The `div` operator in C++ performs integer division, returning the result of the division without any fractional component.
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a / b; // Integer division
std::cout << "The result of " << a << " div " << b << " is: " << result << std::endl;
return 0;
}
Understanding Division in C++
What is Division?
Division is a fundamental mathematical operation that calculates how many times one number is contained within another. In programming, especially in C++, division is a crucial operation that allows us to perform calculations, manipulate data, and solve complex problems.
How Division Works in C++
In C++, the division operator is represented by the `/` symbol. This operator can be used with various data types, including integers and floating-point numbers. Understanding how division works under different circumstances is essential for effective programming.
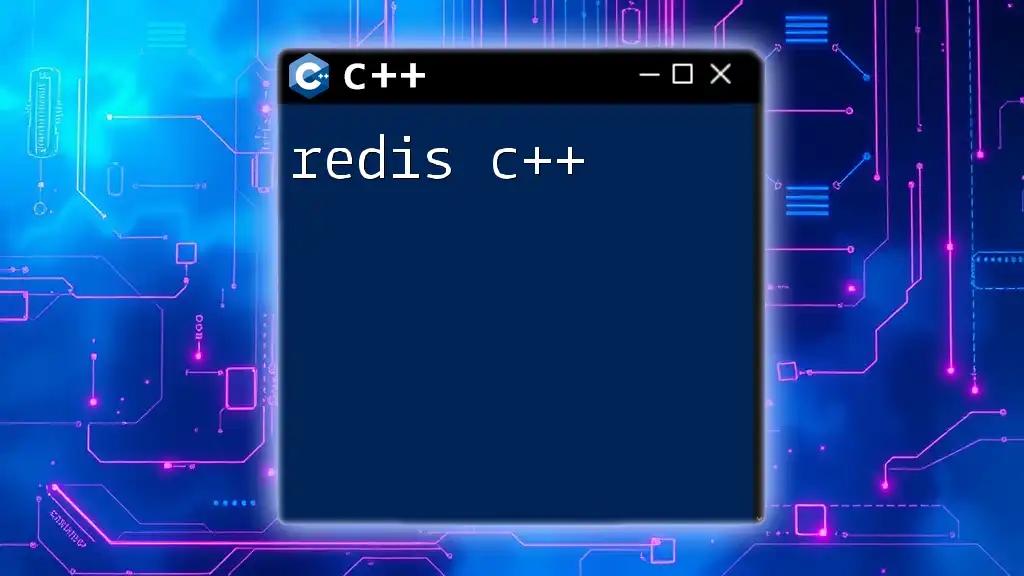
Types of Division in C++
Integer Division
Definition and Explanation Integer division occurs when both operands are integers. In this case, the result is also an integer, with any fractional part discarded. For example, when dividing 10 by 3, instead of getting a result of 3.33, integer division will yield 3.
Code Snippet: Integer Division
int a = 10;
int b = 3;
int result = a / b;
std::cout << "Integer Division: " << result << std::endl; // Outputs 3
Floating-Point Division
Definition and Explanation Floating-point division is used when at least one of the operands is a floating-point number (either `float` or `double`). This type of division retains the fractional part of the result, providing a more precise outcome than integer division.
Code Snippet: Floating-Point Division
double x = 10.0;
double y = 3.0;
double floatResult = x / y;
std::cout << "Floating-Point Division: " << floatResult << std::endl; // Outputs ~3.33

