AUTOSAR C++ is a set of guidelines and standards designed to improve the safety, reliability, and maintainability of C++ software in automotive applications.
Here’s a simple code snippet illustrating a basic class definition in C++ following AUTOSAR practices:
// Example of a simple class in accordance with AUTOSAR C++ guidelines
class Vehicle {
public:
Vehicle(int wheels) : m_wheels(wheels) {}
int getWheels() const {
return m_wheels;
}
private:
int m_wheels;
};
Understanding the Basics of Autosar
What is AUTOSAR?
AUTOSAR, which stands for "AUTomotive Open System ARchitecture," is a global initiative aimed at standardizing the software architecture of automotive systems. Its purpose is to create a common development platform that fosters collaboration among manufacturers, suppliers, and partners, minimizing development costs while increasing flexibility and efficiency.
The importance of AUTOSAR in the automotive industry cannot be overstated. As cars become increasingly reliant on software, the need for a standardized approach to software integration, management, and application development emerges. AUTOSAR addresses these requirements by ensuring that different components can easily communicate and be integrated into a cohesive vehicle architecture.
Overview of the Autosar Standards
AUTOSAR comprises two primary platforms: Classic Platform and Adaptive Platform. The Classic Platform is designed for embedded systems with real-time constraints, primarily serving applications with minimal hardware resources. In contrast, the Adaptive Platform caters to high-complexity applications like automated driving, offering greater flexibility and resource management.
C++ plays a significant role in both platforms, allowing developers to write efficient, object-oriented code that is easy to maintain, understand, and transfer across different projects and teams.
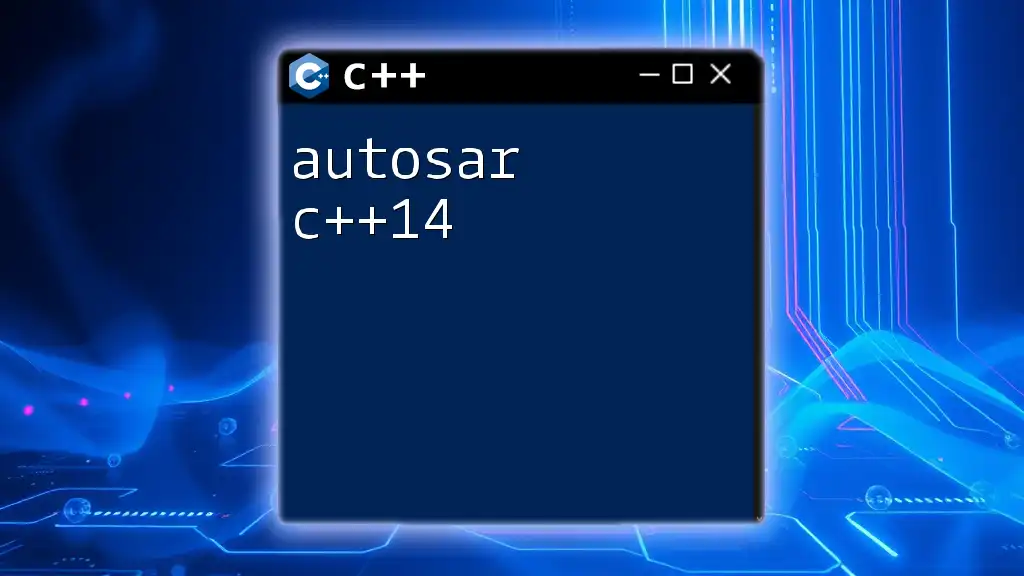
C++ in Autosar: An Overview
Why Use C++ in Automotive Applications?
One of the fundamental reasons to incorporate C++ in automotive applications is its inherent performance and efficiency. C++ allows for low-level memory management, enabling developers to fine-tune applications to maximize speed and resource utilization. This is crucial in environments where performance is key, such as in real-time systems.
Key Features of C++ in Autosar Applications
Object-oriented Programming
C++ is fundamentally an object-oriented programming language, enabling developers to model real-world entities as classes and objects. This leads to:
- Modularity: Breaking down the application into smaller components, making it easier to manage and optimize.
- Reuse: Allowing for the reuse of code across different projects, reducing redundancy.
- Encapsulation: Enhancing security by bundling data and methods into a single unit.
In an automotive context, consider a Vehicle class that could have subclasses like Car and Truck, each inheriting properties from its parent class, thus promoting code reuse and logical organization.
