Ideone is an online compiler and debugging tool that allows users to write, run, and share C++ code snippets easily without the need for local setup.
Here's a simple example of a C++ program that prints "Hello, World!" using Ideone:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Ideone?
Ideone is an online compiler and debugging tool that allows users to write, compile, and execute code in various programming languages, including C++. It serves as a convenient platform for programmers who want to quickly test their code without the need to set up a local development environment. The primary purpose of Ideone is to facilitate quick experimentation with code, making it especially useful for students, educators, and developers collaborating on small projects or code snippets.
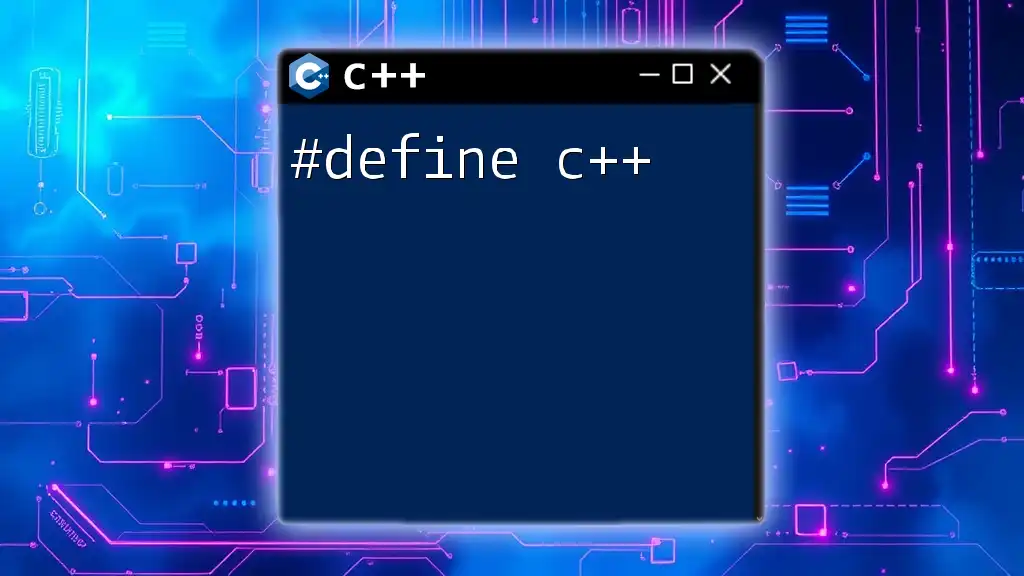
Why Use Ideone for C++ Development?
Using Ideone for C++ development offers several advantages:
- Accessibility and Convenience: Being a web-based tool, Ideone can be accessed from any device with internet connectivity, allowing users to code on the go.
- Collaborating on Code Snippets: Ideone provides sharing options, making it easy to collaborate with others. You can simply share a link to your code, enabling others to view, compile, and run it instantly.
