CMake is a powerful build system generator for C++ projects that allows you to define the build configuration in a simple text file, which can then generate platform-specific build scripts.
Here's a basic example of a `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyExecutable main.cpp)
This script sets the minimum CMake version, names the project, specifies the C++ standard, and creates an executable from the `main.cpp` source file.
What is CMake?
CMake is an open-source, cross-platform build system generator designed specifically for managing the build process of software projects using a compiler-independent approach. Its primary purpose is to automate the building of software so developers can focus on writing code rather than managing configurations.
Originally released in the early 2000s, CMake has evolved dramatically to become a standard tool in C++ development, appreciated for its flexibility and capability to handle complex build environments. While many other build systems exist, such as Make and Autotools, CMake distinguishes itself through its ability to generate native build files for various platforms, making it an essential tool for cross-platform development.

Installing CMake
System Requirements
CMake is compatible with most operating systems, including Windows, macOS, and Linux. It is essential to ensure that your system meets the minimum requirements, which typically include having a compatible compiler installed.
Installation Methods
Using Package Managers
For users on Linux, installing CMake can often be done effortlessly through package managers. For example, on Ubuntu, you can install CMake by running:
sudo apt install cmake
Downloading Pre-Built Binaries
For those on Windows or macOS, CMake offers pre-built binaries that you can download directly from the [CMake website](https://cmake.org/download/). Select the appropriate version for your operating system, follow the installation instructions, and you're ready to go.
Building from Source
If you require the latest version or specific features not available in pre-built binaries, you can also build CMake from source. For detailed steps on this process, refer to the documentation provided on the CMake website.

Understanding CMakeLists.txt
What is CMakeLists.txt?
CMakeLists.txt is the heart of a CMake project. This text file contains all the configuration directives necessary for CMake to generate the appropriate build files for your application. Each project can have multiple CMakeLists.txt files, though a primary one typically exists in the root directory.
Key Components
Within the CMakeLists.txt file, several core components play crucial roles in configuring your project:
- Setting the Minimum Required Version: This ensures that users of your project have the correct version of CMake installed.
cmake_minimum_required(VERSION 3.10)
- Project Name and Version: This section identifies your project and specifies its version.
project(MyProject VERSION 1.0 LANGUAGES CXX)
- Language Settings: You can specify which language CMake should process, with CXX being the typical identifier for C++.
Defining Targets
Targets define executable or library components of your project. You can create executable targets using the `add_executable` command and library targets using `add_library`.
add_executable(MyExecutable main.cpp)
add_library(MyLibrary src/lib.cpp)

CMake Command Basics
Important Commands in CMake
Understanding how to use CMake commands effectively is key to generating successful builds. The `cmake` command is the principal interface. To configure a project, navigate to the build directory and run:
cmake ..
This command prepares the build system, generating necessary files.
Common CMake Commands
-
find_package(): This command allows you to find and configure external libraries that your project depends on, making it essential for managing dependencies.
-
include_directories(): Use this command to specify additional directories containing header files that your project may need.
-
link_directories(): This command allows you to specify directories where the linker should search for libraries.

Configuring Build Options
Debug vs. Release Builds
When developing software in C++, it’s crucial to manage builds sensibly. CMake supports multiple configurations, most notably Debug and Release builds. You can set the desired build type in your CMakeLists.txt file:
set(CMAKE_BUILD_TYPE Debug)
In Debug mode, the compiler includes debugging information in the generated binaries. In contrast, Release mode optimizes the binaries for performance.
Using CMake GUI and ccmake
CMake offers a graphical user interface (GUI) for configuring projects that may be preferable for users seeking a visual experience. For those who prefer the command-line, `ccmake` is a terminal-based utility that provides an interactive interface to adjust settings.
Cache Variables
CMake uses cache variables to store configuration options persistently across builds. This allows for reloading existing settings without the need to redefine them each time you configure your project.
