Atom is a customizable text editor that supports C++ development with features like syntax highlighting, and users can enhance their programming experience by using packages specifically designed for C++.
Here's a simple C++ code snippet that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Atom for C++ Development
Installing Atom
To kickstart your coding journey, the first step is downloading Atom, a versatile and customizable text editor perfect for C++ development. Head over to [Atom's official website](https://atom.io/) and download the installer compatible with your operating system.
Be sure to check the following system requirements to ensure smooth installation:
- Minimum Requirements:
- 2GB RAM
- 300MB disk space
- Recommended Requirements:
- 4GB RAM or more
- SSD for improved performance
Configuring Atom for C++
Once you have Atom installed, it's essential to configure it for optimal C++ development. Adding must-have packages can significantly enhance your programming experience.
Must-Have Packages
Atom has a vibrant community that contributes several packages designed to improve code writing and management. Here are two essential packages:
-
gpp-compiler: This package allows you to compile and run C++ code directly from the Atom editor. To install:
- Open Atom
- Go to `Settings > Install`
- Search for gpp-compiler and click install.
-
script: It allows you to run scripts directly in Atom, offering a seamless development experience. Installation is similar to gpp-compiler.
Customizing Your Environment
Customizing your Atom environment enhances your coding experience. Consider the following:
-
Themes: Choose a theme that is easy on the eyes and promotes productivity. Look into popular themes by visiting Atom's community packages.
-
Fonts and Styling: Adjust the font size and type according to your preference. A clean and readable font can make a huge difference in your coding sessions.
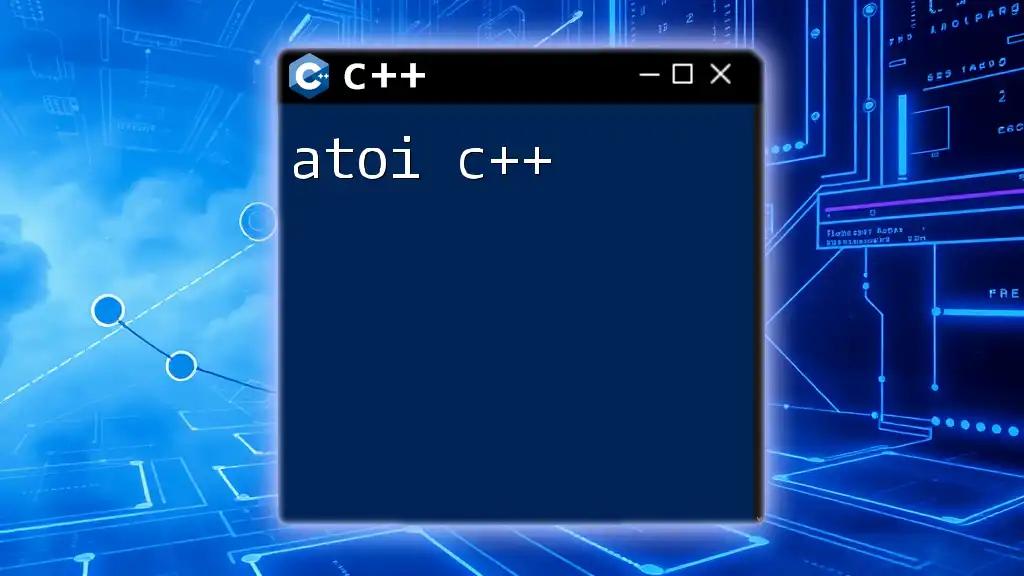
Writing Your First C++ Program in Atom
Creating a New C++ File
Creating a new C++ file is straightforward. Click on `File > New File` and save the file with a `.cpp` extension.
Adhering to file naming conventions is crucial. Use descriptive names (e.g., `hello_world.cpp`) to make it easier to identify the purpose of the file later.
Entering Your Code
When you start coding, it’s helpful to understand the basic structure of a C++ program. Here’s a simple example to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the Code:
- `#include <iostream>`: This line includes the input-output stream library necessary for output functionality.
- `int main()`: This marks the beginning of the main function.
- `std::cout << "Hello, World!" << std::endl;`: This line prints the string to the console.
- `return 0;`: Terminates the program.
Compiling and Running Your Program
Once you’ve finished writing your code, it’s time to compile and run it. Using gpp-compiler, follow these steps:
- Open the terminal in Atom.
- Navigate to the directory of your file.
- Compile your C++ code with the following command:
g++ hello_world.cpp -o hello_world
- Execute your compiled program using:
./hello_world
You should see "Hello, World!" displayed in the console, indicating that your setup is correctly configured.
