AUTOSAR C++14 is a set of guidelines designed to promote best practices in C++ programming for automotive software development, focusing on safety and maintainability while leveraging features of the C++14 standard.
Here’s a code snippet that demonstrates a simple class definition adhering to AUTOSAR C++14 guidelines:
#include <iostream>
class Vehicle {
public:
Vehicle(const std::string& name) : name_(name) {}
void Display() const {
std::cout << "Vehicle Name: " << name_ << std::endl;
}
private:
std::string name_;
};
int main() {
Vehicle car("Toyota");
car.Display();
return 0;
}
Understanding the Basics of AUTOSAR
What is AUTOSAR?
AUTOSAR, or Automotive Open System Architecture, is a global development partnership of automotive interested parties. Its main objective is to standardize the software architecture of vehicle systems, ensuring that they are reusable, scalable, and adaptable across different manufacturers and projects. The architecture is divided into three main layers: the Application Layer, the Runtime Environment (RTE), and the Basic Software Layer. Each layer has its specific functions, which facilitate communication and operational harmony in electronic control units (ECUs).
Importance of C++ in AUTOSAR Development
C++ serves as a cornerstone in modern automotive software development. Its object-oriented features enable developers to model complex systems effectively. Additionally, the language offers high performance, which is crucial for applications that require real-time processing, such as those found in safety-critical automotive systems.
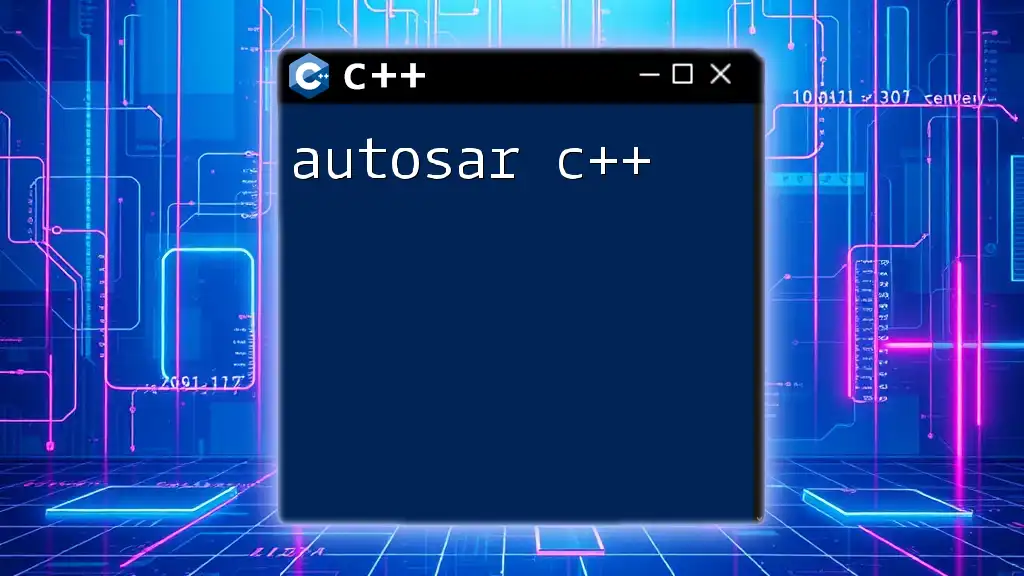
Overview of C++14 Features
New Features in C++14
C++14 introduced various enhancements and new features that are particularly beneficial for developers working with AUTOSAR. Some significant additions include:
- Generic Lambdas: These allow the use of `auto` in parameter lists, making lambda expressions more flexible.
auto lambda = [](auto x, auto y) { return x + y; };
- User-Defined Literals: These enhance the expressiveness of code and allow developers to create custom literals.
constexpr long double operator"" _km(long double val) {
return val * 1000.0;
}
- Enhanced Return Type Deduction: Developers can use `auto` for return types in functions, simplifying code maintenance and readability.
auto multiply(double x, double y) {
return x * y;
}
Benefits of C++14 for AUTOSAR
By integrating C++14 into AUTOSAR systems, developers can take advantage of enhanced safety features, improved performance, and reduced code complexity. For instance, the introduction of generic lambdas allows for more reusable and adaptable code components, which is a significant advantage in the context of the modular design of AUTOSAR.
