`iostream` is a header file in C++ that provides functionalities for input and output through streams, allowing the use of objects like `cin` for input and `cout` for output.
Here's a simple code snippet demonstrating its use:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of Input and Output
What is Input and Output in Programming?
Input and output (I/O) in programming refer to the methods through which a program interacts with its environment, often communicating with the user or other software systems. Input might come from a user typing on a keyboard, a file being read from disk, or data received over a network. Conversely, output is how a program communicates results back to the user, whether through displaying information on a screen or writing to a file.
Why C++ Uses iostream?
C++ evolved from the C programming language, which utilized functions like `printf` and `scanf` for formatted output and input, respectively. The advent of C++ brought about a more sophisticated model of I/O that is type-safe, object-oriented, and easier to use. The iostream library enhances the programming experience by allowing developers to use a unified approach for both input and output through the use of streams, providing a more seamless integration with object-oriented programming principles.
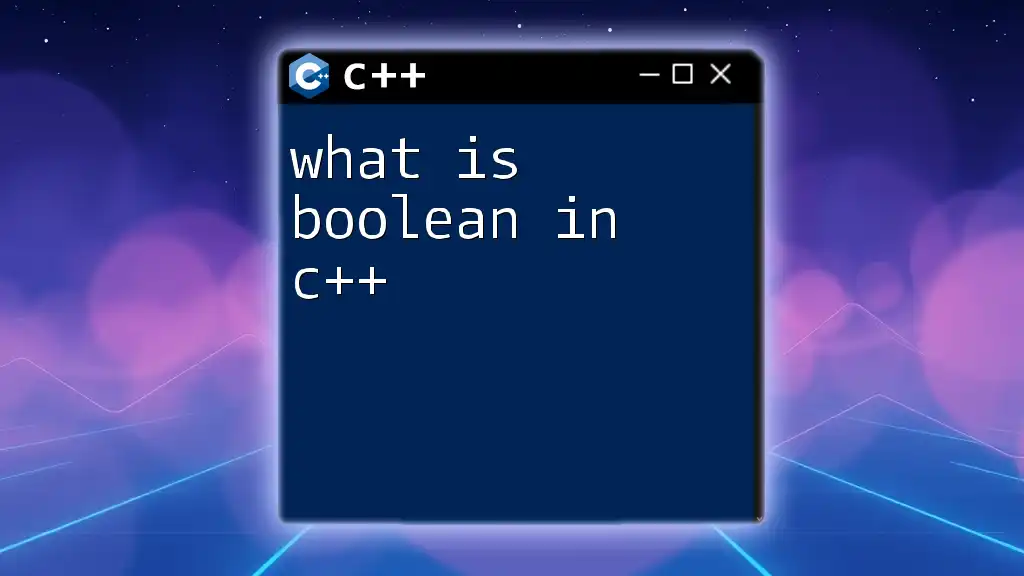
What is iostream?
Definition of iostream
The term iostream refers to a family of classes in the C++ standard library designed to handle input and output. It acts as the bridge between a program and various data sources or destinations, allowing for standardized and formatted data manipulation. The library offers different types of streams, most notably input streams (istream) and output streams (ostream), each with specialized functions.
Header Files and Namespaces
To utilize the iostream library, one must include the `<iostream>` header file in the program:
#include <iostream>
Additionally, using the `std` namespace is common practice, enabling access to standard library components without prefixing them:
using namespace std;
This practice allows the programmer to use `cout` and `cin` directly, avoiding potential name conflicts or confusion.
