The `#include <iostream>` directive in C++ imports the standard input/output stream library, enabling the use of features like `std::cout` and `std::cin` for console input and output.
Here’s a code snippet to demonstrate its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a Header File?
Definition of Header Files
In C++, a header file is a file that contains declarations of functions, classes, and variables. It acts as an interface between the programmer and the built-in functions of the C++ language. By organizing and separating code, header files help maintain clarity and allow for easy reuse of code.
The Role of #include Directives
The `#include` directive tells the compiler to include the contents of a specified file when a program is compiled. This is essential for accessing library functions and allows programmers to take advantage of existing code, thereby enhancing productivity and code maintainability.
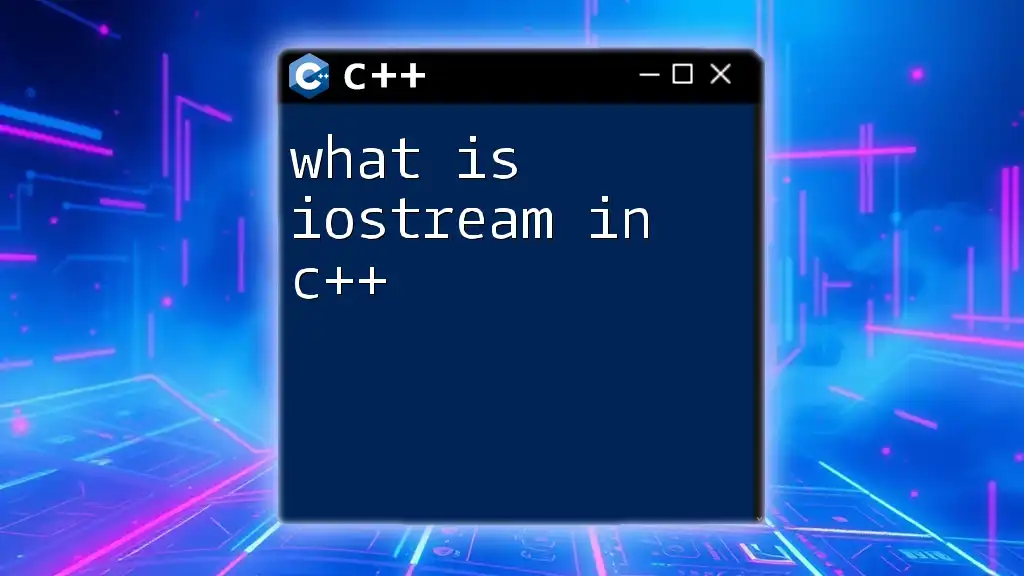
Understanding the iostream Library
What is iostream?
The `iostream` library is one of the standard libraries in C++. It provides essential features for handling input and output operations. Specifically, it encompasses functionalities that allow for reading data from the console (input) and writing data to the console (output). This library forms the backbone of data exchange between a user and a C++ application.
Components of iostream
Standard Input and Output Streams
The `iostream` library primarily consists of standard input and output streams:
- `cin`: This is used to read input from the standard input device, usually the keyboard. For example, when you want to get data from users.
- `cout`: This is used to write output to the standard output device, usually the console. It’s used for displaying information back to the user.
Other Components
In addition to `cin` and `cout`, the iostream library also includes:
- `cerr`: Stands for "character error" output stream. It is used to display error messages directly to the user. Output through `cerr` is unbuffered, meaning it is displayed immediately.
- `clog`: Similar to `cerr`, it is used for logging purposes. Output through `clog` is buffered, making it useful for performance optimization.
