In C++, `meaning` is not a specific keyword or function, but it can refer to the significance or interpretation of various phrases and commands within the language's context; for instance, the keyword `class` is essential for defining a new data type.
Here's a code snippet demonstrating the definition of a class in C++:
class Car {
public:
string model;
int year;
void display() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
What is 'in' in C++?
The term 'in' as used in programming generally refers to the intention of checking membership or containment, such as whether a specific value exists within a collection or data structure. In many programming languages, 'in' is a reserved keyword that allows for operations like membership testing. However, in C++, 'in' is not a keyword and does not possess a designated meaning in the same manner as it does in languages like Python or JavaScript.
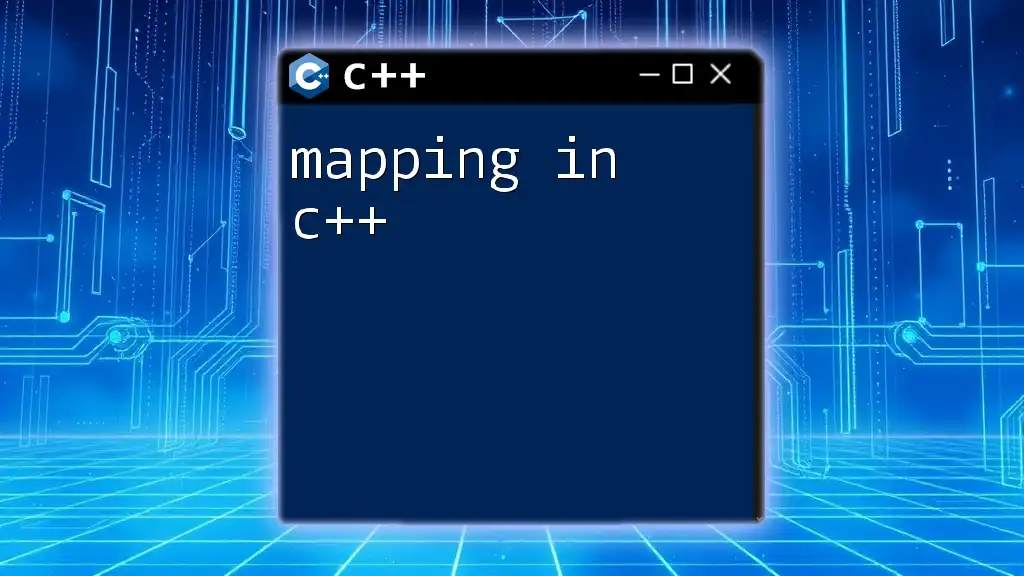
The Concept of Keywords in C++
Keywords are predefined, reserved words in programming languages that have special meanings. In C++, keywords are fundamental building blocks dictated by the C++ syntax, which programmers cannot redefine or use for other purposes. Understanding the distinction between keywords and identifiers is crucial for effective coding practices.
Keywords vs. Identifiers
- Keywords: Reserved words in the language (e.g., if, for, return) that define the language's syntax.
- Identifiers: User-defined names for variables, functions, classes, etc. They can include letters, digits, or underscores but cannot start with a digit.