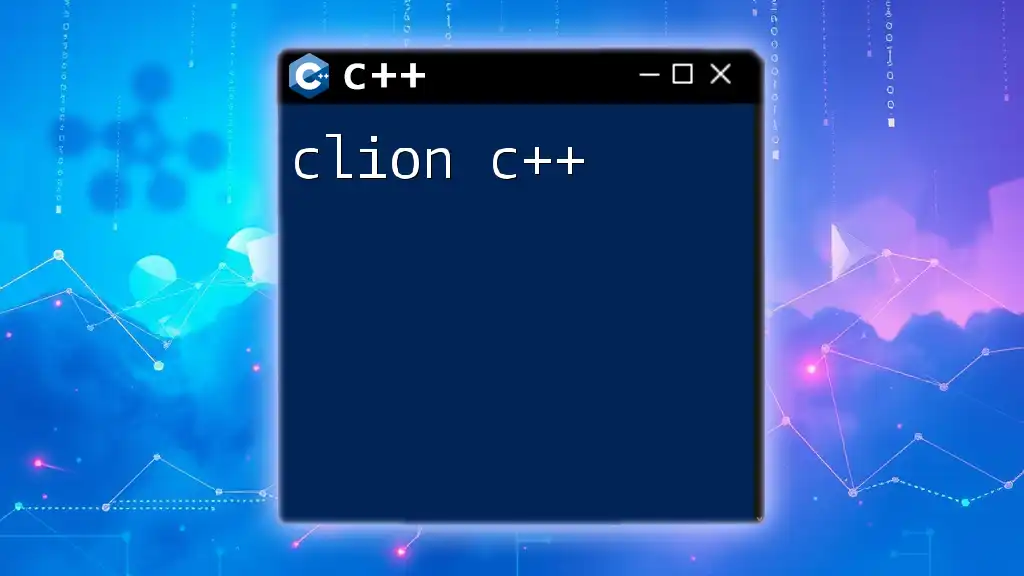
Getting Started with Ideone
Creating an Account
Creating an account on Ideone is a straightforward process.
- Visit the Ideone website and click on the sign-up button.
- Fill in the requisite information, such as your email address and password.
- Verify your email to activate your account.
Having an account allows you to save your code snippets and access them later, along with additional features such as better management of your submissions.
Navigating the User Interface
Upon logging in, you'll be greeted by the user-friendly interface of Ideone:
- Code Editor: This is where you will write your C++ code. The editor provides basic syntax highlighting to help you organize and read your code.
- Output Window: Displays the output of your compiled code or any error messages that may occur during execution.
- Settings: You can select the programming language (in this case, C++) and tweak various other settings to suit your coding style.
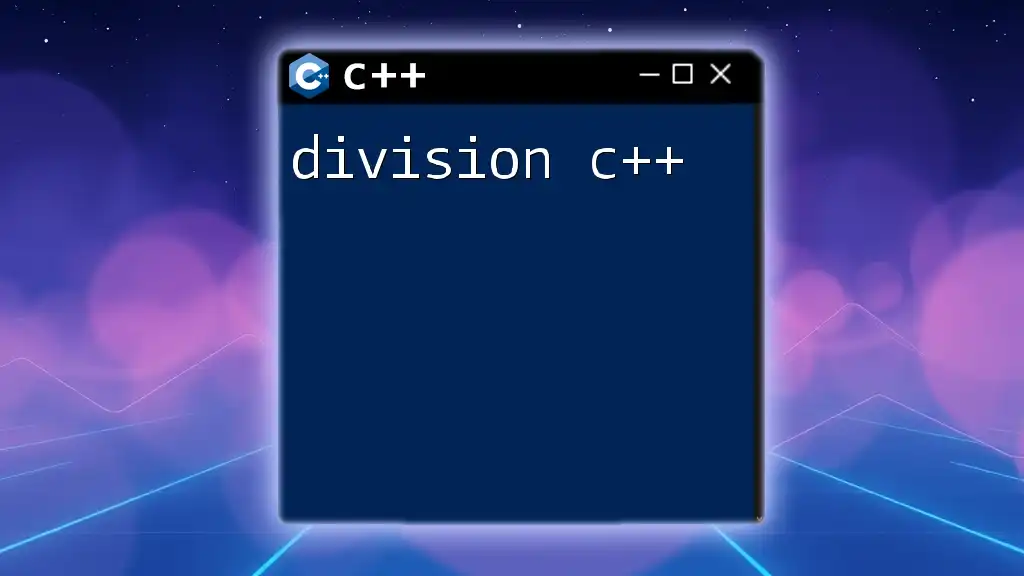
Writing C++ Code on Ideone
Writing Your First C++ Program
Let’s create a simple "Hello, World!" program to demonstrate how to use Ideone for writing C++ code.
Here’s how you can do it:
- In the editor, type the following code:
#include<iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- Click on the Run button to compile and execute your code.
