In C++, the `#include` directive is used to include the contents of a file (typically a header file) in your program, allowing you to utilize functions, classes, and objects defined in that file.
#include <iostream> // This includes the standard input-output stream library
int main() {
std::cout << "Hello, World!" << std::endl; // Outputs "Hello, World!" to the console
return 0;
}
What are Includes in C++?
In C++, include directives are essential components that allow programmers to bring in external code into their programs. The `#include` statement tells the compiler to include the contents of a specified file in the program during the preprocessing stage before compilation begins. This is crucial for enabling the use of libraries, creating modular code, and promoting code reusability.
How Includes Work
When a C++ program is compiled, it undergoes several stages, one of which is preprocessing. During this preprocessing phase, the compiler processes any directives that begin with the `#` symbol. This includes the `#include` directive, where the preprocessor replaces the line with the contents of the included file. Therefore, understanding how the preprocessor operates is vital when working with includes, as this stage ultimately determines the structure of your program before it is compiled.
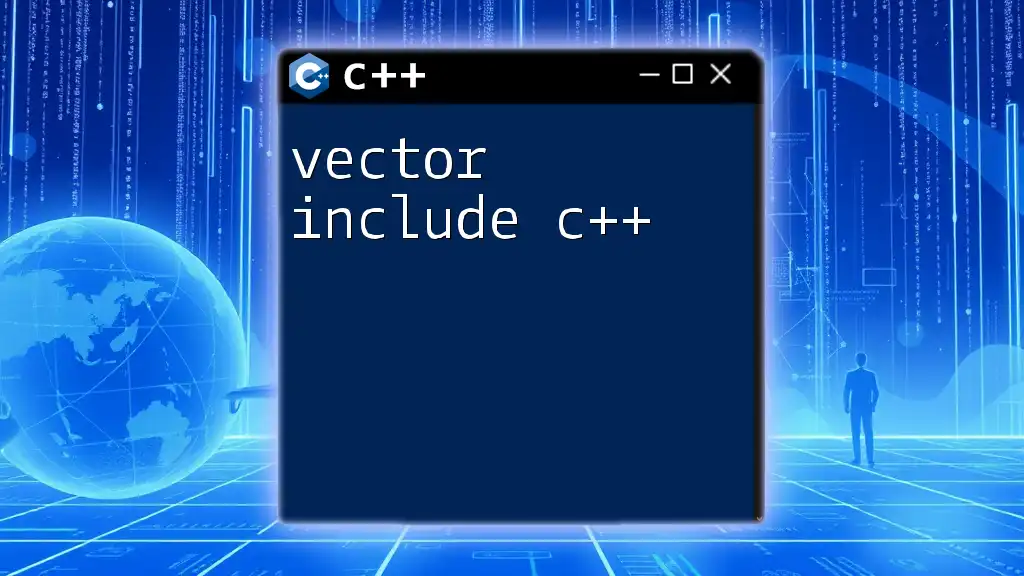
Using the #include Directive
The syntax for the `#include` directive is straightforward but powerful:
#include <iostream>
This line indicates that the program needs access to the Standard Input/Output Stream library to use functions like `cout` and `cin`.
Differentiating Between Angle Brackets and Quotes
Depending on where the file is located, the `#include` directive can use two different syntaxes:
-
Angle Brackets: When using angle brackets (e.g., `<file>`), the preprocessor searches for the file in the system's standard library directories. This is the typical method to include system libraries or standard libraries.
-
Double Quotes: Using double quotes (e.g., `"file"`) tells the preprocessor to first look in the directory of the current file before moving to the standard library paths. This syntax is primarily used for custom headers.
Standard Library Includes
The C++ Standard Library provides many functionalities that are critical for developing robust applications. Some commonly included standard library headers are:
- `<iostream>` for input and output operations
- `<vector>` for dynamic arrays
- `<string>` for string manipulation
Here’s an example using `iostream`:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, we include the `iostream` library and use `cout` to display text on the console—demonstrating how includes make it easier to perform basic tasks.
