The `strtol` function in C++ is used to convert a string to a long integer, allowing for base specification and error handling.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "12345";
char* end;
long num = strtol(str, &end, 10);
if (*end == '\0') {
std::cout << "Converted number: " << num << std::endl;
} else {
std::cout << "Conversion error or extra characters: " << end << std::endl;
}
return 0;
}
What is strtol?
The `strtol` function, part of the C standard library, is utilized for converting a string to a long integer. Its versatility allows it to handle various numeric bases and provide feedback on the conversion process.
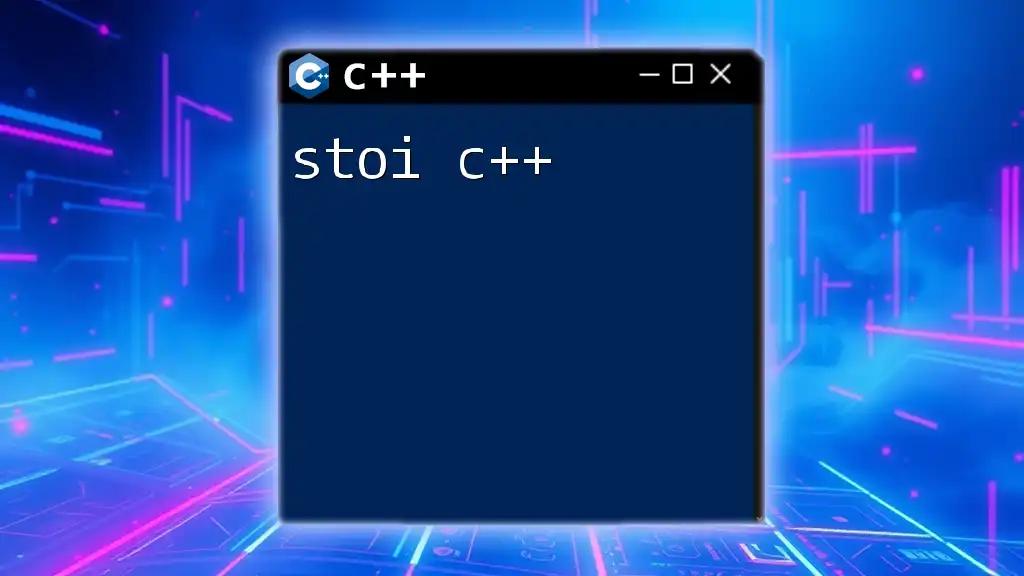
Why Use strtol in C++?
When working with string data, developers often need to convert text representations of numbers into a usable numeric format. While C++ offers several methods for string conversion, such as `std::stoi`, `strtol` provides performance and flexibility, especially when handling different numeric bases. Furthermore, `strtol` offers better control over conversion errors and allows for extensive error handling, which is essential in robust applications.
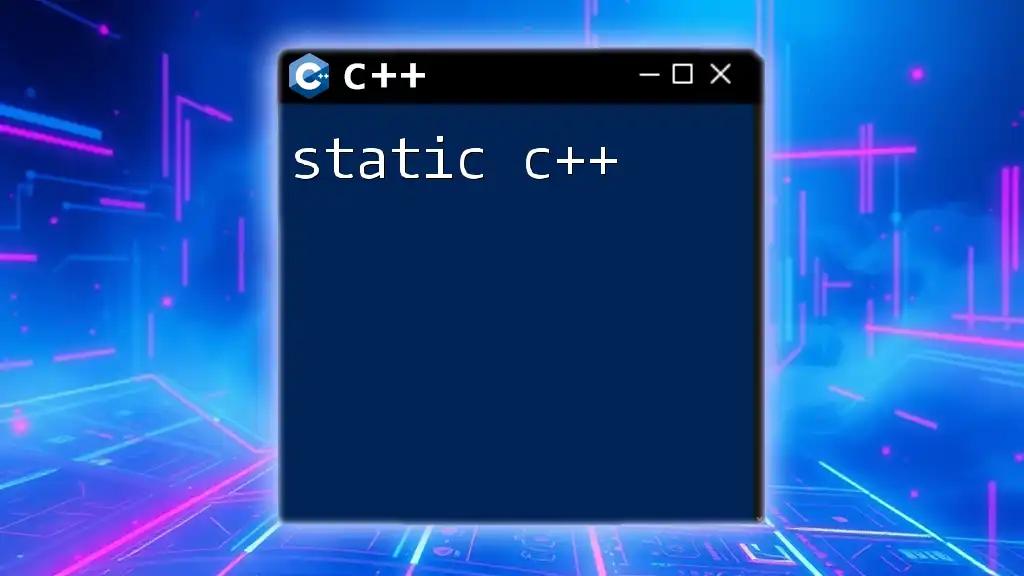
Understanding the Syntax of strtol
Function Prototype
The function definition for `strtol` is as follows:
long int strtol(const char *str, char **endptr, int base);
Parameters Breakdown
-
`str`: This parameter is the input string to convert. It is essential that this string contains a valid representation of a number, otherwise conversion will fail.
