In C++, addition can be performed using the `+` operator to sum two numbers, as demonstrated in the following example:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 3;
int sum = a + b;
cout << "The sum is: " << sum << endl;
return 0;
}
Basics of C++ Addition
Understanding Data Types
In C++, the concept of addition is tied closely to the data types being used. The primary data types that come into play during addition are:
- Integers: Whole numbers without decimal points.
- Floating-Point Numbers: Numbers that contain decimal points (e.g., `float`, `double`).
- Characters: While not typically added in traditional contexts, characters can be manipulated as integers in ASCII.
When you perform operations involving different data types, C++ automatically applies type promotion. For instance, if you add an integer to a floating-point number, the integer will be promoted to a floating-point number. This is essential for avoiding data loss and ensuring precision.
C++ Addition Operators
The addition operation in C++ mainly involves the plus operator (+). This operator can be used in various contexts and is quite versatile:
-
Basic Usage: The simplest form of addition.
-
Operators in Expressions: Addition can be a fundamental part of larger expressions. For example:
int main() {
int a = 5;
int b = 10;
int sum = a + b; // sum now holds the value 15
return 0;
}
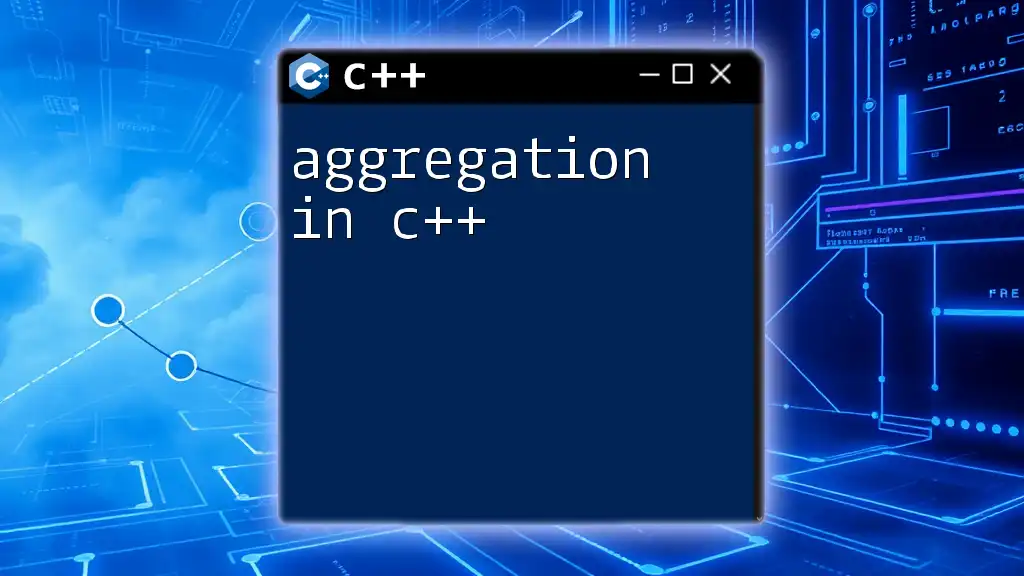
How to Add in C++
Simple Addition Examples
Adding numbers in C++ is straightforward. Let's consider a few examples:
Adding Integers:
int main() {
int a = 5;
int b = 10;
int sum = a + b; // sum is 15
return 0;
}
Adding Floating-Point Numbers:
int main() {
float x = 3.5f;
float y = 2.5f;
float total = x + y; // total is 6.0
return 0;
}
Adding Different Data Types:
It's important to understand how type conversion works when adding different types. For example:
int m = 2;
float n = 10.5f;
float result = m + n; // result is 12.5, int m is promoted to float
Addition in Expressions