Expected Output: Upon successful execution, "Hello, World!" will be displayed in the output window. This simple program serves as a foundation for understanding the mechanics of C++.
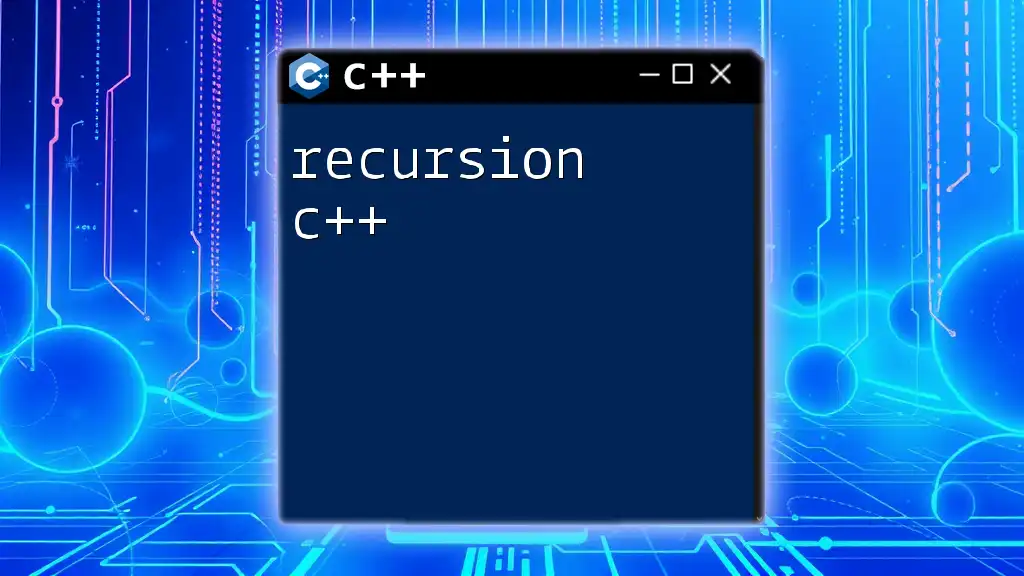
Compiling and Running C++ Code
Compiling Your Code
Once you’ve entered your code in the editor, it's time to compile it. Clicking on the Run button will trigger the compilation process.
Running Your Code
After the code is compiled, it automatically runs, and the output is presented in the output window. You can see the results of your code execution, along with any error messages if applicable.
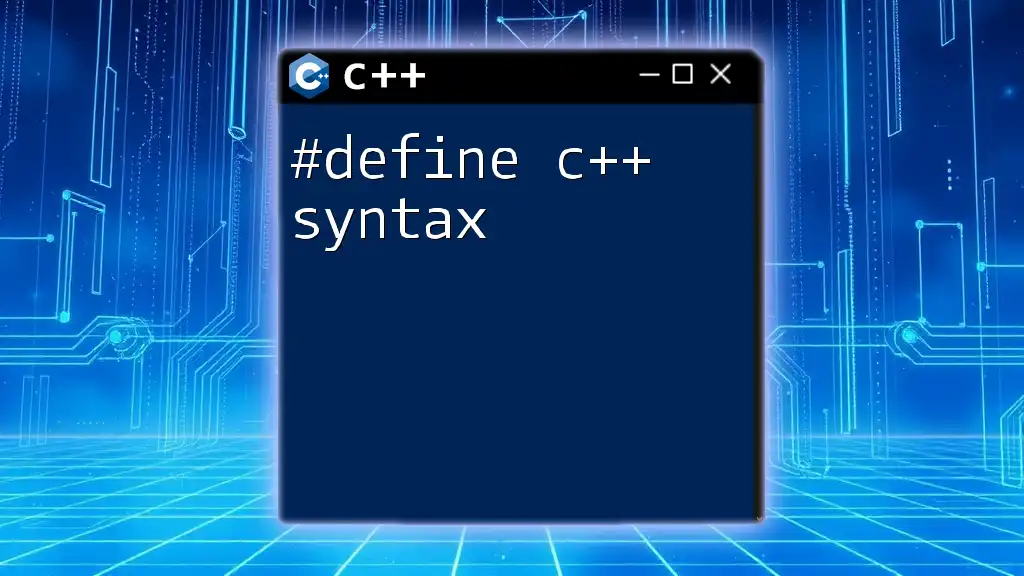
Debugging in Ideone
Common Errors
When coding, you may encounter syntax errors, runtime errors, or logical errors. Ideone is designed to help you identify these issues quickly.