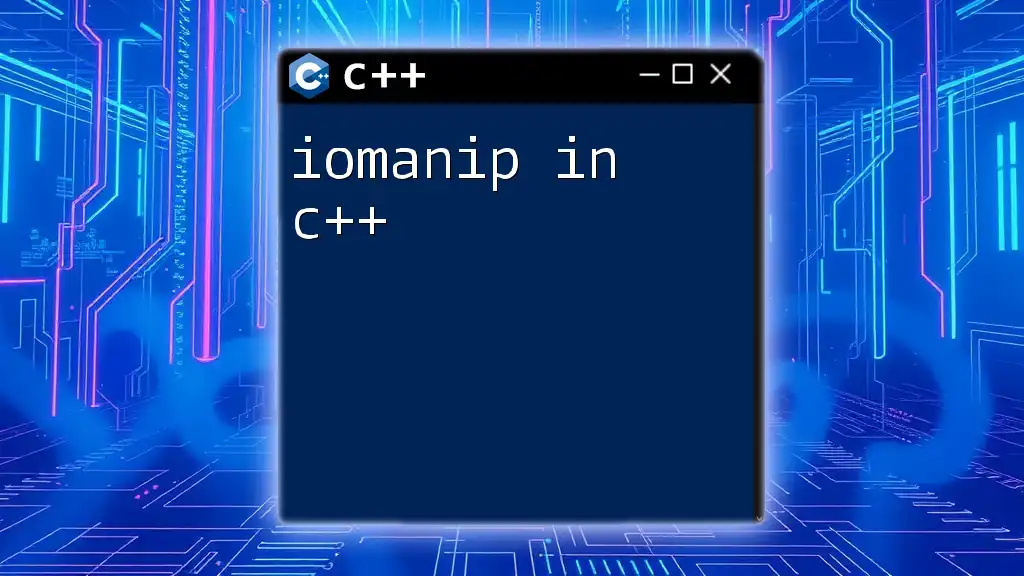
'in' in C++: A Language Overview
Given the previous discussion, it's essential to recognize that 'in' does not exist as a keyword in C++. This sets it apart from its behavior in other programming languages. In languages like Python, 'in' allows for concise membership checks using syntax like `if value in collection`. C++, however, requires different approaches to achieve similar functionality.
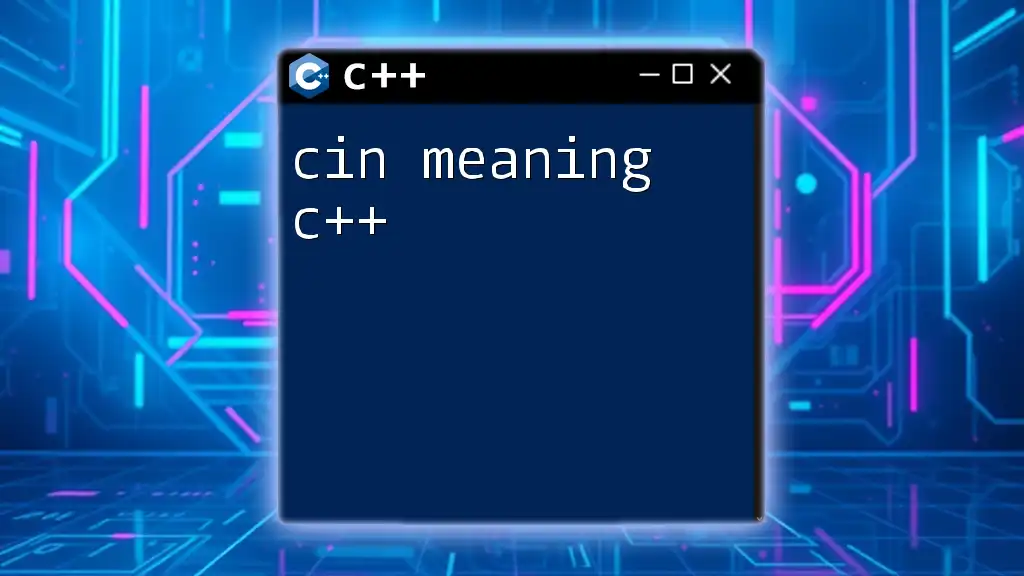
Common Contexts Where 'in' Appears
Iterating through Collections
One of the most common use cases that might involve the conceptual use of 'in' is iterating through collections such as arrays or vectors. In C++, you would typically utilize loops to achieve this.
- Using `for` loops: The traditional for loop looks like this:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
This code iterates through each element of the vector numbers, utilizing an index to access the elements.
- Range-based for loop: The more modern range-based for loop, introduced in C++11, simplifies this process:
for (int num : numbers) {
std::cout << num << " ";
}
In this syntax, the loop reads more clearly and succinctly suggests that num is in the collection of numbers.
The Role of 'in' in Functions
In C++, you often pass parameters to functions either by value or by reference. Understanding the distinction is essential as it impacts performance and function behavior.
