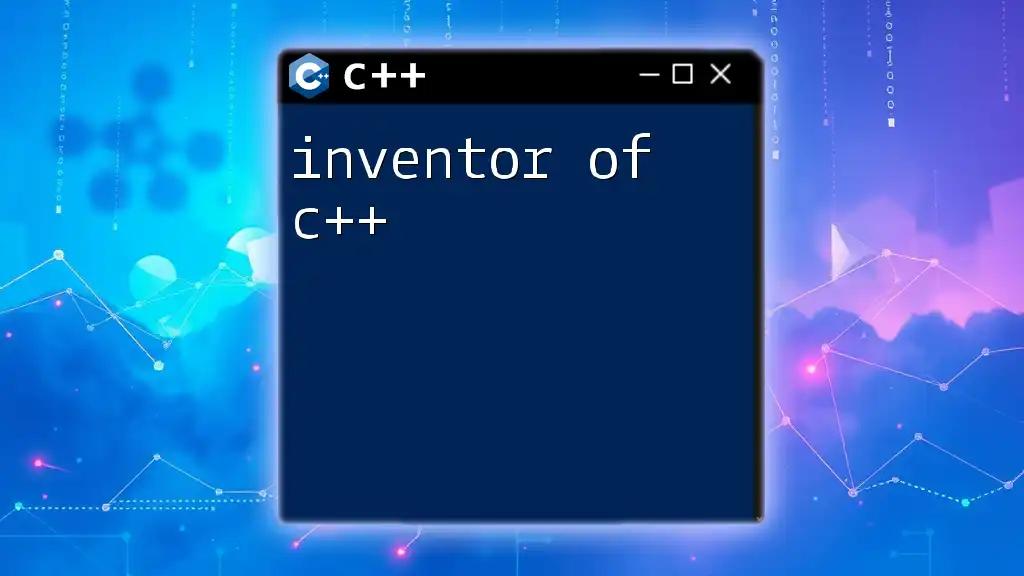
History of C++
Background of Programming Languages
Before delving into the invention of C++, it’s essential to understand the context in which it was developed. Early programming languages like Assembly, Fortran, and ALGOL laid the groundwork for modern programming. These languages were primarily procedural, focusing on the linear execution of commands, which presented limitations in managing complex software systems.
The introduction of object-oriented programming (OOP) in languages such as Simula and Smalltalk marked a pivotal shift towards organizing code around data rather than actions. This transition emphasized concepts like encapsulation, inheritance, and polymorphism, which became foundational principles for later languages, especially C++.
Creator of C++
Bjarne Stroustrup, a Danish computer scientist, initiated the development of C++ at Bell Labs in the late 1970s. His vision was to enhance the C programming language by introducing OOP features while maintaining the efficiency and performance that C is known for. Stroustrup’s efforts were driven by a desire to overcome the inherent limitations of C, aiming to create a language that allowed for both high-level programming and low-level system access.

The Motivation Behind C++
Need for Object-Oriented Features
The invention of C++ was primarily motivated by the need for a language that could handle growing complexities in software design. While C was widely appreciated for its speed, it lacked object-oriented capabilities that could aid in managing large applications. Bjarne Stroustrup aimed to merge the clarity and conciseness of high-level programming with the power and efficiency of C.
Combining Efficiency with Abstraction
One of the core motivations behind C++ was to enable developers to write efficient code while abstracting away unnecessary details. C++ brought the best of both worlds:
- Efficiency: Direct memory manipulation and system-level programming capabilities.
- Abstraction: The introduction of classes and objects facilitated better organization and understanding of code.
This duality contributed to C++’s popularity in system programming, where resource management is critical.