- Syntax Errors: If there’s something wrong with the way your code is written, Ideone will highlight this issue with a detailed error message.
- Runtime Errors: These occur when your code compiles successfully but fails during execution (for example, division by zero).
Debugging Techniques
Debugging is an essential skill for any programmer. Here are some tips for effectively using Ideone:
- Read Error Messages: Make sure to take note of the error messages provided by Ideone; these messages often indicate line numbers and the nature of the error.
- Break Down Your Code: If an error is encountered, simplify your code to isolate the problematic section. This can make it easier to troubleshoot.
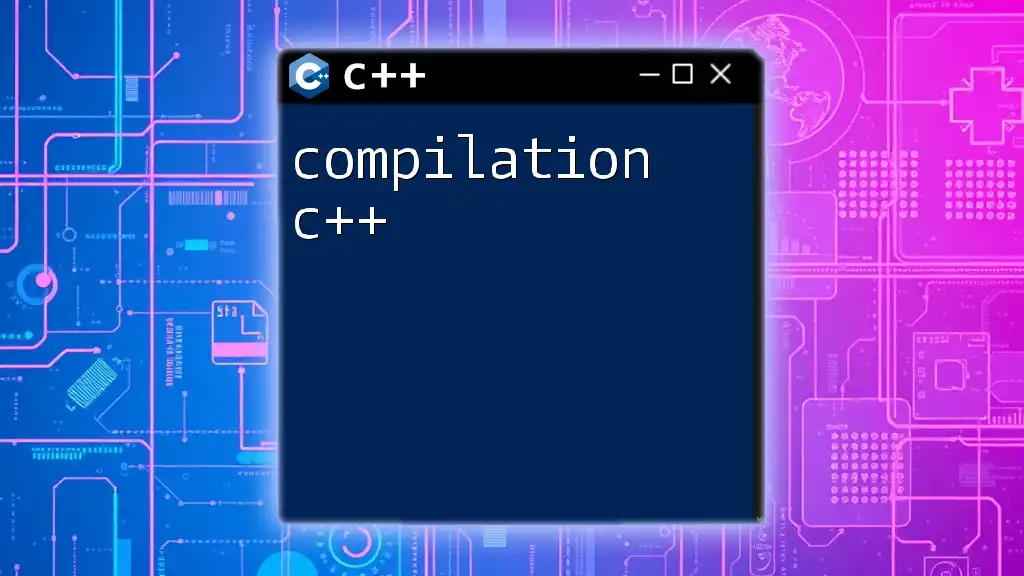
Using C++ Libraries and Features
Including Libraries in Ideone
C++ is equipped with various libraries that facilitate advanced programming features. To include a library, you simply start your program with the appropriate `#include` directive.
For example, to use vectors:
#include<iostream>
#include<vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
cout << num << " ";
}
return 0;
}
Understanding C++ Features Available
Ideone supports multiple versions of C++. It's essential to write code compatible with the selected standard. Common features include:
- C++11: This version introduces various enhancements like ranged-based for loops.