-
`endptr`: This parameter is a pointer to the character that follows the last character converted. It's not mandatory, but using it helps in determining where the conversion stopped, which is particularly useful in error handling.
-
`base`: This integer defines the numerical base for conversion. The base can range from 2 to 36, and if you use 0, the function determines the base automatically based on the prefix of the string (e.g., "0x" for hexadecimal).
Return Value
The return value of `strtol` is the converted long integer. If the string cannot be converted, zero is returned. Additionally, to control for potential overflow or underflow, the return value can depend on specific circumstances such as input range.
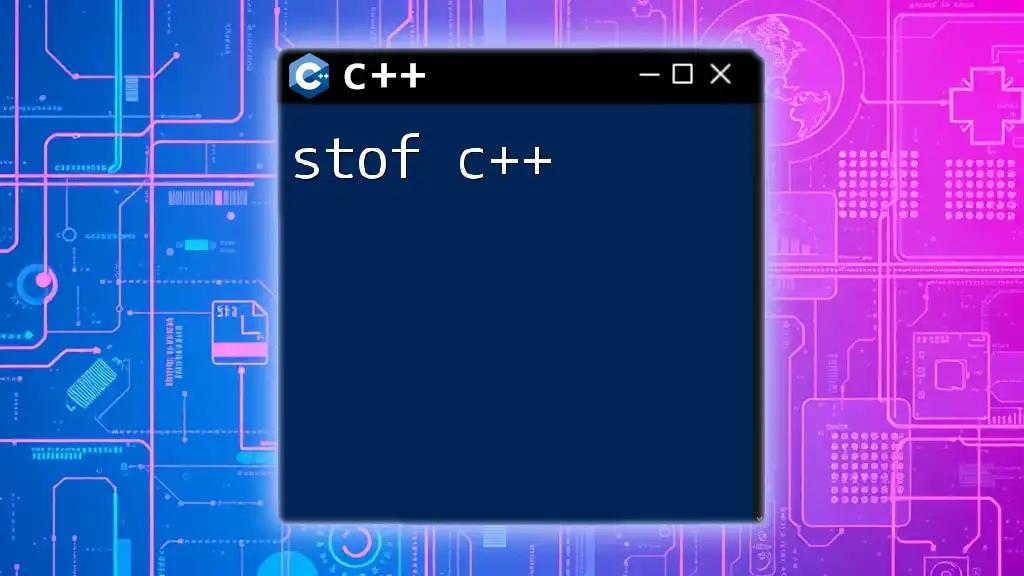
Practical Application of strtol in C++
Basic Example of Using strtol
You can see the simplicity of using `strtol` by looking at the following example:
#include <iostream>
#include <cstdlib>
int main() {
const char* numStr = "12345";
char* endPtr;
long int num = strtol(numStr, &endPtr, 10);
std::cout << "Converted Number: " << num << std::endl;
return 0;
}
In this snippet, `strtol` is used to convert a string representing a decimal number into a `long int`. The output you will see upon running this code will affirm the successful conversion.
Handling Errors with strtol
One of the key advantages of using `strtol` is its ability to gracefully handle errors. By leveraging the `endptr`, you can detect if any conversion was actually performed, which can prevent issues from invalid inputs. For example:
if (endPtr == numStr) {
std::cerr << "No conversion could be performed." << std::endl;
}
If `endPtr` is equal to `numStr`, it indicates that no valid conversion was accomplished, allowing for proper error management.
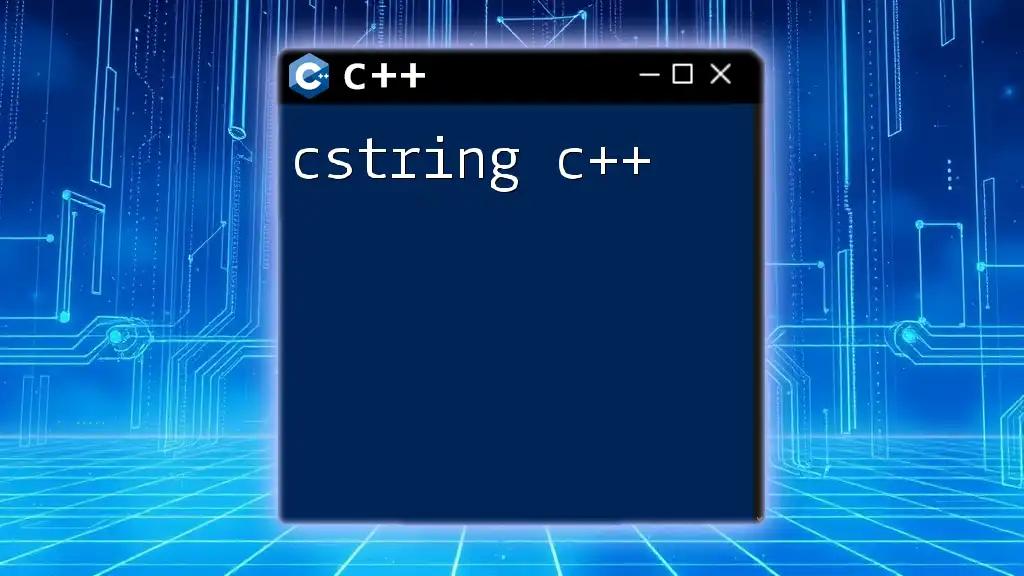
Advanced Usage of strtol
Converting Different Bases
`strtol` shines when it comes to converting strings in various numeric bases. This utility becomes essential when dealing with representations like hexadecimal.