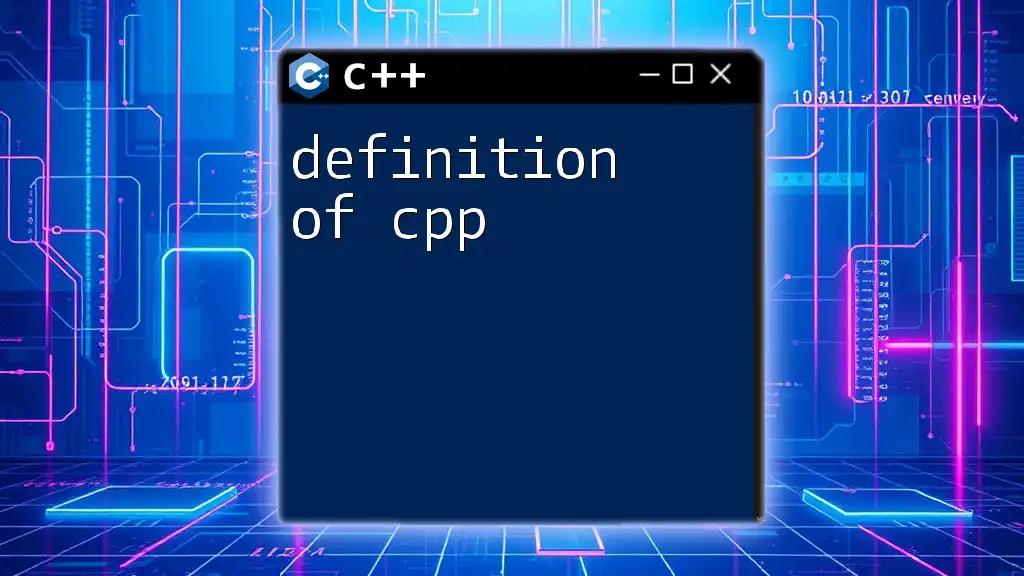
Development of C++
The Evolution Timeline
The journey of C++ began with a prototype known as C with Classes, which combined the features of C with the emerging principles of OOP. After several iterations, C++ was officially released in 1985. The language has undergone significant updates over the years:
- C++98: Standardized the core features and introduced the Standard Template Library (STL).
- C++03: Addressed issues and bugs identified in C++98.
- C++11: A major overhaul with features like auto keyword, nullptr, and lambda expressions.
- C++14, C++17, and C++20: Continued to evolve the language with enhancements for safer and more efficient programming, such as smart pointers and structured bindings.
Key Features Introduced Over Time
Each version of C++ brought forth essential features that contributed to its versatility and robustness:
-
Basic OOP concepts: C++ introduced the concept of classes and objects, allowing developers to encapsulate data and methods.
Example of a simple class in C++:
class Dog { public: void bark() { std::cout << "Woof!" << std::endl; } };
-
Standard Template Library (STL): STL made it easier to handle data structures and algorithms, promoting code reusability. It includes a collection of template classes like vectors, lists, maps, etc.
-
Modern C++ features: C++11 and beyond introduced lambda expressions for inline functions, smart pointers for automatic resource management, and type inference using the auto keyword, which simplified code writing.
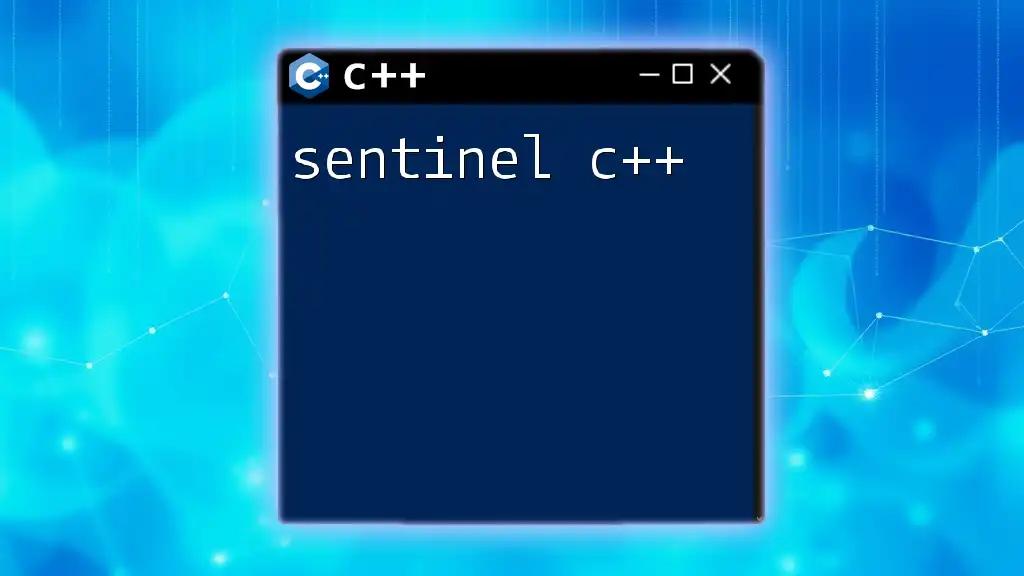
Core Principles of C++
Object-Oriented Programming
Object-oriented programming is at the heart of C++. It allows developers to model real-world entities as objects, enabling a cleaner code structure. Classes serve as blueprints for objects, defining properties and behaviors.