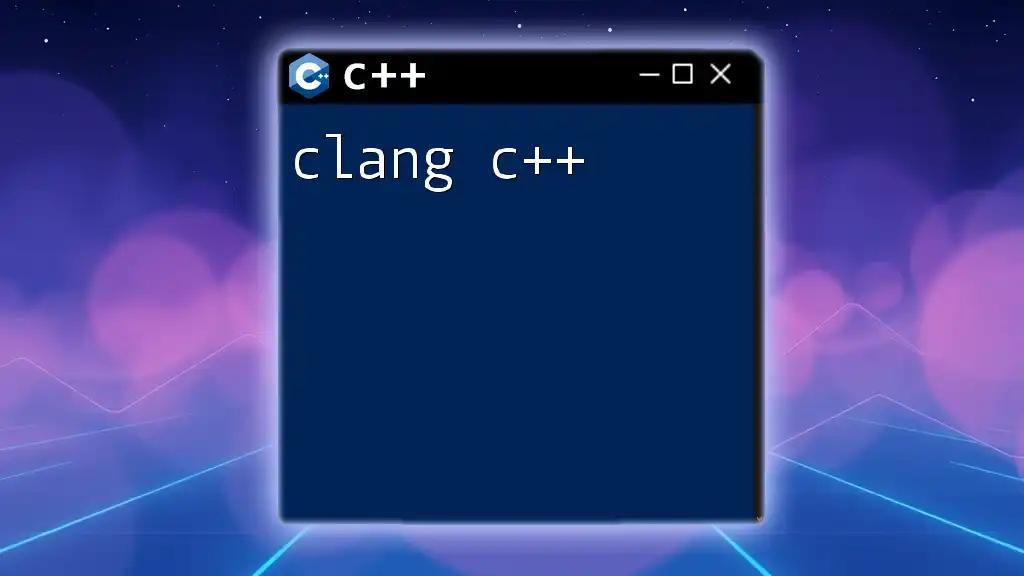
Adding Dependencies
Using External Libraries
One of CMake's strengths lies in its ability to manage dependencies elegantly. To include an external library, you can use the `find_package()` function. For instance, if you wanted to utilize the Boost library within your project, your CMakeLists.txt file would include something like:
find_package(Boost REQUIRED)
target_link_libraries(MyExecutable Boost::Boost)
This example outlines how to locate Boost and link it with your target executable.
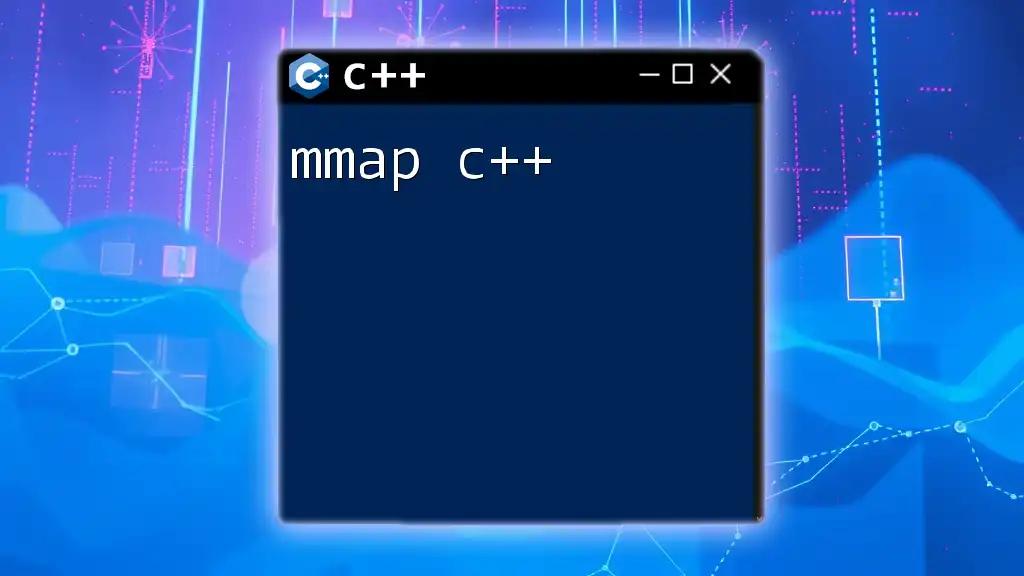
Advanced CMake Features
Custom Commands and Targets
CMake allows the definition of customized commands and targets to automate processes beyond standard builds. Use `add_custom_command()` to specify arbitrary commands to run as part of the build. Additionally, use `add_custom_target()` to create dummy targets that you can build without needing to link files.
