In C++, the Standard Template Library (STL) provides a `queue` container class that allows you to manage a collection of elements in a FIFO (First In, First Out) manner.
Here’s a simple example of how to use a queue in C++:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
myQueue.push(10); // Add 10 to the queue
myQueue.push(20); // Add 20 to the queue
myQueue.push(30); // Add 30 to the queue
std::cout << "Front element: " << myQueue.front() << std::endl; // Access the front element
myQueue.pop(); // Remove the front element
std::cout << "Front element after pop: " << myQueue.front() << std::endl; // Check the new front element
return 0;
}
What is a Queue?
A queue is a linear data structure that serves as a collection of elements, adhering to the principle of First In First Out (FIFO). This means that the first element added to the queue will be the first one to be removed, similar to a line of people waiting for service. A queue has several essential characteristics that make it a compelling choice in various situations:
Key Terminology
- Enqueue: This operation involves adding an element to the end of the queue.
- Dequeue: This is the process of removing an element from the front of the queue.
- Front: This refers to the first element of the queue, which will be removed next.
- Back: The last element in the queue, which can be accessed but not directly removed.
- Size: This denotes the total number of elements currently in the queue.
- Empty: This checks whether the queue has any elements.
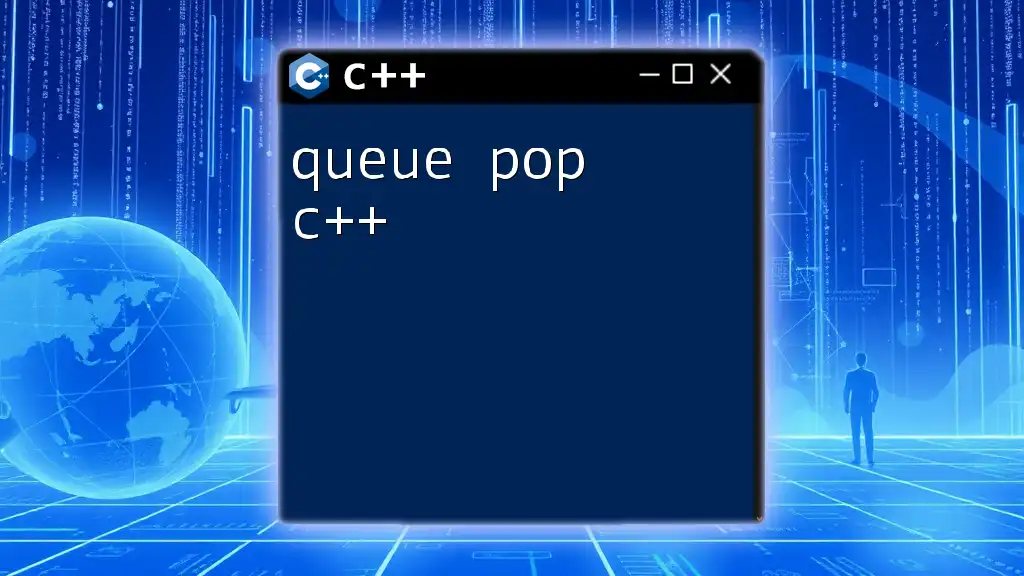
C++ Queue Implementation
Including the Necessary Header
To use queues in C++, you need to include the appropriate library by adding the following line at the top of your code:
#include <queue>
This statement fetches all the functionalities associated with queue data structures from the C++ Standard Template Library (STL).
Creating a Queue
In C++, you can create a queue which can store elements of any data type. Below are examples of how to initialize queues for different types:
std::queue<int> intQueue; // Queue of integers
std::queue<std::string> stringQueue; // Queue of strings
Using templates, you can define queues for user-defined data types, enhancing the flexibility of your queue implementations.