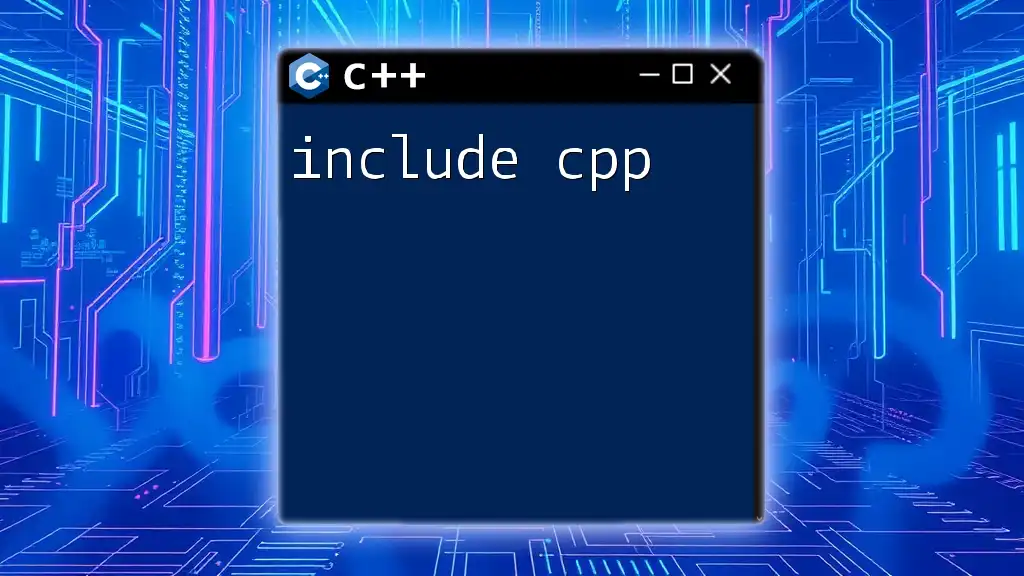
Creating Your Own Header Files
Creating a custom header file allows for better organization of your code. To create a header file, follow these steps:
- Create a new file, e.g., `my_functions.h`, and define any functions or classes you want to reuse:
// my_functions.h
void greet();
- To use this custom header in a C++ source file, include it as shown below:
#include "my_functions.h"
#include <iostream>
using namespace std;
void greet() {
cout << "Hello from my function!" << endl;
}
int main() {
greet();
return 0;
}
In this example, `greet()` is declared in the header file and defined in the source file. This highlights how you can modularize your code by separating declarations from implementations.
Best Practices for Using Includes
To make the best use of includes in C++, follow these best practices:
-
Avoid Circular Includes: Circular includes occur when two files include each other directly or indirectly, leading to compilation errors. To manage dependencies effectively, consider using include guards or the `#pragma once` directive.
-
Keep Includes Organized and Minimal: Only include headers that are necessary for your code. This reduces compilation time and minimizes the risk of unnecessary dependencies.
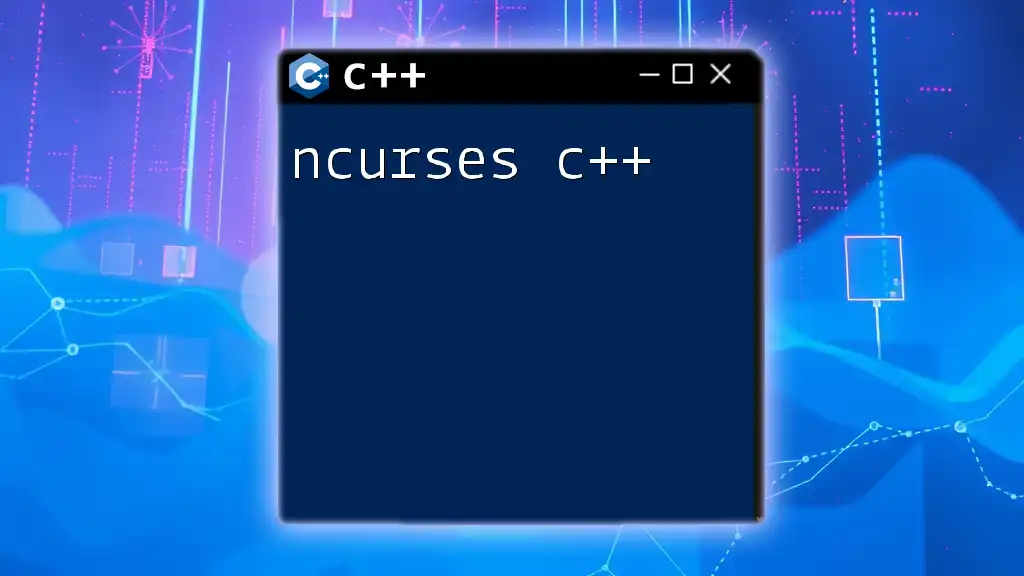
Conditional Compilation with Includes
Conditional compilation allows developers to include certain sections of code based on specific conditions. This is especially useful for creating platform-specific code or for debugging purposes.
You can use preprocessor directives like `#ifndef`, `#define`, and `#endif` to prevent multiple inclusions of a file:
#ifndef MY_FUNCTIONS_H
#define MY_FUNCTIONS_H
void greet();
#endif
In this example, header guards ensure that the contents of `my_functions.h` are only included once, preventing conflicts that could arise from multiple definitions.
