The `#include <iostream>` directive in C++ is used to include the standard Input/Output stream library, allowing the use of features like `cout` for outputting data to the console.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `#include <iostream>`?
Definition of Header Files
Header files in C++ are essential components that contain declarations for functions and classes. They serve as a bridge between various parts of a C++ program, allowing you to reference code without having to rewrite it. By including header files, you can leverage pre-existing functionalities and simplify your code.
Purpose of `iostream`
The `iostream` header file is specifically designed for input and output operations in C++. It encapsulates the stream classes that are foundational for any interaction with standard input and output devices, including the keyboard and monitor. Understanding `iostream` is crucial, as it allows you to perform various operations, such as reading user input and displaying output on the screen.
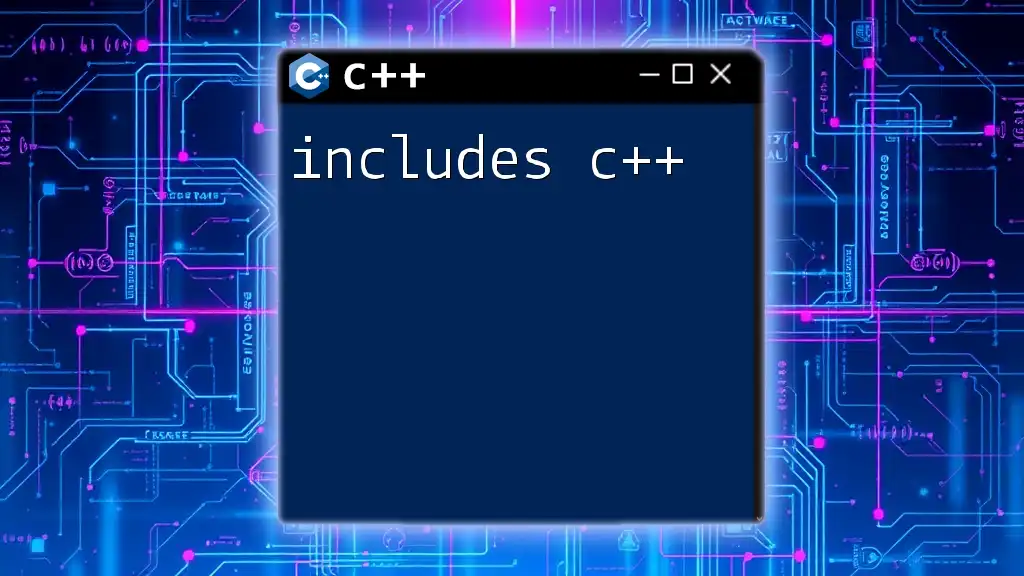
How to Include `iostream` in Your C++ Programs
Basic Syntax
To utilize the functionalities provided by the `iostream` library, you need to include it at the beginning of your C++ program. The syntax is straightforward:
#include <iostream>
Placing this line at the top of your code enables you to make use of its features throughout your program.
Proper Placement in Your Code
The `#include` statement should always be placed before the main function and any other code segments. This practice ensures that the compiler recognizes the library and its functionalities before executing the rest of the code. Always remember to position your `#include` statements at the top of your C++ files for clarity and organization.
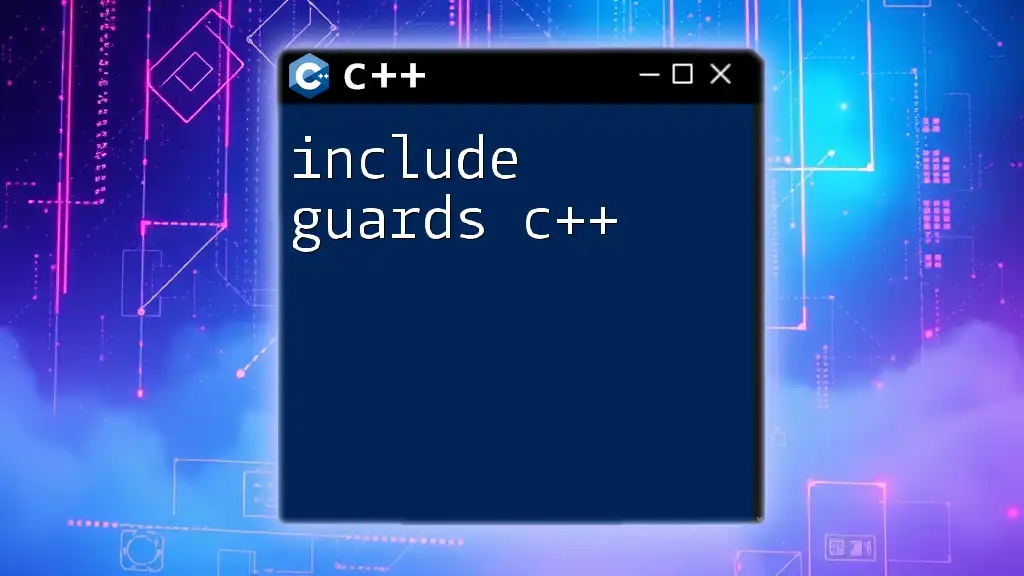
Core Functionalities of `iostream`
Input and Output Streams
At the core of `iostream` are the concept of streams. Think of a stream as a flow of data: you can read from an input stream or write to an output stream. In C++, streams provide an efficient and effective way to process data, making input and output operations seamless.
