In C++, input/output (I/O) operations are primarily handled using the `cin` and `cout` streams from the `<iostream>` library for reading and writing data to the console.
Here's a simple example demonstrating basic I/O:
#include <iostream>
int main() {
std::cout << "Enter your name: ";
std::string name;
std::cin >> name;
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
Understanding I/O Streams
What are I/O Streams?
In C++, I/O streams are essential for handling input and output operations. They allow developers to read from or write to various data sources, like the console or files. A stream represents a sequence of bytes, which can be processed as a flow of information that enters or leaves the program.
There are two primary types of streams:
- Input Streams: These streams provide data to the program, typically from devices like the keyboard or files.
- Output Streams: These streams send data from the program to devices like the console or files.
Overview of Standard Streams
C++ provides several standard streams that are part of the iostream library, making it easier to perform I/O tasks. The following are the most commonly used:
- `cin`: Input stream used to read data from the standard input device (keyboard).
- `cout`: Output stream used to display data to the standard output device (console).
- `cerr`: Output stream used for displaying errors. It is generally unbuffered, meaning output appears immediately.
- `clog`: Similar to `cerr`, but it is buffered, which may be preferable for logging purposes.
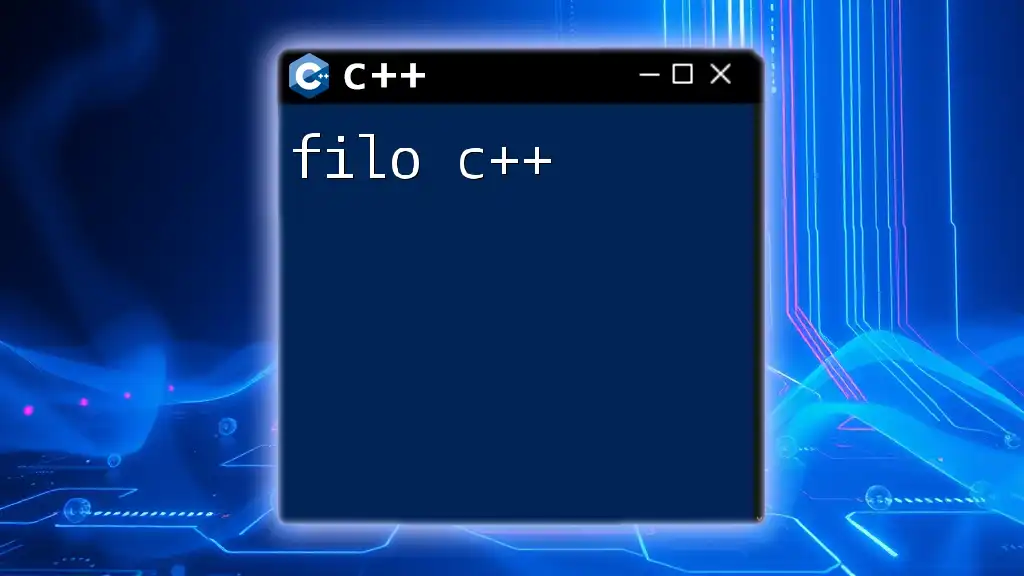
Basic Input and Output
Using `cout` for Output
The `cout` object is part of the `iostream` library and is used to output data to the console. The syntax for using `cout` is straightforward:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Outputs: Hello, World!
return 0;
}
In this example, the `endl` manipulator is used to insert a newline character and flush the output buffer.
Using `cin` for Input
The `cin` object captures user input through the console. The basic syntax for using `cin` is:
#include <iostream>
using namespace std;
int main() {
int age; // Variable to store user input
cout << "Enter your age: ";
cin >> age; // Reads input from the user
cout << "Your age is: " << age << endl; // Outputs the age entered
return 0;
}
In this snippet, the user is prompted to enter their age, which is then read into the variable `age`. This showcases how easily we can interact with the user through console input.
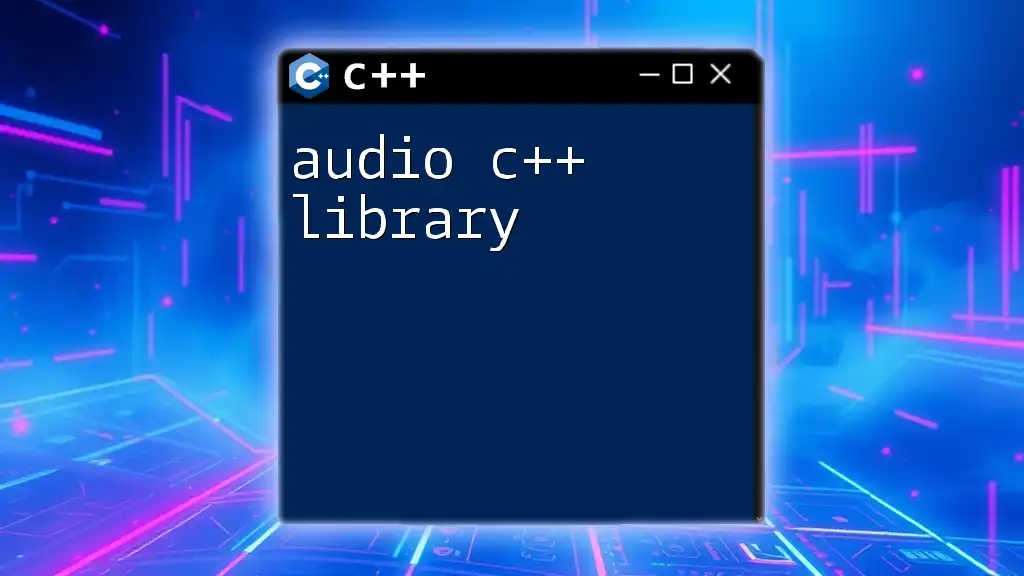
Advanced Output Formatting
Format Flags
C++ allows for sophisticated output formatting through the use of format flags. For instance, you can adjust number output using manipulators like `std::hex` (for hexadecimal), `std::dec` (for decimal), and more.
