In C++, iteration can be achieved using loops like `for`, `while`, and `do-while`, allowing execution of a block of code multiple times.
Here’s a simple example using a `for` loop to iterate through an array:
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
What is Iteration in C++?
Iteration in C++ refers to the process of executing a set of statements repeatedly, allowing programmers to handle loops efficiently and manipulate data in a structured manner. Understanding how to iterate in C++ is crucial, as it forms the backbone of handling collections, performing repeated tasks, and running algorithms effectively.
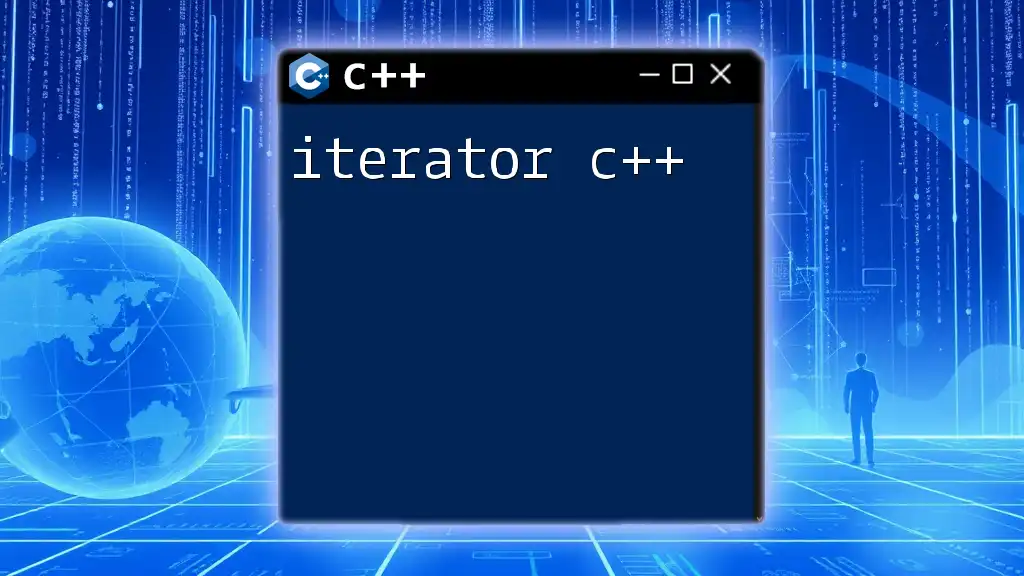
Understanding Loops
Loops are constructs that allow you to execute a block of code multiple times. In C++, there are mainly three types of loops: for loops, while loops, and do-while loops. Each type serves unique purposes depending on the problem at hand.
For Loop
The for loop is typically used when the number of iterations is known beforehand. Its syntax is straightforward:
for (initialization; condition; increment) {
// Code to be executed
}
Use Cases for For Loop:
- Counting iterations in predictable sequences.
- Iterating through arrays, vectors, or other sequential data structures.
Example: Basic For Loop
for (int i = 0; i < 10; i++) {
std::cout << i << " ";
}
In this example, the loop will print numbers from 0 to 9. The `initialization`, `condition`, and `increment` statements work together to control how the loop executes.
Nested For Loop
A nested for loop is a loop within another loop. This construct is useful when dealing with multi-dimensional arrays.
Example: Nested For Loop
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
std::cout << "(" << i << ", " << j << ") ";
}
}
This code will output pairs of indices (i, j) for a 3x3 grid.
While Loop
The while loop is suited for scenarios where the number of iterations is not known, and it continues until a specified condition is no longer met.
Syntax of While Loop:
while (condition) {
// Code to be executed
}
Use Cases for While Loop:
- Repeatedly execute a code block until a condition is false.
Example: Basic While Loop
int i = 0;
while (i < 10) {
std::cout << i << " ";
i++;
}
In this case, the loop prints numbers from 0 to 9 and stops when `i` reaches 10.
Do-While Loop
The do-while loop is similar to the while loop, but it guarantees at least one execution of the code block before checking the condition.
Syntax of Do-While Loop:
do {
// Code to be executed
} while (condition);
Use Cases for Do-While Loop:
- When at least one iteration is necessary, regardless of the condition.
