The `main` function in C++ is the entry point of any C++ program, where execution begins.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the `main` Function
What is the `main` Function?
The `main` function is the backbone of every C++ program. It acts as the entry point, where program execution begins. Whenever you run a C++ program, the system looks for this function to determine where to start executing the code.
Syntax of the `main` Function
The basic structure of the `main` function is straightforward. The simplest form looks like this:
int main() {
// code goes here
return 0;
}
In this declaration, `int` indicates that the function returns an integer. The function typically returns `0` to signify successful execution, while any non-zero value generally indicates an error.
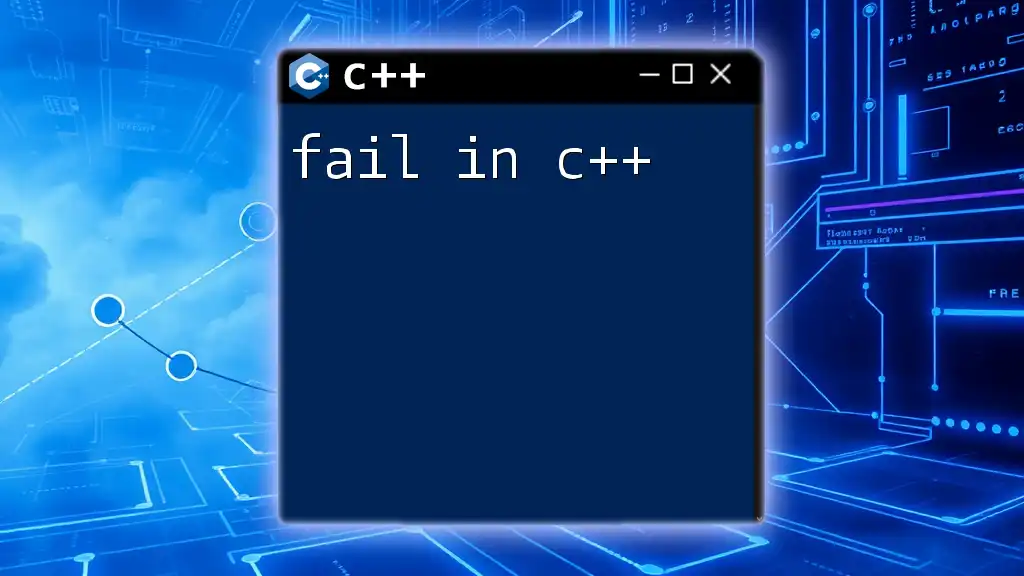
Types of `main` Function Declarations
Standard Declaration
The most common declaration of the `main` function is as follows:
int main() {
// code
}
This basic form allows programmers to define a block of code that will execute upon program startup. Such simplicity makes it easy for newcomers to grasp the basics of C++.
Command-Line Arguments
Understanding `argc` and `argv`
C++ provides the capability to receive input from the command line through the `main` function. This is done via two parameters: `argc` and `argv`.
- `argc` (Argument Count): This represents the number of command-line arguments, including the program's name.
- `argv` (Argument Vector): This is an array of C-string arguments.
Here's an example of its declaration:
int main(int argc, char *argv[]) {
// code to utilize argc and argv
}
Practical Use Cases for Command-Line Arguments
Command-line arguments can enhance your C++ applications in various ways:
- User Input Handling: Instead of hardcoding values, user-specified inputs can be used to drive program logic. For instance, a program can accept filenames or configuration options directly via the command line.
- Dynamic Data Processing: When dealing with files containing data, such as specifying file paths, you can avoid recompiling by just passing different arguments.
Alternative Declarations
Using `void main()`
Some might encounter `void main()`, which looks like this:
void main() {
// code
}
However, this is non-standard and should be avoided. The C++ standard dictates that `main` should return an integer. Using `void main()` can lead to undefined behavior in many environments and is thus not compliant with the language standards.