For example, let’s look at a class representing an Animal:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
In this way, C++ provides a framework for encapsulation, ensuring that the implementation details remain hidden from users, thereby reducing complexity.
Encapsulation, Inheritance, and Polymorphism
These three principles are fundamental to understanding C++'s design philosophy:
-
Encapsulation: This principle is about bundling data and methods together and restricting access to some components. It protects the internal state of objects.
-
Inheritance: C++ allows classes to inherit properties and behaviors from other classes. This promotes code reuse and logical hierarchy. For instance:
class Cat : public Animal { public: void meow() { std::cout << "Meow!" << std::endl; } };
-
Polymorphism: This allows methods to do different things based on the object it is acting upon, enabling dynamic behavior. Function overloading and virtual functions are examples of this concept.
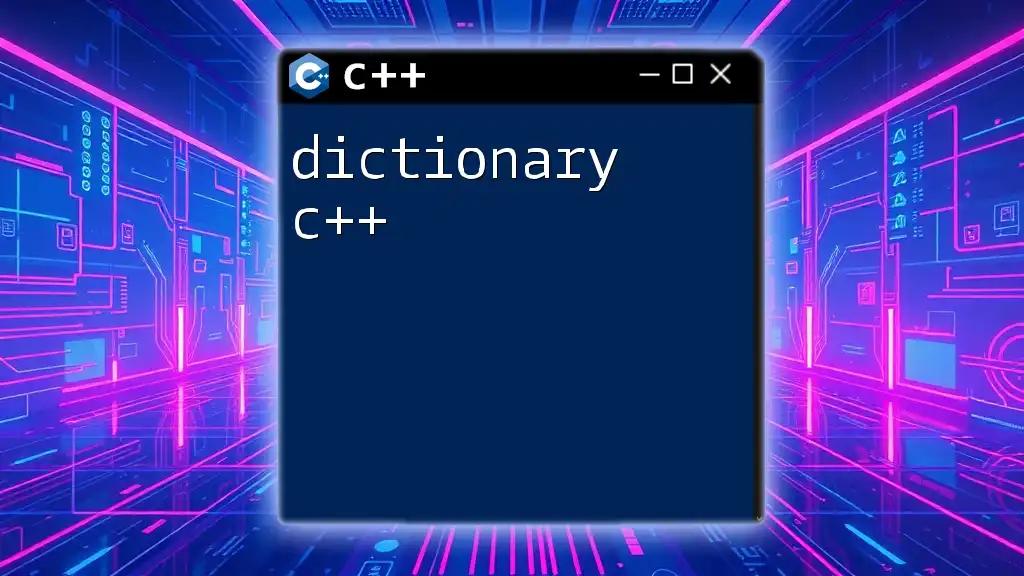
Impact of C++
C++ in Industry
Since its invention, C++ has been widely adopted in various industries due to its performance and capabilities. It is particularly favored for:
- Game development: Many game engines use C++ due to its efficiency and control over system resources.
- Real-time systems: Applications requiring high performance, like trading systems and avionics, benefit from C++’s deterministic nature.
