In C++, `and` and `or` are alternative representations of the logical operators `&&` (logical AND) and `||` (logical OR), respectively, which can be used to combine boolean expressions.
Here’s a simple example using both:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a and b) {
std::cout << "Both are true" << std::endl;
} else if (a or b) {
std::cout << "At least one is true" << std::endl;
} else {
std::cout << "Neither are true" << std::endl;
}
return 0;
}
What are Logical Operators?
Definition of Logical Operators
Logical operators in programming are used to perform logical operations on boolean values. They evaluate expressions and return either `true` or `false`. Unlike arithmetic operators, which manipulate numerical values, logical operators determine the truthiness of conditions, making them essential for control flow in programming.
Importance of Logical Operators
Logical operators play a critical role in decision-making within your code. They allow programmers to create complex conditions that guide how a program responds in different situations, such as controlling the execution of statements and loops based on multiple criteria. In C++, the two primary logical operators are 'AND' (`&&`) and 'OR' (`||`).
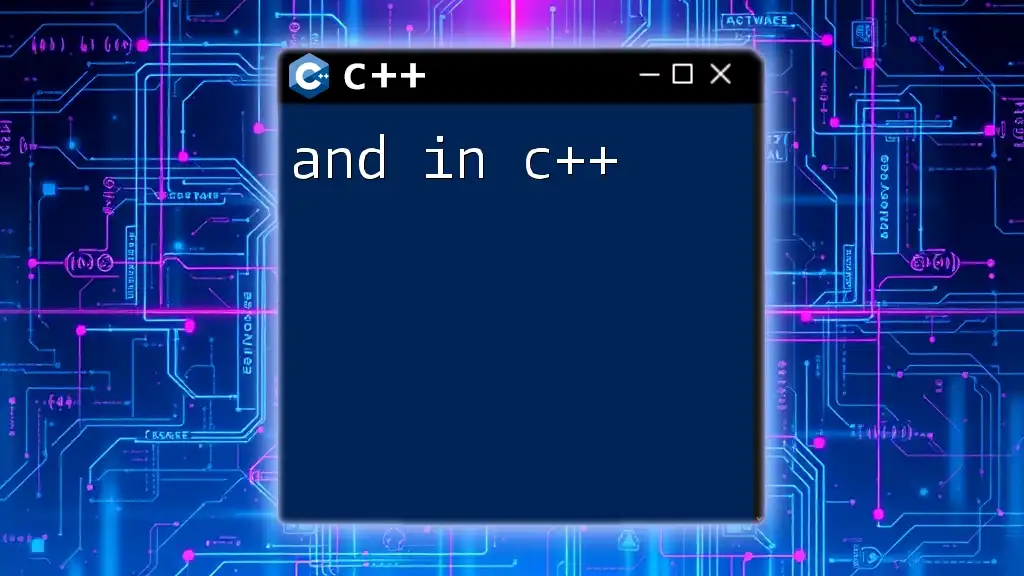
The 'AND' Operator in C++
Definition and Syntax
The 'AND' operator in C++, represented by `&&`, is used to combine two boolean expressions. It evaluates to `true` only if both operands are `true`. The syntax can be expressed as follows:
condition1 && condition2
How the 'AND' Operator Works
To better understand how the 'AND' operator functions, consider the following truth table:
Condition 1 | Condition 2 | Result |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
This table illustrates that the result is only `true` when both conditions are `true`.
Practical Example of 'AND' Operator
Here is an example using the 'AND' operator in a simple C++ program:
#include <iostream>
using namespace std;
int main() {
int age = 25;
bool hasLicense = true;
if (age >= 18 && hasLicense) {
cout << "You are eligible to drive." << endl;
} else {
cout << "You are not eligible to drive." << endl;
}
return 0;
}
In this example, the program checks both the age and the possession of a license. If both conditions are satisfied, the user receives a message indicating eligibility to drive, demonstrating the utility of the 'AND' operator.
Common Use Cases
The 'AND' operator is particularly useful in scenarios where multiple conditions must be met:
- User Authentication: Validating multiple login credentials.
- Input Validation: Ensuring multiple fields are filled before processing form data.
- Complex Game Logic: Including multiple criteria for game rules—for instance, when a player qualifies for an award only if they achieve a certain score AND complete specific tasks.