- Passing by reference: When passing by reference, you can prevent unnecessary copies of data, making your code more efficient. Here’s an example:
void printVector(const std::vector<int>& vec) {
for (const int& num : vec) {
std::cout << num << " ";
}
}
This method showcases how the vec is utilized within the function without modifying the original vector due to the presence of `const`.
'in' Usage in Object-Oriented Programming
When developing in an Object-Oriented paradigm, the concept of 'in' can relate to how methods interact with class instances. This idea becomes evident when invoking class methods or accessing object states:
class MyClass {
public:
void display() {
std::cout << "Object in action!" << std::endl;
}
};
MyClass obj;
obj.display();
In this example, you can interpret the invocation of `display()` as the object existing "in action". This illustrates the interaction of an object with function calls and states.
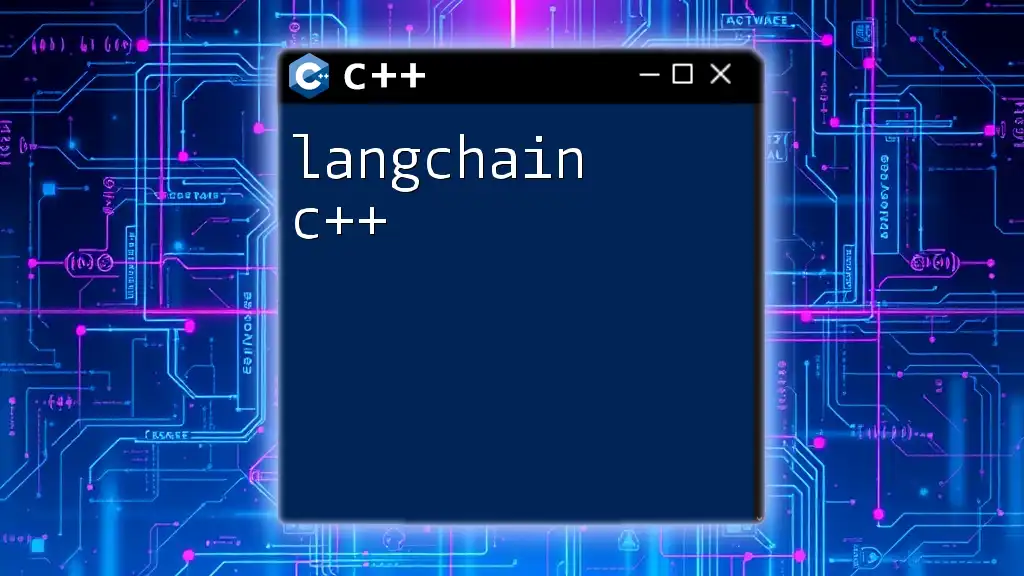
The Misconception of 'in' in C++
It is crucial to clarify that 'in' is not a reserved word in C++. A common misunderstanding arises among new programmers who might be familiar with languages where 'in' is used for iteration or membership tests. Recognizing this distinction helps prevent confusion while writing C++ code.
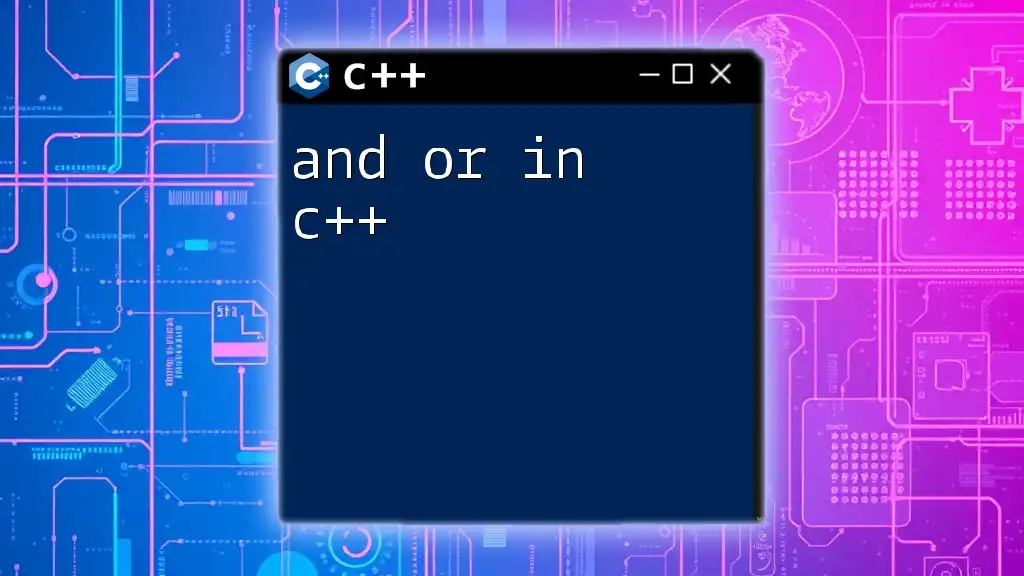
Alternative Keywords and Constructs
To achieve similar functionalities typically associated with 'in', C++ has alternative constructs.