Conditional Statements
Conditional statements enable you to adapt your configuration based on various conditions. CMake provides constructs like `if()`, `else()`, and `elseif()` to execute different commands conditionally.
if(MSVC)
# Windows-specific settings
else()
# Non-Windows settings
endif()
Modules and Packages
To streamline the usage of different components, CMake supports the creation of modules. Developers can create their own CMake modules, allowing for better organization and reusability of code across multiple projects.
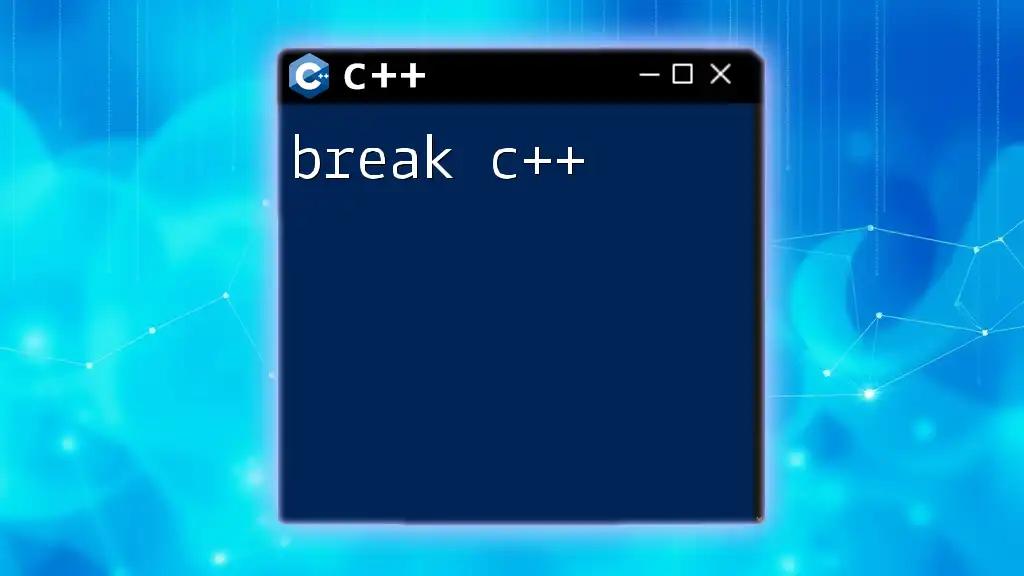
Best Practices in CMake
Organizing CMakeLists.txt Files
As projects grow in complexity, organizing the CMakeLists.txt files becomes crucial. A common practice is to maintain a separate CMakeLists.txt in each project directory, allowing for modularity and better management of components.
Avoiding Common Pitfalls
A few common issues many developers face when using CMake include improper file paths and configuration errors. To debug these, ensure that your paths are correct and consult CMake’s verbose output for error messages to troubleshoot effectively.