Example: Basic Do-While Loop
int i = 0;
do {
std::cout << i << " ";
i++;
} while (i < 10);
This loop behaves similarly to the while loop, ensuring that it runs at least once.
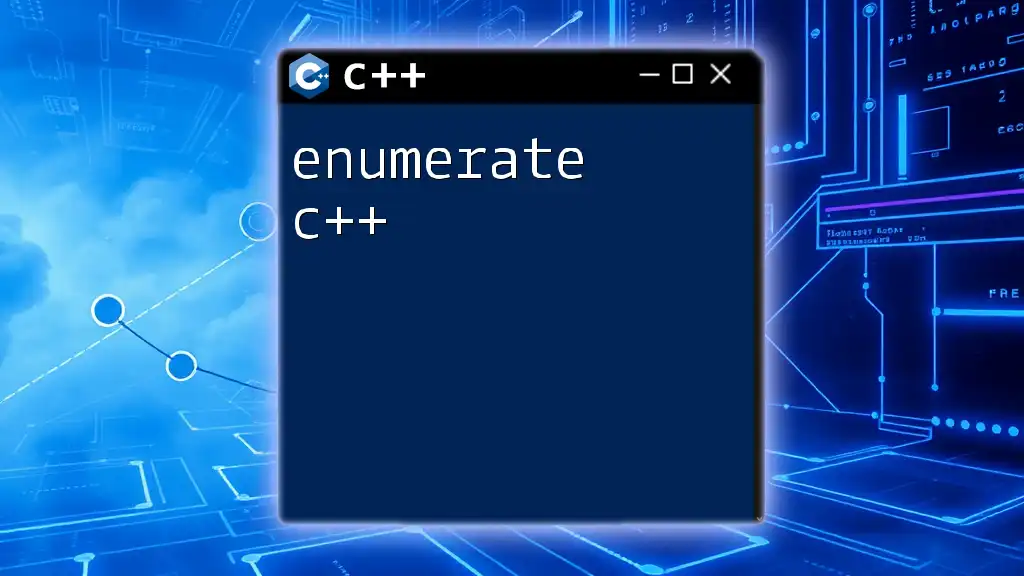
Advanced Iteration Techniques
Range-Based For Loop
Introduced in C++11, the range-based for loop simplifies iteration over containers such as arrays and vectors.
What is a Range-Based For Loop?
This loop provides a more concise syntax for iterating through a sequence of elements without needing to explicitly manage an index.
Syntax and Benefits:
for (declaration : collection) {
// Code to be executed
}
Example: Using Range-Based For Loop with Arrays
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << " ";
}
This code outputs the elements of the vector `numbers` in a straightforward manner.
Iterators
What are Iterators?
Iterators are objects that allow you to traverse the elements of a container, such as a vector or list, without exposing the underlying structure.
Using Iterators with STL Containers:
You can use iterators to safely iterate through elements while providing flexibility for different container types.
Example: Iterating through a Vector
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
In this example, `it` acts as an iterator pointing to the elements of `numbers`, allowing you to access each element using `*it`.