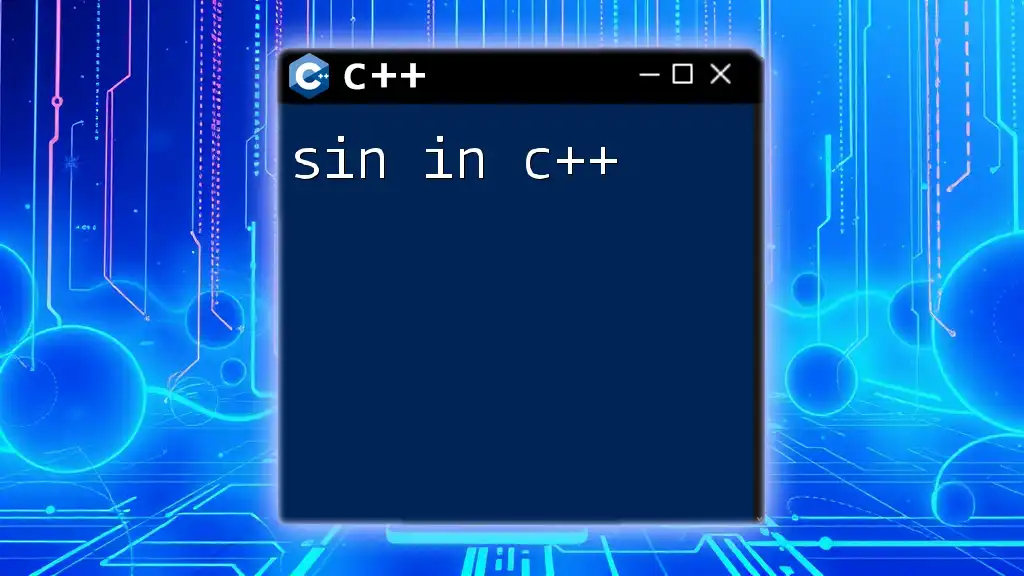
Flow of Execution in `main`
Execution Start
When a C++ program starts, the operating system sets up the execution environment and looks for the `main` function. It is here that initialization occurs, and any global variables or static initializations are resolved.
Return Statement
The return statement is crucial for signaling the end of program execution. The conventional practice is to return `0` to indicate success:
int main() {
// code
return 0; // Indicates successful execution
}
Returning a non-zero value can convey that an error has occurred, and this action can inform calling processes about the program's termination status.
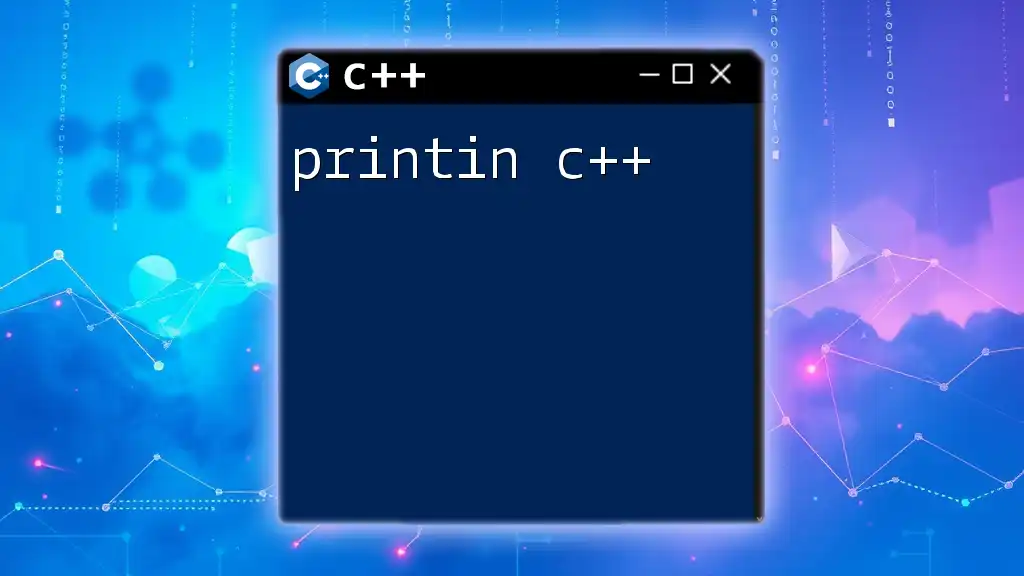
Best Practices for the `main` Function
Keep it Simple and Concise
A central tenet of good programming is to keep the `main` function simple and direct. Overly complex logic can diminish readability and maintainability. Consider the following:
This is an example of a cluttered `main`:
int main() {
// Multiple tasks performed here
initializeSettings();
runCalculations();
displayResults();
cleanUp();
// Etc.