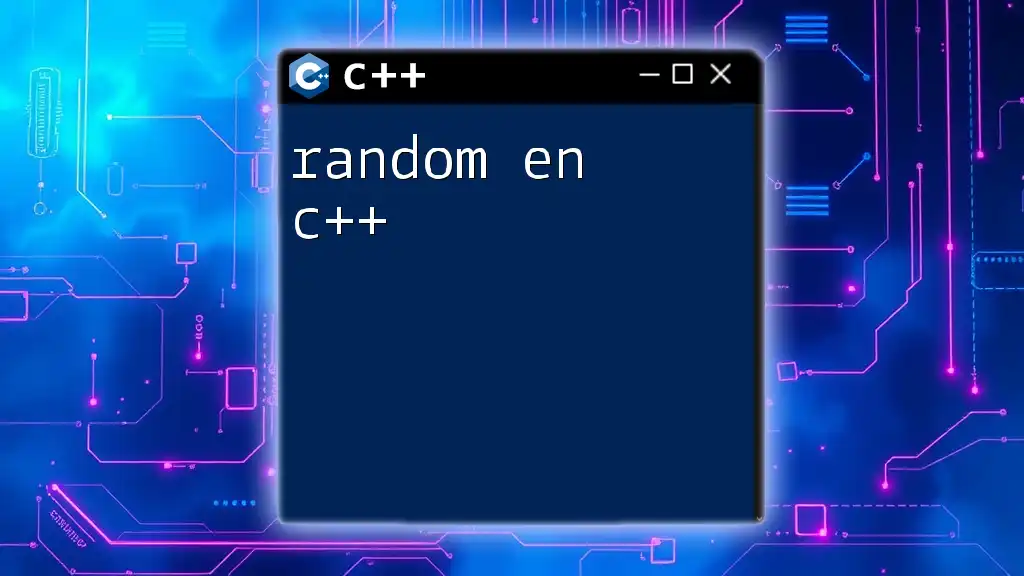
The 'OR' Operator in C++
Definition and Syntax
The 'OR' operator, denoted by `||`, is used to combine two boolean expressions where the result evaluates to `true` if at least one of the operands is `true`. The basic syntax is as follows:
condition1 || condition2
How the 'OR' Operator Works
Understanding how the 'OR' operator evaluates conditions can be visualized through the following truth table:
Condition 1 | Condition 2 | Result |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
This table shows that the result is `true` if at least one of the conditions is true.
Practical Example of 'OR' Operator
Here’s a practical example of 'OR' in a simple C++ program:
#include <iostream>
using namespace std;
int main() {
int age = 16;
bool hasConsent = true;
if (age >= 18 || hasConsent) {
cout << "You can join the event." << endl;
} else {
cout << "You cannot join the event." << endl;
}
return 0;
}
In this snippet, the program checks if the age is at least 18 or if the user has parental consent. If either condition holds, the participant is allowed to join the event, showcasing the utility of the 'OR' operator in decision-making.
Common Use Cases
Common scenarios for using the 'OR' operator in C++ include:
- Access Control: Granting permissions if a user meets at least one criterion, such as an age minimum or special permissions.
- Input Validation: Allowing alternative inputs to pass validation.
- User Experiences: Providing flexible options to achieve goals or complete processes in software applications.
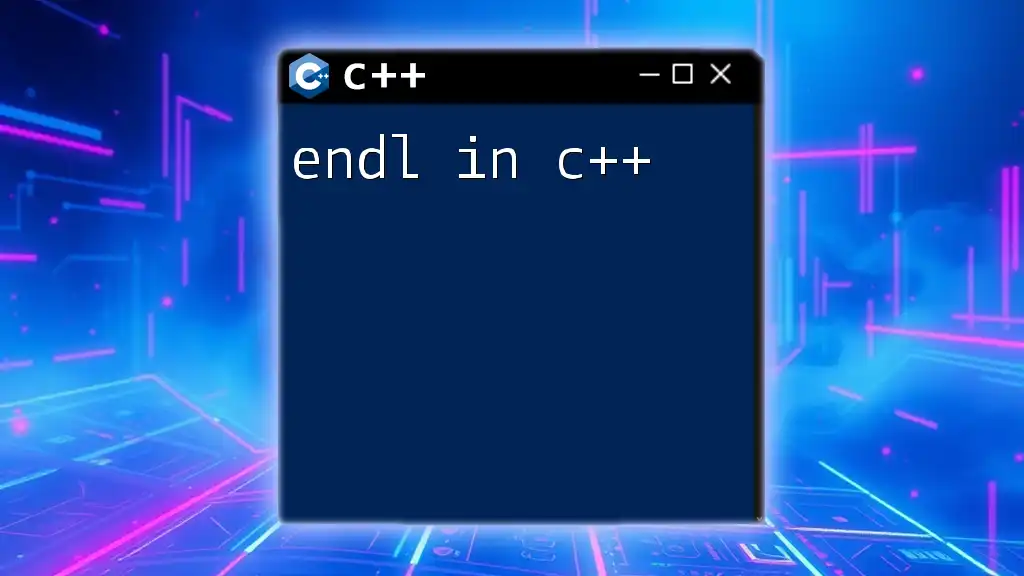
Combining 'AND' and 'OR' Operators
Understanding Operator Precedence
When using logical operators in C++, it’s crucial to understand their precedence—`&&` has a higher precedence than `||`. This means that in an expression where both operators are present, the 'AND' conditions will be evaluated before the 'OR' conditions. To ensure clarity and correctness, it is often a good practice to use parentheses.
