C++11 is a significant update to the C++ programming language that introduced features like auto type deduction, range-based for loops, and lambda expressions, enhancing both usability and performance.
Here's a code snippet demonstrating the use of a lambda expression in C++11:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n * n << " "; // Print square of each number
});
return 0;
}
What is C++11?
C++11 is a significant update to the C++ programming language that introduced numerous powerful features aimed at improving both the functionality and performance of the language. It was released as the first major revision since the C++03 standard, and it was designed to make C++ more accessible to programmers, promote modern programming paradigms, and enhance the language's ability to create robust and high-performance applications.
Historically, as software development became increasingly complex, the need for enhancements to C++ became clear. C++11 addressed developer demands for better memory management, ease of use, and improved performance capabilities.
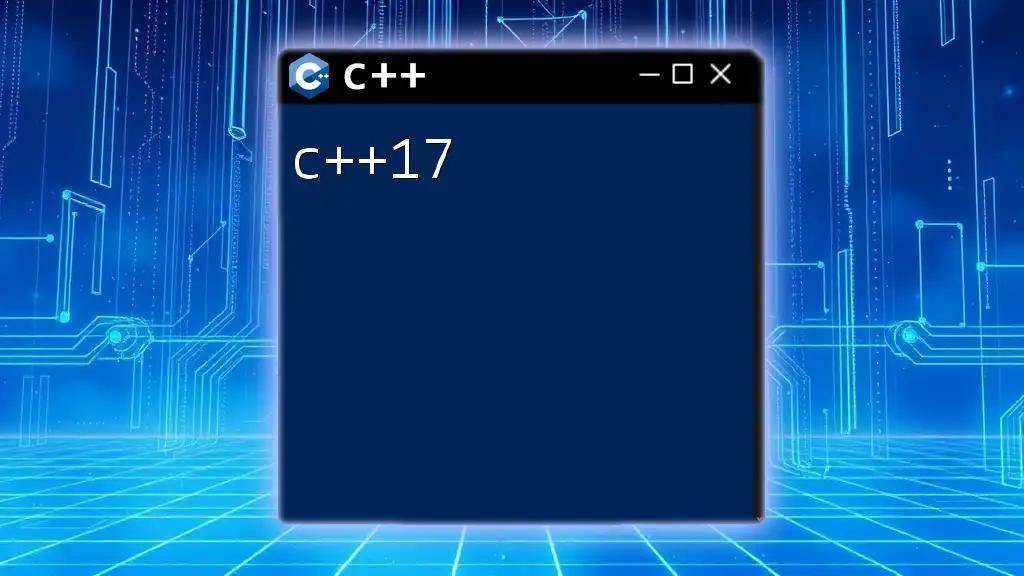
Major Features of C++11
New Language Features
Auto Keyword
The `auto` keyword is a cornerstone of C++11 that enables type inference. This allows developers to avoid specifying variable types explicitly, making code cleaner and more concise.
For example:
auto x = 5; // x is deduced as int
auto y = 3.14; // y is deduced as double
Here, `auto` simplifies declarations, especially for complex types, and helps prevent type mismatches, making the code both safer and easier to read.
Range-Based For Loops
C++11 introduced range-based for loops, which provide a simpler syntax for iterating over containers such as arrays and vectors. This new syntax improves code readability and reduces the chances of off-by-one errors.
Example:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
Using the range-based for loop, you can iterate through all elements in a container without worrying about iterators or indices.
Lambda Expressions
Lambda expressions bring an accessible way to define anonymous functions right in the place where they are needed. This feature is useful for callback functions, standard algorithms, and more.
Example:
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Outputs 8
The above code snippet defines an `add` lambda function, which can be called inline, enhancing code readability and maintainability.
Smart Pointers
Memory management is one of the more challenging aspects of C++. C++11 introduced smart pointers, specifically `std::unique_ptr` and `std::shared_ptr`, to help manage memory automatically and minimize memory leaks.
Example:
std::unique_ptr<int> ptr = std::make_unique<int>(10);
Using smart pointers not only simplifies the management of dynamic allocations but also makes code safer, reducing the chances of memory leaks and dangling pointers.
Concurrency Support
Thread Library
C++11 introduced a comprehensive thread library, making it easier to write multi-threaded programs. With threads, developers can execute multiple functions simultaneously, improving performance in CPU-bound applications.
Example:
std::thread t([] { std::cout << "Hello from thread!\n"; });
t.join();
In this snippet, a new thread is created that executes a simple function. Using `join()` ensures that the main thread waits for the new thread to finish before proceeding, maintaining program integrity.
Mutex and Locks
With multi-threading comes the need for thread safety. C++11 introduced `std::mutex` to allow multiple threads to coordinate access to shared resources.
Example:
std::mutex mtx;
void safePrint() {
std::lock_guard<std::mutex> lock(mtx);
std::cout << "Thread-safe print\n";
}
In this code, `std::lock_guard` ensures that the mutex is locked during the execution of `safePrint()`, preventing data races and ensuring thread-safe operations.
Enhanced Standard Libraries
Regular Expressions
C++11 added support for regular expressions, enabling developers to perform complex pattern matching and text processing tasks more efficiently.
Example:
std::regex reg("(\\d+)");
std::string s = "There are 42 apples.";
std::smatch match;
std::regex_search(s, match, reg);
In this example, `std::regex` is utilized to search for digit patterns in a string, significantly simplifying text parsing operations.
Random Number Generation
The introduction of a new random number library in C++11 allows for sophisticated random number generation, making it easier to create applications that require randomness, like games or simulations.
Example:
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> distrib(1, 6);
std::cout << distrib(gen); // Random number between 1 and 6
This code showcases how easy it is to generate random numbers using the new libraries, improving both performance and ease of use.

Benefits of C++11
C++11 brings about numerous enhancements that can significantly improve productivity, performance, and resource management for developers.
Increased Productivity: By simplifying syntax, offering powerful features, and reducing boilerplate code, C++11 allows developers to write clearer and more efficient code in less time.
Improved Performance: Many of the new features—such as move semantics—allow for better resource management and optimization, leading to faster execution times and lower resource consumption.
Better Resource Management: The introduction of smart pointers means that developers can focus on writing functionality rather than worrying about memory management, which often leads to bugs and vulnerabilities.

Common Use Cases for C++11
Game Development
C++11's threading support and ease of use in managing resources make it particularly attractive for game development, where performance is critical and complex game systems need to run efficiently.
Systems Programming
Moreover, C++11's powerful standard library features and concurrency improvements make it a preferred choice for systems programming, especially in developing servers or high-performance applications that require efficient management of resources.

How to Get Started with C++11
Setting Up Your Development Environment
To utilize C++11, developers should ensure they are using an IDE or compiler that supports it. Popular choices include Microsoft Visual Studio, GCC, and Clang, all of which have built-in support for C++11 standards.
Practice Resources
For those interested in delving deeper into C++11, numerous resources are available, including online tutorials, comprehensive books dedicated to the C++ language, and platforms like Codecademy or Udacity that offer interactive learning experiences.

Conclusion
C++11 represents a pivotal advancement in the C++ programming world, addressing many of the challenges faced by developers. Its powerful features promote efficient coding standards, simplify tasks, and enhance resource management. As the C++ language continues to evolve, seeing how this version laid a strong foundation for future enhancements, such as C++14 and C++17, can inspire ongoing learning and adaptation in this ever-changing landscape.
By embracing C++11, developers are well-equipped to leverage modern programming paradigms, making the most of their coding efforts. It's an exciting time to explore and implement C++11 features in both legacy applications and new projects.