The `std` namespace in C++ is a standard library that contains common classes and functions, allowing developers to use features such as input/output operations, algorithms, and data structures without needing to define them from scratch.
Here’s a simple example demonstrating the use of `std::cout` to print a message to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the std Namespace
In C++, the std namespace plays a critical role as the repository for all classes and functions defined by the Standard Library. This prevents name collisions, ensuring that standard library identifiers don’t conflict with user-defined names. Accessing elements within this namespace typically requires prefixing with 'std::'. For example, to use the `cout` stream, you would write `std::cout`.

Key Components of std
Containers
Containers are vital components of the C++ Standard Library, providing ways to store and manage collections of data. They come in various forms, each serving unique purposes tailored to different tasks.
Sequential Containers
These store data in a linear order. Common sequential containers include:
-
Vector: A dynamic array that can change size. Elements are stored in contiguous memory, making access via index very fast.
-
List: A doubly-linked list that allows for efficient insertions and deletions from any position, but does not allow random access.
-
Deque: A double-ended queue that allows for efficient addition and removal from both ends.
Example: Using std::vector
Here’s a simple demonstration of how to use a vector involving basic operations like adding elements and iterating through the vector.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Creation
numbers.push_back(6); // Adding an element
for (int num : numbers) { // Iterating through the vector
std::cout << num << " ";
}
return 0;
}
Associative Containers
These store elements formed of key-value pairs, where each key is unique. They enable fast searches.
-
Set: A collection of unique elements sorted by value.
-
Map: A collection of key-value pairs, where each key must be unique.
Unordered Containers
These are optimized for fast access and do not retain the order of elements.
-
Unordered Set: A collection of unique elements without any specific order.
-
Unordered Map: A collection of key-value pairs with no order, focusing on quick access.
Algorithms
Algorithms provide powerful functionalities to manipulate collections. They are defined within the std namespace, allowing for operations like sorting and searching.
Commonly Used Algorithms
-
Sorting Algorithms: `std::sort` is one of the most frequently used algorithms when we need to sort a container.
-
Searching Algorithms: `std::find` allows for searching elements in a container.
Example: Using std::sort
Here’s a demonstration using `std::sort` to sort a vector of integers.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {4, 2, 5, 1, 3}; // Unsorted vector
std::sort(nums.begin(), nums.end()); // Sorting the vector
for (int num : nums) { // Displaying sorted numbers
std::cout << num << " ";
}
return 0;
}
Iterators
Iterators are essential tools that allow for traversal through containers without exposing underlying implementations. Think of them as generalized pointers.
Types of Iterators
- Input Iterators allow reading of data in a single pass.
- Output Iterators facilitate writing data.
- Forward Iterators are capable of multiple passes through data.
- Bidirectional Iterators can traverse both directions.
- Random Access Iterators allow jumping to any position.
Example: Using Iterators with std::set
The following example highlights how to iterate through a set using iterators.
#include <set>
#include <iostream>
int main() {
std::set<int> mySet = {4, 2, 5, 1, 3}; // Set creation with initial values
for (std::set<int>::iterator it = mySet.begin(); it != mySet.end(); ++it) { // Iterating through the set
std::cout << *it << " "; // Dereference iterator to get value
}
return 0;
}
Function Objects and Functors
In C++, a functor is essentially an object that can be called as if it were a function. They present a way to encapsulate function behavior alongside state.
Using std::function
The `std::function` template can store any callable object, making it a powerful tool for callback functions.
Example: Using std::function
This example demonstrates how to pass functions as parameters using `std::function`.
#include <iostream>
#include <functional>
void process(std::function<void(int)> func) {
func(10); // Invoke the function with the argument 10
}
int main() {
process([](int x) { std::cout << x * 2; }); // Lambda function used as callback
return 0;
}

Additional Features of std
Smart Pointers
Memory management is crucial in C++ programming. Smart pointers help prevent memory leaks and manage object lifetimes effectively.
Types of Smart Pointers
- std::unique_ptr: Manages unique ownership of an object.
- std::shared_ptr: Allows multiple pointers to own an object, managing shared ownership automatically.