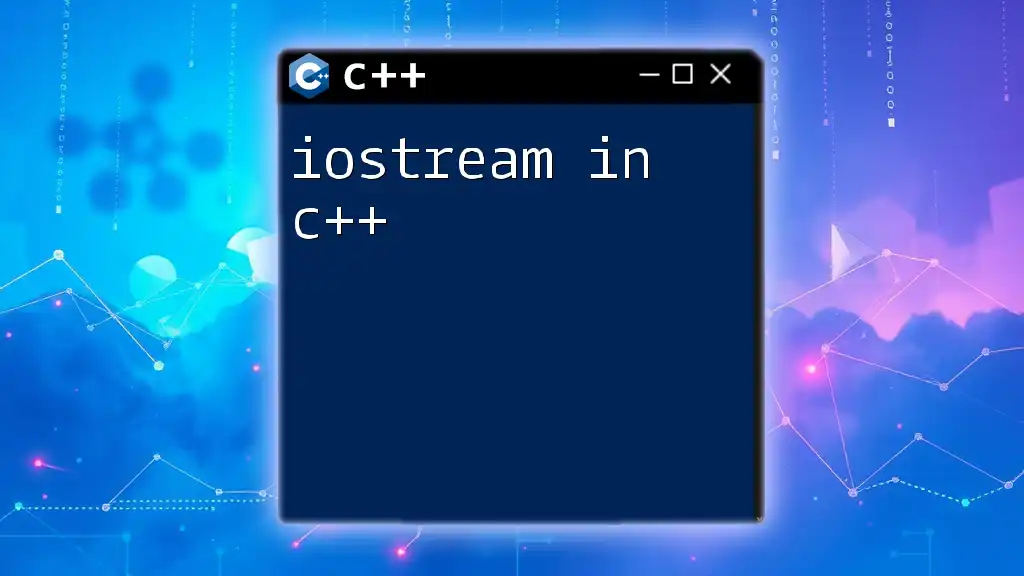
Core Components of iostream
Input Stream (istream)
The input stream, represented by the `istream` class, is pivotal for reading data into a program. It interacts primarily through the `cin` object, allowing developers to capture user inputs efficiently.
The primary functions of `istream` include methods like `>>`, which extracts data from the input buffer. For example, the following code snippet captures an integer from user input:
int main() {
int num;
cout << "Enter a number: "; // Output prompt to the user
cin >> num; // Input capture
return 0;
}
Output Stream (ostream)
The output stream, handled by the `ostream` class, focuses on sending data out of a program. The `cout` object is commonly used to display information to the user.
Key functions of `ostream` involve the `<<` operator, which sends data to the output buffer. Here’s how you might display a message:
int main() {
cout << "Hello, World!" << endl; // Simple output to the console
return 0;
}
Combined Stream (iostream)
iostream serves as the combined class for both input and output streams. The `cin` and `cout` objects allow developers to work with both input and output in a seamless manner.
Here's an example that showcases both operations within a single program:
int main() {
string name;
cout << "Enter your name: "; // Output prompt
cin >> name; // Input capture
cout << "Hello, " << name << "!" << endl; // Combined output
return 0;
}
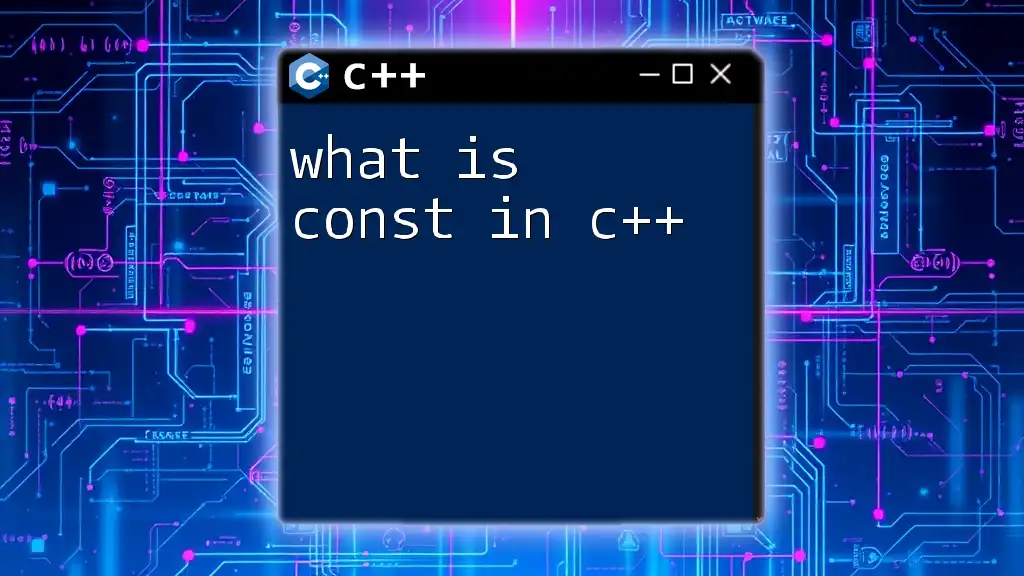
iostream Functions and Manipulators
Essential Functions in iostream
The iostream library comprises several essential functions, allowing for efficient data handling. The key functions include:
- `cin`: Standard input stream used to capture user input.