Understanding `cin` and `cout`
The two principal components of the `iostream` library are:
- `cin`: This stands for character input and is used to receive input from the user.
- `cout`: This stands for character output and is used to display output to the user.
Code Snippet Example
To illustrate how to use `cin` and `cout`, consider the following code snippet:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In this example, the program prompts the user to enter a number, stores the user input in the variable `number`, and then outputs it back to the console. This simple interaction demonstrates the fundamental utilities provided by `iostream`.
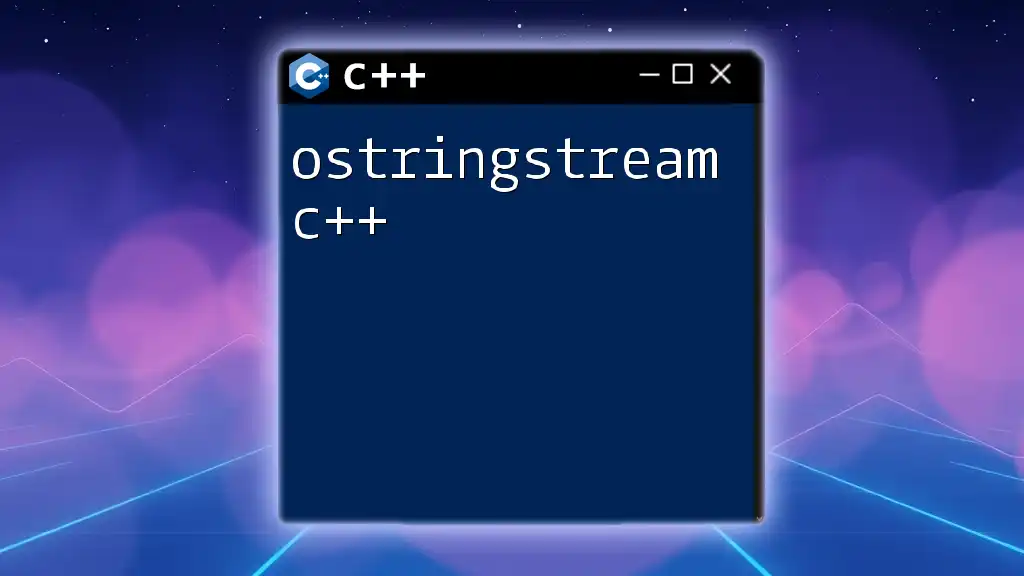
Error Handling with `iostream`
Importance of Error Checking
Error handling is a vital part of any program, especially to ensure robustness. When dealing with user inputs, it's crucial to handle errors gracefully to prevent crashes or undefined behavior.
Using `cin.fail()`
The method `cin.fail()` is instrumental in error handling. It checks whether the previous input operation failed due to invalid data types or other similar issues. By incorporating it, you can make your programs more resilient.
Example: Error Handling with `cin`
The following example presents a way to handle invalid inputs effectively:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
while (!(cin >> number)) {
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard the input
cout << "Invalid input. Please enter a number: ";
}
cout << "You entered: " << number << endl;
return 0;
}
In this code, a `while` loop continues to prompt the user until they input a valid number, demonstrating excellent error handling practices in C++.
