The `stoi` function in C++ converts a string to an integer, allowing for easy type conversion when handling numerical data in string format.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "42";
int number = std::stoi(str);
std::cout << "The converted integer is: " << number << std::endl;
return 0;
}
What is `stoi`?
`stoi` stands for String to Integer and is a standard function in C++ that allows you to convert a `std::string` representation of an integer into an actual integer type. The ability to convert strings into integers is fundamental in programming, as many applications require processing user input, reading data from files, or parsing strings dynamically.
Why Use `stoi`?
Using `stoi` has several advantages:
- Simplicity: It simplifies the process of converting strings to integers without needing to manually iterate over characters.
- Speed: Being a part of the C++ Standard Library, `stoi` is optimized for performance, making it faster than custom solutions.
- Error Handling: It provides built-in error handling through exceptions, which is crucial for robust applications.
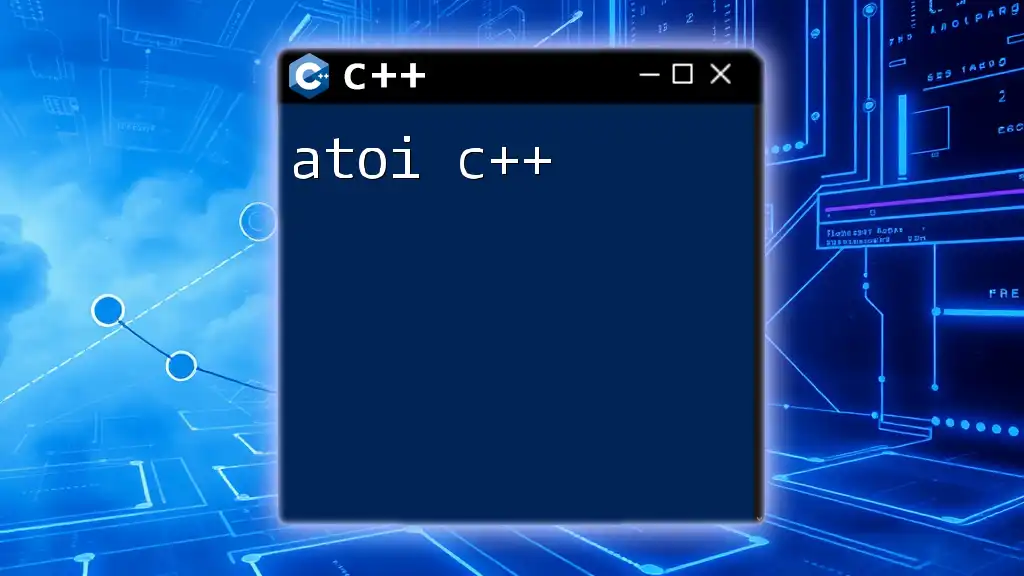
Understanding `stoi`
Syntax of `stoi`
The `stoi` function has the following syntax:
int stoi(const std::string& str, std::size_t* idx = 0, int base = 10);
Breaking this down:
-
Parameters:
- `str`: The string you want to convert to an integer.
- `idx` (optional): A pointer to a `std::size_t` where the position of the first unconverted character in the string is stored. If you don't need this information, you can omit this parameter.
- `base` (optional): The numerical base used for conversion. It accepts values between 2 to 36 and defaults to 10.
-
Return Value: Returns the converted integer. If the string is not a valid representation of an integer or if the value is out of the range of the integer type, exceptions are thrown.
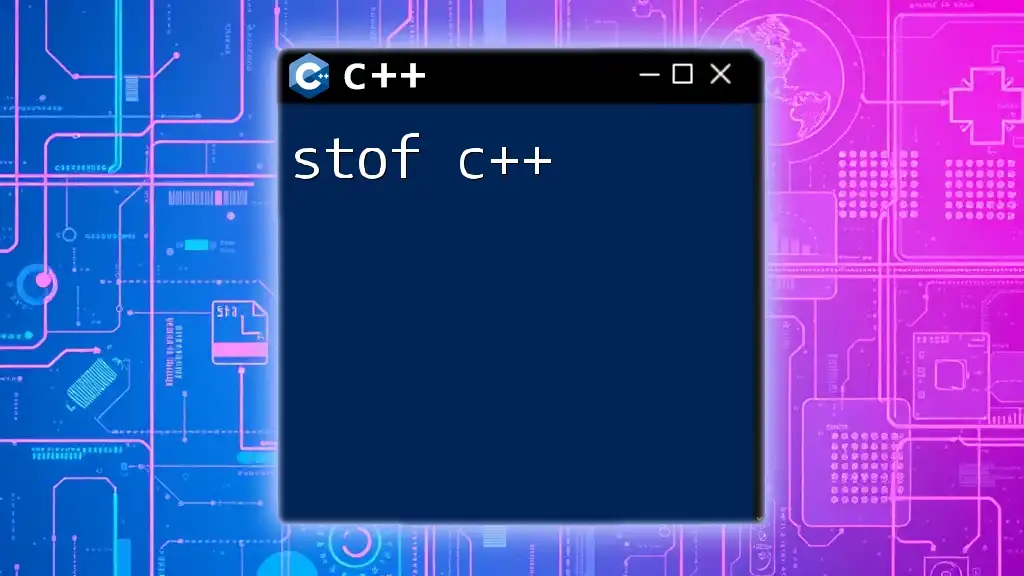
How to Use `stoi`
Basic Usage
The most straightforward way to use `stoi` involves simply passing a string that contains a valid integer. Here's a basic example:
#include <iostream>
#include <string>
int main() {
std::string numString = "12345";
int number = std::stoi(numString);
std::cout << "The number is: " << number << std::endl;
return 0;
}
In this example, the string `"12345"` is converted to the integer `12345`, which is then printed to the console.