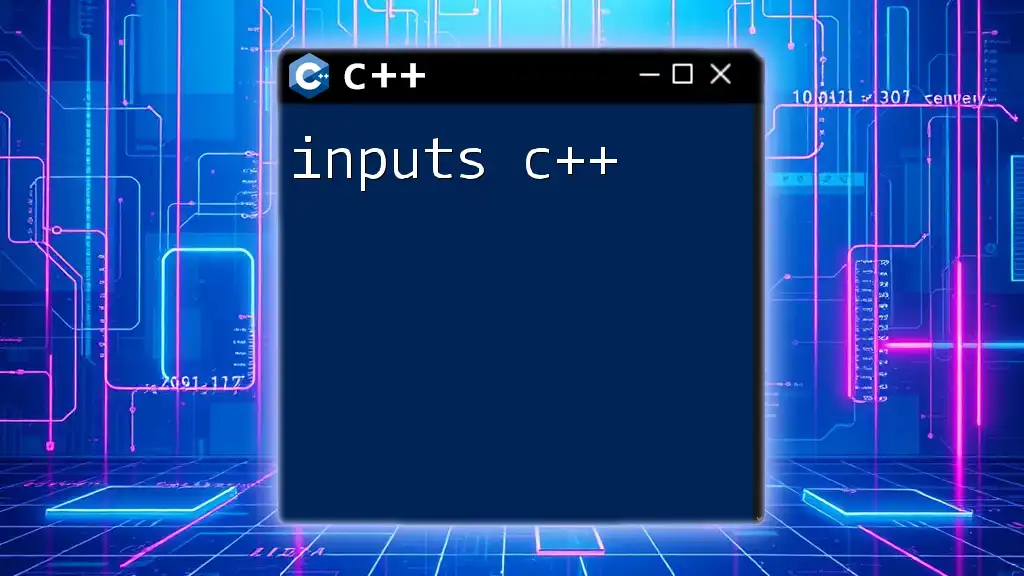
Common Mistakes and How to Avoid Them
When working with includes in C++, developers often encounter several common pitfalls. Here are some mistakes to watch out for:
-
Forgetting to Include Necessary Headers: Always check that you have included the correct libraries required for the functions or features you are using. Missing includes can lead to compile-time errors.
-
Incorrect File Paths or Filenames: Ensure that your custom header files are located in the appropriate directories. If using double quotes, the preprocessor will start searching for the file in the current directory first.
-
Redundant Includes: Remove unnecessary include statements that do not serve any purpose. Tools like `clang-tidy` can help identify and clean up redundant includes in your code.
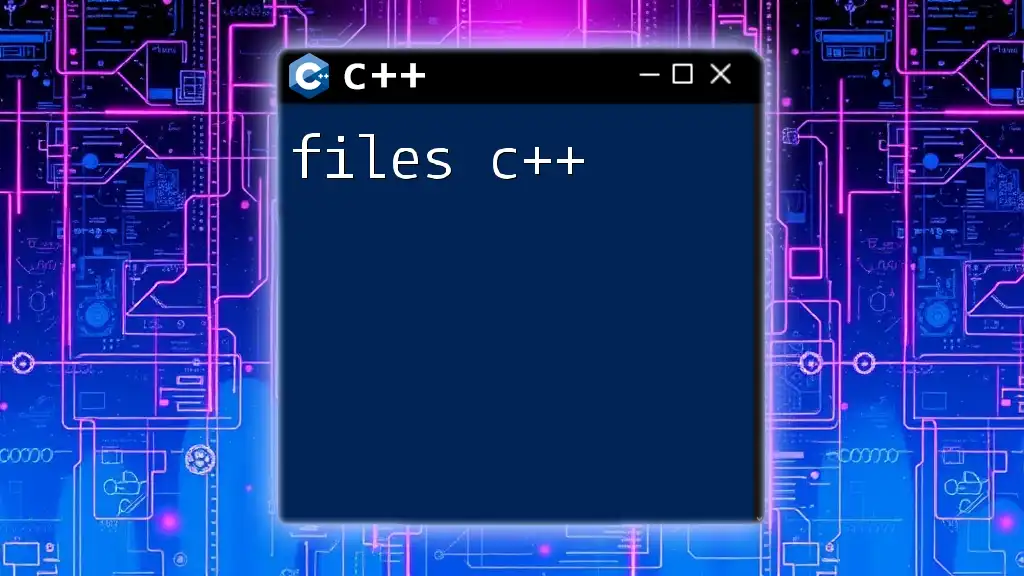
Conclusion
In conclusion, understanding how to effectively use includes in C++ is vital for building well-structured programs. By mastering the `#include` directive, you not only promote code reusability but also foster modularity in your coding practices. It’s essential to follow best practices, manage dependencies appropriately, and avoid common mistakes associated with includes.
Now is the perfect time to practice with includes in your own projects, ensuring you consolidate your knowledge and skills in this fundamental area of C++ programming.