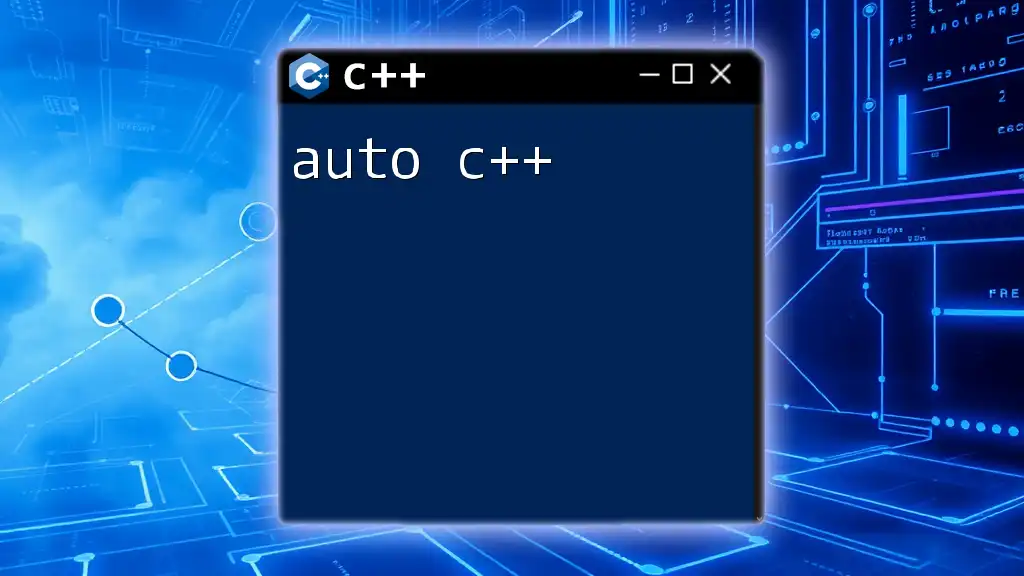
Implementing C++14 in AUTOSAR
Understanding AUTOSAR C++14 Guidelines
The AUTOSAR C++14 guidelines promote safe and effective coding practices. These guidelines address the specific needs of automotive software, focusing on memory safety, runtime error handling, and the avoidance of undefined behavior.
Key Guidelines for Safe Coding
Maintaining code safety is crucial in AUTOSAR applications due to their often safety-critical nature. The guidelines recommend avoiding certain language features that could lead to potential runtime errors. For example, the use of pointers can lead to bugs and vulnerabilities, and developers are encouraged to leverage smart pointers instead.
Code Example: Contrast between 'safe' and 'unsafe' practices in C++14:
// Unsafe: raw pointer
int* p = new int(10);
delete p;
// Safe: smart pointer
#include <memory>
std::unique_ptr<int> p = std::make_unique<int>(10);
Memory Management in C++14
Effective memory management is crucial in embedded systems, where resources are limited. C++14 introduces `std::unique_ptr` and `std::shared_ptr`, which help manage memory dynamically by ensuring that resources are correctly released.