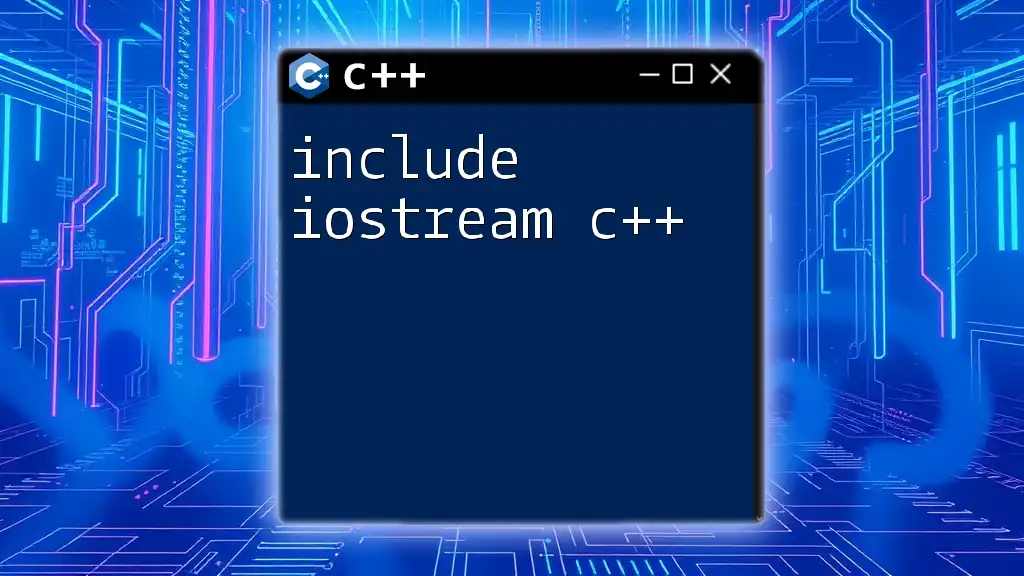
How to Use #include <iostream>
The Syntax
The syntax for including the iostream library in your C++ program is simple:
#include <iostream>
This line of code should be placed at the top of your C++ source file to make the iostream functionality available throughout your program.
Including iostream in Your Program
When you include the iostream library in a C++ program, it enables the use of input and output streams directly. Below is a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this simple program, we use `cout` to print "Hello, World!" to the console, demonstrating the basic functionality of the iostream library.
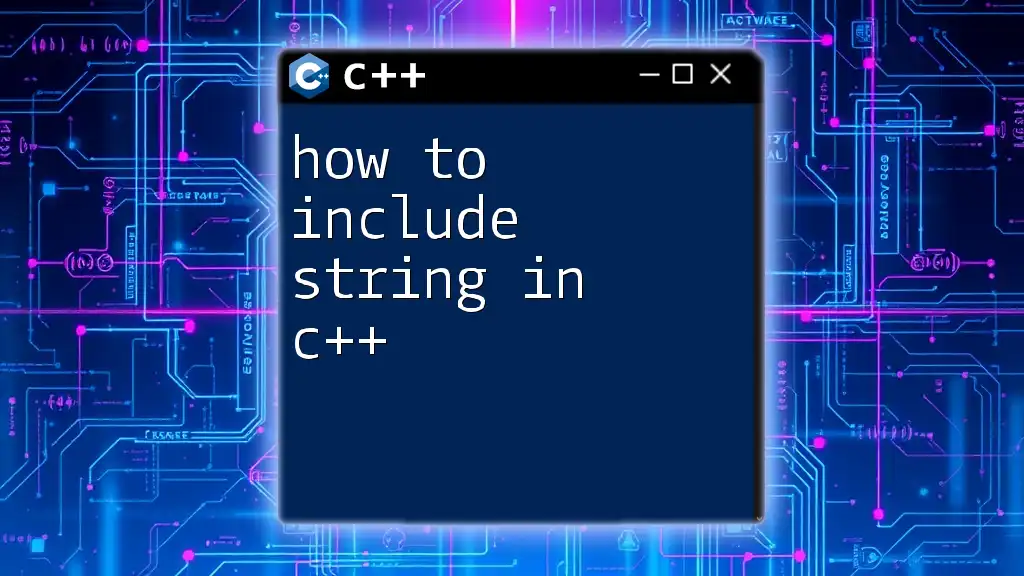
The Standard Namespace
What is the Standard Namespace?
The `std` namespace is where the C++ Standard Library’s entities (functions, classes, etc.) are defined. To use these entities without prefixing them with `std::`, understanding the namespace becomes essential.