}
In contrast, a clean `main` function focuses on high-level logic, delegating tasks to separate functions:
int main() {
initialize();
process();
display();
cleanup();
return 0; // Clear and focused
}
Error Handling
Error handling should be considered from the start when writing the `main` function. This provides a way to manage potential issues gracefully. For example, validating command-line inputs is crucial:
if (argc < 2) {
std::cerr << "Usage: " << argv[0] << " <arguments>" << std::endl;
return 1; // Error code
}
This example checks if the right number of arguments are passed and provides error feedback if needed.
Comments and Documentation
Commenting within the `main` function can profoundly impact the code's clarity. This documentation can aid both current and future developers in understanding the purpose and flow of a program.
Here's an example of a well-documented code snippet:
int main() {
// Initialization of required resources
initializeResources();
// Run the primary processing function
processData();
// Cleanup resources to prevent memory leaks
cleanUpResources();
return 0; // Successful execution
}
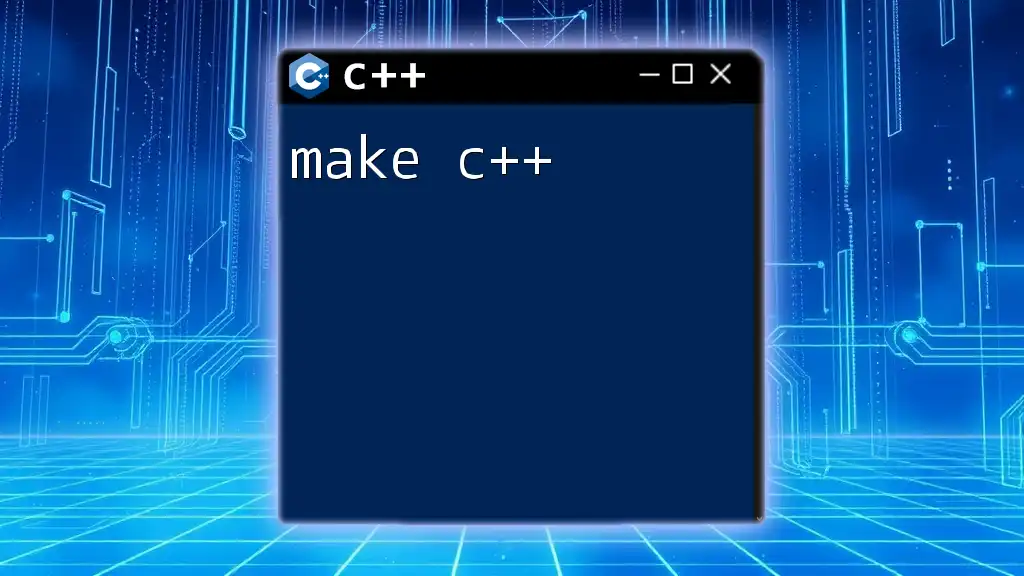
Common Mistakes with the `main` Function
Misusing Return Types
A prevalent mistake is declaring `main` as `void`. This leads to unpredictable behavior and gaps in error reporting. Always use `int` as the return type for the `main` function.
Ignoring Command-Line Arguments
Another common pitfall is neglecting to handle command-line arguments. Ignoring these inputs often results in missed opportunities for user interaction and could lead to unnecessary recompiling or code changes.
