Dev C++ 5.11 is an integrated development environment (IDE) for C and C++ programming that provides users with a straightforward platform to write, compile, and debug their code efficiently.
Here's a simple code snippet demonstrating how to print "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of Dev-C++
Dev-C++ is a widely used integrated development environment (IDE) for programming in C and C++. It provides users with a convenient interface and robust functionality, making it an excellent tool for both beginners and experienced developers. The latest stable release, Dev-C++ 5.11, continues to support C++ programming while incorporating several enhancements that cater to the needs of today's developers.
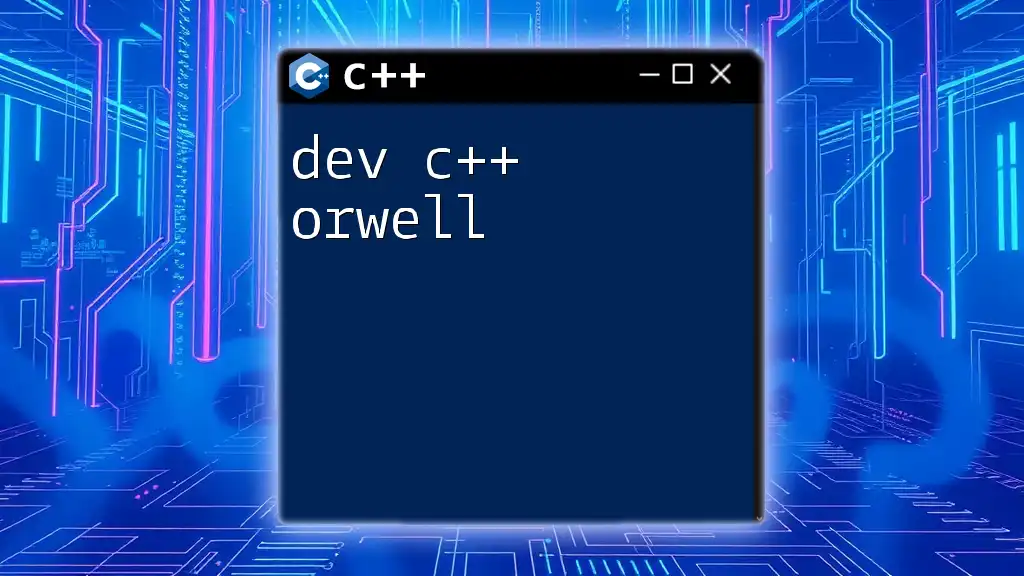
Features of Dev-C++ 5.11
Lightweight IDE
One of the standout features of Dev-C++ 5.11 is its lightweight nature. The IDE is designed to be fast and responsive, allowing you to focus on coding without being overwhelmed by resource-intensive operations. This makes Dev-C++ particularly appealing for users who may be working on older hardware or those who simply prefer a simpler environment.
User-friendly Interface
The user-friendly interface of Dev-C++ promotes ease of use, especially for beginners. The layout is intuitive, with clearly labeled menus and toolbars that guide users seamlessly through the coding process.
Integrated Compiler
At the core of Dev-C++ is its integrated MinGW GCC compiler, which offers a powerful environment for compiling and running C++ code. This integral feature eliminates the need for additional installations, allowing users to write and execute code within the same platform.
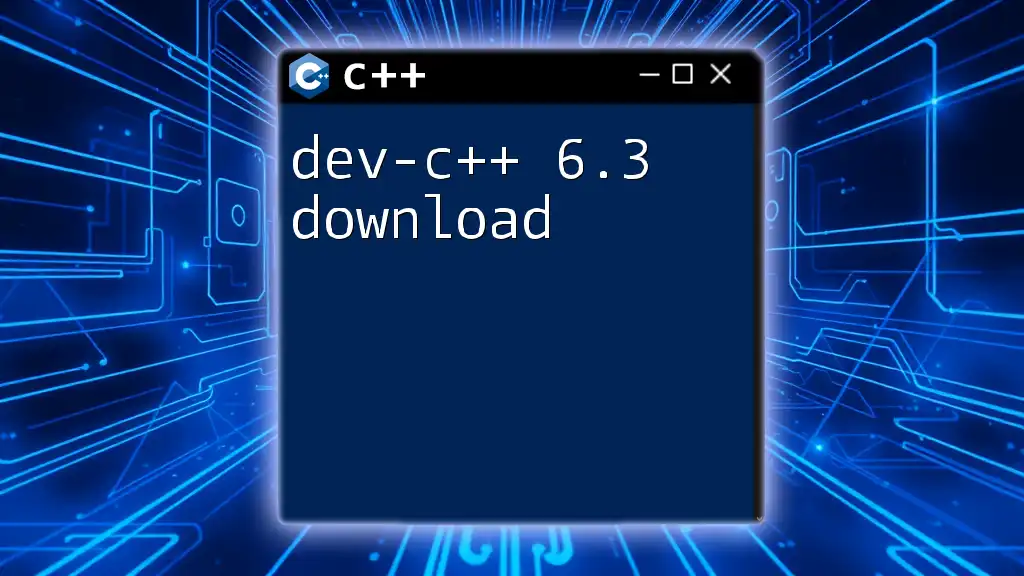
Installation of Dev-C++ 5.11
System Requirements
Before installing Dev-C++, ensure that your system meets the following requirements:
- Operating System Compatibility: Dev-C++ runs smoothly on various Windows versions, including Windows 7, 8, and 10.