How to Use std with iostream
When using components from the iostream library, you typically prefix them with `std::`. For example:
std::cout << "Enter your name: ";
std::string name;
std::cin >> name;
Here, `std::cout` and `std::cin` clearly indicate that these components belong to the `std` namespace.
Alternative: Using `using` Directive
To simplify your code, you can use the `using` directive which allows you to use `cout` and `cin` without the `std::` prefix. This can make your code cleaner and easier to read. Here’s how you can employ it:
using namespace std;
int main() {
cout << "Enter your age: ";
int age;
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
Though convenient, using `using namespace std;` can lead to naming conflicts, so it should be used judiciously.
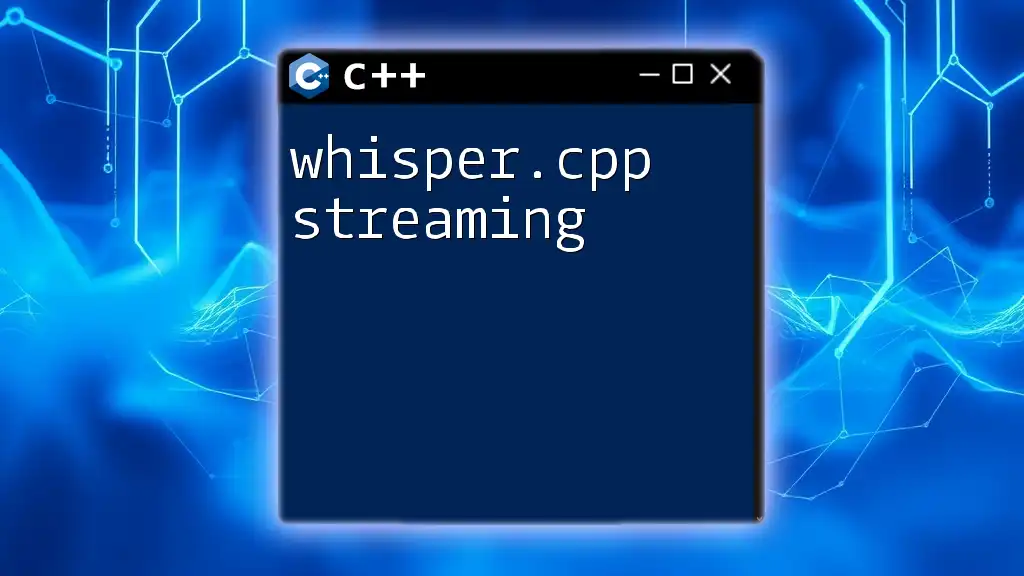
Practical Example Using #include <iostream>
Complete Program Demonstration
Let’s consider a practical coding example that combines user input and output:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this example:
- The program prompts the user for their age.
- It captures the input and then displays it back to the user.
Explanation of Code
- Line 1: The program starts by including the iostream library to access the input/output stream functions.
- Line 2: The `using namespace std;` directive allows the use of standard library objects directly.
- Lines 3-5: The `main` function is defined, which is the entry point of the program.
- Line 4: Displays the prompt "Enter your age: ".
- Line 5: The program waits for user input and stores it in the variable `age`.
- Line 6: It outputs the statement, "You are X years old", dynamically replacing X with the age entered by the user.
