In C++, the `const` keyword is used to define constants, ensuring that the variable's value cannot be modified after its initialization.
Here’s a simple example:
#include <iostream>
int main() {
const int MAX_VALUE = 100;
// MAX_VALUE = 200; // This line would cause a compilation error
std::cout << "The maximum value is: " << MAX_VALUE << std::endl;
return 0;
}
Understanding `const`
What is `const`?
In C++, the `const` keyword is used to define constants, indicating that the value of a variable cannot be modified after its initial assignment. By declaring a variable as `const`, you are enforcing immutability on that variable, which, in turn, enhances the reliability of your code.
Using `const` is a powerful way to signal to both the compiler and developers that the value should remain unchanged. This is particularly useful in large codebases, where keeping track of variable values and their intended use is crucial.
Why Use `const`?
There are several advantages to using `const` in your C++ code:
-
Immutability: By declaring a variable as `const`, you prevent accidental changes to its value, which could lead to bugs or unexpected behavior.
-
Code Readability: When other developers read your code, encountering `const` signals that they should not expect the variable to change. This makes your code easier to understand.
-
Optimization: The compiler can optimize the code better knowing that certain variables will not change, which can lead to more efficient machine code.
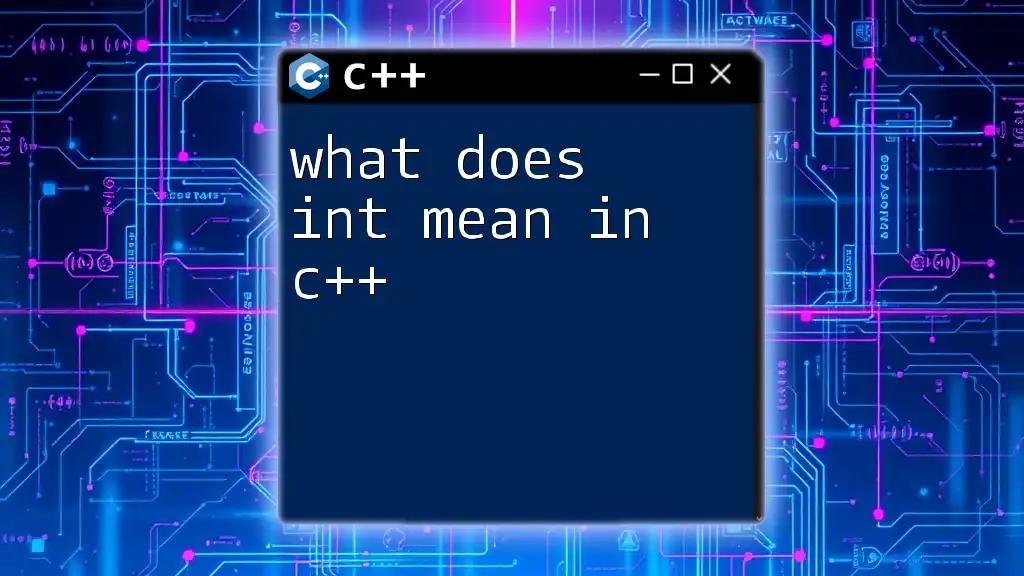
Usage of `const` in C++
Declaring Constants
Const Variables
To declare a variable as constant, you simply precede the type with the `const` keyword. For instance:
const int MAX_VALUE = 100;
In this example, `MAX_VALUE` is a constant integer. Any attempt to modify `MAX_VALUE` in subsequent lines of code, such as:
MAX_VALUE = 200; // This will cause a compilation error
will result in a compilation error, ensuring that the value remains constant throughout the program.
Const Pointers
The `const` keyword can also be applied to pointers, allowing you to declare pointers that point to constant data or make the pointer itself constant.