Code Snippet: Demonstrating smart pointers in action:
#include <memory>
void example() {
std::unique_ptr<int[]> p(new int[10]); // unique_ptr for an array
p[0] = 1; // Safe access
} // Automatically deallocated
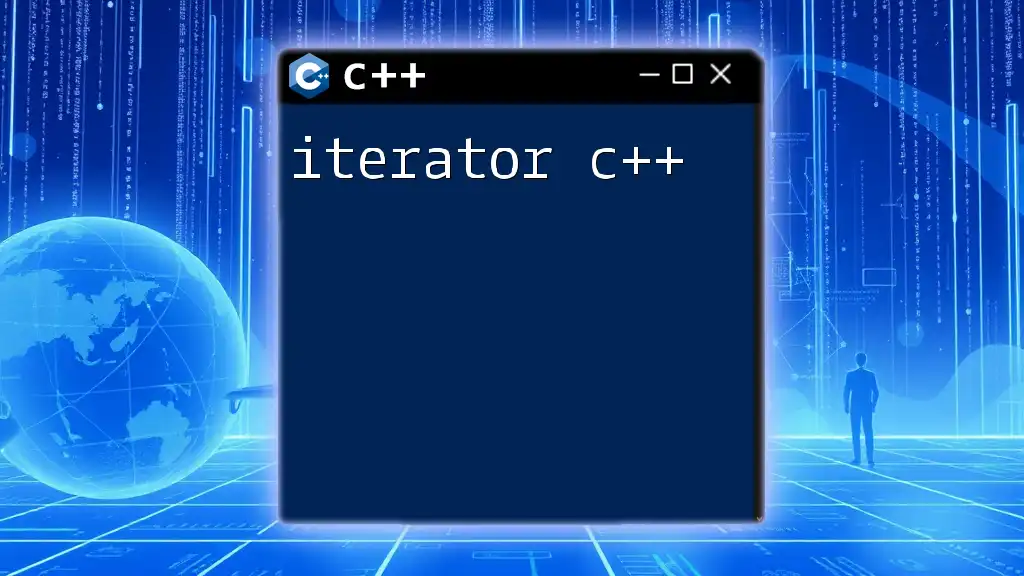
Application Scenarios
Using C++14 to Develop AUTOSAR Software Components
To develop a sample AUTOSAR software component using C++14, you would typically start by defining a clear architecture according to AUTOSAR guidelines. This would involve creating modules for communication, diagnostics, and other functionalities, followed by implementing them in C++14.
The development environment may include tools like Eclipse with the AUTOSAR plug-in, enabling developers to design and visualize system architecture effectively.
Case Study: C++14 in Real Automotive Projects
Some automotive projects have successfully integrated C++14 within the AUTOSAR infrastructure. These projects showcased the enhanced capabilities of C++14, such as using generic lambdas for event handling in the RTE. The developers faced challenges related to legacy C++ codebases, which required careful adaptation to C++14 features. The key takeaway was that modernizing code led to improved performance and maintainability.
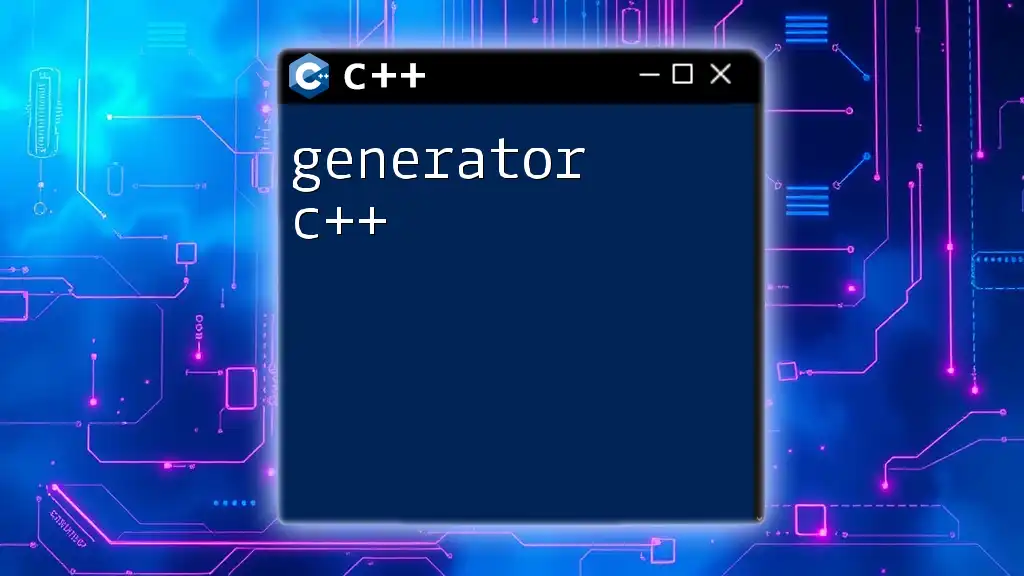
Testing and Validation
Best Practices for Testing AUTOSAR with C++14
Incorporating robust testing methodologies needs to be a priority when using C++14 in AUTOSAR projects. Unit testing is essential for verifying that individual components function as intended, while integration testing ensures that all parts of the system work together correctly. Tools like Google Test can facilitate this.