Templates and Generic Programming
C++ templates enable developers to write generic functions and classes, facilitating code reuse and flexibility. This allows developers to implement algorithms or data structures without committing to specific data types. For example, consider a template for a sorting function:
template <typename T>
void sort(T array[], int size) {
// Sorting logic here
}
When implementing this in an AUTOSAR project, a developer can apply the same sorting function for both int and float arrays without rewriting the code.
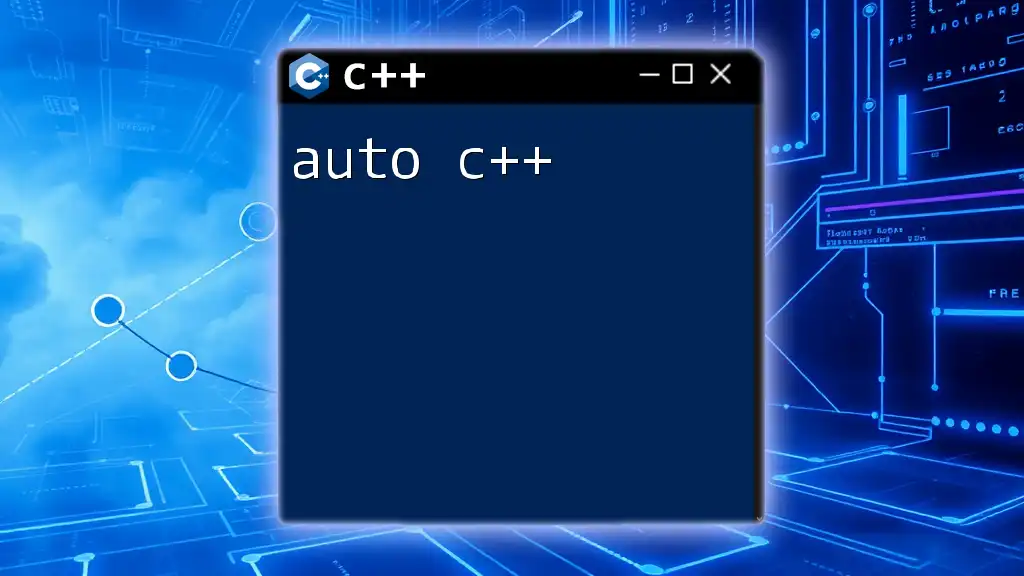
Key C++ Concepts for Autosar
C++ Language Features
Advanced Data Structures
Effective data management is critical in automotive applications. C++ provides various advanced data structures, such as:
- Vectors: Dynamic arrays that grow as needed, ideal for scenarios where the size of the dataset is unknown at compile time.
- Maps: Associative containers that store key-value pairs, useful for quick data retrieval based on a unique key.
Example of using a vector to manage vehicle sensors:
#include <vector>
class Sensor {
public:
void readData();
// Additional sensor methods
};
std::vector<Sensor> sensors;
Smart Pointers
In modern C++, smart pointers such as `std::unique_ptr` and `std::shared_ptr` are recommended over raw pointers. They automatically manage memory, ensuring that resources are freed when no longer needed, thereby reducing memory leaks. Here's a sample code snippet showing a unique pointer usage:
#include <memory>
class Vehicle {
public:
Vehicle() { /* Constructor */ }
~Vehicle() { /* Destructor */ }
};
std::unique_ptr<Vehicle> myVehicle = std::make_unique<Vehicle>();
Exception Handling in C++
In automotive systems, where safety is paramount, exception handling becomes essential. It allows a program to deal with unexpected events gracefully, preventing crashes or erratic behaviors.
try {
// Code that may throw an exception
throw std::runtime_error("Error occurred");
} catch (const std::runtime_error& e) {
std::cerr << "Caught runtime error: " << e.what() << std::endl;
}
In AUTOSAR, careful implementation of exception handling is needed to ensure that critical systems continue to operate in the face of unexpected situations.
