The `stoi` function in C++ converts a string to an integer, enabling you to easily transform string representations of numbers into their corresponding int values.
Here's a quick example:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << num << std::endl; // Output: 123
return 0;
}
What is stoi?
In C++, `stoi` (short for "string to integer") is a standard library function that converts a string to an integer. It is defined in the `<string>` header file. The basic syntax is as follows:
int stoi(const std::string& str, size_t* idx = 0, int base = 10);
Parameters of stoi
-
String Parameter: This is the primary input for the function, which represents the numeric value in string format that you wish to convert into an integer.
-
Index Parameter: This optional parameter allows you to track the subsequent character position in the string after the numeric value. When provided, it is passed by reference, enabling the function to modify the value of this argument, even if `stoi` encounters non-numeric characters.
-
Base Parameter: This optional parameter specifies the numerical base for the conversion (e.g., decimal, hexadecimal). The default is base 10, but you can specify other bases as needed, such as binary (base 2) or hexadecimal (base 16).
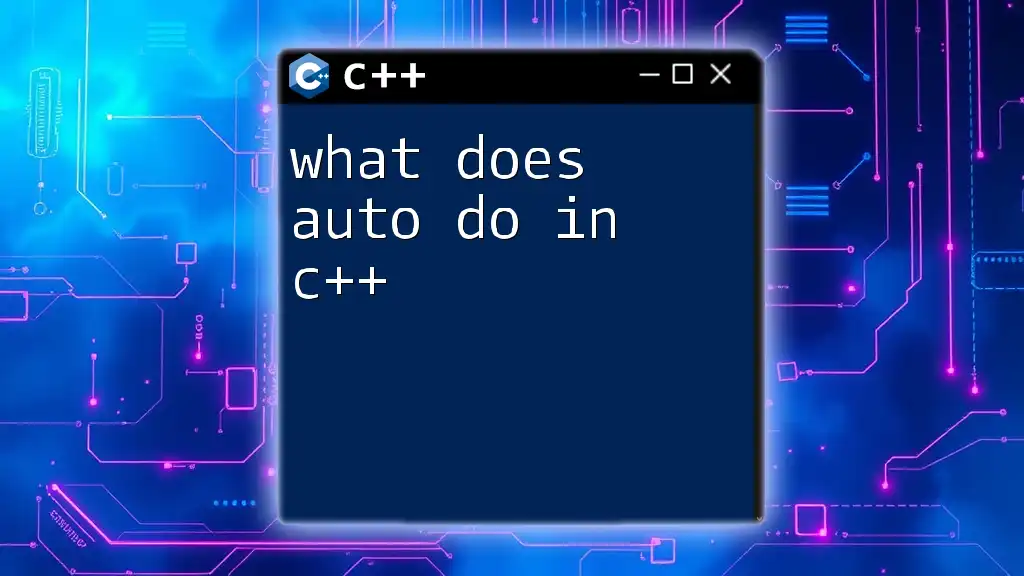
How Does stoi Work?
`stoi` internally parses the provided string, extracting the characters that represent valid decimal digits. The conversion process can be summarized as follows:
-
Leading Whitespace Handling: `stoi` ignores leading whitespace characters until it encounters a valid starting character.
-
Sign Handling: It supports both positive and negative integer representations. If a '-' sign is detected at the beginning of the number, the returned integer will be negative.
-
Non-Numeric Characters: The function will stop processing the string when it encounters a non-numeric character, unless the character was expected (like a sign).
Error Handling
Using `stoi` effectively requires understanding that it can throw exceptions under certain circumstances:
- std::invalid_argument: This exception is thrown if the input string contains no numbers or is invalid in any way.
- std::out_of_range: This occurs if the converted value exceeds the range of representable values by `int`. This is particularly important for ensuring your code handles edge cases gracefully.
Thus, it's essential to implement exception handling using `try` and `catch` blocks to ensure your program does not crash under errant conditions.