Verification of Safety Standards
The automotive industry is heavily regulated, making compliance with safety standards such as ISO 26262 crucial. Using C++14 with AUTOSAR requires a focus on writing safe and reliable code. These standards often demand rigorous testing and validation processes, which can be streamlined with the best practices highlighted in the AUTOSAR C++14 guidelines.
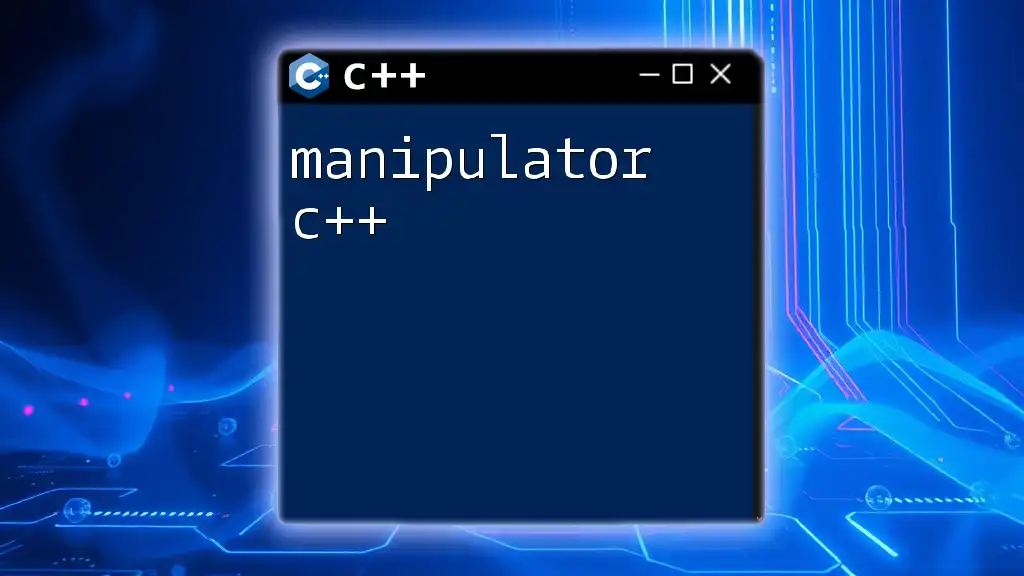
Conclusion
Integrating C++14 into the AUTOSAR framework enhances safety, efficiency, and maintainability of automotive software systems. By leveraging the features of C++14, including generic lambdas and smart pointers, developers can create robust applications that meet the demands of modern automotive technology. As the industry evolves and new C++ standards emerge, understanding and applying these practices will be pivotal for the success of future automotive projects.