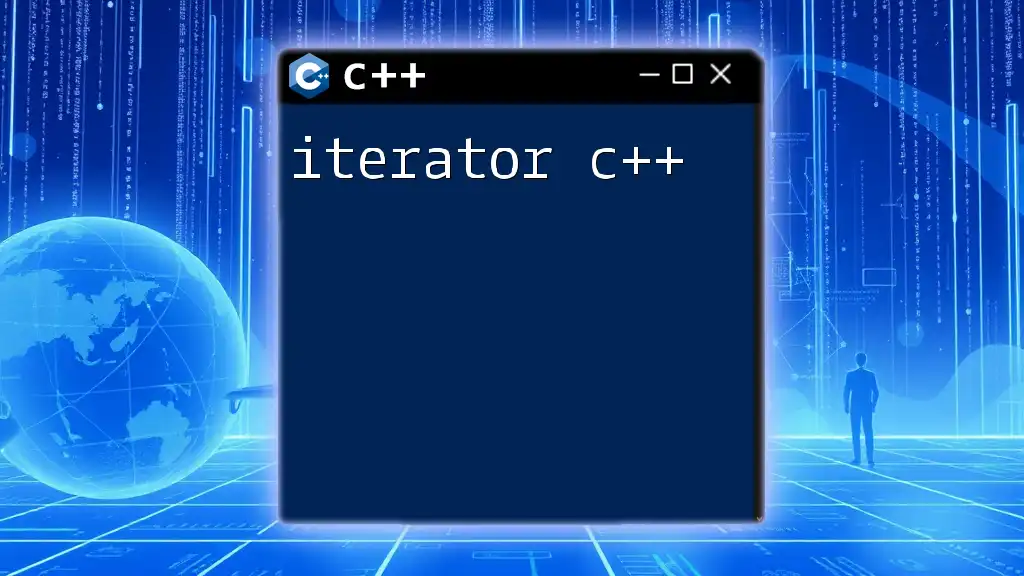
Implementing C++ in Autosar
Setting Up the Environment
Before diving into actual coding, it’s essential to have the right development environment set up. Popular IDEs like Eclipse and Visual Studio are widely used for C++ development. You’ll also need to integrate the relevant AUTOSAR tools, libraries, and frameworks to support your development process.
Building a Sample Autosar C++ Application
One of the best ways to learn is through hands-on experience. Here’s a simplified step-by-step guide to creating a basic software component in an AUTOSAR application.
Creating a Simple Software Component
- Define the Software Component (SWC): Create header files for your class definitions.
// Vehicle.h
class Vehicle {
public:
void start();
void stop();
};
- Implement Class Methods: Provide implementations for your class methods in the corresponding `.cpp` file.
// Vehicle.cpp
#include "Vehicle.h"
void Vehicle::start() {
// Start vehicle logic
}
void Vehicle::stop() {
// Stop vehicle logic
}
- Configuration Files: Define XML configuration files for AUTOSAR-specific settings that describe the component's interfaces and behavior.
Integrating with the RTE (Runtime Environment)
After defining your software component, integration with the Runtime Environment (RTE) is crucial. The RTE serves as the middleware that efficiently manages communication between software components and hardware.
Integration typically involves configuring your RTE to acknowledge your new SWC, which includes creating a configuration file that outlines how your component interacts with other components.
Testing and Debugging C++ Applications in Autosar
Importance of Testing in Automotive Software
Due to the critical nature of automotive applications, testing is not just a suggested step; it is mandatory. When working with autosar c++, rigorous testing methodologies such as unit testing, integration testing, and system testing should be employed to ensure the software operates correctly under various conditions.
Debugging Tips and Tools
Debugging is an integral part of software development. Tools tailored for AUTOSAR applications can assist in identifying and resolving issues effectively. Utilizing debugger programs integrated within IDEs, such as GDB for C++, enables step-by-step execution and inspection of variables throughout the runtime.
A practical approach involves using assertions to validate assumptions in your code, which can significantly aid in debugging.
#include <cassert>
void processVehicleData(int data) {
assert(data >= 0); // Ensure data is non-negative
// Process data
}
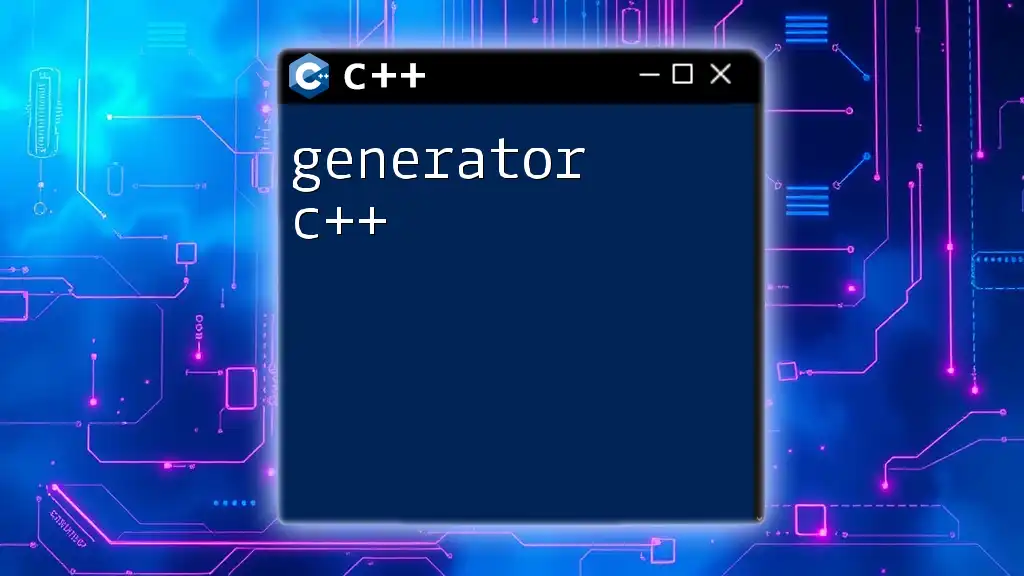
Best Practices for C++ Development in Autosar
Code Standards and Guidelines
To maintain code quality in autosar c++ projects, adherence to established coding standards is crucial. Popular standards include MISRA C++ and AUTOSAR coding guidelines. These standards provide rules and recommendations that help developers write clean, safe code, reducing the likelihood of defects.