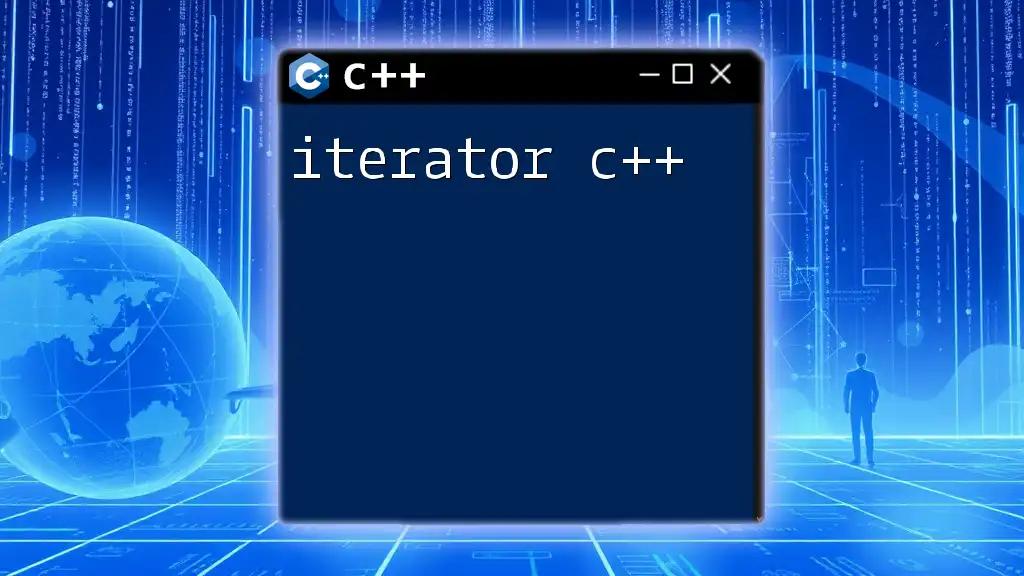
Working with C++ Features in Atom
Code Snippets and Autocompletion
Atom offers powerful features like code snippets and autocompletion to improve your coding efficiency.
To create personalized snippets for commonly used C++ structures, explore the snippet feature under `Packages > Snippets`. For instance, you can create a snippet for a function structure, allowing you to insert it quickly anytime you need it.
Syntax Highlighting and Linting
Syntax highlighting is important for readability, and Atom provides excellent support for this. Additionally, implementing linting helps prevent common coding mistakes.
One recommended package for linting is linter-gcc, which highlights errors and warnings in your code. To install:
- Navigate to `Settings > Install`
- Search for linter-gcc and click install.
Debugging in Atom
Debugging is an essential skill for any programmer. Atom makes it easier with debugger integration.
You can install the atom-debugger package, which helps identify and resolve errors within your code. After installation, you can step through your code, inspect variables, and understand the flow of your C++ program.
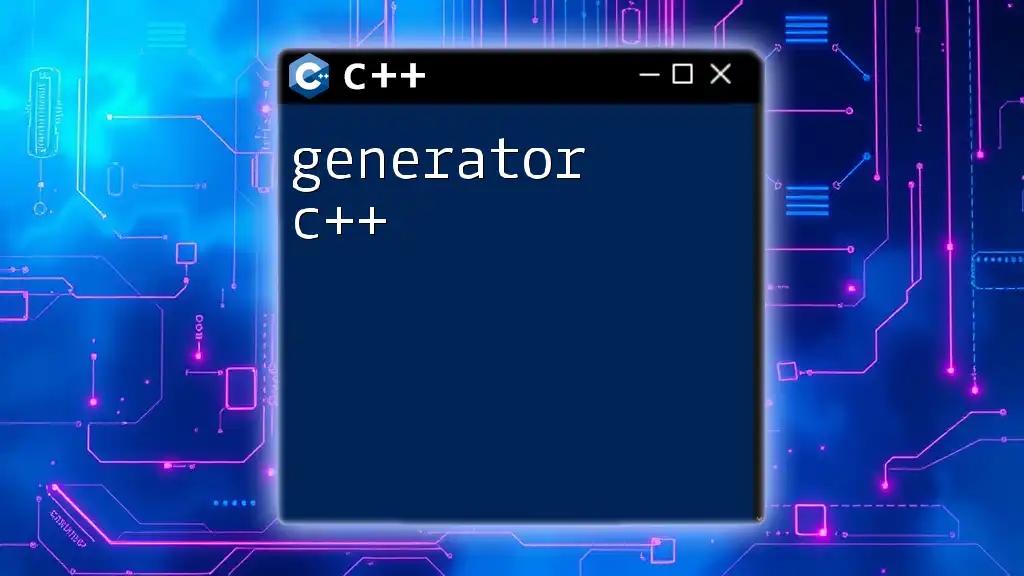
Advanced Topics
Using Multiple Files in a C++ Project
For larger projects, it’s beneficial to separate your code into multiple files. A recommended structure might look like this:
- /my_project
- `main.cpp`: Contains the `main` function.
- `functions.h`: Declares functions.
- `functions.cpp`: Defines functions.
Having separate header files makes your code easier to manage and understand, enhancing team collaboration if you're working with multiple developers.
Version Control with Git in Atom
Integrating Git into your workflow allows for better version control—critical when working on larger projects or collaborations.
- Ensure Git is installed on your system.
- Open Atom and navigate to the `Git` tab in the bottom pane to initialize or clone a repository.
- Use Git commands directly from Atom to commit, push, and pull changes.