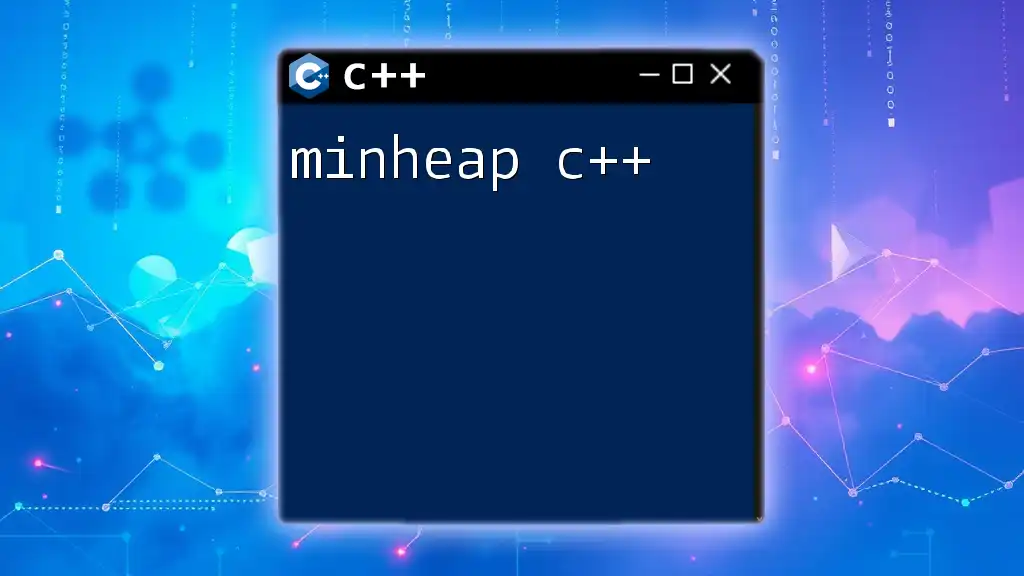
Conclusion
In summary, the main en C++ function serves as the foundation of every program, marking the starting point of execution. Understanding its structure, capabilities, and best practices is essential for writing effective C++ applications. Embracing these principles will enhance not only your coding skills but also your ability to create robust and user-friendly software.
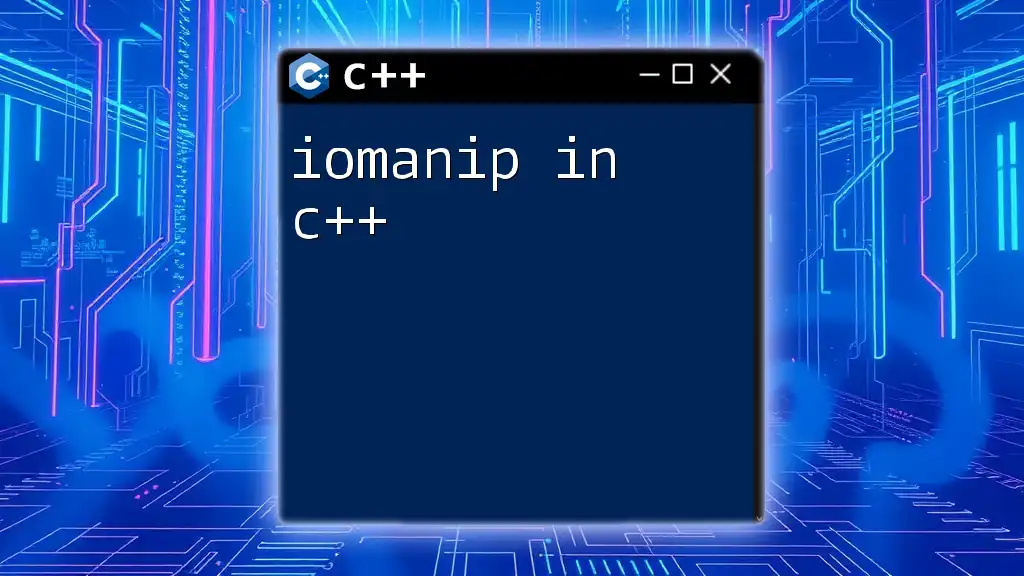
Additional Resources
For those seeking to deepen their understanding, consider exploring the official C++ documentation or investing time in recommended books and online courses that cover fundamental and advanced topics in C++. This exploration will enrich your programming journey and bolster your proficiency in C++.