'for' and 'foreach'
C++ provides loop constructs such as 'for' and 'foreach' (in the range-based sense). Understanding these built-in loops eliminates the need for the 'in' keyword.
For example:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
This 'for' loop effectively iterates through the vector similar to how one might think of 'in' being used in other languages.
'contains' and Related Functions
In C++, there are various functions and algorithms available for checking contents within collections. For instance, using the `<algorithm>` header allows you to determine membership effectively:
#include <algorithm>
if (std::find(numbers.begin(), numbers.end(), target_num) != numbers.end()) {
std::cout << "Number found!";
}
Here, we check if target_num is found within the 'numbers' vector, which replicates the conceptual operation one might expect from using 'in' without actually using that word.
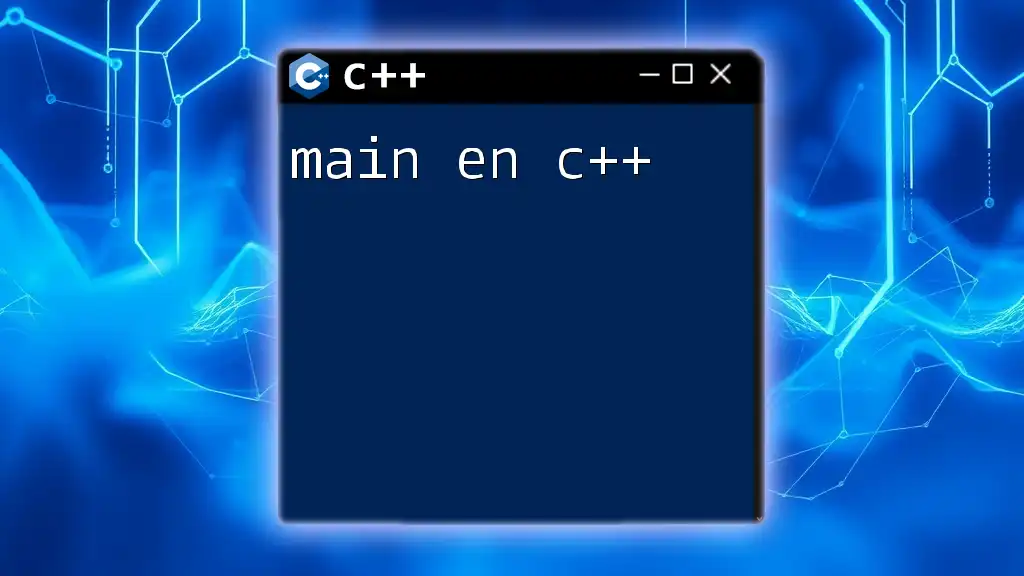
Conclusion
In summary, understanding the meaning of 'in' in C++ requires comprehension of its absence as a keyword. Instead, C++ offers powerful alternatives that facilitate operations resembling membership checks and iteration through collections. By focusing on C++ structures like loops, functions, and algorithms, programmers can achieve their goals effectively without the confusion that may arise from other languages. As you deepen your exploration of C++, remember that experimentation and practice will enhance your knowledge and skills in navigating code efficiently.