Basic Git commands you should be familiar with include:
- `git init`: Initializes a new Git repository.
- `git commit -m "message"`: Saves your changes with a message describing the update.
- `git push origin main`: Sends your committed changes to the remote repository.
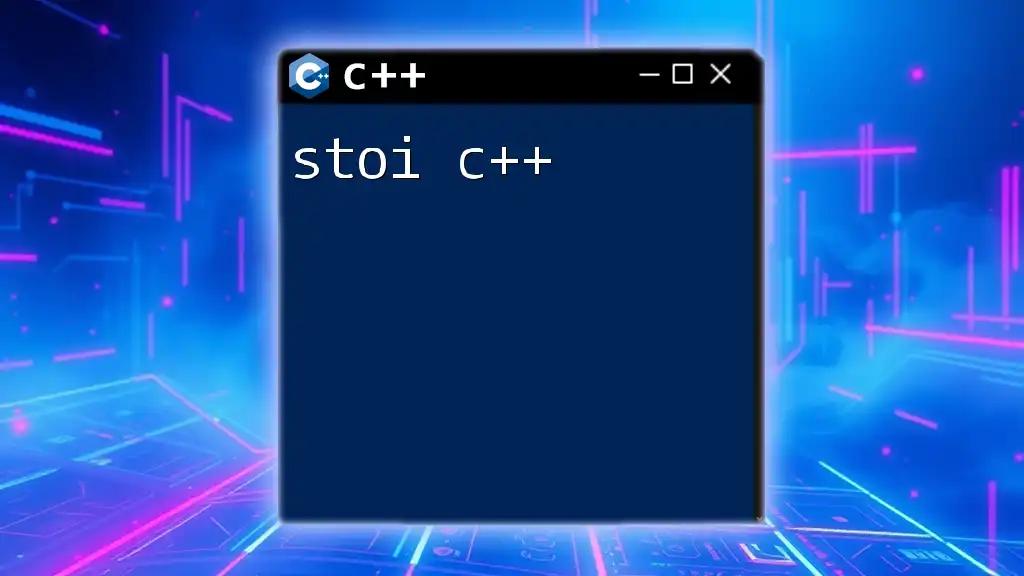
Community Resources and Further Learning
Online C++ Communities
Joining online communities can accelerate your learning. Consider engaging with these platforms where you can seek advice, share resources, and learn from experienced developers:
- Stack Overflow: A vast Q&A platform for programming-related queries.
- Discord Channels: Many programming communities exist on Discord, allowing for real-time interaction.
Recommended Resources
To continue growing your skills in C++, explore various resources such as books, online courses, and tutorials. Some highly regarded options include:
- Books: “C++ Primer” and “Effective C++”
- Courses: Online platforms like Coursera and Udacity offer excellent C++ courses.
Always prioritize continuous learning to enhance your programming skills.
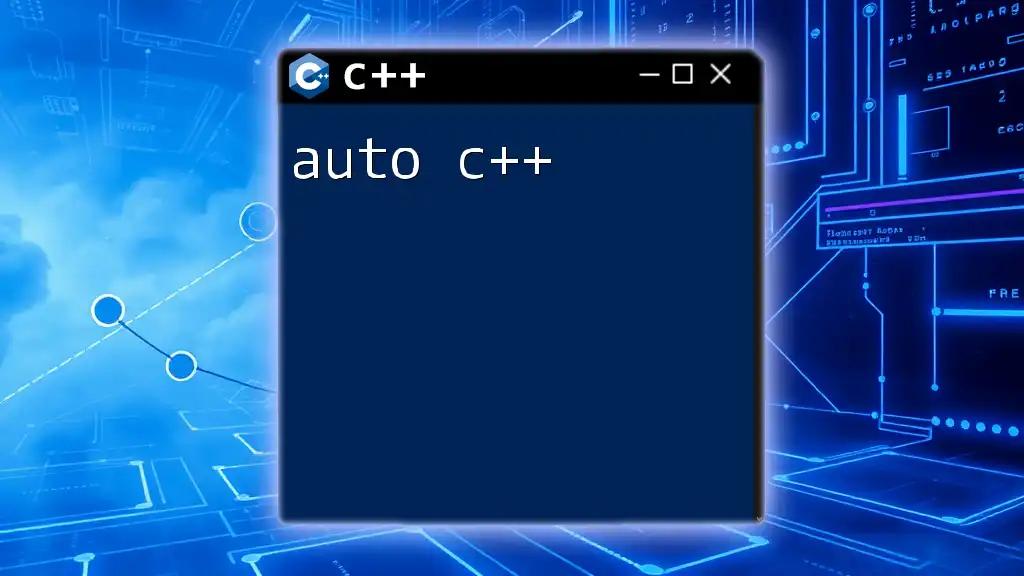
Conclusion
In this comprehensive guide, we explored Atom C++ development, covering installation, configuration, and essential programming techniques. With the right setup and knowledge of Atom's features, you're well on your way to becoming a proficient C++ developer. Embrace the learning journey, engage with communities, and take on projects that challenge your skills! If you have any questions or insights, feel free to reach out!