The `iostream` library in C++ facilitates input and output (I/O) operations through standard streams like `cin` for input and `cout` for output.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Outputs "Hello, World!" to the console
return 0;
}
What is iostream?
The iostream library in C++ is an essential component that provides the standard input and output functionalities. At its core, it allows programmers to perform operations involving data input and output with ease. Understanding the workings of iostream is crucial as it serves as the foundation for interacting with users and external files in a C++ application.
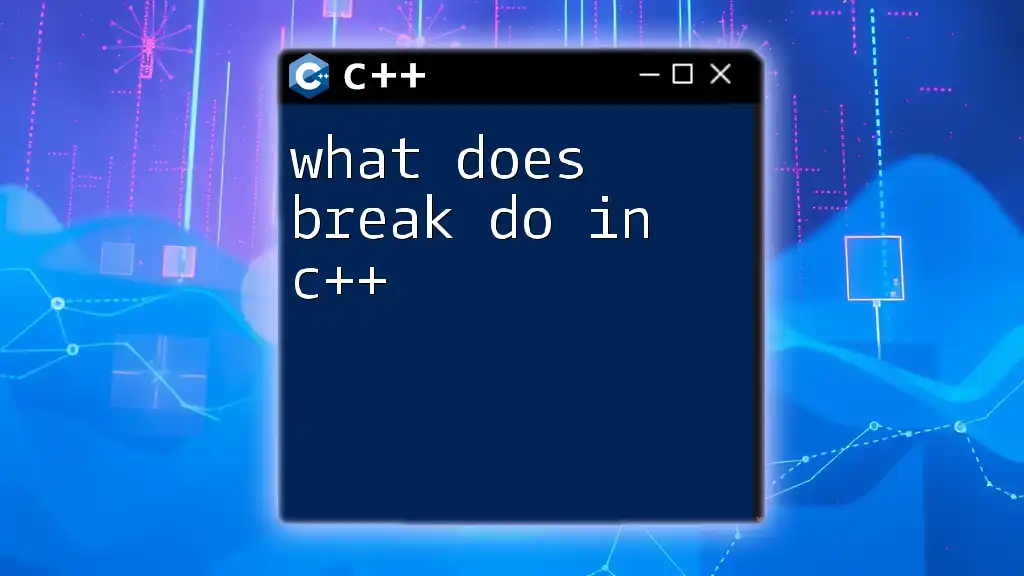
Origins and Evolution
The iostream library has its roots in early C++ programming, evolving significantly through its various standards. Originally derived from the C standard library, it was redesigned to incorporate features of C++, including type safety, objects, and functionality. The evolution of C++ standards has only solidified the importance of iostream, making its understanding pivotal for both new and experienced programmers.
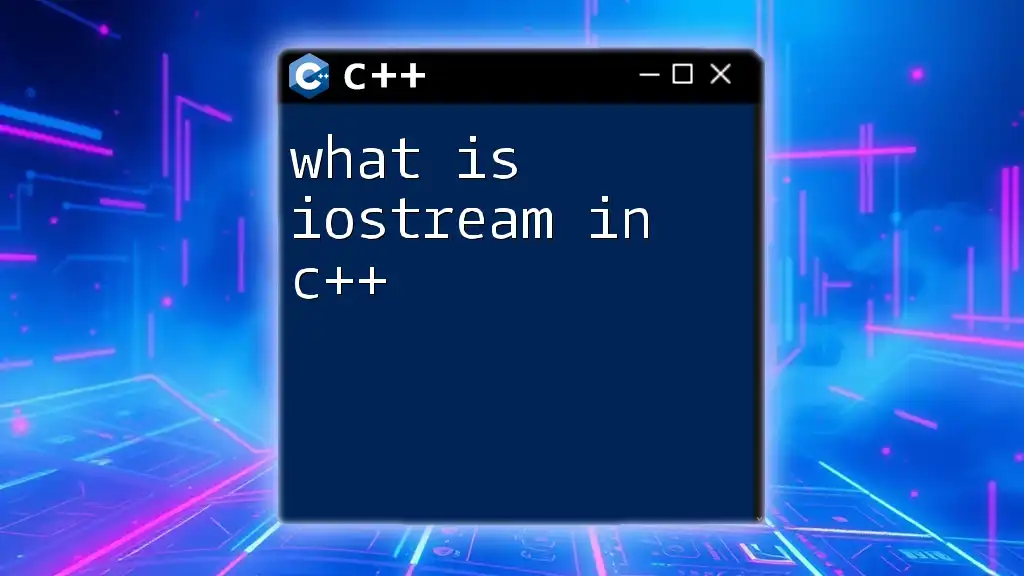
Components of iostream
Overview of Standard Input/Output Streams
Streams in programming are abstractions that represent the flow of data. In C++, there are mainly two types of streams relevant to beginners:
- Input Streams: These are used to receive data from input devices, such as keyboards or files.