Practical Example: Combining Both Operators
To illustrate the combined use of both operators, consider the following code snippet:
#include <iostream>
using namespace std;
int main() {
int age = 20;
bool hasLicense = false;
bool hasConsent = true;
if ((age >= 18 && hasLicense) || (age < 18 && hasConsent)) {
cout << "You can drive." << endl;
} else {
cout << "You cannot drive." << endl;
}
return 0;
}
In this example, the program allows driving under two circumstances: being of age with a license, or being underage but having parental consent. This demonstrates how to effectively combine both 'AND' and 'OR' operators in decision-making logic.
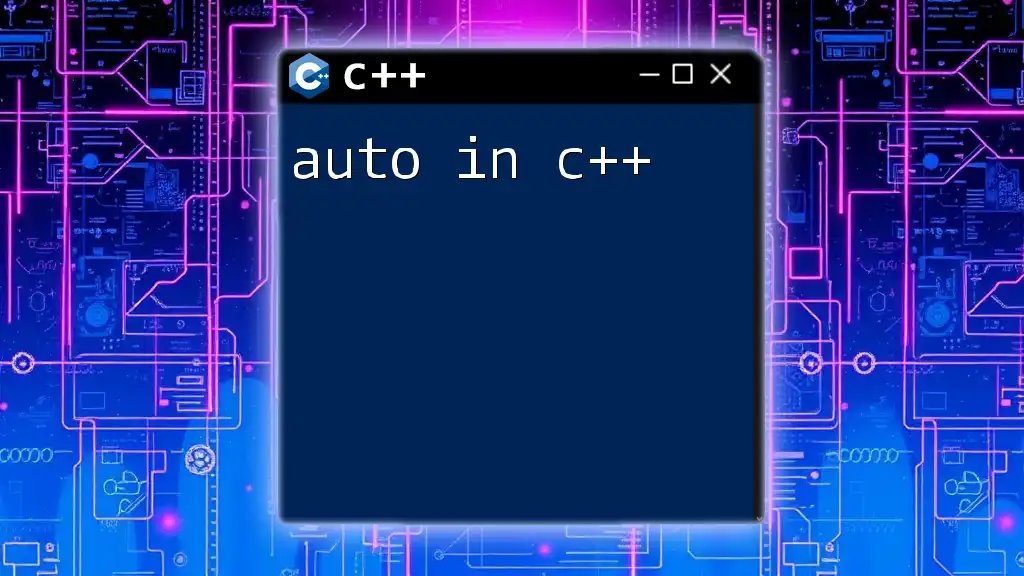
Best Practices for Using 'AND' and 'OR' Operators
Writing Clear and Readable Code
When using logical operators, clarity is paramount. Establish clear variable names that describe their purpose and ensure conditions are readable. Returning to the previous example, it’s better to use descriptive conditions rather than vague or cryptic meanings.
Avoiding Common Mistakes
Common pitfalls when using logical operators include:
- Operator Precedence Errors: Misunderstanding which operator evaluates first can lead to unexpected results. Always use parentheses to explicitly denote the desired evaluation order.
- Short-circuit Evaluation: C++ employs short-circuit evaluation which stops evaluating further conditions when the outcome is already known. Understand when this applies, particularly in the context of function calls within conditions that might have side effects.