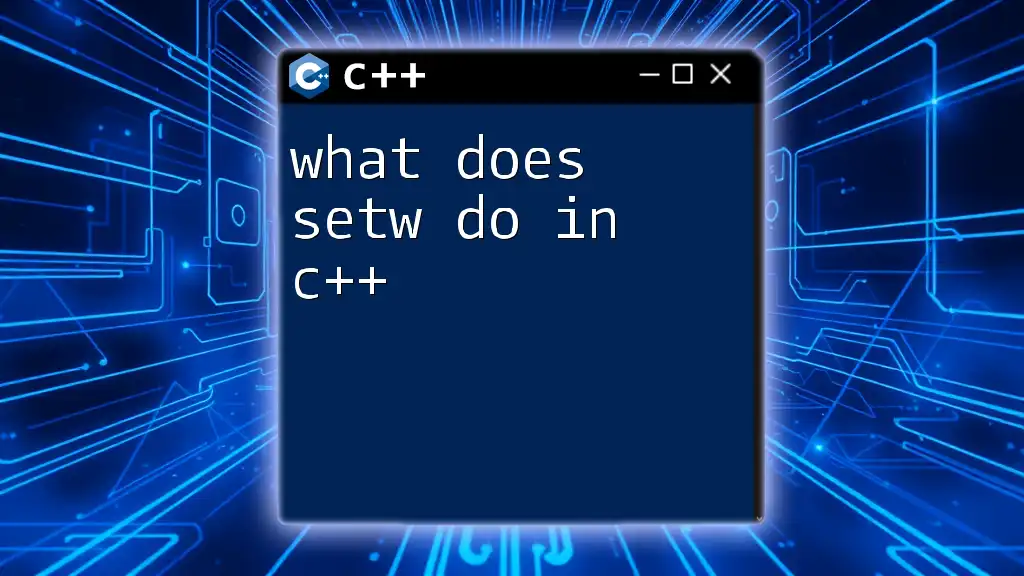
Examples of Using stoi
Basic Usage
Here is a basic example demonstrating how `stoi` converts a valid numeric string into an integer:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << "The number is: " << num << std::endl; // Output: The number is: 123
return 0;
}
In this case, `stoi` successfully converts the string "123" into the integer 123, which is then printed.
Handling Exceptions
To ensure robust programming practices, handling exceptions becomes crucial:
#include <iostream>
#include <string>
int main() {
std::string str = "abc"; // Invalid input
try {
int num = std::stoi(str);
} catch(const std::invalid_argument& e) {
std::cerr << "Invalid argument: " << e.what() << std::endl;
}
return 0;
}
In this example, attempting to convert "abc" results in an `std::invalid_argument` exception being thrown. The program captures this with a `try` block and reports the error without crashing.
Using the Index Parameter
The index parameter is particularly useful for tracking how much of the original string was converted:
#include <iostream>
#include <string>
int main() {
std::string str = "123abc";
size_t idx;
int num = std::stoi(str, &idx);
std::cout << "The number is: " << num << ", next character index: " << idx << std::endl; // Output: The number is: 123, next character index: 3
return 0;
}
Here, `stoi` converts the initial "123" and then updates the `idx` variable to point to the character immediately following the numeric portion, which is crucial for continued parsing if necessary.
Using Different Bases
The base parameter allows conversion from various numeral systems:
#include <iostream>
#include <string>
int main() {
std::string binaryStr = "1010"; // Base 2
int num = std::stoi(binaryStr, nullptr, 2);
std::cout << "Binary 1010 to decimal is: " << num << std::endl; // Output: 10
return 0;
}
In this case, the binary string "1010" is successfully converted to its decimal equivalent, 10, by specifying base 2.