- Output Streams: These are used to send data to output devices, such as screens or files.
Main Headers in the iostream Library
The iostream library includes several important headers:
-
iostream: This header file is at the heart of C++ input and output functionality, integrating both input and output streams.
-
istream: It specializes in input stream functionalities, allowing for reading data from different sources.
-
ostream: This handles output stream functionalities, enabling data to be written to output devices.
Other Related Headers
-
fstream: This header allows you to perform file input and output operations.
-
sstream: This header provides functionalities for string input and output, allowing for more flexible data manipulation.
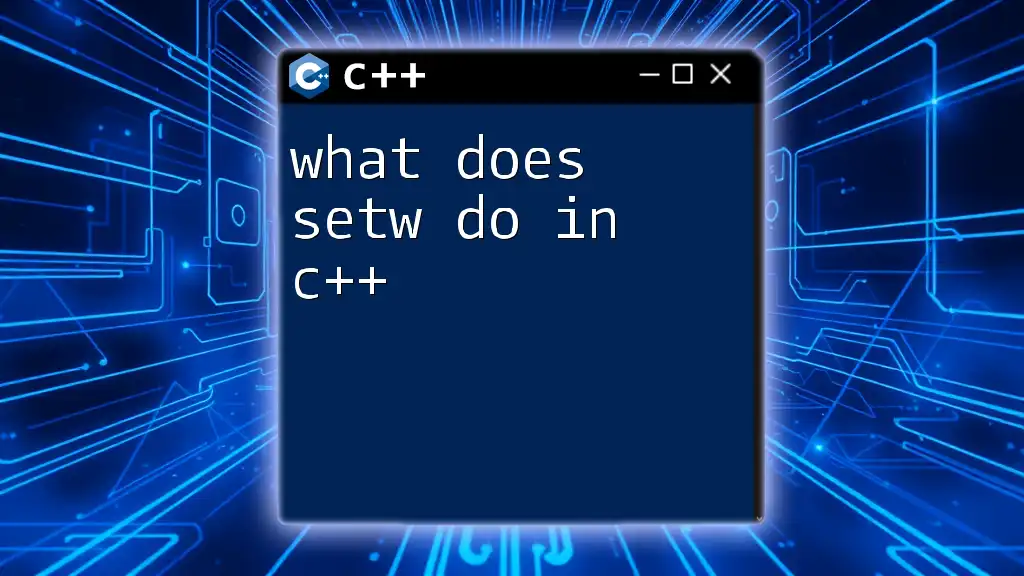
Core Concepts of iostream
Input and Output Streams Explained
Understanding how streams work is fundamental in mastering iostream. Streams operate using a concept known as buffered input/output, where data is collected in a buffer before being processed. This method increases the efficiency of I/O operations.
Furthermore, streams maintain various states which help manage and diagnose issues:
- Good State: Indicates no errors, and the stream is able to perform I/O operations.
- End-of-File State (eof): Signifies that all data has been read from the stream.
- Fail State: Indicates that a non-recoverable error occurred during I/O operations.
Standard Input and Output Objects
Two primary objects are fundamental when using the iostream library: cout and cin.
cout: The Standard Output Stream
The cout object allows you to send data to the standard output (usually the console). It uses the insertion operator (`<<`) for output.
Here’s a code example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, "Hello, World!" is printed to the console using cout.
cin: The Standard Input Stream
The cin object is instrumental for receiving input from the user. It uses the extraction operator (`>>`) to read data.
Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "Your age is " << age << endl;
return 0;
}
The program prompts the user for their age, captures the input using cin, and then outputs it.
Using cin and cout Together
Combining both cin and cout within the same program is common practice. Here's an illustrative example:
#include <iostream>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
cin >> name; // Taking input
cout << "Hello, " << name << "!" << endl; // Outputting the input
return 0;
}
In this example, the program welcomes the user by name, demonstrating the seamless interaction made possible by iostream.