Here's how to format outputs:
#include <iostream>
#include <iomanip> // Required for manipulators
using namespace std;
int main() {
int num = 255;
cout << "Decimal: " << dec << num << endl; // Decimal: 255
cout << "Hexadecimal: " << hex << num << endl; // Hexadecimal: ff
return 0;
}
In this example, we switch between decimal and hexadecimal output formats, emphasizing the versatility of output manipulation in C++.
Width and Fill
C++ also allows you to specify the width of the output and to set fill characters. This is useful for aligning output easily:
#include <iostream>
#include <iomanip> // Import for manipulators
using namespace std;
int main() {
cout << setw(10) << setfill('*') << 42 << endl; // Outputs: *****42
return 0;
}
This code uses `setw()` to define a width of 10 characters and `setfill()` to specify the filling character as `*`. It showcases how you can present data in a visually appealing way.
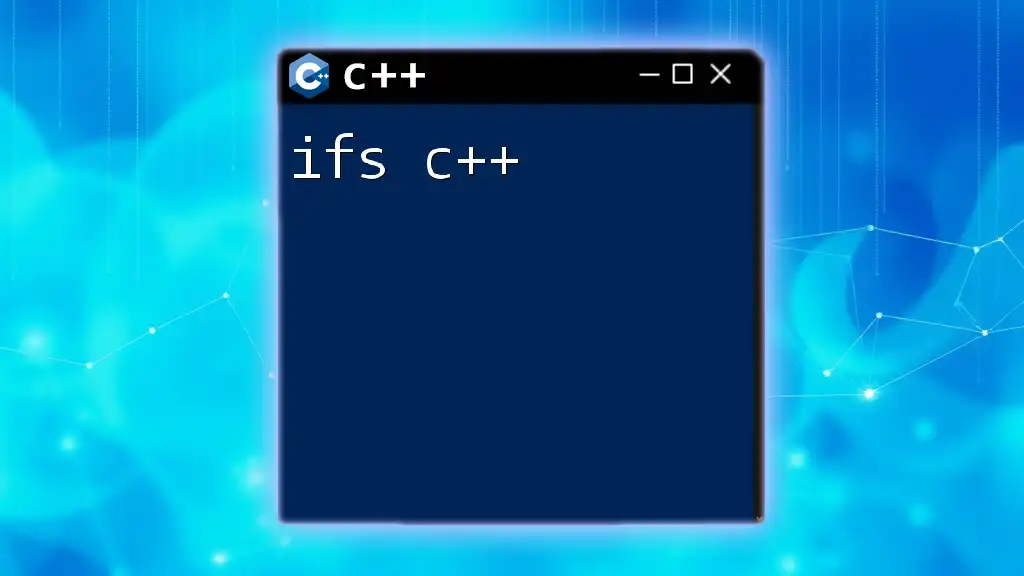
File I/O in C++
Introduction to File Streams
File streams are crucial for data persistence, allowing applications to read and write data to files. C++ provides two main types of file streams: `ifstream` for input file streams and `ofstream` for output file streams.
Reading from Files
To read data from a file, you can use the `ifstream` class as follows:
#include <fstream>
#include <iostream>
#include <string> // Required for string class
using namespace std;
int main() {
ifstream inputFile("data.txt"); // Open data.txt
string line;
// Check if the file opened successfully
if (!inputFile) {
cerr << "Error opening file!" << endl;
return 1; // Exit with error code
}
while (getline(inputFile, line)) { // Read line by line
cout << line << endl; // Output each line to console
}
inputFile.close(); // Close the file
return 0;
}
This example reads from a file named `data.txt`, displaying its content line by line in the console. Proper error handling is incorporated to ensure the file opened successfully.
Writing to Files
To write data to a file, use the `ofstream` class like this:
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ofstream outFile("output.txt"); // Open output.txt
if (!outFile) {
cerr << "Error opening file!" << endl;
return 1; // Exit with error code
}
outFile << "Hello, File!" << endl; // Write a line to the file
outFile.close(); // Close the file
return 0;
}
Here, the program opens a file called `output.txt` and writes a string into it. As with reading files, checking if the file opened successfully is important.