Safety and Security Considerations
In the automotive domain, safety is paramount. Applying best practices in security measures in your C++ code ensures that your application is resilient against various attacks and vulnerabilities, thus protecting both the software and users.
Performance Optimization Techniques
In performance-critical applications, profiling and optimization become essential. Effective profiling techniques help identify performance bottlenecks. Common methods include:
- Benchmarking: Measure the performance of specific parts of the code.
- Code Analysis Tools: Use tools to analyze execution paths and memory usage to identify inefficiencies.
Here’s an example for optimizing a simple loop by using the Reserve method for vectors:
std::vector<int> numbers;
numbers.reserve(100); // Reserve space for 100 elements to avoid reallocations
for (int i = 0; i < 100; ++i) {
numbers.push_back(i);
}
Resource Management
Efficient resource management is vital. Always identify appropriate data scopes and lifetime management to minimize memory overhead and ensure that system resources are used effectively. C++ offers various features such as smart pointers, which automatically handle memory cleanup.
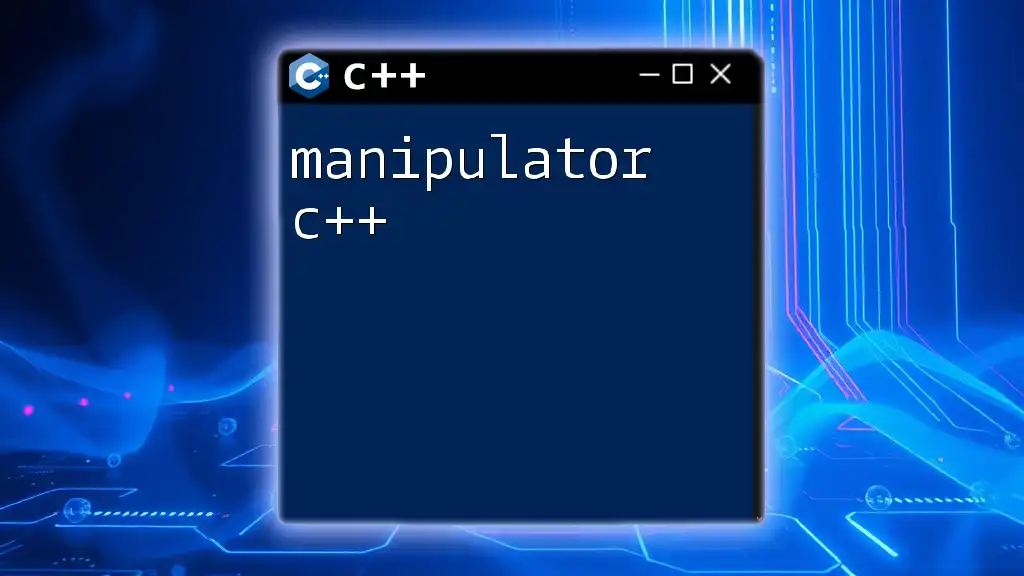
Common Challenges and Solutions
Challenges in Using C++ within Autosar
Despite its advantages, using C++ within an AUTOSAR framework presents challenges, especially regarding integration complexity and maintaining compliance with varied standards.
Solutions and Workarounds
To tackle integration challenges, developers can rely on modular programming principles that encourage separation of concerns. For compliance, consistently following established guidelines and leveraging code standards can help in navigating obstacles and ensuring code safety and reliability.