- Auto Keyword: This is particularly useful for type inference:
auto x = 10; // Automatic type deduction
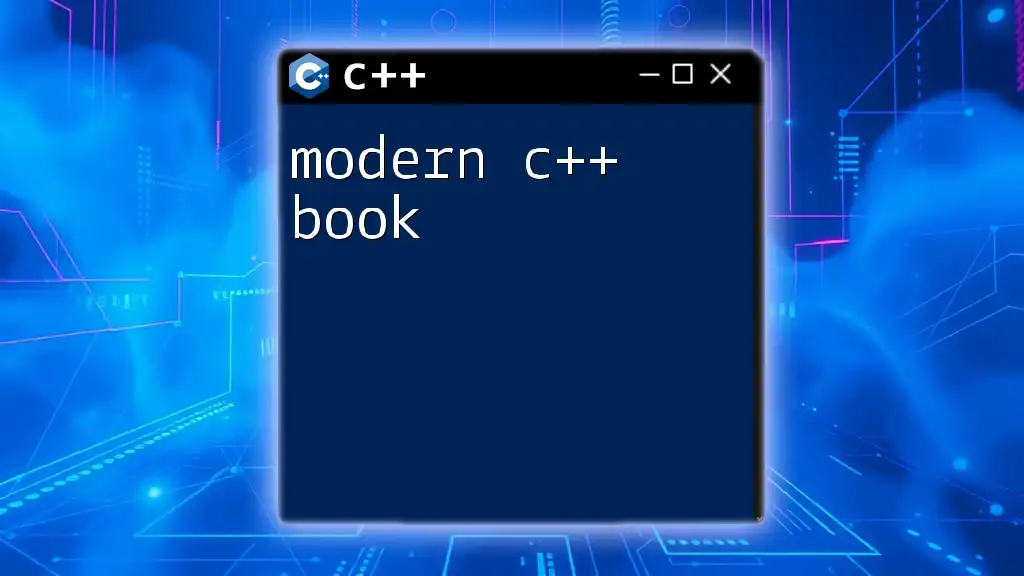
Sharing Your Code
How to Share Code Snippets
One of Ideone's standout features is the ability to share your code snippets. After running your code, click on the Share button. This action will generate a unique URL that you can send to others, allowing them to see and run your code instantly.
Collaborative Features
Ideone’s sharing capabilities make it an excellent tool for collaborative projects. You and your peers can review each other's code and make necessary modifications, facilitating a more effective coding environment.
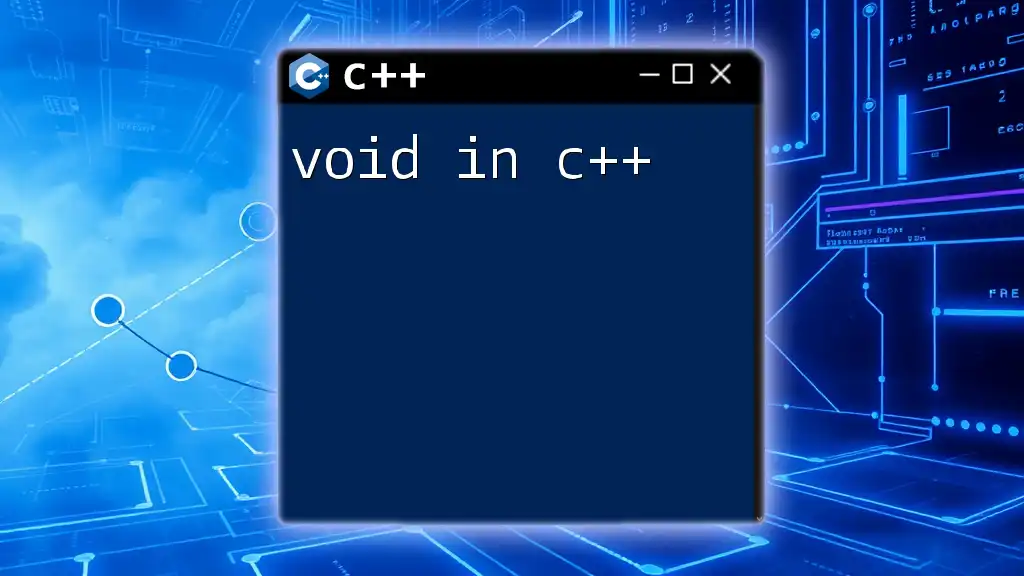
Best Practices for Using Ideone
Writing Clean Code
Clean code is easier to understand and maintain. Always aim to format your code properly, use descriptive variable names, and include comments where necessary.