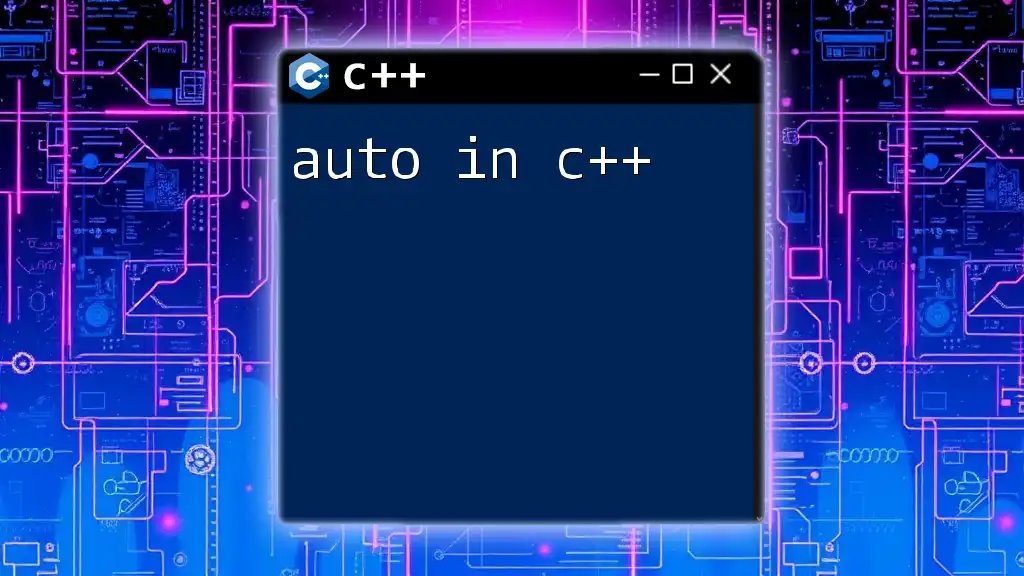
Call to Action
Explore the AUTOSAR C++14 guidelines further to enhance your development practices. If you're eager to dive deeper into implementing effective C++ strategies in automotive software, consider joining our courses designed to equip you with essential skills for success in this field.
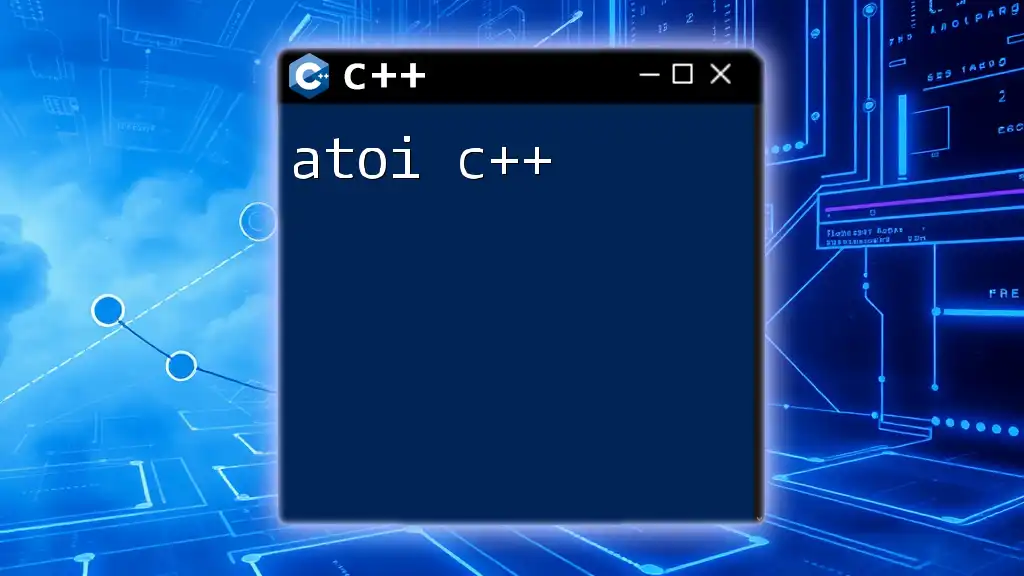
References and Further Reading
For those interested in learning more about AUTOSAR and C++14, refer to official AUTOSAR documentation, C++14 resources, and additional literature on automotive software development best practices.