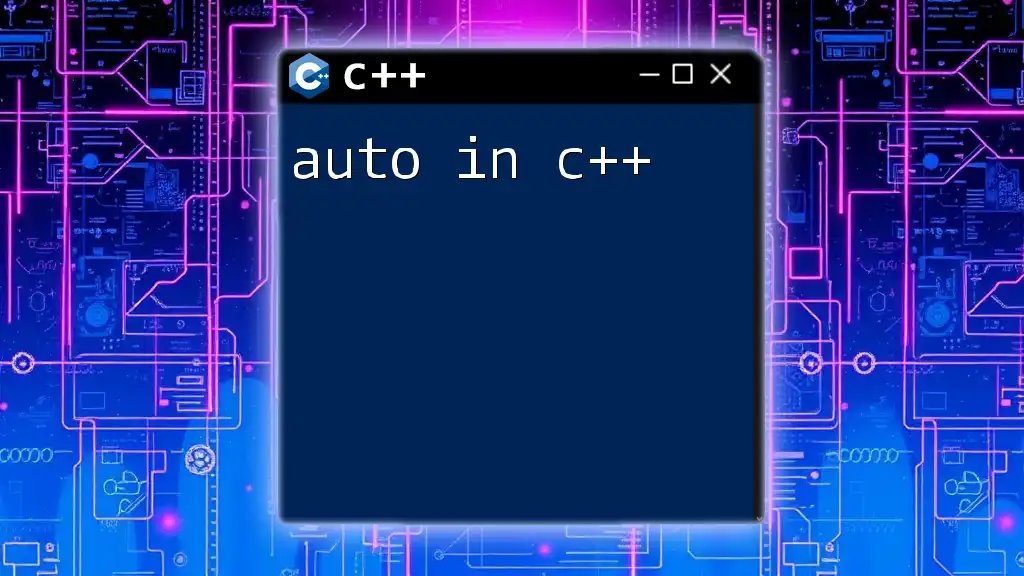
Conclusion
The use of autosar c++ has significant implications for the development of automotive systems. By leveraging C++ features, developers can create robust, high-performance applications that meet the increasing demands of modern vehicles. As the automotive landscape continues to evolve towards greater automation and digitalization, understanding and applying these principles will remain crucial for future developers navigating the complex world of AUTOSAR.
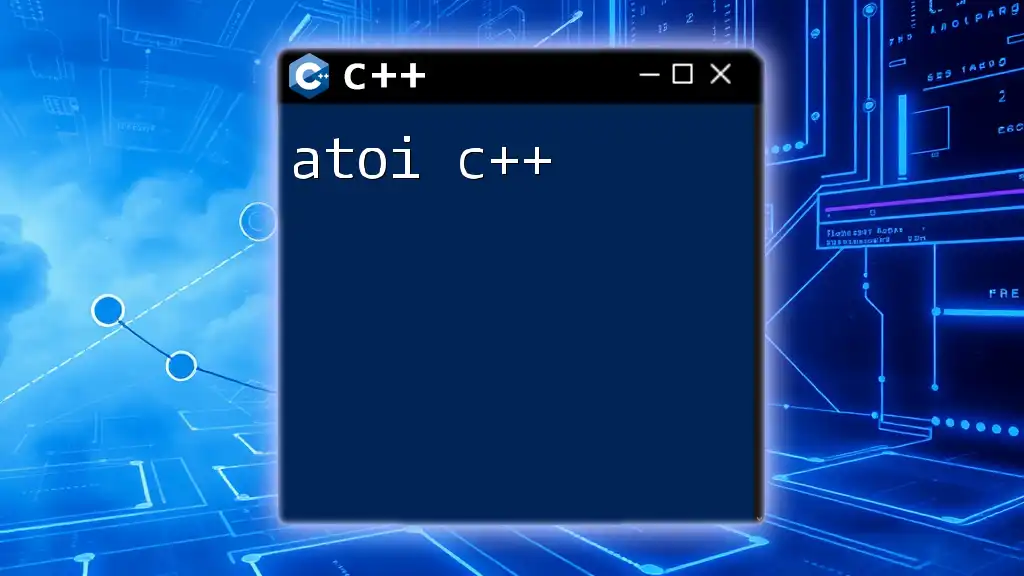
Additional Resources
To deepen your understanding, consider exploring textbooks, scholarly articles, and online tutorials specializing in AUTOSAR and C++. Engaging with communities and forums where professionals share insights and experiences can also provide invaluable knowledge in this rapidly evolving field.