In C++, you can perform addition as part of more complex expressions. The following illustrates:
Combining Multiple Additions:
int a = 1;
int b = 2;
int c = 3;
int result = a + b + c; // result is 6
Using Parentheses for Clarity:
When dealing with multiple operations, parentheses can help clarify the order of execution:
int result = (a + b) * c; // Ensure a + b is calculated first
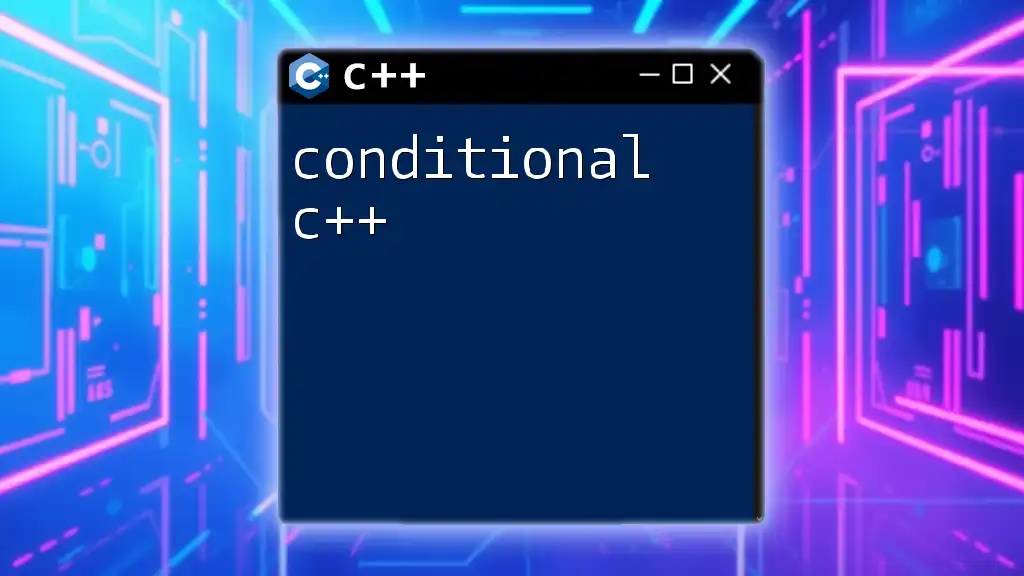
C++ Add: Beyond Simple Addition
Using Functions for Addition
Creating functions to handle addition operations is a common practice in C++. This approach promotes reusability and clean code. Here is an example of a simple addition function:
int add(int x, int y) {
return x + y;
}
You can also take advantage of function overloading to handle different data types:
float add(float x, float y) {
return x + y;
}
Adding Arrays and Vectors
Adding arrays or collections in C++ requires a bit more thought but offers powerful functionality.
Element-wise Addition of Arrays:
int arr1[] = {1, 2, 3};
int arr2[] = {4, 5, 6};
int result[3];
for (int i = 0; i < 3; i++) {
result[i] = arr1[i] + arr2[i]; // Resultant array holds {5, 7, 9}
}
Using `std::vector` for Addition:
Vectors in C++ make handling dynamic arrays easier. Here’s how to perform element-wise addition using vectors:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> result;
for (size_t i = 0; i < vec1.size(); i++) {
result.push_back(vec1[i] + vec2[i]); // Add elements
}
// Output result
for (int res : result) {
std::cout << res << " "; // Outputs: 5 7 9
}
return 0;
}
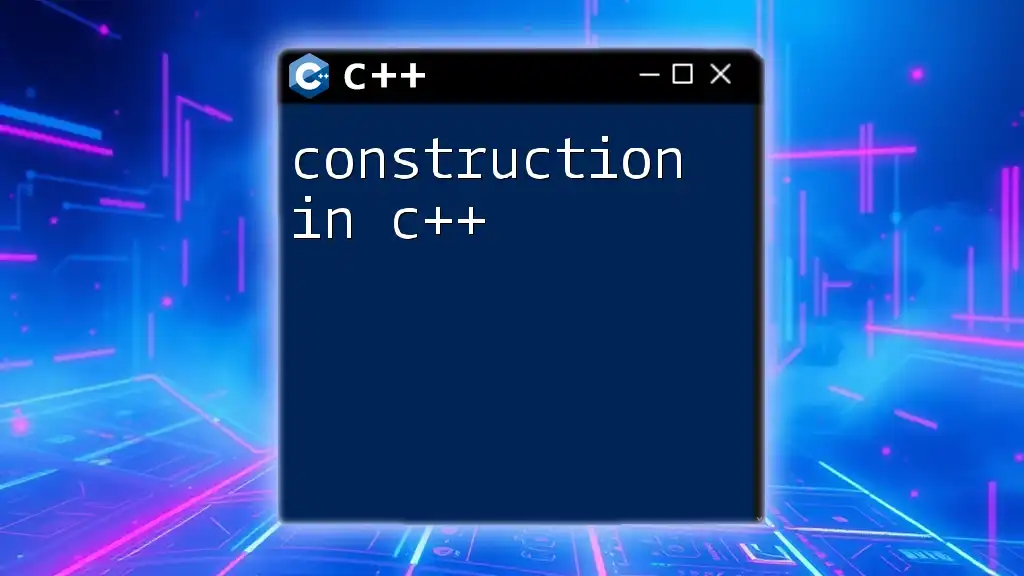
Common Pitfalls in C++ Addition
Overflow and Underflow
When performing addition, especially with integers, you must be cautious of overflow. Adding numbers beyond the maximum limit can lead to unexpected behaviors. For example:
#include <iostream>
#include <limits>
int main() {
int largeNumber = std::numeric_limits<int>::max();
largeNumber = largeNumber + 1; // This causes overflow!