Leveraging Ideone's Features
For optimal use of Ideone, familiarize yourself with its functionalities, such as saving code versions and organizing submissions. This can enhance your overall coding experience.
Security and Privacy Tips
When using Ideone, be cautious about sharing sensitive data. Avoid including any personal information or proprietary code that should remain confidential.
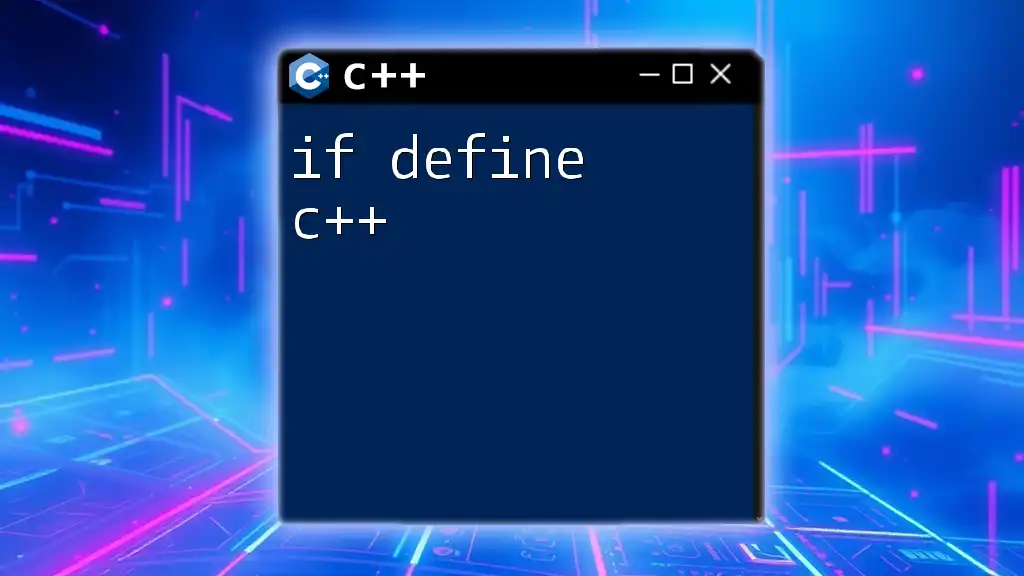
Advanced Usage of Ideone
Using IDE Integrations
For seasoned programmers, Ideone allows integrations with popular IDEs, providing additional functionalities that can improve your coding efficiency. Explore how to set these up for a more streamlined experience.
Running Larger Projects
While Ideone is superb for small snippets, it has limitations. Be aware of file size restrictions and execution time limits when working on larger projects.