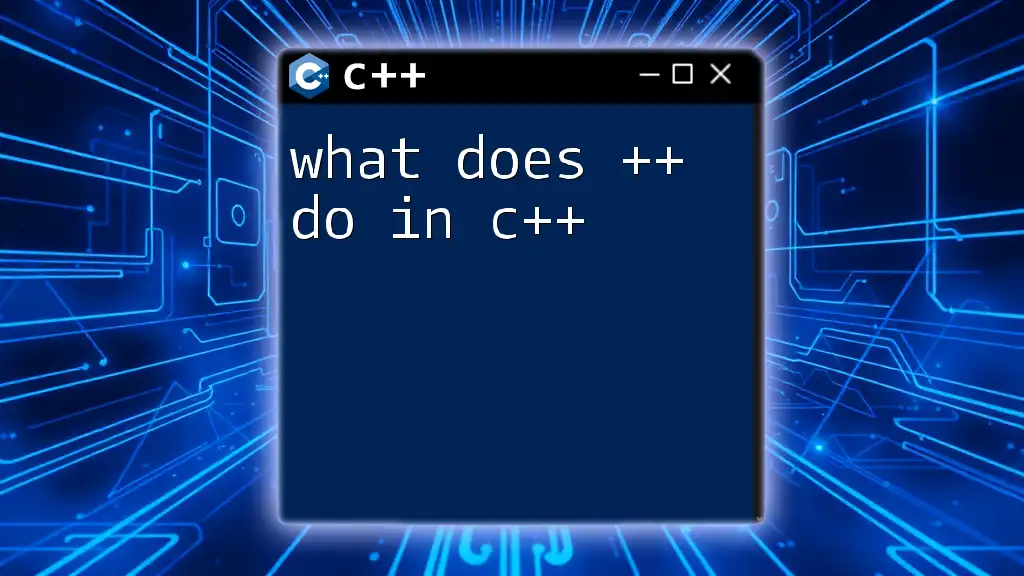
Best Practices for Using stoi
When using `stoi`, consider the following best practices:
- Validate Input: Always ensure the input string is in a valid format before conversion to reduce chances of exceptions.
- Handle Exceptions: Implement `try` and `catch` mechanisms to gracefully deal with possible exceptions.
- Prefer stoi Over Other Functions: When it comes to string to integer conversion, `stoi` is often preferred over older functions like `atoi`, as it provides exception handling that can catch errors effectively.
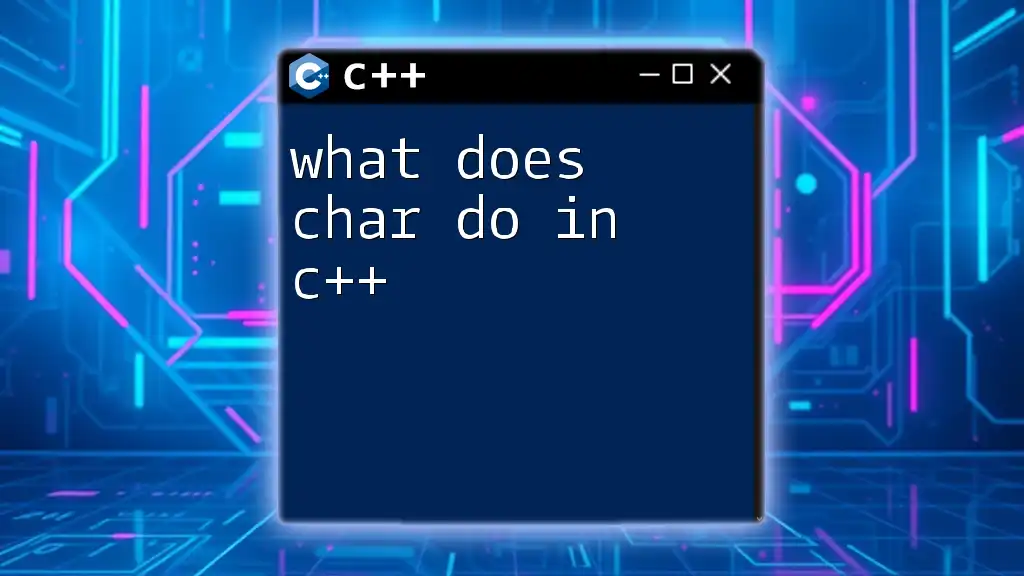
Conclusion
In summary, understanding what does stoi do in C++ is fundamental for any programmer working with string manipulations and numeric conversions. The function efficiently transforms strings into integers while offering essential exception handling and support for various numeral bases. By utilizing `stoi` appropriately and adhering to best practices, you can ensure your code is robust, reliable, and well-prepared for handling user input or data processing tasks.