- std::weak_ptr: A non-owning reference that avoids circular references.
Example: Using std::shared_ptr
Here's how to create and use a shared pointer.
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> ptr1 = std::make_shared<int>(10); // Create a shared pointer
std::cout << *ptr1 << std::endl; // Dereference to access value
return 0;
}
Thread Support Library
With the rise of multi-core processors, the C++ Standard Library includes features for multithreading, allowing concurrent execution of code.
Overview of Thread Classes
- std::thread: Represents a single thread of execution.
- std::mutex: Provides mutual exclusion for shared data.
Example: Using std::thread
The following code demonstrates how to create and manage a simple thread.
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from thread!" << std::endl; // Code executed by the thread
}
int main() {
std::thread t(threadFunction); // Create and start a thread
t.join(); // Wait for the thread to finish before continuing
return 0;
}

Best Practices in Using std
When working with the C++ Standard Library, there are several best practices to enhance performance and maintainability:
- Choosing the Right Container: Assess your data handling requirements (like size, speed, and element types) to select the most suitable container type.
- Utilizing Algorithms Effectively: Take advantage of built-in algorithms to minimize the amount of custom code you write, making your code cleaner and more efficient.
- Optimizing Performance Using Smart Pointers: Use smart pointers wherever possible to manage resources automatically, reducing human error and memory leaks.
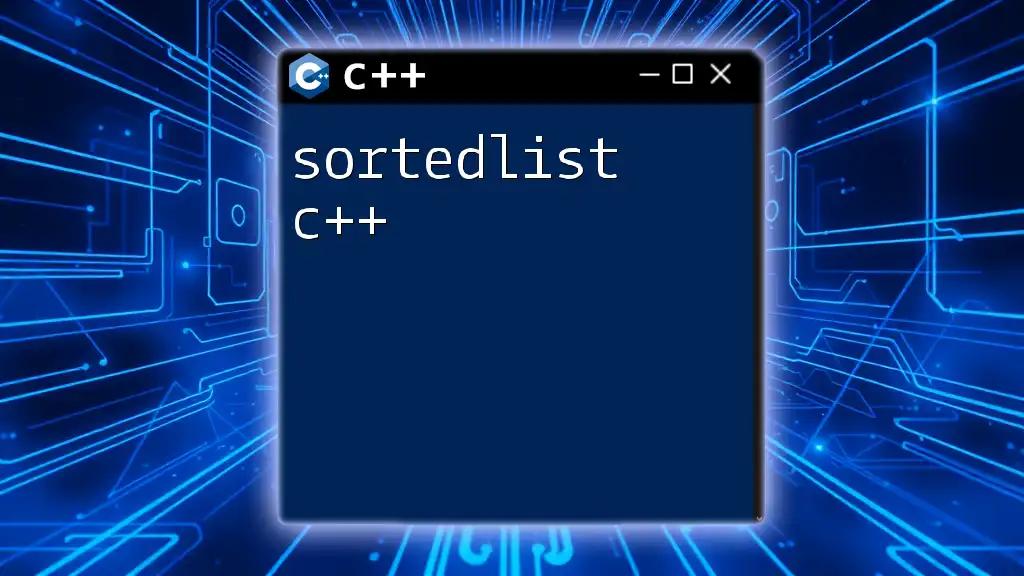
Common Pitfalls When Using std
While the C++ Standard Library is powerful, several common pitfalls can occur:
- Misuse of Containers and Iterators: Not adhering to the container guarantees can lead to bugs, such as accessing out-of-bounds elements.
- Memory Leaks with Raw Pointers: Using new/delete excessively can lead to memory leaks; prefer smart pointers to manage memory automatically.
- Thread Safety Concerns: Be cautious about shared resources in parallel executions; always protect shared data with mutexes or other synchronization mechanisms.