Handling Division by Zero
Understanding Division by Zero
Dividing by zero is mathematically undefined and results in undefined behavior in C++. This can lead to runtime errors and crashes in programs if not properly handled. Thus, it is critical for programmers to anticipate this situation to ensure program stability.
Best Practices to Avoid Division by Zero
To prevent division by zero, always check if the denominator is zero before performing the operation.
Code Snippet: Safe Division
int numerator = 10;
int denominator = 0;
if (denominator != 0) {
std::cout << "Result: " << numerator / denominator << std::endl;
} else {
std::cout << "Error: Division by zero!" << std::endl;
}
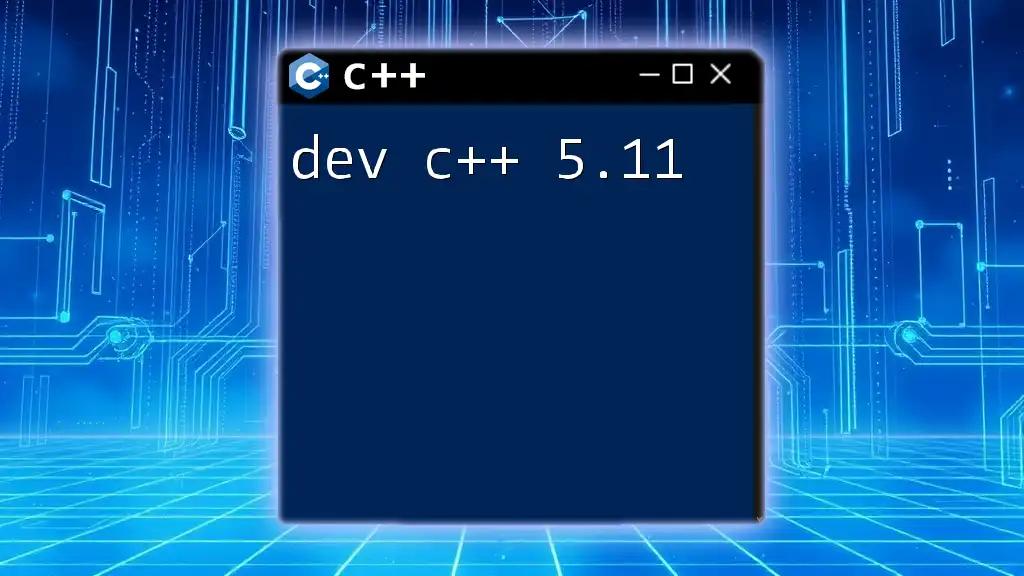
Rounding Results in Division
Importance of Rounding
Rounding can be essential when dealing with floating-point numbers. Due to the way computers handle floating-point arithmetic, results can be imprecise and may require rounding to achieve the desired outcome.
Different Rounding Techniques
C++ offers several rounding functions such as `floor`, `ceil`, and `round`. Understanding these functions will help developers handle results correctly.
Code Snippet: Rounding in Division
double result = 10.0 / 3.0; // ~3.33
std::cout << "Using floor: " << std::floor(result) << std::endl; // Outputs 3
std::cout << "Using ceil: " << std::ceil(result) << std::endl; // Outputs 4
std::cout << "Using round: " << std::round(result) << std::endl; // Outputs 3

Advanced Division Techniques
Using Division in Algorithms
Division plays a significant role in various algorithms, such as sorting and searching. For example, in quicksort, dividing the dataset into two halves is essential for the algorithm's efficiency.
Divisor Properties
Understanding divisor properties, such as the Greates Common Divisor (GCD), is crucial when dealing with fraction simplification or number theory problems.
Code Snippet: Finding GCD using Division
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
std::cout << "GCD of 10 and 5: " << gcd(10, 5) << std::endl; // Outputs 5

Common Mistakes with Division in C++
Common Pitfalls
One prevalent mistake in C++ is the misunderstanding of integer division, which often leads to unintentional truncation of results. It’s vital to recognize how the division operator behaves differently based on the data types of its operands.
Debugging Division Issues
When encountering problems with division in C++, carefully consider how variables have been defined and the types being used. Pay close attention to potential places where division by zero might occur and validate inputs accordingly.

Conclusion
Mastering division in C++ is fundamental for any programmer. Through understanding integer and floating-point division, managing division by zero, employing rounding techniques, and utilizing division in algorithms, you can build more robust applications. By applying the concepts discussed, you will enhance your coding proficiency and confidence in using division effectively. Experiment with the provided code examples to deepen your understanding and become adept at using the division operator in C++.