const char* hexStr = "1A3F";
long int hexValue = strtol(hexStr, nullptr, 16);
std::cout << "Hex to Decimal: " << hexValue << std::endl;
This example demonstrates converting a hexadecimal string to a long integer, showcasing `strtol`'s versatility.
Negative Values and strtol Behavior
`strtol` can also handle negative values effectively, expanding its utility. Below is an example that illustrates this feature:
const char* negStr = "-456";
long int negNum = strtol(negStr, nullptr, 10);
std::cout << "Converted Negative Number: " << negNum << std::endl;
The output will properly reflect the negative number, demonstrating `strtol`'s capability in handling signed integers.

Common Pitfalls and Best Practices
Common Mistakes
When using `strtol`, developers may encounter several common pitfalls:
-
Null Input Strings: Passing a null pointer instead of a valid string can lead to undefined behavior.
-
Incorrect Base Values: Failing to use valid ranges for the `base` parameter can lead to unexpected results.
Best Practices
To ensure robust C++ code, consider the following best practices when using `strtol`:
-
Always check the `endptr` after calling `strtol` to ascertain that the conversion was successful.
-
Handle overflow and underflow conditions by checking the return value against `LONG_MAX` and `LONG_MIN` to avoid misrepresentation of data.
For example:
if (num == LONG_MAX || num == LONG_MIN) {
std::cerr << "Number out of range!" << std::endl;
}
This snippet illustrates a safe way to check for potential range errors when converting strings.
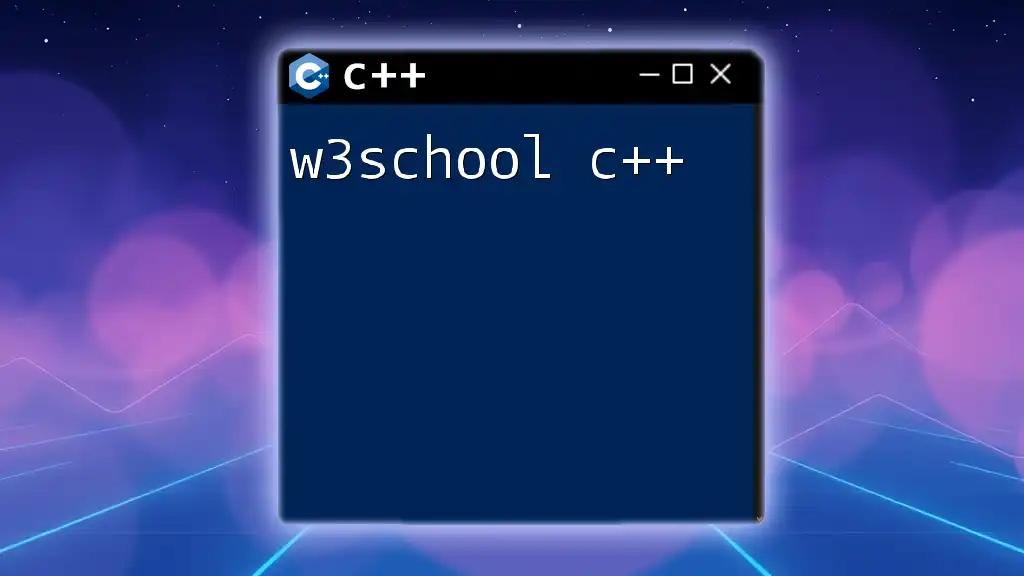
Conclusion
`strtol` is a powerful function in C++ for converting strings to long integers, known for its flexibility in handling various numerical bases and effective error management. Proper understanding and application of `strtol` can lead to more robust and reliable C++ applications, especially when dealing with user input or data from external sources.

FAQs about strtol in C++
What happens if I pass an invalid string to strtol?
If you pass an invalid string to `strtol`, the conversion will fail. If nothing can be converted, `strtol` returns zero, and the `endptr` will point to the original string.
Can strtol handle floating-point numbers?
No, `strtol` is designed specifically for integers and does not convert floating-point numbers. For floating-point conversions, consider using `strtof` or `stof`.
How does strtol relate to other C++ string functions?
`strtol` offers advantages of performance and error handling over `std::stoi` or similar functions, especially in scenarios where extensive error tracking and base conversions are necessary.