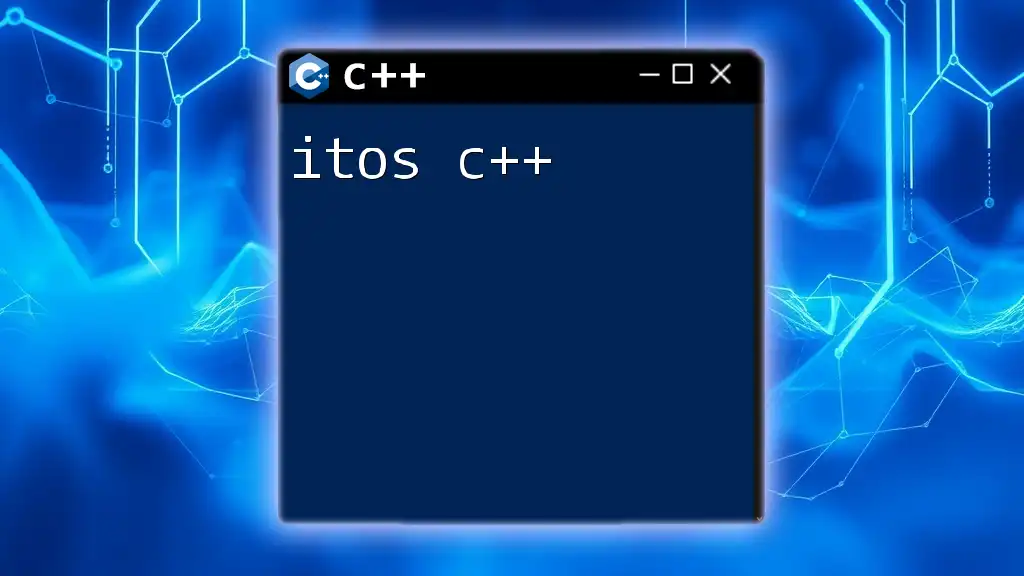
Error Handling in C++ I/O
Common I/O Errors
I/O operations can lead to various errors, such as file not found, read failures, and write errors. Understanding how to handle these errors is crucial in developing robust applications.
Using Exceptions
C++ offers exception handling to manage these situations. For example:
#include <fstream>
#include <iostream>
#include <stdexcept> // Required for exceptions
using namespace std;
int main() {
try {
ifstream file("nonexistent.txt");
if (!file) {
throw runtime_error("File not found"); // Throw an exception
}
} catch (exception& e) {
cout << "Error: " << e.what() << endl; // Catch and display error
}
return 0;
}
This code demonstrates how to handle file opening errors using exceptions, allowing for greater control over error management.
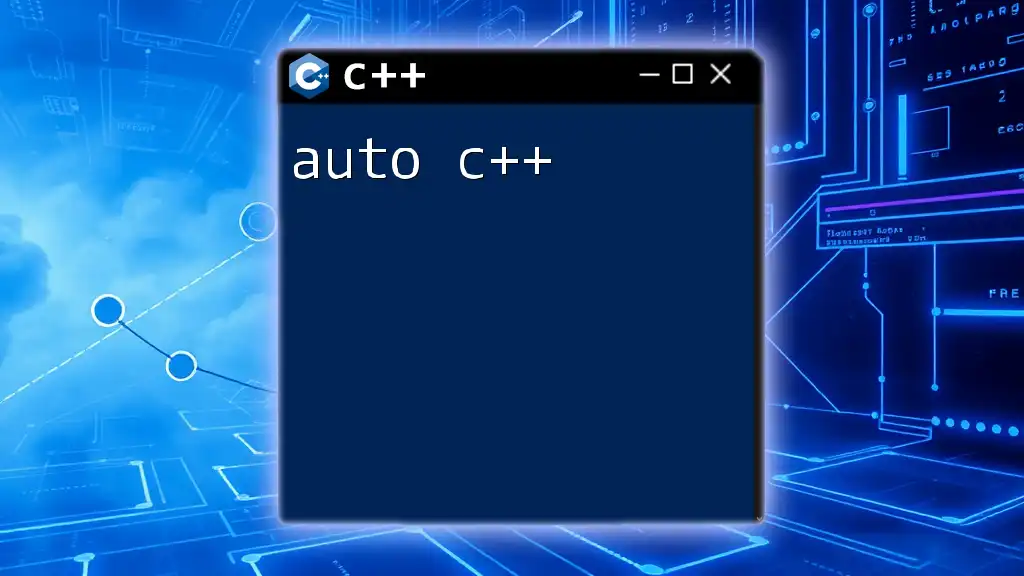
Conclusion
Mastering I/O in C++ is vital for effective programming, enabling you to read from and write to various data streams. By understanding the different types of streams and their usage, along with adept output formatting and file I/O operations, you build a strong foundation for more complex programming tasks. Practicing these concepts will not only enhance your coding skills but also your ability to handle real-world data in C++.