- Hardware Requirements: A minimum of 1 GB RAM and a dual-core processor is recommended for optimal performance, alongside sufficient storage space on your hard drive.
Step-by-Step Installation Guide
-
Downloading Dev-C++ 5.11: You can download the latest version from the official website or trusted sources like SourceForge. Ensure you choose the right version for your operating system.
-
Installing the Software: Once downloaded, run the installer and follow the on-screen instructions. The installation process is straightforward and user-friendly.
-
First-time Setup: Upon launching Dev-C++ for the first time, you may want to configure your settings. Consider adjusting the text font and project directories for a more comfortable working environment.
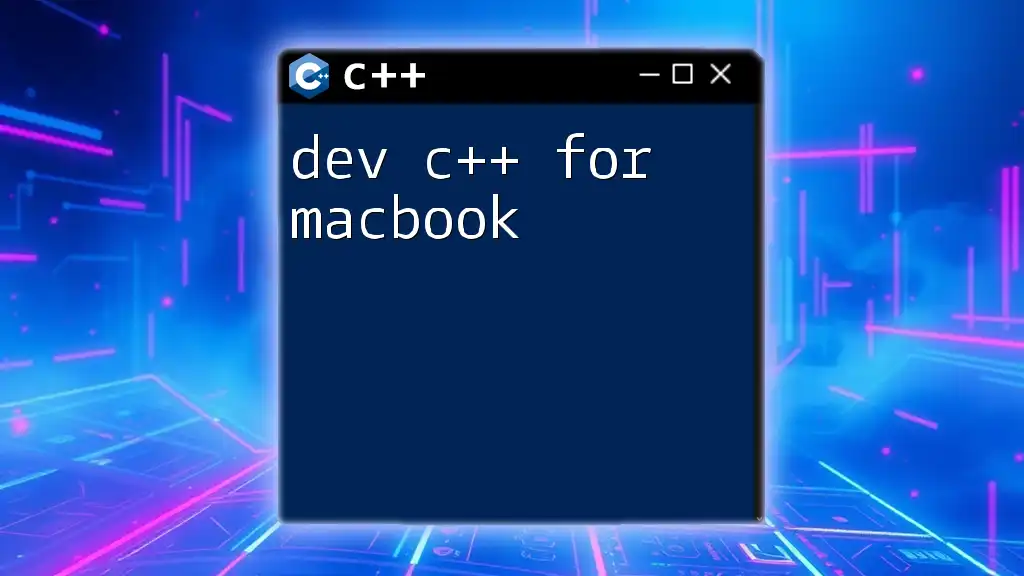
Getting Started with Dev-C++
Creating Your First Project
After successfully installing Dev-C++, it’s time to create your first project.
-
Starting a New Project: Click on `File > New > Project`. A dialog will prompt you to select a project type. For beginners, choosing a `Console Application` is recommended.
-
Choosing Project Types: Decide between a Console Application (for command-line interactions) or a Windows Application (for graphical interfaces). This choice impacts how you will write and run your code.
-
Navigating the Project Explorer: The Project Explorer pane on the left provides an overview of your project files and structure, allowing for efficient organization.
Writing Your First C++ Program
To get a feel for Dev-C++, let's write a simple C++ program that prints "Hello, World!" to the console.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Code Explanation:
- `#include <iostream>`: This line includes the iostream library, which facilitates input and output operations.
- `using namespace std;`: This directive allows you to use standard library entities without needing to prefix them with `std::`.
- `cout << "Hello, World!" << endl;`: This line outputs the text "Hello, World!" to the console, followed by a newline.
- `return 0;`: This indicates successful termination of the program.
Compiling the Program: To compile, select `Execute > Compile & Run` from the menu, or press `F9`. The IDE will alert you if there are any errors in your code.