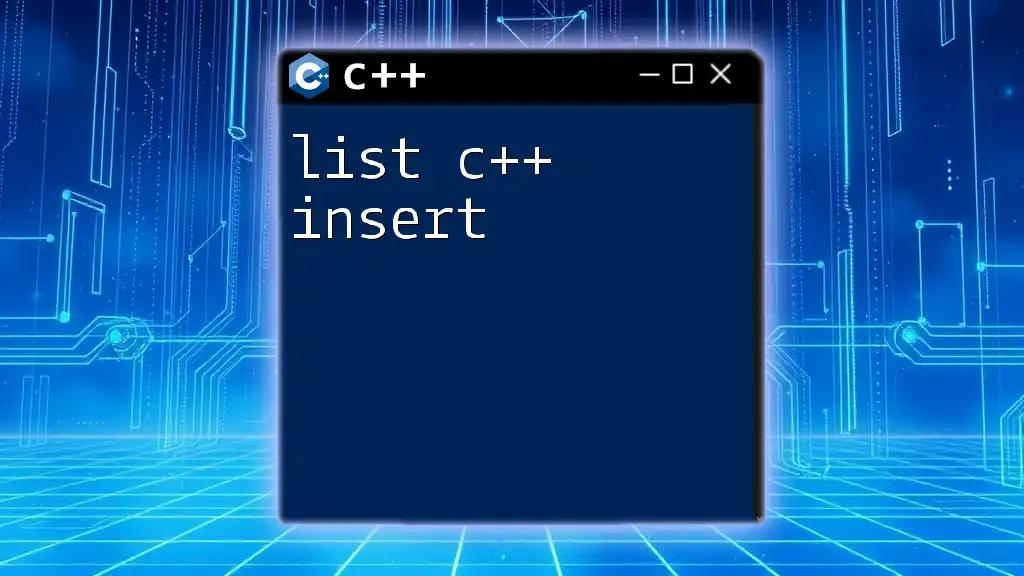
Conclusion
As you explore the std c++ library, remember its power and flexibility in managing data, performing algorithms, and facilitating memory management. The examples and practices outlined can help you build a strong foundation in using the Standard Library effectively. Engaging with these concepts is essential for becoming proficient in C++.
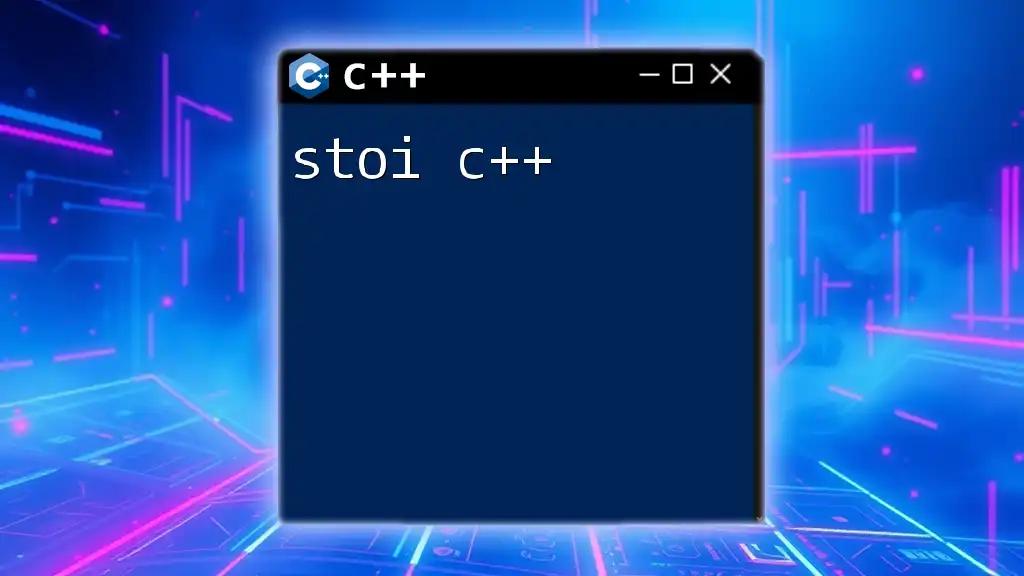
Further Reading and Resources
To deepen your understanding, consider exploring recommended books on C++ and various online tutorials and documentation. Additionally, joining community forums can provide you access to diverse perspectives and insights from experienced developers.