In C++, `return 0;` is used in the `main` function to indicate that the program has executed successfully and is exiting without errors.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0; // Indicates successful termination
}
Understanding Return Statements in C++
Definition of a Return Statement
A return statement is a fundamental component in C++, essential for defining the exit behavior of a function. It serves to signify the termination of the function execution and can provide a value back to the function caller. In general, return statements are pivotal since they allow functions to communicate outcomes and convey whether an operation was successful or if it encountered issues.
Role of the `main()` Function
The `main()` function holds a unique position in C++. It acts as the entry point of a C++ program and is where execution begins. Whenever a C++ program is run, the operating system calls this function. The return type of `main()` is crucial as it indicates the success or failure of the program when it finishes execution. By convention, a program that reaches the end of the `main()` function without errors should return `0`.
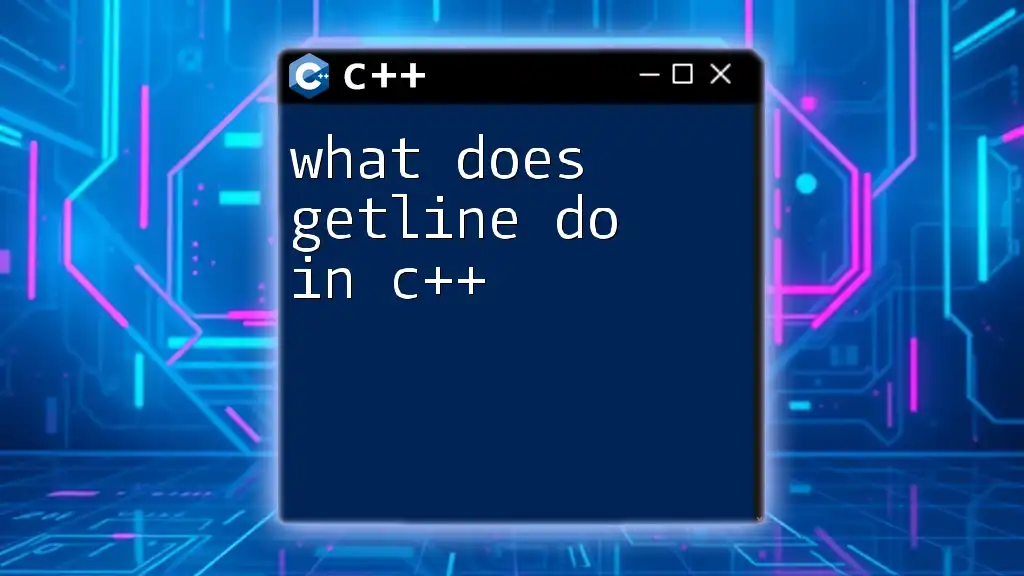
The Meaning of `return 0`
What Does Return 0 Indicate?
When you write `return 0;` at the end of the `main()` function, you are signaling to the operating system that the program executed successfully. In essence, `return 0` is synonymous with success in C++. It tells the user and the system that everything has proceeded as intended without any issues.
Comparison to Other Return Values
Other return values have specific meanings as well. For instance:
- `return 1;` often signifies a general error.
- `return -1;` can also indicate errors, but its meaning fluctuates based on the context of the application.
Using different return values allows developers to communicate specific statuses to other parts of the program or to any programs that might interact with their code.
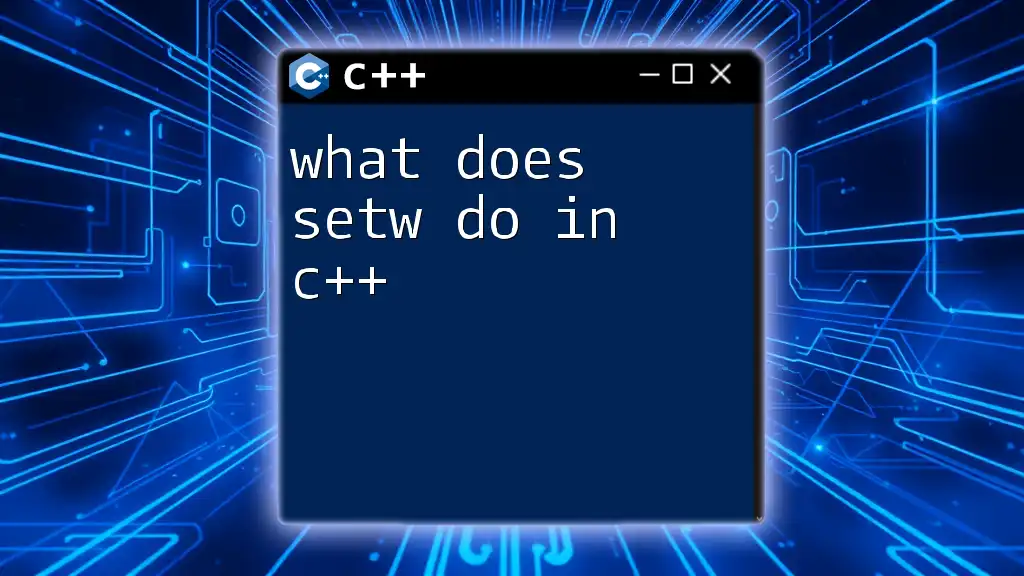
Situations Where `return 0` is Used
Basic Example of `return 0`
Consider the following simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example:
- `std::cout << "Hello, World!" << std::endl;` outputs the text "Hello, World!" to the console.
- `return 0;` communicates that the program ended successfully.
The usage of `return 0` here serves to ensure that the system inquires about the program's execution state upon its completion.