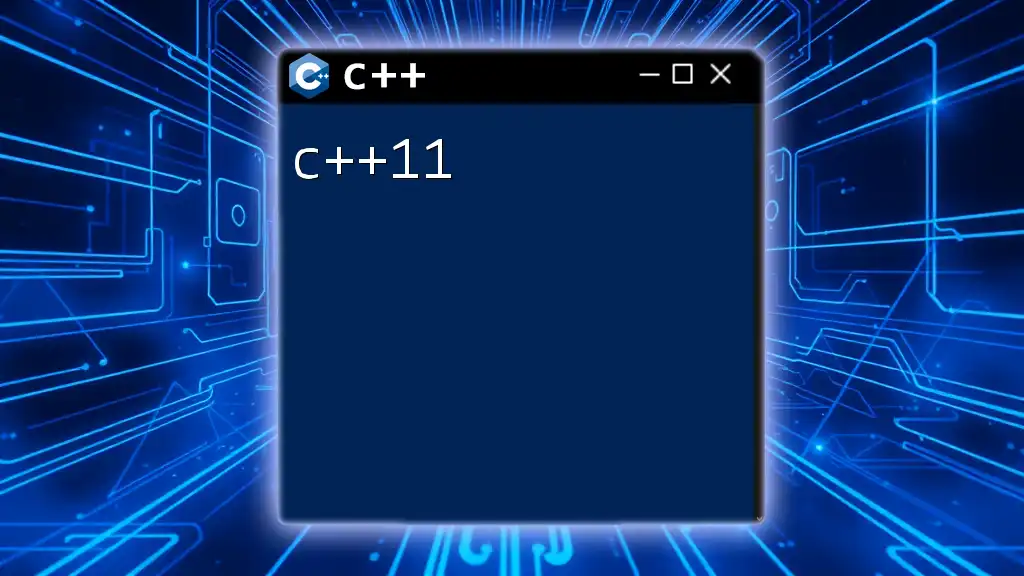
Features of Dev-C++ 5.11
Code Editing Features
The IDE boasts several beneficial features that enhance the coding experience:
-
Syntax Highlighting: Syntax highlighting improves code readability by applying different colors to keywords, types, and variables. This visual aid helps developers quickly identify coding elements.
-
Code Completion: As you type, Dev-C++ suggests completions for functions and classes, significantly reducing coding time and errors.
-
Error Checking: Real-time error checking promptly alerts you to syntax issues, allowing for immediate corrections. This feature streamlines the development process and minimizes frustration.
Debugging Tools
Effective debugging is crucial for any programming environment. Dev-C++ provides:
-
Built-in Debugger: The integrated debugger makes it easy to step through your code and examine variables.
-
Setting Breakpoints: Establish breakpoints to pause execution at specific points in your code. This allows you to inspect the state and determine what’s happening at each step.
-
Watch Variables and Call Stack: Utilize watch variables to monitor specific data points in your program. The call stack tool shows the function calls made, helping you trace the path of execution.
Customization Options
To enhance your experience:
-
Themes and Fonts: You can adjust the appearance of Dev-C++ by selecting themes and fonts that suit your style and improve readability.
-
Shortcut Keys: Learn the various keyboard shortcuts—such as `Ctrl + S` to save and `Ctrl + N` to create a new file. Familiarizing yourself with these shortcuts can greatly enhance your productivity.
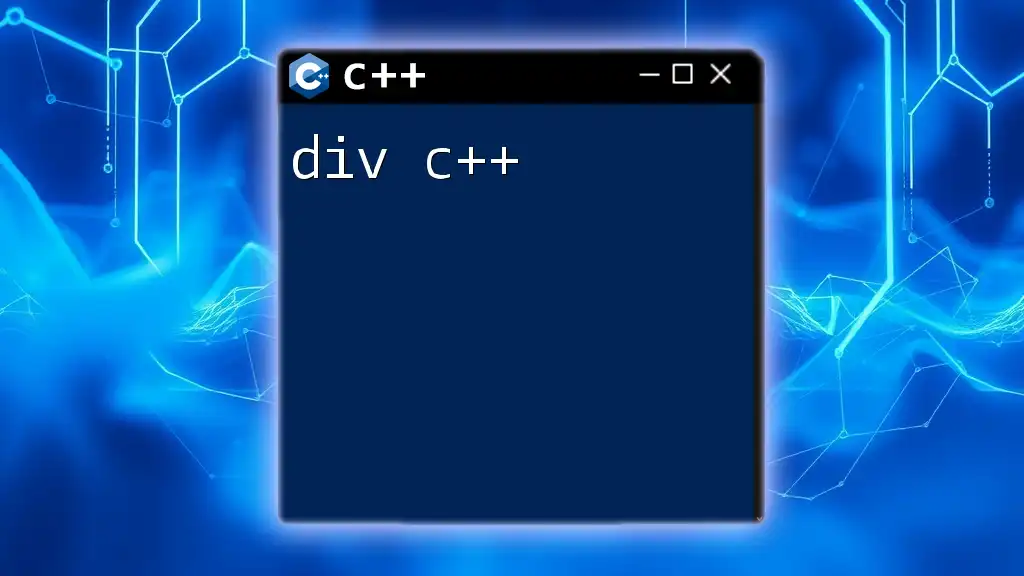
Working with Libraries in Dev-C++ 5.11
Importing Libraries
Expanding your C++ capabilities often requires external libraries.