- Simulations: C++ is used in scientific computing and simulations, where both computation speed and accuracy are crucial.
Prominent companies such as Microsoft, Adobe, and Google have leveraged C++ in their products—demonstrating its continued relevance in the tech landscape.
C++ in Academia
C++ remains a primary language taught in computer science programs due to its foundational principles in programming and its performance capabilities. It reinforces concepts of memory management, data structures, and software design. Educators emphasize its importance in understanding both classic programming techniques and modern software practices.
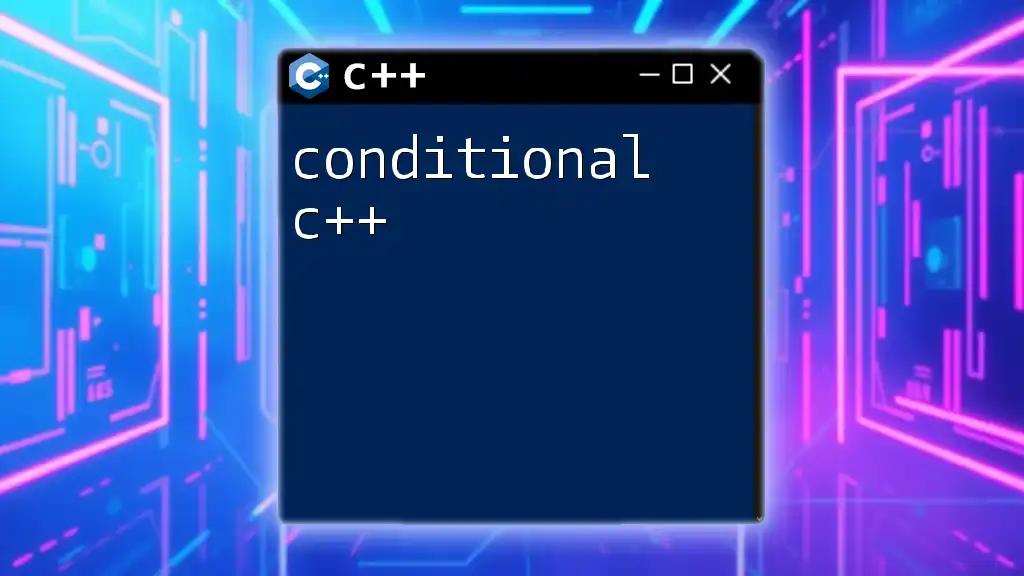
The C++ Community and Standards
Role of ISO/IEC in C++ Standardization
The invention of C++ set the stage for its journey towards standardization by the International Organization for Standardization (ISO). The standardization ensures that C++ remains consistent across platforms while enabling developers to implement best practices.
Through community contributions via the International C++ Standards Committee (WG21), C++ is continually refined and updated. This collaborative effort helps maintain its relevance in the ever-evolving landscape of software development.
Resources for C++ Developers
Aspiring C++ developers have access to a wealth of resources:
- Books: Titles like "The C++ Programming Language" by Bjarne Stroustrup and "Effective C++" by Scott Meyers provide in-depth knowledge.
- Online Courses: Platforms like Coursera and Udemy offer structured courses for learners at all levels.
- Community Forums: Websites like Stack Overflow and Reddit allow developers to seek help and share knowledge.
Developers are encouraged to follow best practices, participate in discussions, and contribute to open-source projects to further enhance their skills.