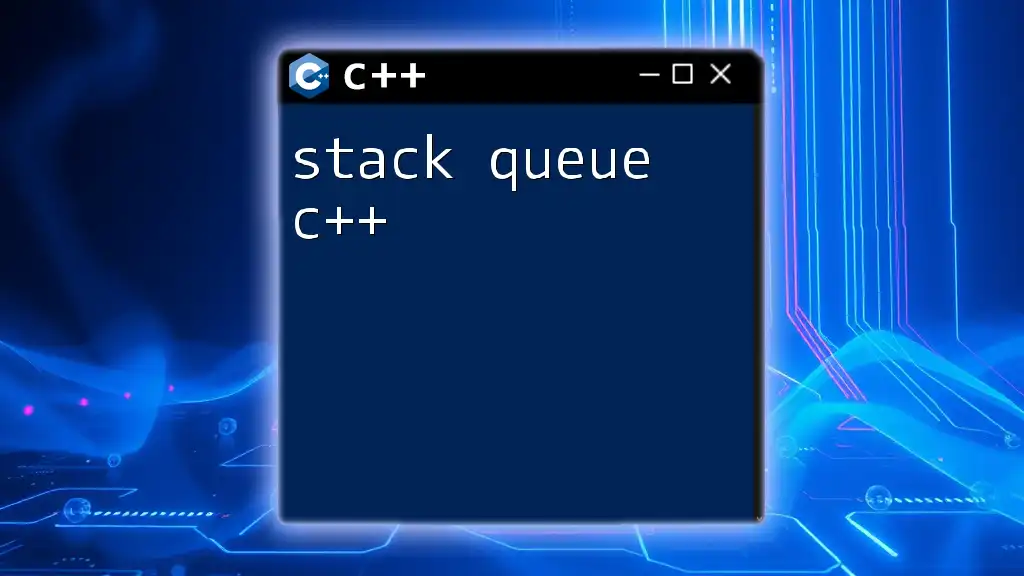
Basic Queue Operations
Enqueuing Elements
The enqueue operation allows you to add elements to the queue, enabling you to build a collection:
intQueue.push(1);
intQueue.push(2);
intQueue.push(3);
With the `push()` method, you can append new items easily, which will be stored at the end of the queue.
Dequeuing Elements
When you need to remove an element, you will use the dequeue operation, as follows:
intQueue.pop();
The `pop()` method removes the element at the front of the queue, making the next item in line the new front element.
Accessing Front and Back Elements
To peek at the elements without removing them, you can use the following methods:
int frontElement = intQueue.front(); // Gets the first element
int backElement = intQueue.back(); // Gets the last element
- `front()`: Provides access to the first element.
- `back()`: Provides access to the last element. These methods help you monitor the queue without altering its state.
Checking Size and Emptiness
To determine how many items are in your queue or whether it’s empty, you can use these methods:
size_t queueSize = intQueue.size(); // Returns the number of elements in the queue
bool isEmpty = intQueue.empty(); // Checks if the queue is empty
- size(): Returns the count of elements.
- empty(): Returns a boolean indicating if the queue contains any items.
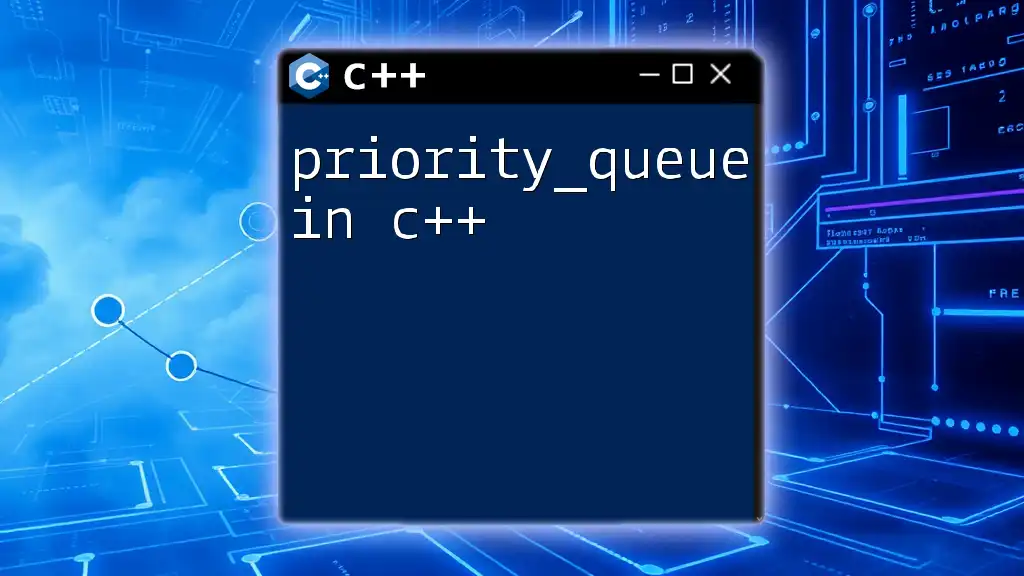
Advanced Queue Operations
Customizing Queue with User-Defined Types
You can expand the utility of queues by using custom types. Below is an example of how a queue can manage structures defined by the user:
struct Person {
std::string name;
int age;
};
std::queue<Person> personQueue; // Queue that stores Person objects
When using custom data types, simply ensure the necessary properties are in place, and you can enqueue and dequeue them just like primitive types.