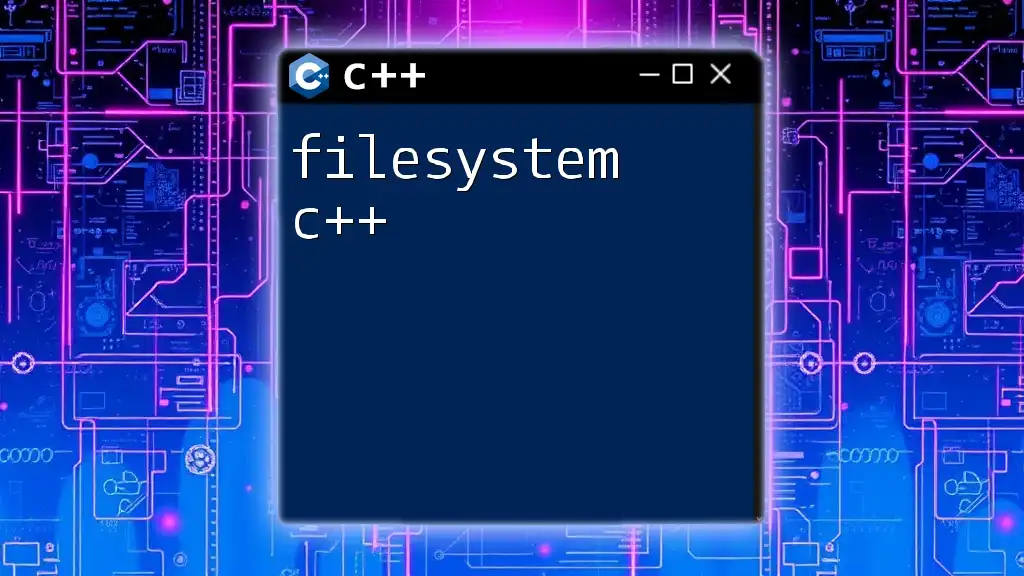
Advanced Input/Output Using `iostream`
Working with Strings
Beyond basic integer and character data types, `iostream` also supports string inputs and outputs. This capability allows you to handle textual data seamlessly, enhancing the versatility of your applications.
Example: String Input/Output
Here’s an example demonstrating how to handle string inputs:
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
getline(cin, name); // Using getline to capture full line input
cout << "Hello, " << name << "!" << endl;
return 0;
}
In this example, `getline` captures an entire line of user input, accommodating names with spaces, which adds more flexibility to user interactions.
Using `endl` vs. `\n`
When it comes to ending a line, you have two main options: `endl` and `'\n'`. While both serve to create a new line, `endl` not only moves the cursor to the next line but also flushes the output buffer. This may impact performance if used excessively. In contrast, `'\n'` merely inserts a new line without flushing the buffer, making it more efficient for frequent uses.
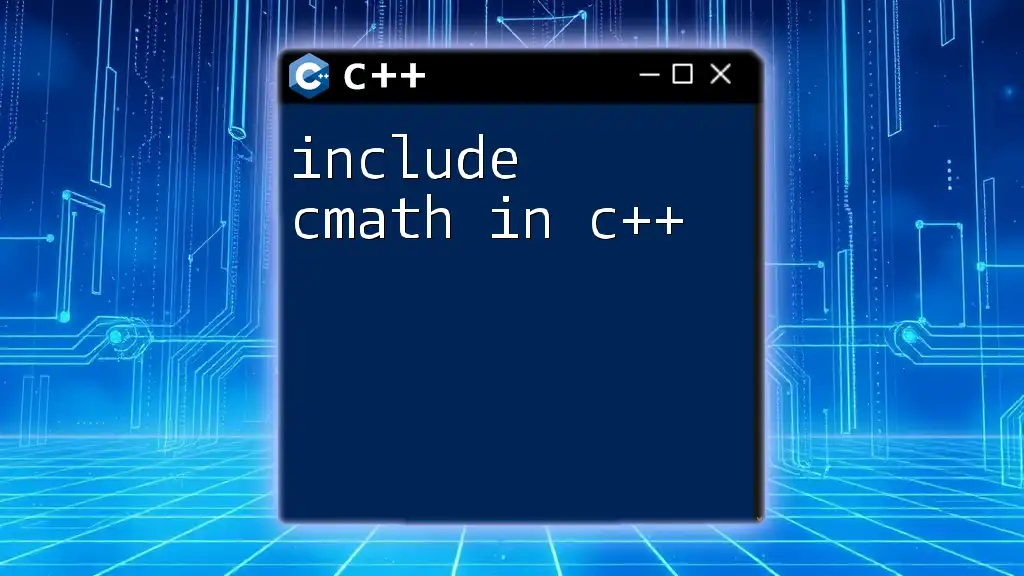
Customizing Output with `iostream`
Formatting Output
The `iostream` library provides manipulators that allow you to format your output. Functions like `std::fixed` and `std::setprecision` enable you to control how your output is displayed, particularly with floating-point numbers.
Example: Formatting Floating-Point Numbers
The following example illustrates how to format a floating-point number for better readability:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
double pi = 3.141592653589793238;
cout << fixed << setprecision(2); // Fix to 2 decimal places
cout << "Value of pi: " << pi << endl;
return 0;
}
In this example, `setprecision(2)` formats the output to display the value of pi with two decimal places, enhancing clarity and presentation.