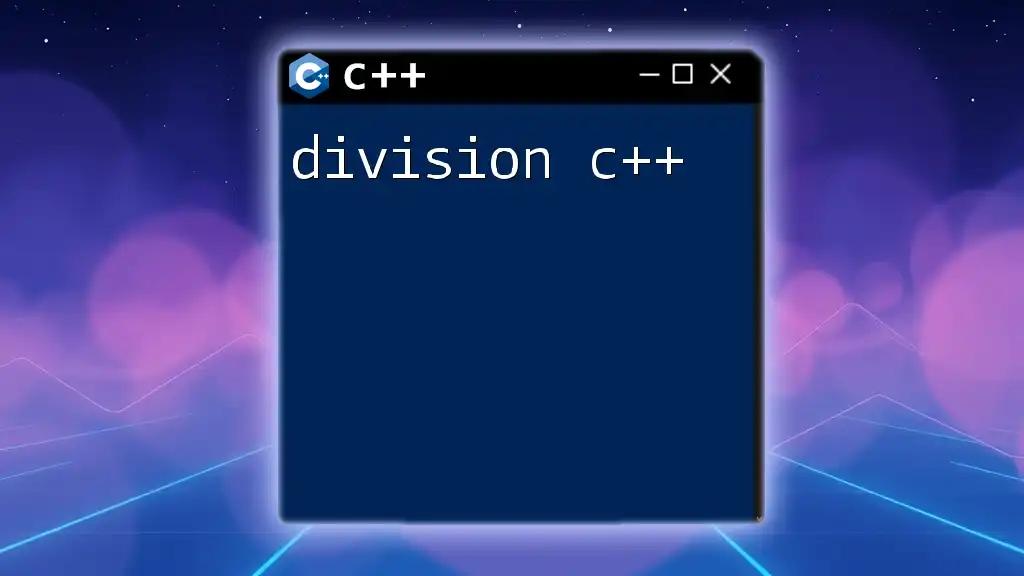
Conclusion
The invention of C++ ushered in a new era of programming that integrated the efficiency of procedural languages with the flexibility of object-oriented design. Its ongoing evolution and widespread adoption solidify its place as one of the most powerful and versatile programming languages available today. As developers, embracing C++ offers an opportunity to engage with a rich legacy while paving the way for future innovations in software engineering.
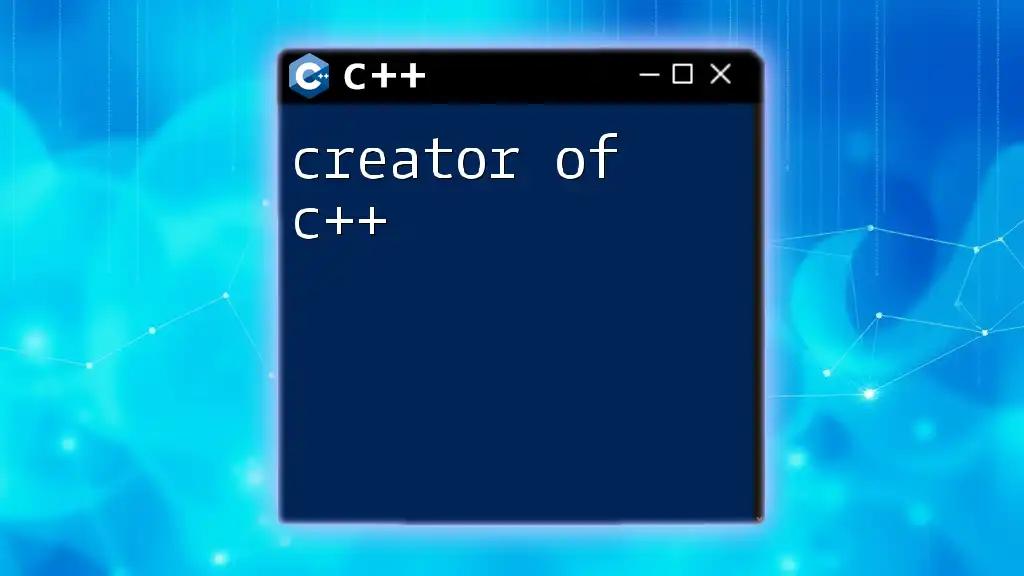
Call to Action
If you're eager to learn C++ and enhance your programming skills, join our community for quick, concise lessons that cater to your needs. Subscribe for updates, tutorials, and resources that will help you master C++ effortlessly!
Additional Resources
For further reading and in-depth understanding, here are some recommended resources:
- Books, articles, and online courses that delve deeper into C++ programming.
- Access to forums and communities that facilitate learning and knowledge sharing among developers interested in C++.