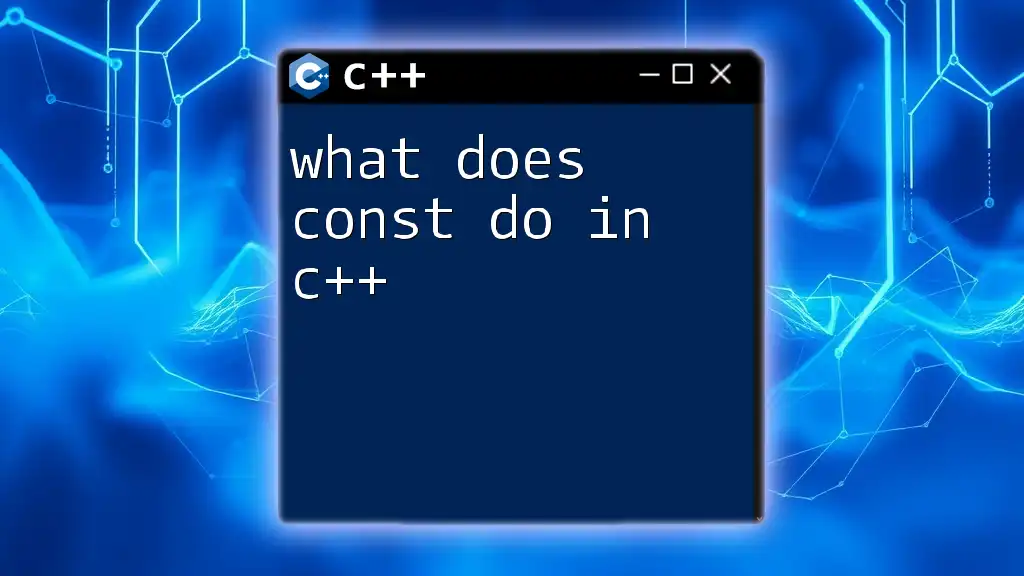
Formatting Output
Manipulators in iostream
C++ provides several output manipulators to format the output displayed with cout. Notable manipulators include:
- std::endl: This manipulator inserts a newline character and flushes the stream.
Here’s an example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Outputs message and flushes
return 0;
}
- std::setw(): This manipulator is used for setting the width of the next output field.
An example of using `setw`:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
cout << setw(10) << "Item" << setw(10) << "Price" << endl;
cout << setw(10) << "Apple" << setw(10) << 1.50 << endl;
return 0;
}
- std::setprecision(): It controls the number of decimal places for floating-point output.
Example of using this manipulator:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
double pi = 3.14159;
cout << fixed << setprecision(2) << pi << endl; // Outputs: 3.14
return 0;
}
Custom Formatting with iostream
You can create custom manipulators to format output as per your requirements. For example, consider a custom manipulator that prints asterisks around a string:
#include <iostream>
using namespace std;
ostream& asterisks(ostream& os) {
return os << "***";
}
int main() {
cout << asterisks << "Hello, World!" << asterisks << endl;
return 0;
}
In this example, the text "Hello, World!" is framed by asterisks.
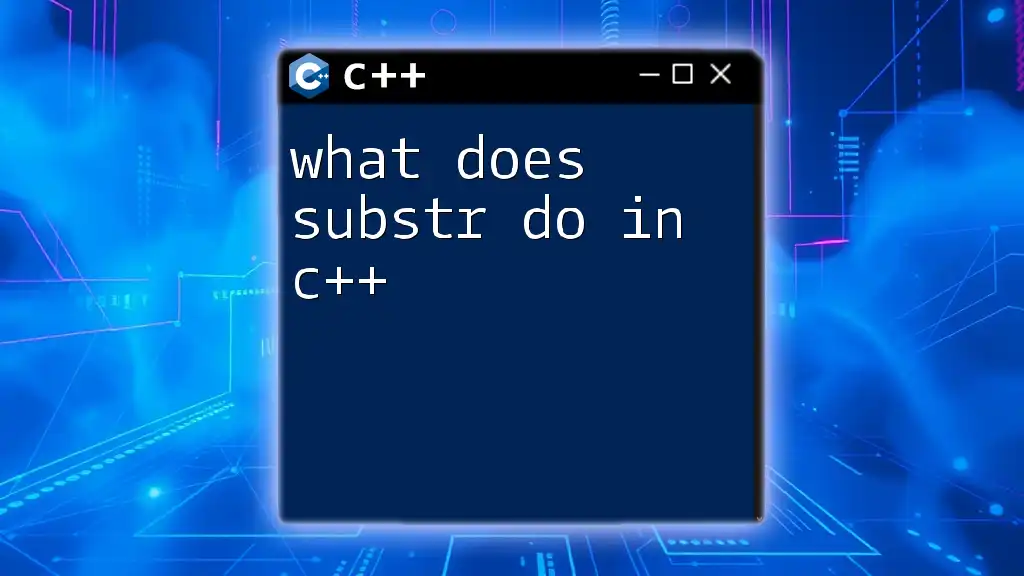
Error Handling in Input/Output
Stream States and Exceptions
Understanding stream states is vital for managing errors effectively. The key stream state functions include:
- good(): Checks if the stream is in a good state for operations.