std::cout << largeNumber; // Undefined behavior
return 0;
}
Type Casting and Conversion Issues
In addition operations, implicit and explicit conversions often come into play. Understanding these can save you from prominent errors:
int a = 3;
float b = 2.5f;
float result = a + b; // Implicit conversion, safe
However, it's crucial to be vigilant about potential data loss, especially when downcasting or mixing types.
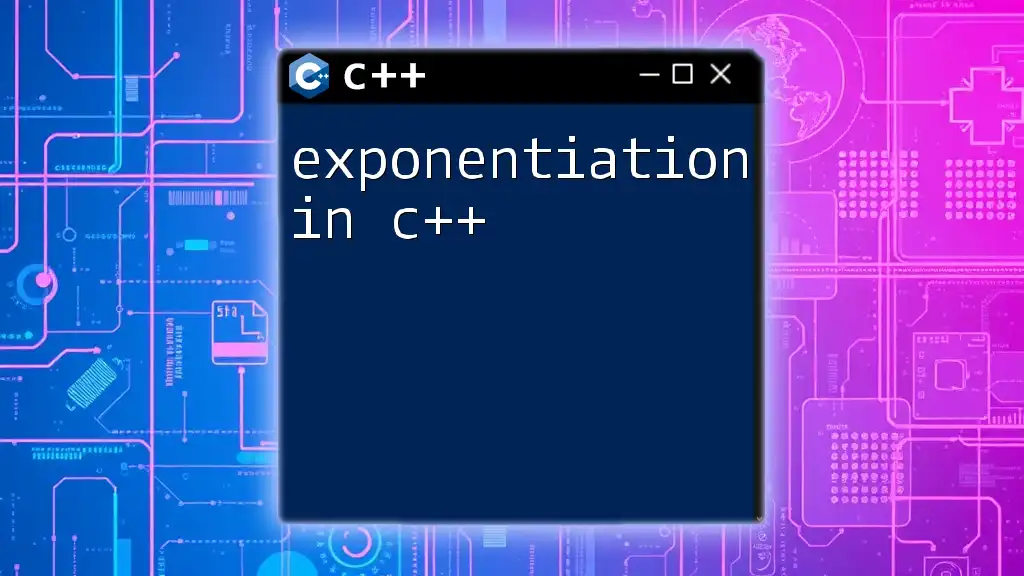
Best Practices for Performing Addition in C++
When working with addition in C++, consider the following best practices:
- Choosing the Right Data Type: Understand the limits of each type to prevent overflow.
- Using Functions for Reusability: Grouping addition logic into functions can improve code clarity and maintenance.
- Handling Edge Cases: Always be mindful of scenarios that could lead to overflow or unexpected behavior and handle them gracefully.