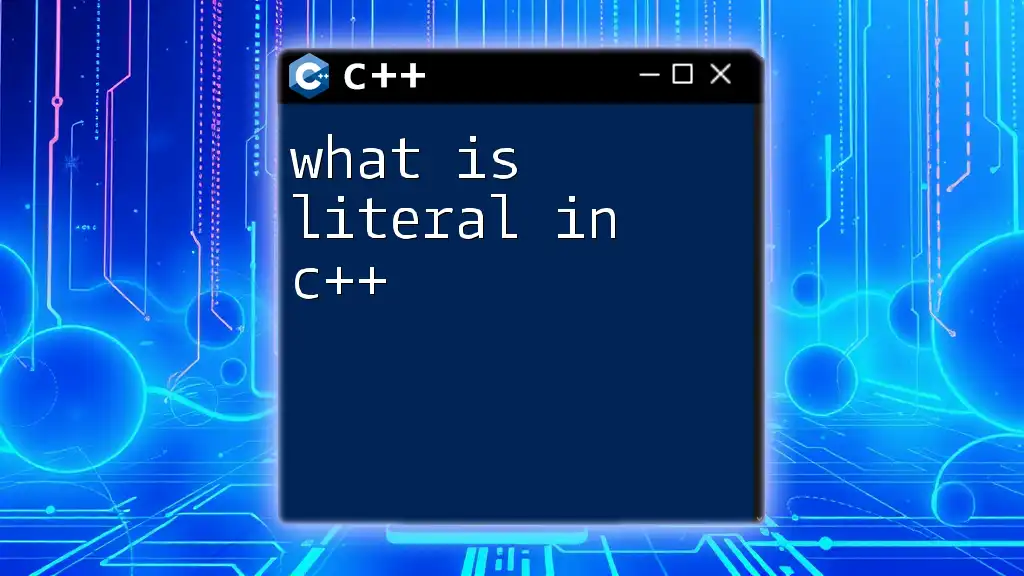
Common Mistakes with #include <iostream>
Forgetting the `std` Prefix
One common error is forgetting to use the `std` prefix when trying to use `cout` or `cin`, leading to compilation errors. For example, if you try:
cout << "Hello!";
You might encounter an error indicating that `cout` is not defined. The correct line should be:
std::cout << "Hello!";
Including iostream in the Wrong Place
Another mistake is including `#include <iostream>` in the wrong place in your source file. Always include it at the top of your file, well before any function definitions. This ensures that the compiler recognizes the functions and streams when it parses the code.
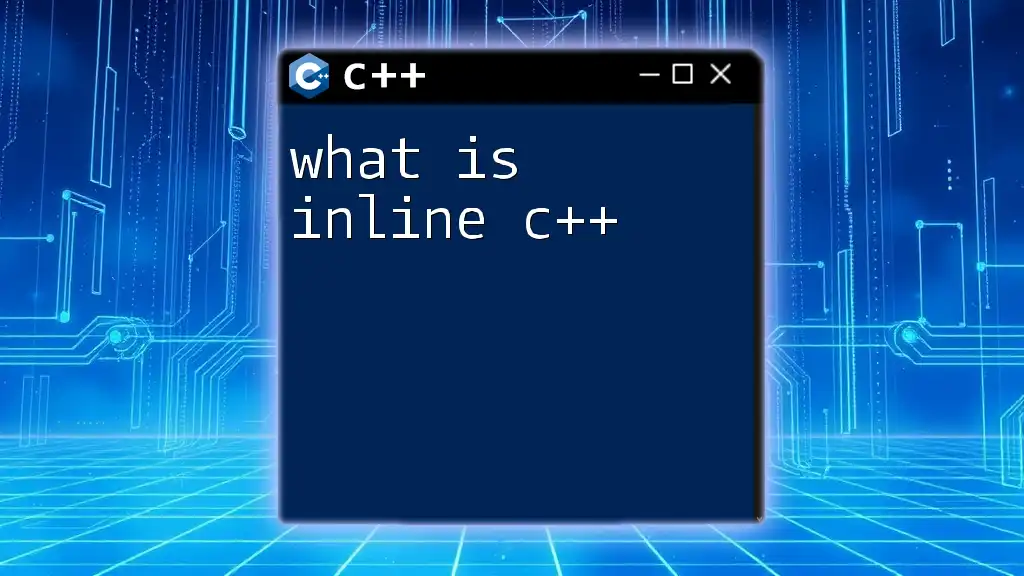
Conclusion
Understanding what is #include iostream in C++ is fundamental for anyone wishing to master this programming language. The `#include <iostream>` directive allows programmers to leverage powerful input and output functionalities, enabling dynamic interaction with users through the console. Mastering iostream not only enhances your C++ coding skills but also serves as a gateway to understanding more complex programming concepts. Experimenting with this library and its various components will provide invaluable hands-on experience vital for any budding C++ developer.