- eof(): Checks if the end of the file has been reached.
- fail(): Checks if a logical error occurred on the stream.
Exception Handling with iostream
To create robust input/output operations, integrating error handling through exceptions is essential. A typical approach involves using try-catch blocks:
#include <iostream>
#include <stdexcept>
using namespace std;
int main() {
int number;
try {
cout << "Enter a number: ";
if (!(cin >> number)) {
throw runtime_error("Input error: Please enter a valid number.");
}
cout << "You entered: " << number << endl;
} catch (const runtime_error& e) {
cerr << e.what() << endl; // Displaying the error
}
return 0;
}
Here, if the user fails to input a valid number, an exception is thrown, and a user-friendly message is displayed.
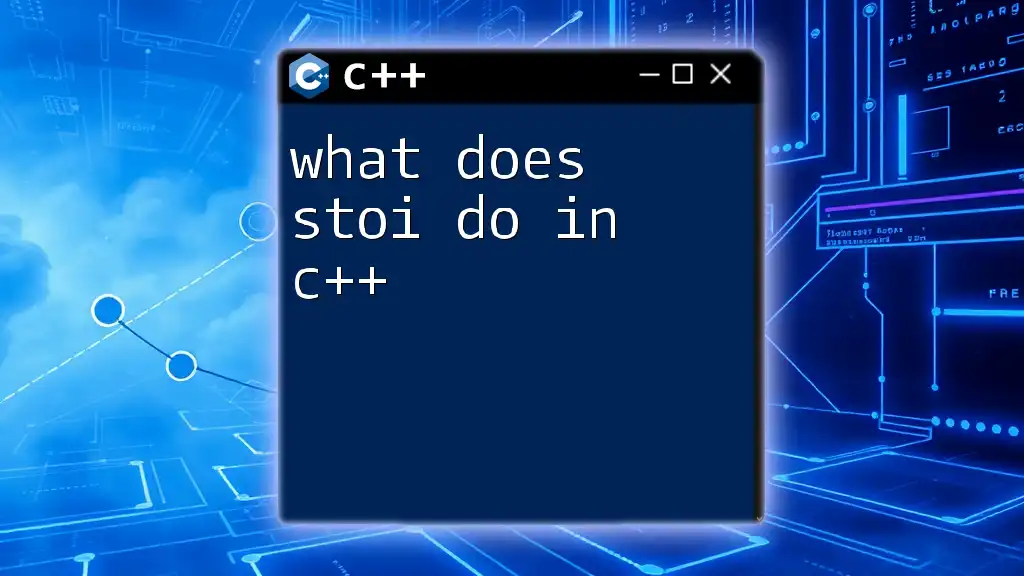
Advanced Features of iostream
Buffering and Performance
Buffering plays a key role in I/O performance. By temporarily storing data in a buffer, the program can perform more efficiently. This is because writing or reading in large chunks reduces the number of I/O operations, which are often costly in terms of time.
File Input and Output with fstream
The fstream class allows for reading from and writing to files through two primary modes: `ifstream` for reading and `ofstream` for writing.
A basic file input/output example looks like this:
#include <fstream>
#include <iostream>
using namespace std;
int main() {
// Writing to a file
ofstream outFile("example.txt");
outFile << "Hello, File!" << endl;
outFile.close(); // Always close the file after writing
// Reading from a file
string line;
ifstream inFile("example.txt");
while (getline(inFile, line)) {
cout << line << endl; // Output each line read from the file
}
inFile.close(); // Always close the file after reading
return 0;
}
In this program, we first write to a file and then read from it, demonstrating the versatility of iostream when managing files through fstream.