Priority Queues vs. Regular Queues
While a standard queue strictly follows the FIFO principle, a priority queue allows elements to be processed based on priority rather than just order of arrival. Here’s a brief illustration:
#include <queue>
std::priority_queue<int> pQueue; // Defines a priority queue for integers
In a priority queue, elements with higher priority get dequeued before those with lower priority, making it useful in scenarios like task scheduling.
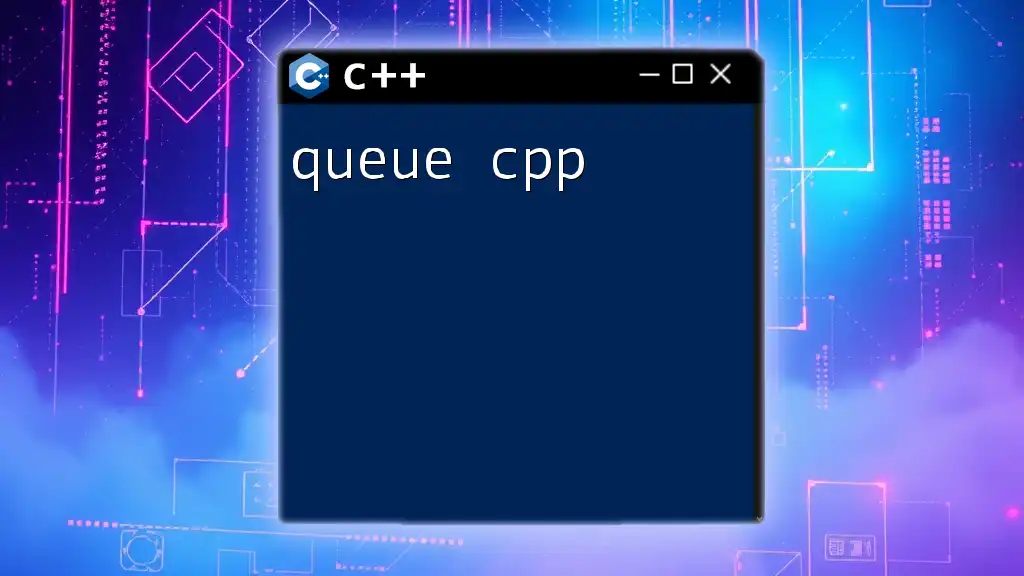
Common Use Cases for Queues
Job Scheduling
Queues are widely implemented in job scheduling contexts, where tasks are managed in the order they arrive. For example, a printer queue manages print jobs in the order they are received, thus ensuring efficiency and fairness.
Handling Requests in Web Servers
Web servers use queues to handle incoming requests, ensuring that each request is processed in an orderly manner. By maintaining a queue for incoming requests, servers optimize resource allocation and reduce latency.
BFS Algorithm in Graphs
Queues also play a crucial role in the Breadth-First Search (BFS) algorithm for traversing or searching tree or graph data structures. Here's a simplified code snippet exemplifying BFS implementation using a queue:
std::queue<Node*> bfsQueue;
bfsQueue.push(rootNode);
while (!bfsQueue.empty()) {
Node* currentNode = bfsQueue.front();
bfsQueue.pop();
// Process the current node...