- `cout`: Standard output stream used to display data to the console.
- `cerr`: Standard output stream for errors, unbuffered for immediate feedback.
- `clog`: Buffered stream for logging messages, typically used for debugging.
Each of these functions has its unique characteristics, especially concerning buffering and output behavior.
Manipulators in iostream
Manipulators are special functions in iostream that alter the formatting of the output. They help in enhancing readability and tailoring output for specific requirements.
Common manipulators include:
- `endl`: Inserts a new line and flushes the output buffer.
- `setw(n)`: Sets the width of the next output to `n` spaces.
- `setprecision(n)`: Configures the decimal precision for floating-point numbers.
Here’s a practical example of using manipulators:
#include <iostream>
#include <iomanip> // for std::setw and std::setprecision
int main() {
double pi = 3.14159;
cout << "Value of pi: " << setprecision(2) << fixed << pi << endl; // Manipulator example for precision
return 0;
}
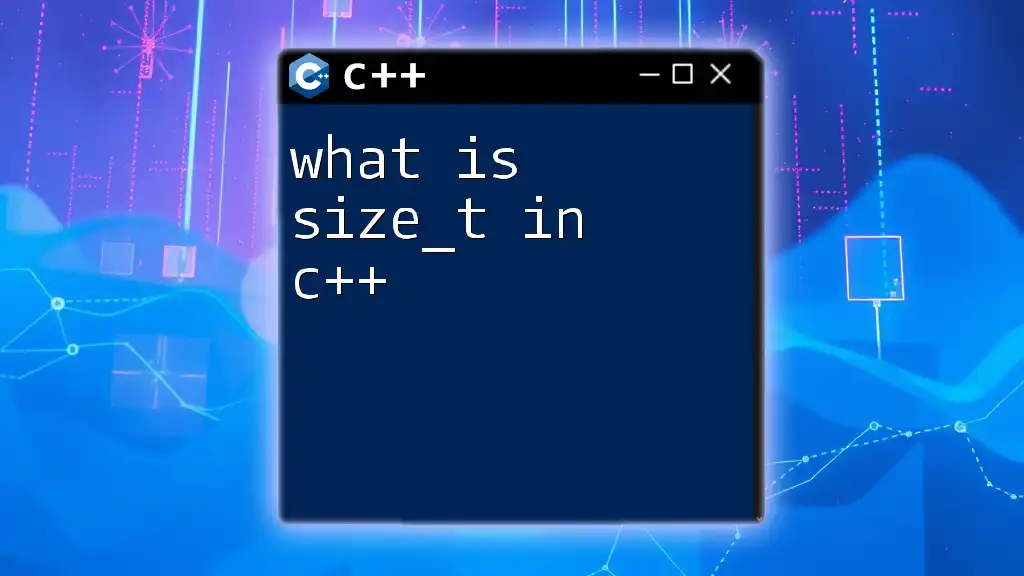
Error Handling in iostream
Understanding Error States
Robust programs often need to handle potential errors, especially during user input. The iostream library provides mechanisms to check for various error states, such as input failure. When the program attempts to read invalid data, it is crucial to manage the error to maintain stability.