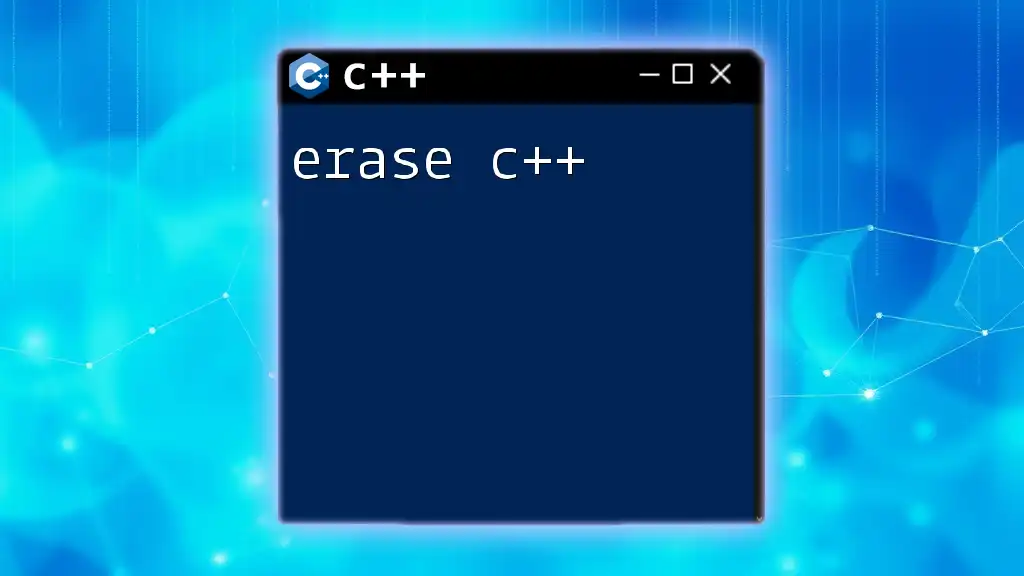
Iterating Through Complex Data Structures
Iterating Through Maps
In C++, maps are associative containers that store elements in key-value pairs, which can also be iterated.
Understanding Maps in C++
Maps are typically implemented as red-black trees, ensuring that keys are sorted and unique.
Example: Iterating Through a Map
std::map<std::string, int> scores = {{"Alice", 90}, {"Bob", 85}};
for (const auto& pair : scores) {
std::cout << pair.first << ": " << pair.second << "\n";
}
This code iterates through the `scores` map, printing each key and its corresponding value.
Iterating Through Nested Data Structures
Iterating through nested data structures can require additional loops.
Example: Nested Vectors
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}};
for (const auto& row : matrix) {
for (const auto& col : row) {
std::cout << col << " ";
}
std::cout << "\n";
}
In this case, the outer loop iterates over each row, while the inner loop accesses and prints each element of the row.
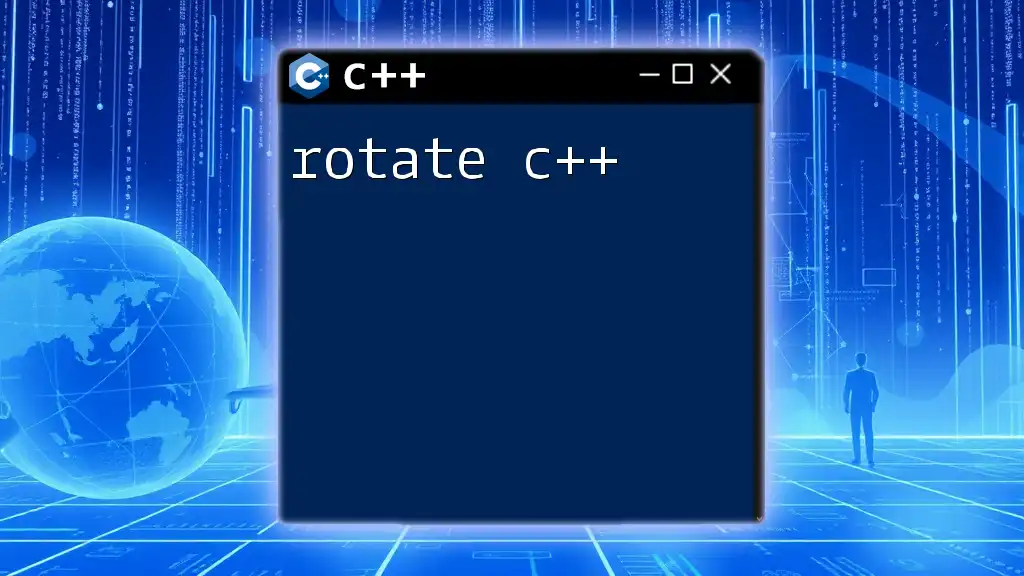
Summarizing Key Points
Through loops, C++ provides powerful tools to iterate over data structures efficiently. Understanding when and how to use different types of loops—for, while, and do-while—is essential for effective programming. The introduction of range-based for loops and iterators in modern C++ further simplifies the iteration process, making code more expressive and easier to understand.