Using the `idx` Parameter
The `idx` parameter allows you to identify the first unconverted character in the string, which can be useful when parsing more complex strings. Here's how you use it:
#include <iostream>
#include <string>
int main() {
std::string numString = "123abc";
std::size_t idx;
int number = std::stoi(numString, &idx);
std::cout << "The number is: " << number << std::endl;
std::cout << "First unconverted character: " << numString[idx] << std::endl;
return 0;
}
In this example, the function converts `"123abc"` into `123` and provides the index position of the first non-numeric character, which is `a`.
Conversion with Different Bases
The base parameter allows for converting numbers represented in bases other than decimal. Here’s how you can convert a hexadecimal number:
#include <iostream>
#include <string>
int main() {
std::string hexString = "1A";
int hexNumber = std::stoi(hexString, nullptr, 16);
std::cout << "Hexadecimal 1A to decimal: " << hexNumber << std::endl;
return 0;
}
In this case, the hexadecimal string `"1A"` is converted to its decimal equivalent, which is `26`.
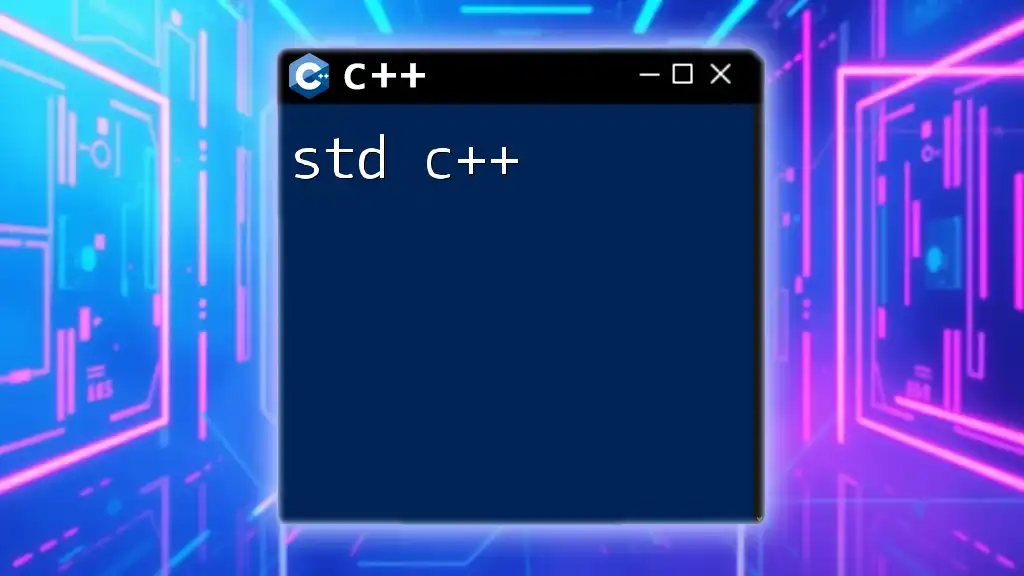
Error Handling with `stoi`
Common Exceptions
When using `stoi`, it is essential to account for possible exceptions, which include:
- `std::invalid_argument`: This is thrown when the provided string does not contain any digits.
- `std::out_of_range`: This occurs when the converted value would fall out of the range of the result type. For instance, converting a very large string that exceeds the limits of `int`.
Example of Exception Handling
Here’s how to implement error handling while using `stoi`:
#include <iostream>
#include <string>
int main() {
std::string invalidString = "abc";
try {
int number = std::stoi(invalidString);
} catch (const std::invalid_argument& e) {
std::cout << "Error: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cout << "Error: " << e.what() << std::endl;
}
return 0;
}
This example demonstrates catching exceptions thrown by `stoi`, allowing your program to handle errors gracefully without crashing.