- Standard Libraries: You can include common libraries with simple commands:
#include <cmath> // For mathematical functions
- Third-Party Libraries: Many open-source libraries are available online. Follow installation instructions on the library’s website for successful integration into your projects.
Linking Libraries
Linking libraries can significantly enhance your application's functionality.
-
Static vs Dynamic Linking: Static linking incorporates the library directly into the executable file, while dynamic linking references external library files. Choose based on your project's needs and complexity.
-
Setting up Library Paths: Navigate to `Tools > Compiler Options > Directories > Libraries` to add the paths for your linked libraries, ensuring the compiler can find them easily.
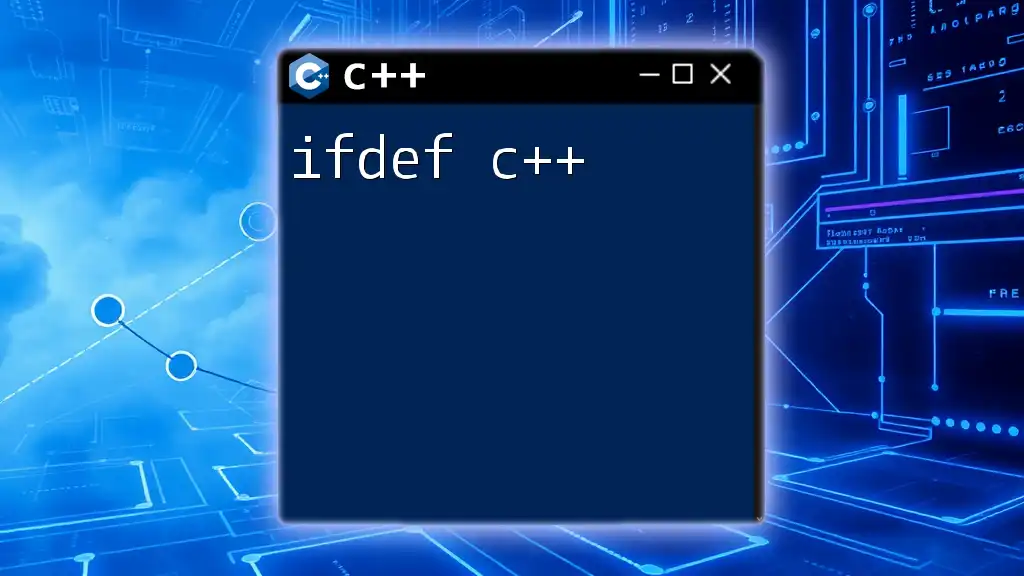
Best Practices for Using Dev-C++
Code Organization
Maintaining an organized codebase is essential for efficient development.
-
File Naming Conventions: Use meaningful names for files that reflect their content or functionality. Avoid generic labels to improve clarity and maintainability.
-
Folder Structure: Organize your project into folders by functionality, such as separating `source files`, `header files`, and `resources`. This structure simplifies navigation and collaboration.
Version Control
Employing version control systems is pivotal in programming.
-
Importance of Version Control: It allows tracking changes, reverting to previous versions, and facilitating collaboration among team members.
-
Using Git with Dev-C++: Integrating Git enables you to maintain a history of your coding projects. Basic commands like `git init`, `git add`, and `git commit` are vital for managing your project's evolution.