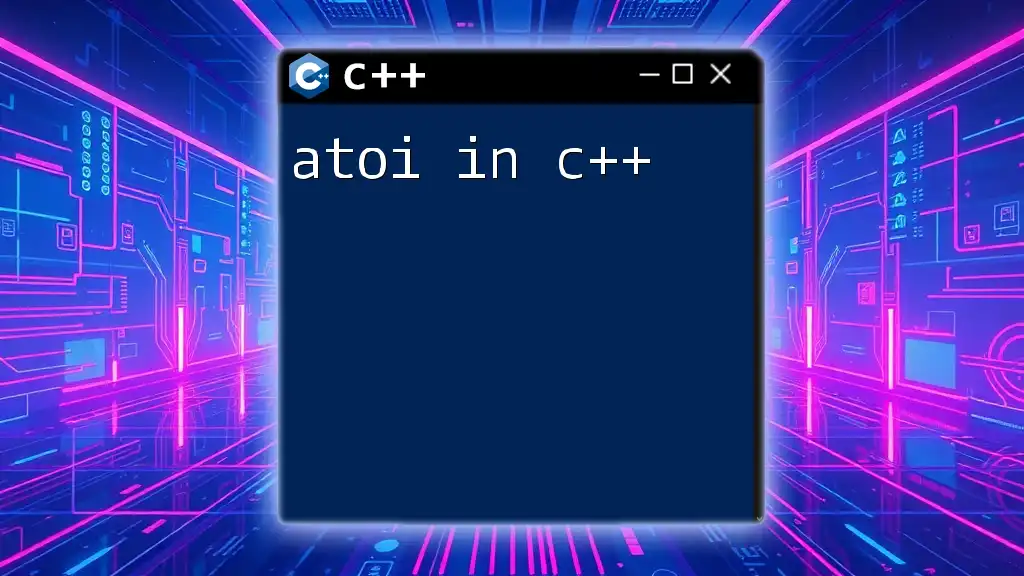
Conclusion
The 'AND' and 'OR' operators are foundational elements of control flow in C++. Their ability to combine conditions enhances the decision-making capabilities of your programs. Understanding how these operators work, their syntax, and their implications is crucial for writing effective and efficient C++ code.
By practicing with these operators in real programming scenarios, you will sharpen your skills and bolster your C++ programming knowledge. Continue learning more about C++ and logical operations to deepen your understanding and improve your coding proficiency!
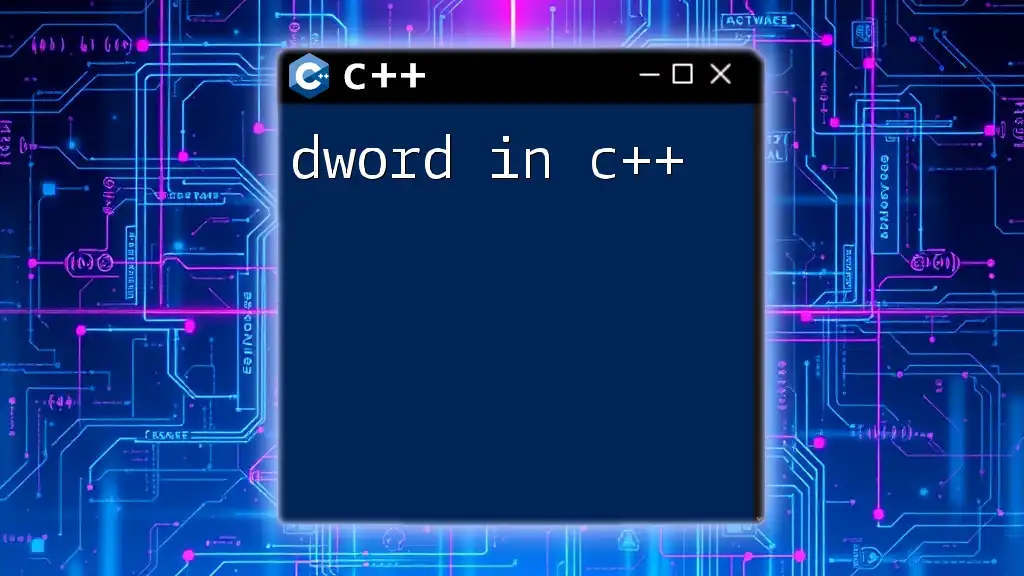
Additional Resources
For those wishing to enhance their knowledge further, consider exploring the official C++ documentation and supplementary tutorials. Books and online courses focused on C++ programming can also provide valuable insights into mastering logical operators and overall coding techniques.