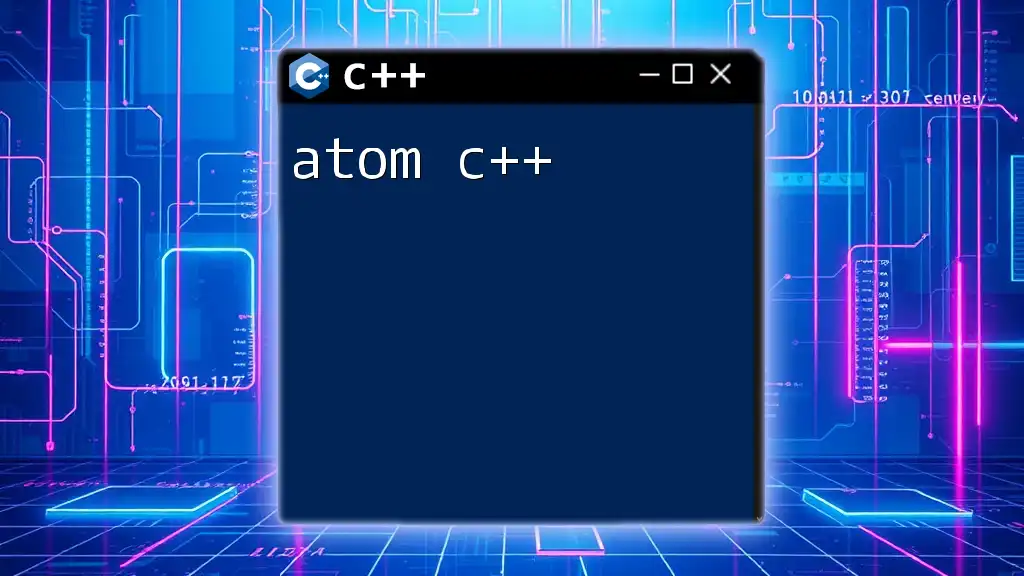
Best Practices for Using `stoi`
Validate Input
Before using `stoi`, it's wise to validate that the string conforms to the expected numeric format. This can prevent unnecessary exceptions and make your code more reliable.
Use Appropriate Exception Handling
Make sure you always implement `try-catch` blocks when parsing user input or processing data that may not be well-formed. This defensive programming approach ensures that your application can handle unexpected input gracefully.
Performance Considerations
While `stoi` is efficient, if you know that your string inputs will always be valid integers, then you could forego error handling to simplify the code. However, in general practice, always prefer `stoi` for converting strings due to its built-in safety and simplicity compared to manual parsing techniques.
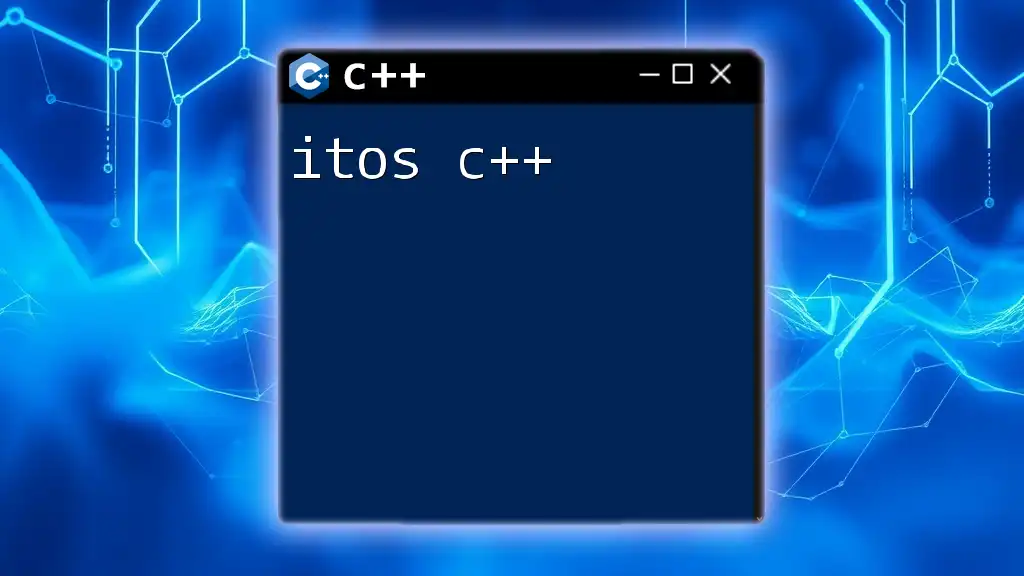
Conclusion
In summary, `stoi` is a powerful and convenient function in C++ for converting strings to integers. Its built-in error handling, support for different bases, and ease of use make it ideal for a variety of applications. By understanding how to use `stoi` effectively, including its capabilities and limitations, you can enhance the robustness and reliability of your C++ programs.