if (currentNode->left) bfsQueue.push(currentNode->left);
if (currentNode->right) bfsQueue.push(currentNode->right);
}
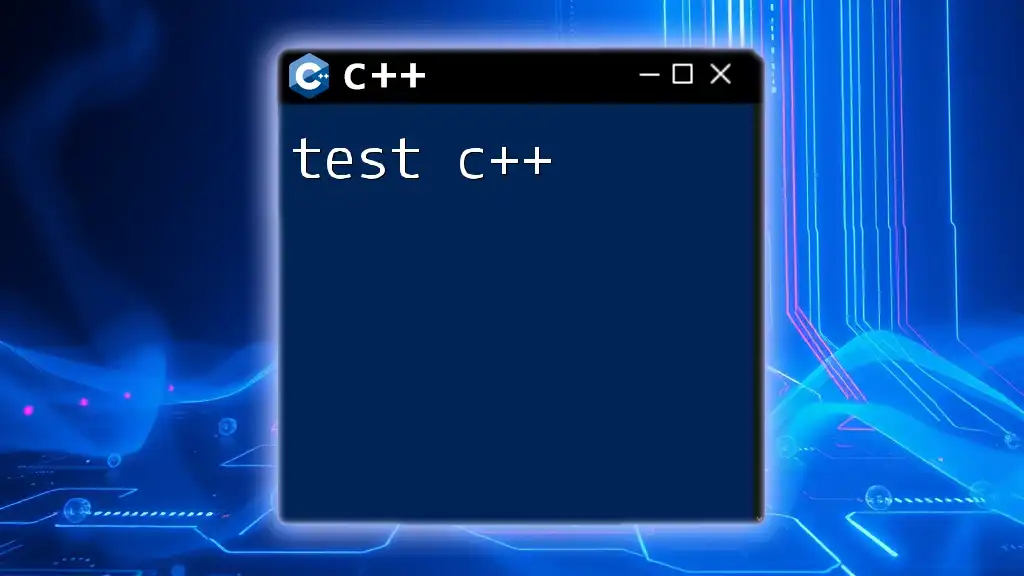
Performance Considerations
Time Complexity of Queue Operations
Understanding the time complexities associated with queue operations can inform developers on when to utilize queues effectively:
- Enqueue (push): O(1) – Constant time performance.
- Dequeue (pop): O(1) – Constant time performance.
- Front/Back: O(1) – Instant access time.
This efficiency makes queues particularly useful in real-time applications.
When to Use Queues
Queues are ideal in scenarios involving order maintenance in linear processing flows. They suit applications like job scheduling, managing asynchronous tasks, handling requests, and traversing structures where sequential access and FIFO principles are crucial.
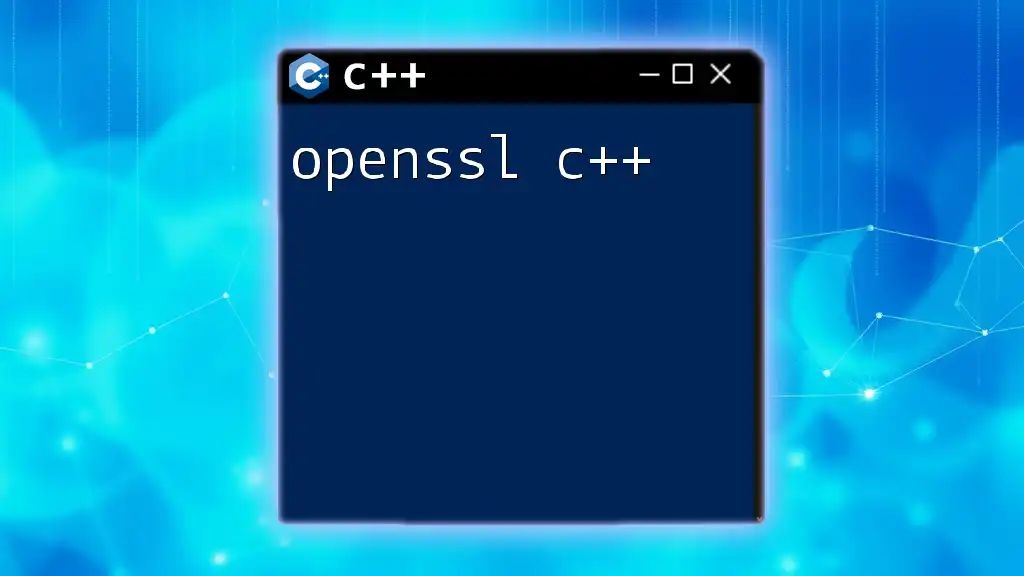
Conclusion
The queue STL in C++ offers invaluable functionalities that enhance your programming toolkit. Through an understanding of queues, including their operations and performance considerations, you can implement organized and efficient algorithms tailored to specific needs. Practice implementing queues and integrate them into your projects to reinforce your knowledge and skills.