Advanced Example in Error Handling
In scenarios involving decision-making, the usage of return values becomes particularly crucial. Here’s an example of more complex logic:
#include <iostream>
int main() {
int number = 10;
if (number < 0) {
return 1; // Return 1 signifies an error
}
return 0; // Success
}
In this situation:
- The condition checks whether `number` is less than zero.
- If it is, the program returns `1`, indicating an error.
- If not, it successfully executes `return 0;`, signaling that everything is functioning correctly.
By using different return values, developers can implement effective error handling, making their programs more resilient and user-friendly.
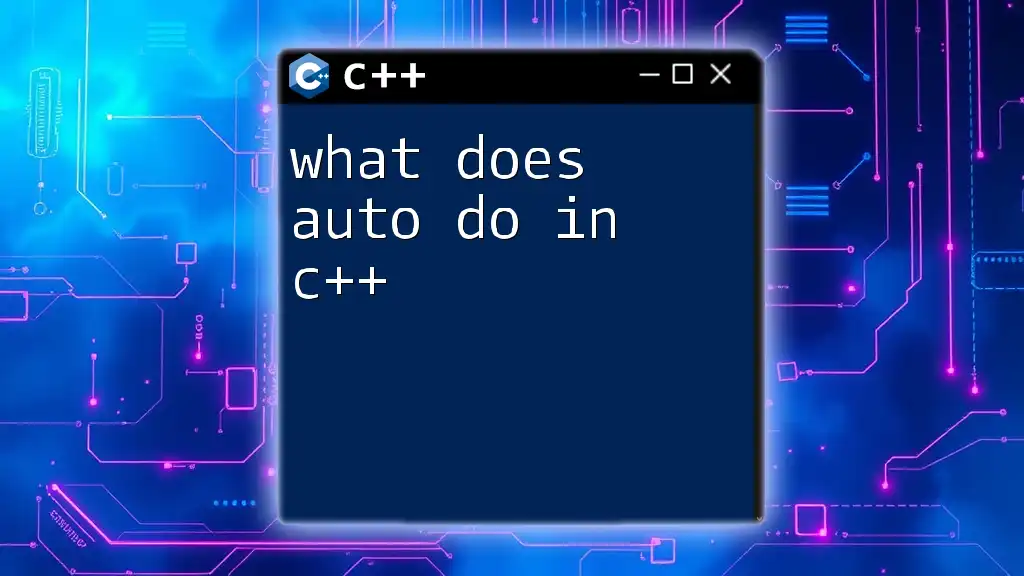
Best Practices with `return 0`
When to Use `return 0`
Using `return 0` is a practice that should be implemented at the natural conclusion of the `main()` function when your code executes successfully. This not only reflects good coding standards but also aids in debugging and clarity for anyone reviewing the code afterward.
It is generally good practice to:
- Ensure every code execution path either ends in `return 0` or some error indicating return value to avoid the program possibly returning an indeterminate value.
Considerations for Non-`int` Return Types
When it comes to functions other than `main()`, if your function is declared to return an `int`, you must uphold the same return expectations. Functions that may lead to failures or errors should reflect these in their return values, enhancing the reliability of your coding.
The convention is that:
- Non-`int` return types should communicate their results clearly through their own return mechanisms, avoiding confusion.
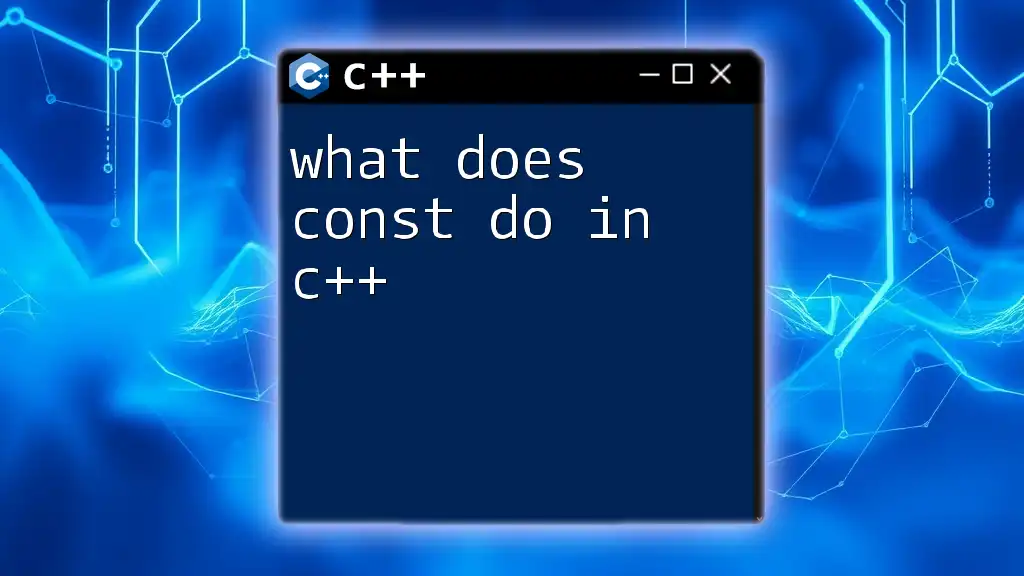
Conclusion
In summary, understanding what does return 0 do in C++ is critical for effective programming. It signifies a successful execution of the `main()` function and properly conveys program completion status to the operating system. Implementing return values correctly enhances your coding quality and enables good practices regarding error handling.
By mastering this essential aspect of C++, you'll foster a deeper understanding of program structure and flow, enabling you to build more robust applications.