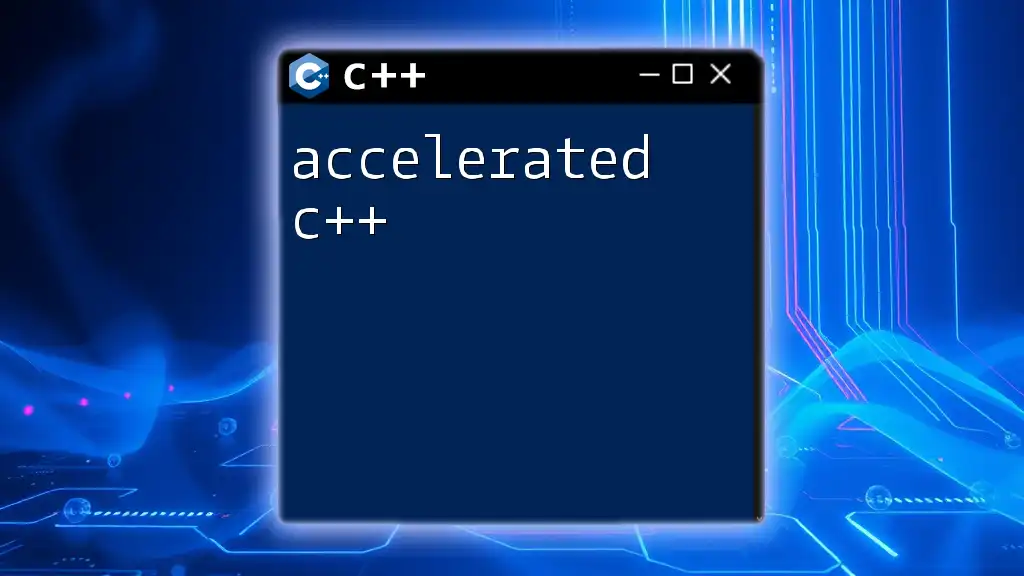
Encouragement to Practice
To truly grasp the concepts discussed, practice using different types of loops with various data structures. Experiment with real-world problems to see how iteration can simplify complex tasks.
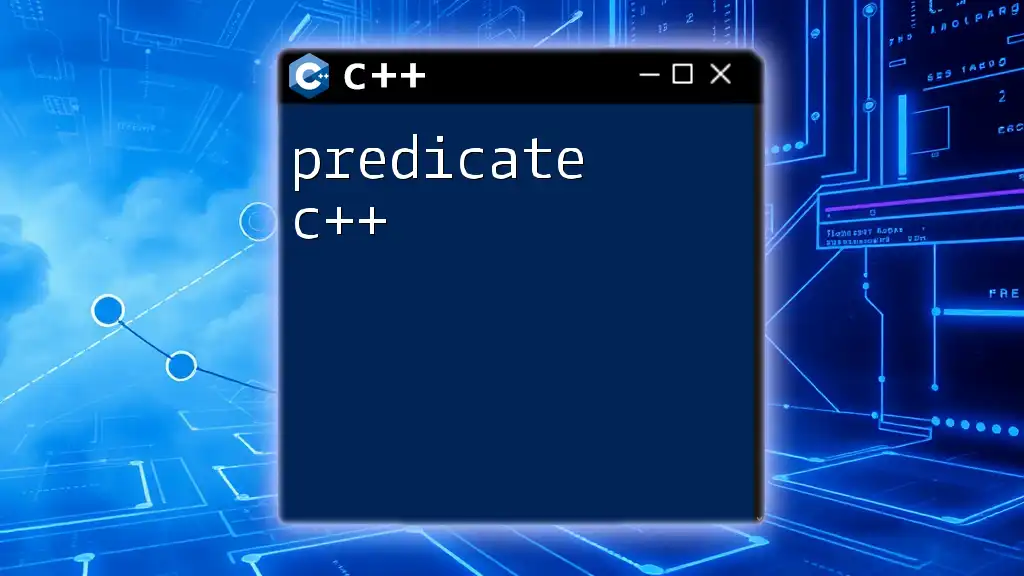
Call to Action
For more tutorials and in-depth resources on iterating in C++, be sure to explore our website, where you’ll find a wealth of knowledge to enhance your programming skills!