Checking the error states allows developers to ensure input validity. Here’s an example that illustrates error handling while reading input:
int main() {
int num;
cout << "Enter a number: ";
while (!(cin >> num)) {
cout << "Invalid input. Please enter a number: ";
cin.clear(); // Clears the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignores bad input
}
cout << "You entered: " << num << endl;
return 0;
}
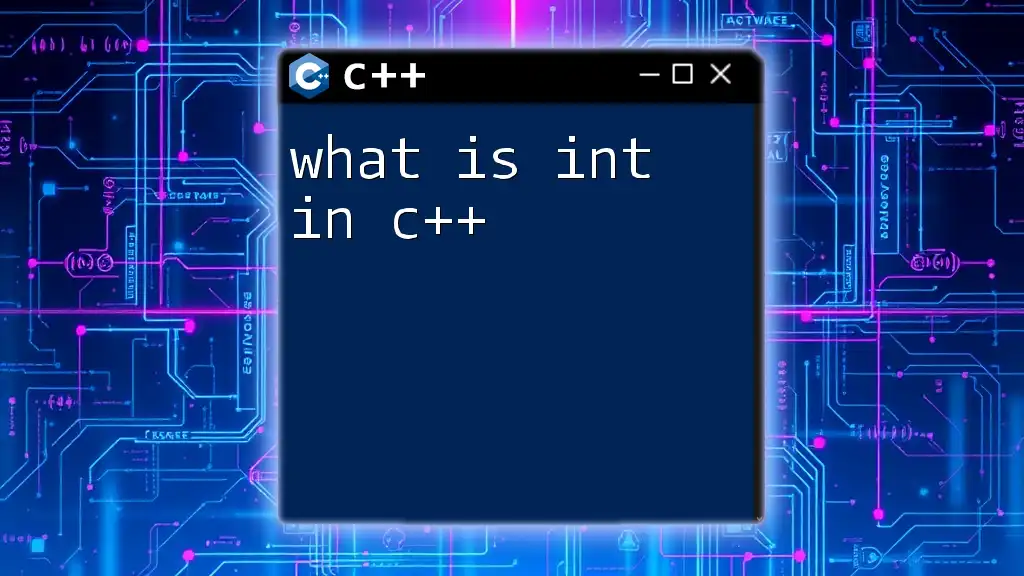
Best Practices When Using iostream
Tips for Effective Input/Output Operations
To produce clean and effective input/output operations, consider these best practices:
- Always prompt the user: Use clear, concise messages to inform users what is expected.
- Manage input errors effectively: Enhance user experience by providing meaningful feedback on invalid inputs.
- Use manipulators judiciously: Apply formatting functions to improve output readability without overcomplicating the output.
Performance Considerations
While the iostream library provides an efficient way to handle I/O operations, performance can be impacted in scenarios with extensive I/O operations. For highly I/O-bound applications, consider alternatives, such as using file streams or buffered I/O to enhance efficiency.
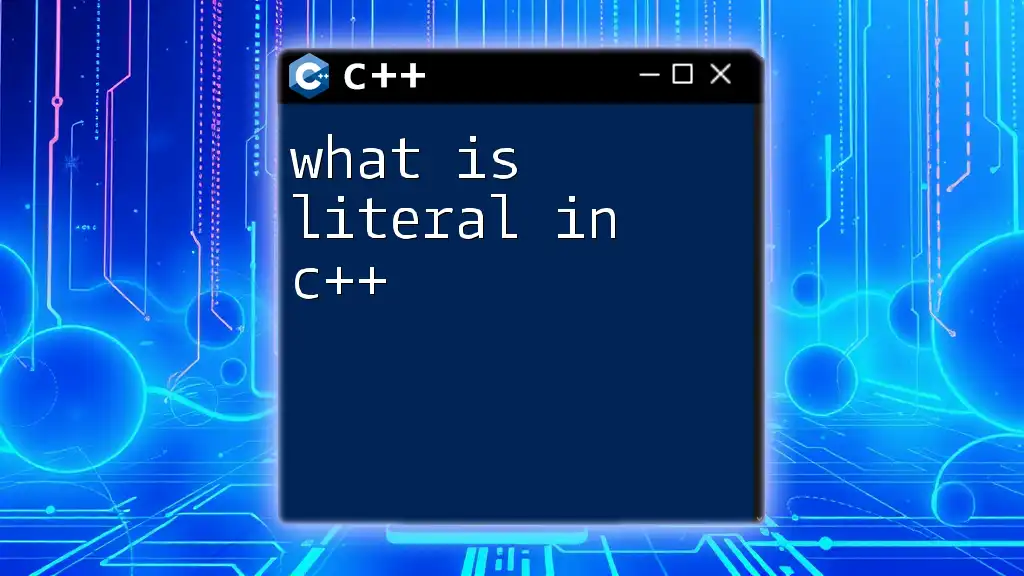
Conclusion
The iostream library is a vital component of C++ that empowers developers to manage input and output with ease. By understanding its core components, functions, and best practices, programmers can create more robust and user-friendly applications.
Encouragement to explore further, experimenting with various features of iostream, will lead to deeper mastery of input and output operations in C++. There are many resources available for those wishing to expand their knowledge further.