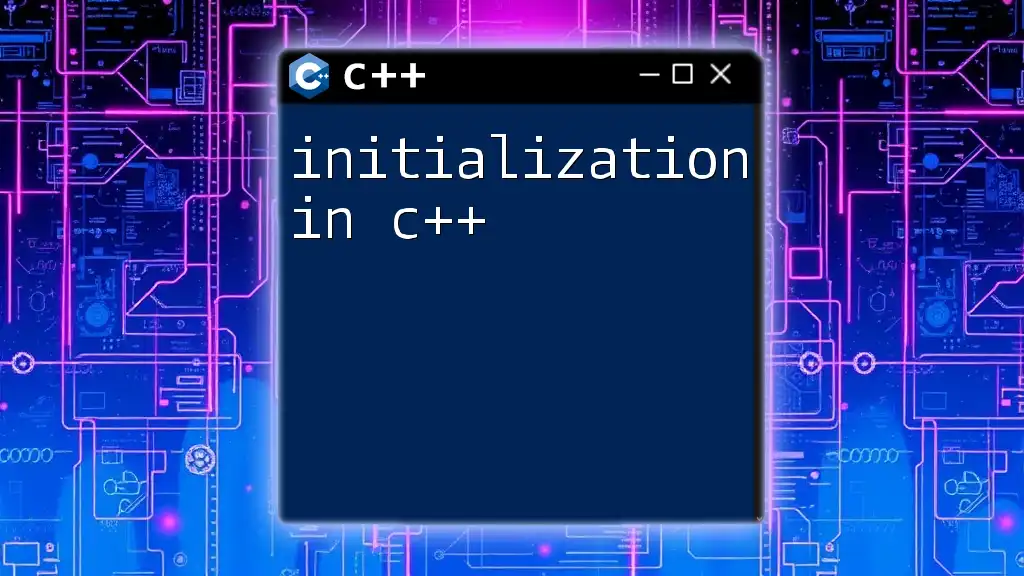
Conclusion
In summary, addition in C++ is a fundamental operation that extends well beyond simple arithmetic. Understanding data types, operators, and the implications of different methods—such as using functions, arrays, and vectors—will empower you to use addition effectively in your C++ programs. As you experiment with addition and its nuances, you will become more adept at leveraging its potential in various programming scenarios.
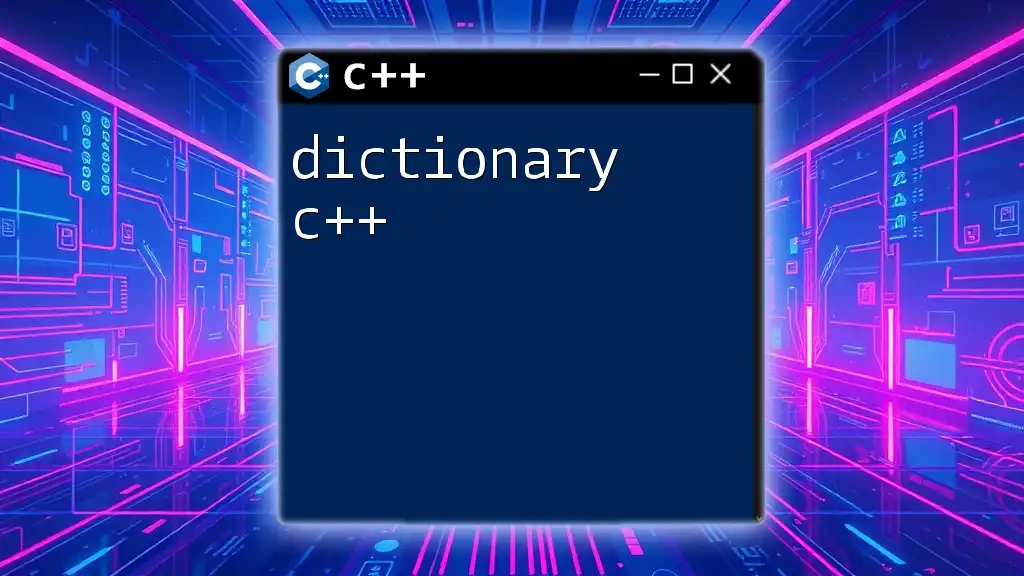
Additional Resources
For those looking to enhance their understanding of C++, various resources such as recommended books and online coding platforms can provide further opportunities to practice and master addition and other topics in the language.