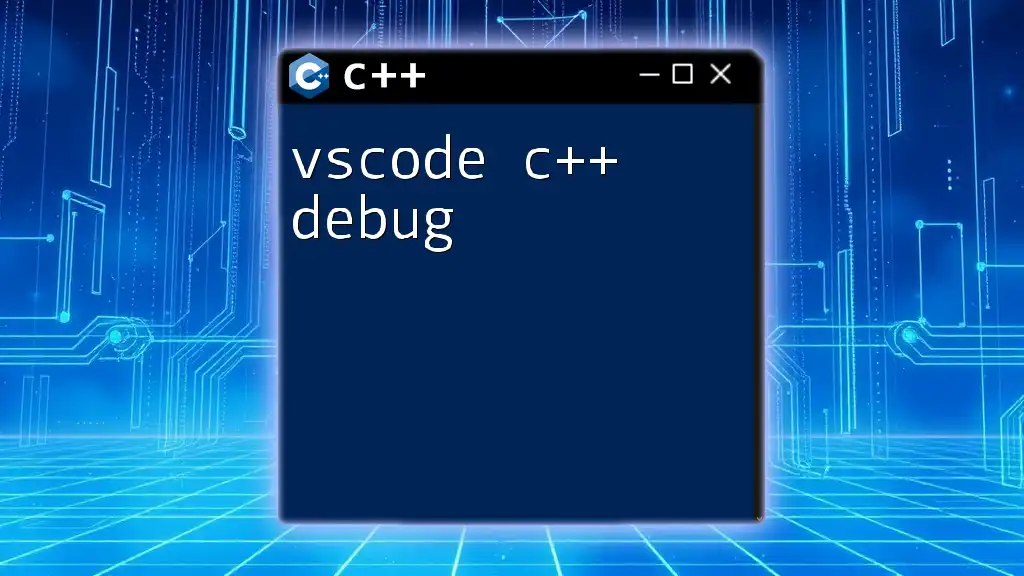
Troubleshooting Common Issues
Installation Issues
Sometimes, users may encounter installation hiccups.
-
Installation Failures: If an error message appears during installation, ensure that your operating system is compatible and that you have the required permissions to install software.
-
Compatibility Problems: Should you experience issues, consult online forums or the Dev-C++ user community for advice on resolving conflicts related to outdated libraries or settings.
Programming Errors
Throughout your programming journey, you’ll encounter various errors.
-
Compilation Errors: Read the compiler’s error messages carefully. They often indicate the line number and type of error, guiding you toward a solution.
-
Logical Errors: These errors may not surface during compilation. Utilize the debugging tools within Dev-C++ to step through your code and assess variable states to identify and rectify mistakes.
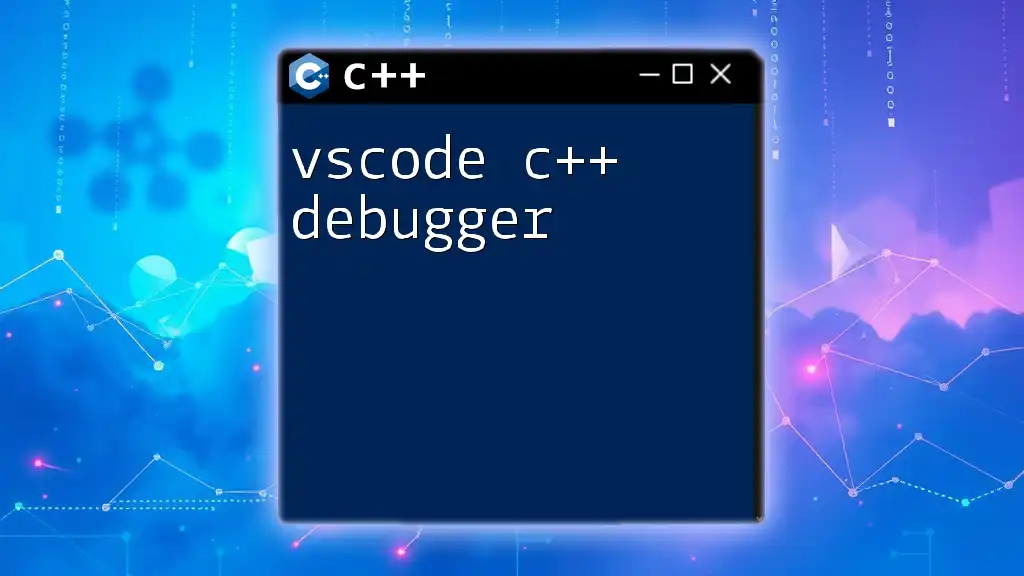
Conclusion
In summary, Dev-C++ 5.11 stands out as a powerful yet user-friendly IDE for C++ programming. Its range of features—from its lightweight nature to the integrated compiler—enhances the development experience for all users. As you explore more complex projects, remember to stay organized, employ version control, and utilize the robust debugging tools to improve your coding journey. Happy coding!