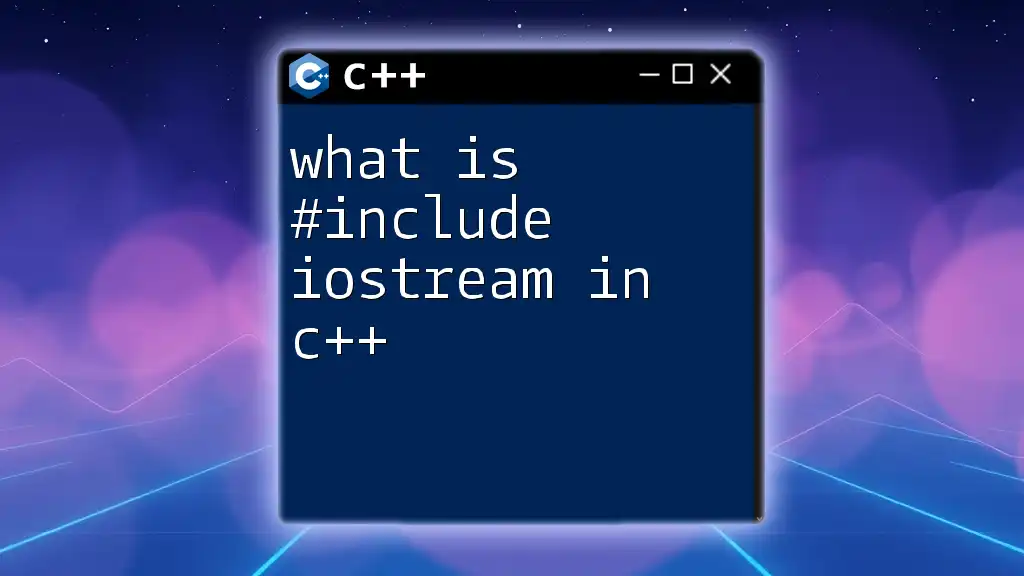
Conclusion
Understanding how to include iostream in C++ is paramount for effective input and output operations. Mastery of this library not only equips you with the necessary tools to interact with users but also enhances the overall efficiency and usability of your programs. Whether you are handling basic data types or delving into more complex string manipulations, `iostream` provides the foundation for seamless communication between your program and its users.
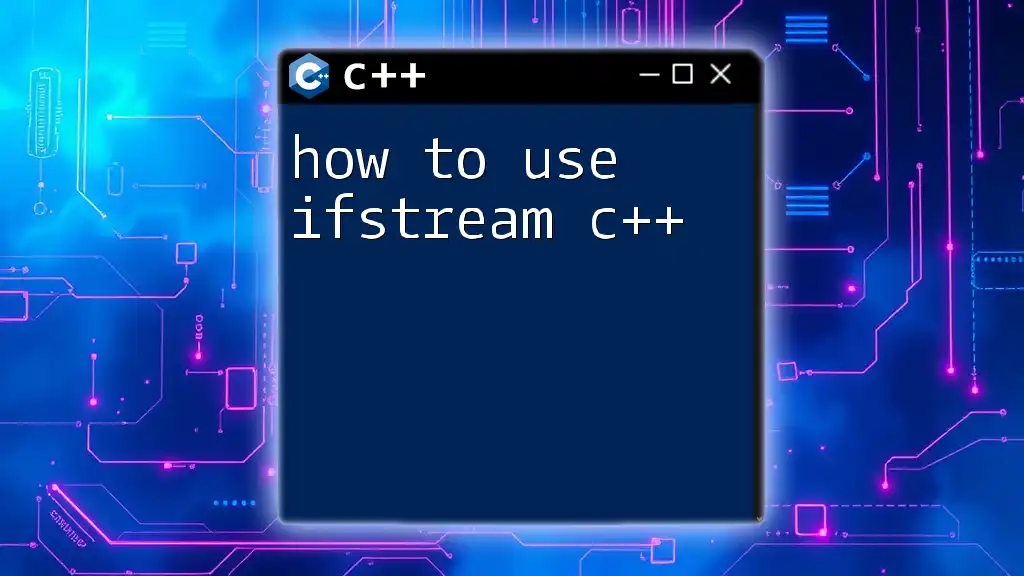
Additional Resources
For those eager to deepen their understanding of C++ and `iostream`, consider exploring resources such as recommended books, online tutorials, and interactive coding platforms. Engaging with diverse learning materials will further solidify your grasp of this fundamental aspect of C++.