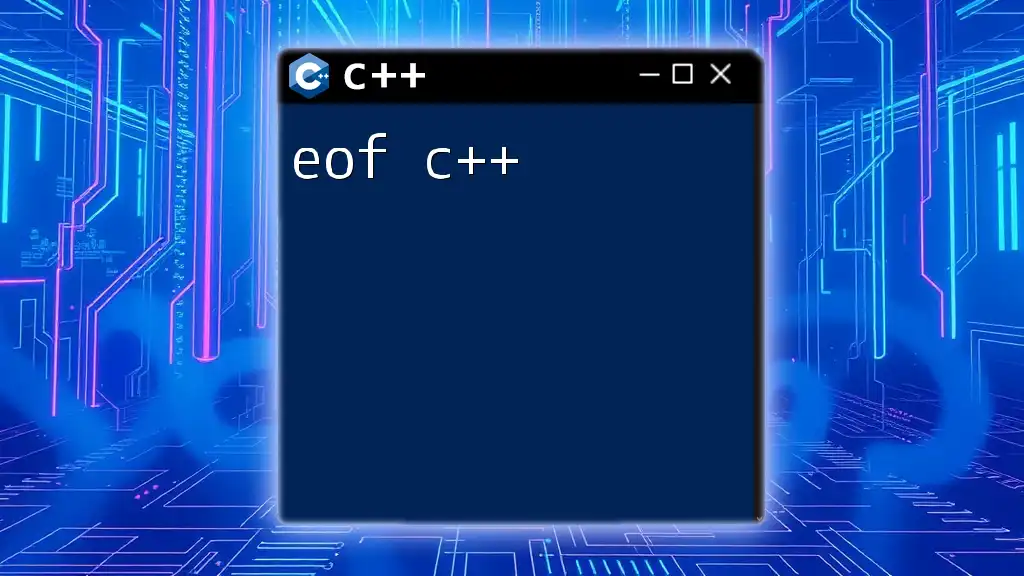
Conclusion
In summary, Ideone is a powerful tool for anyone interested in learning or using C++. Its myriad of features, combined with its accessibility and collaboration capabilities, make it a must-have in any programmer's toolkit. Whether you are debugging code, sharing snippets, or writing complex algorithms, Ideone provides an intuitive platform to enhance your programming journey.
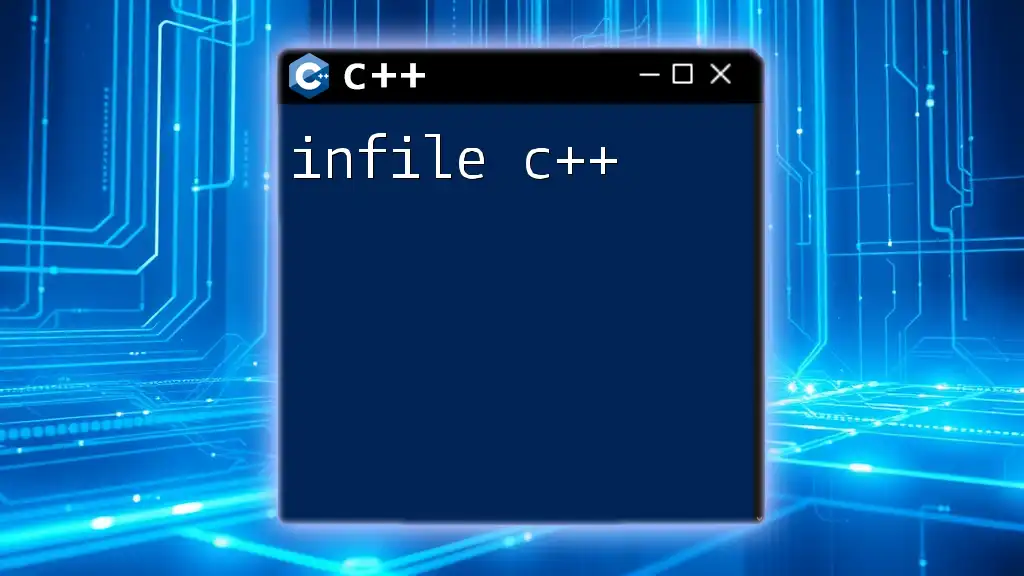
Further Reading and Resources
For those eager to deepen their knowledge of C++, consider exploring the following resources:
- Books: “C++ Primer” and “Effective C++” are excellent for both beginners and experienced programmers.
- Websites: Explore dedicated C++ forums and resources like GeeksforGeeks, Stack Overflow, and LearnCPP for additional guidance.
- FAQs: Addressing common questions can clear many doubts about utilizing Ideone effectively, ensuring you make the most of this great tool.