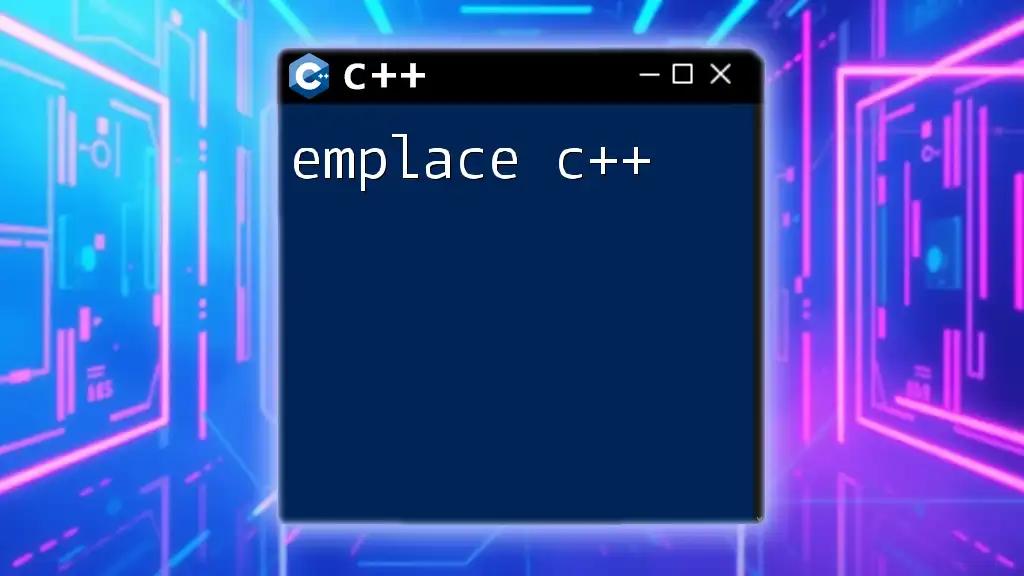
Conclusion
In summary, CMake is an invaluable tool for C++ developers, simplifying the complexity of managing builds, dependencies, and configurations. By mastering CMake, you not only streamline your development process but also achieve greater flexibility in handling cross-platform projects. To further enhance your CMake skills, explore the official CMake documentation and community resources for in-depth tutorials and examples.
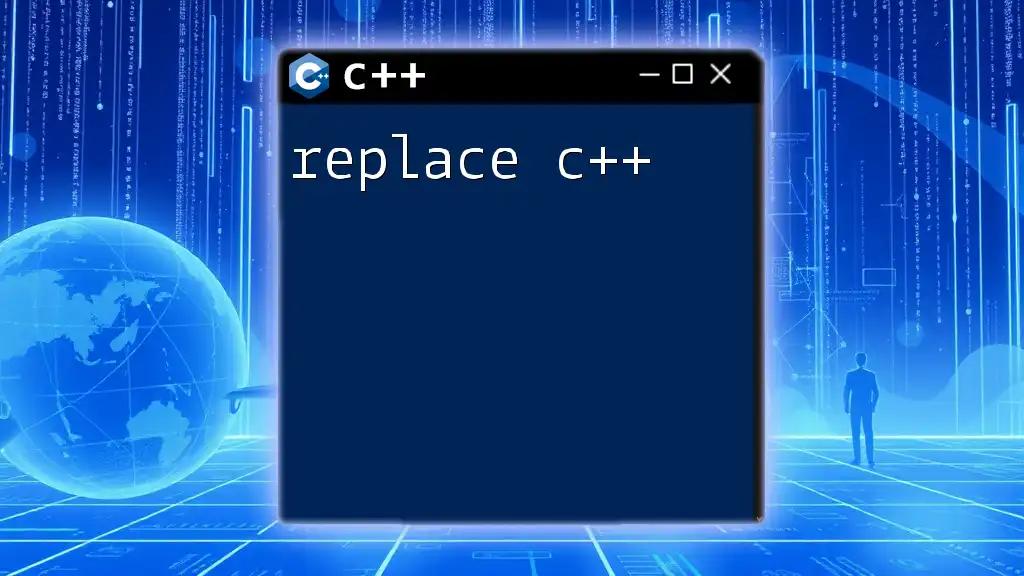
FAQs
What are the advantages of using CMake?
CMake offers several advantages, such as allowing for cross-platform builds, simplifying dependency management, and providing a robust configuration system that scales efficiently with project complexity.
How can CMake improve the build process?
CMake improves the build process by generating native build scripts for various platforms, allowing developers to focus on writing code rather than managing configurations.
Can CMake be used with projects in languages other than C++?
Yes, CMake can support projects in various programming languages, including C, Fortran, and others, making it a versatile tool in the development space.