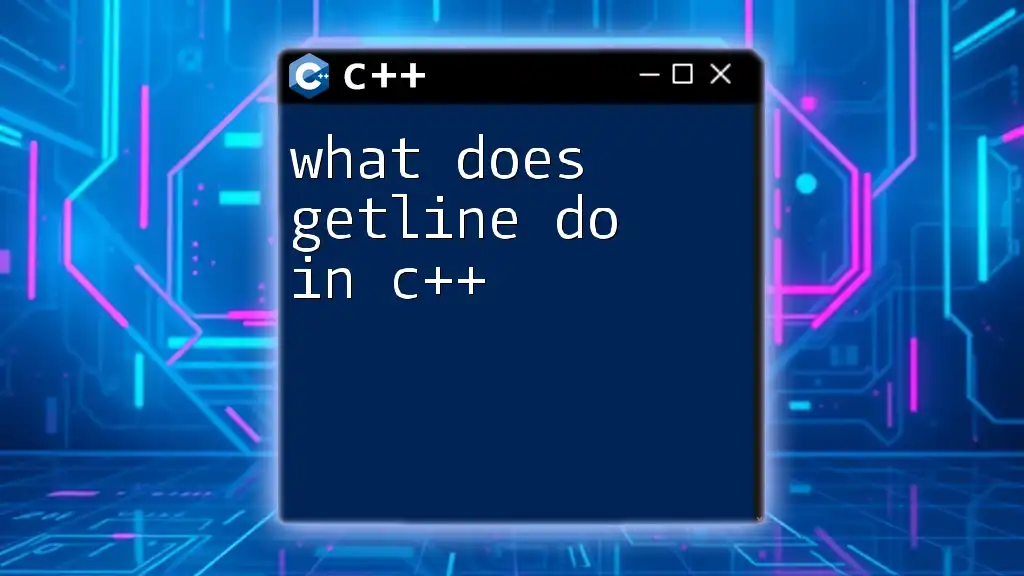
Best Practices for Using iostream
Avoid Common Pitfalls
When working with iostream, there are common mistakes to watch for:
- Forgetting to include `<iostream>` or other relevant headers can lead to compilation errors.
- Not checking for stream states after I/O operations may result in unexpected behavior.
Performance Tips
To enhance performance while using iostream:
- Avoid unnecessary calls to `std::flush` or `std::endl` too frequently, as they can degrade performance by forcing the buffer to flush.
- Use formatted I/O sparingly since it tends to be slower than unformatted output.
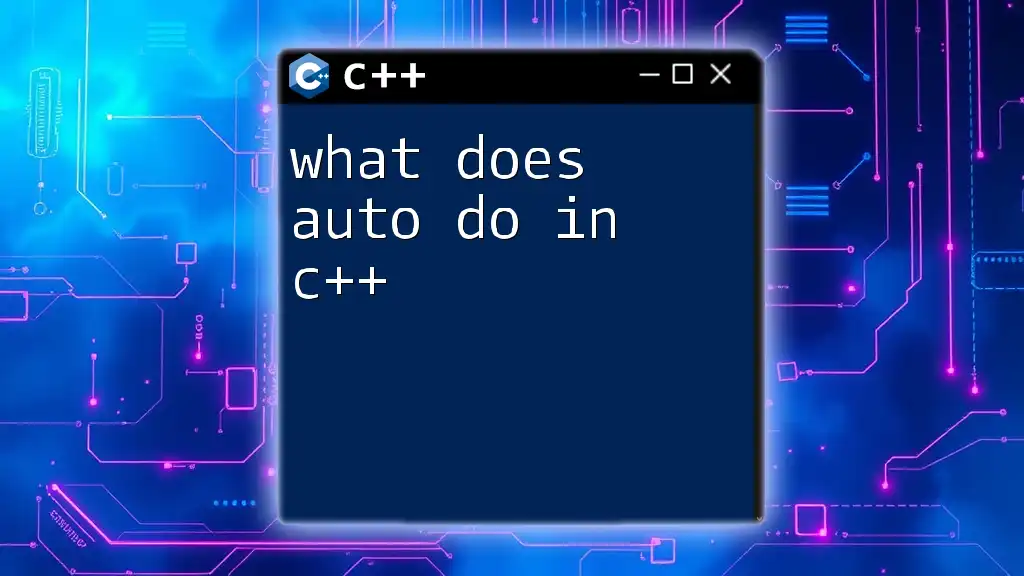
Conclusion
In conclusion, understanding what iostream does in C++ is crucial for effective programming. By mastering basic concepts like streams, input/output operations, manipulators, and error handling, you can significantly enhance your C++ development skills.
Learning how to effectively use the iostream library is vital for creating interactive and robust C++ applications. Consider diving deeper into C++ programming through our comprehensive courses to further your knowledge and skills.