-
Pointer to Const: This pointer can point to a variable, but the variable it points to cannot be modified:
const int* ptrToConst = &MAX_VALUE; // Pointer to const int
-
Const Pointer: This pointer cannot point to a different variable after its initialization:
int value = 5; int* const constPtr = &value; // Const pointer to int
In this case, `constPtr` must always point to the same memory address. You can change the value at that address, but you cannot change what `constPtr` points to.
Const Reference
Why Use Const References?
Using `const` with references allows you to pass parameters to functions without copying the data, which can improve performance while still protecting the data from modification.
Here’s an example of a function that uses a const reference as an argument:
void printValue(const std::string& value) {
std::cout << value << std::endl;
}
In this example, the string passed to `printValue` cannot be modified within the function. This provides efficiency by avoiding unnecessary copying of the data.

Advanced Usage of `const`
Const Member Functions
In C++, you can also define member functions as `const`, which means these functions will not modify the state of the object. This is crucial for ensuring that functions meant to retrieve data do not inadvertently alter it.
For example:
class MyClass {
public:
void display() const {
std::cout << "Constant Method" << std::endl;
}
};
In this case, `display()` is a const member function, indicating that it can be called on const instances of `MyClass`. This adds a layer of safety and const-correctness to your class design.
Const Objects
When you declare an object as `const`, you are indicating that the object is immutable. For instance:
const MyClass obj;
obj.display(); // This is valid because display() is const
Attempting to call a non-const member function would result in a compilation error, reinforcing the integrity of the object’s data.
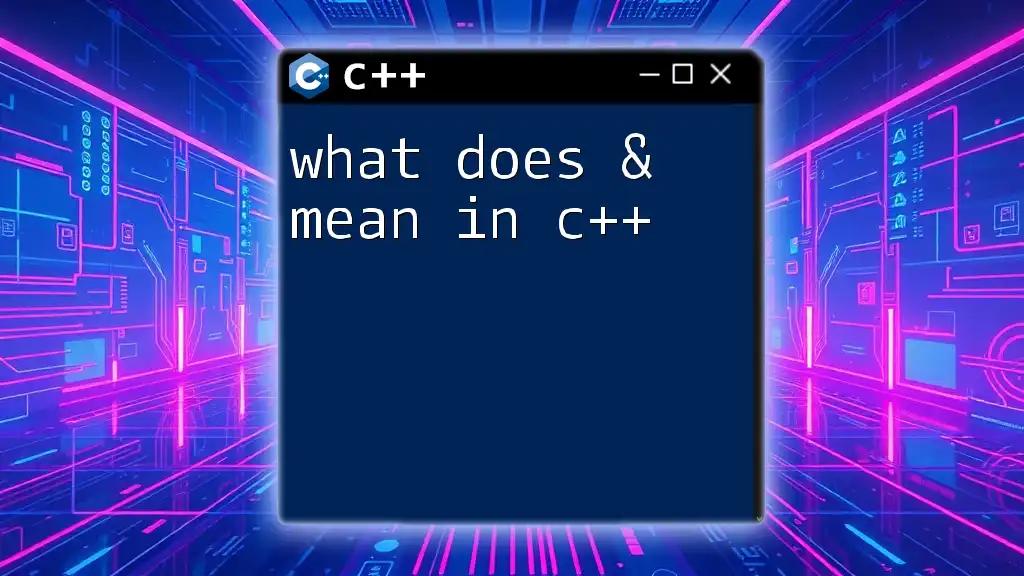
Best Practices with `const`
When to Use `const`
-
Use `const` for parameters: Whenever you pass large objects to functions, use const references to avoid the overhead of copying.
-
Const variables for fixed values: For any value that should not change, declare it as `const`. This includes configuration parameters and constants.
-
Const member functions: When designing class methods that do not modify the object state, declare them as const to prevent accidental changes and improve readability.
Common Mistakes with `const`
-
Neglecting to use `const`: Failing to declare a variable as `const` when it should be can lead to unintended modifications and hard-to-debug issues.
-
Confusing const pointers: Developers often mix up the notions of const pointers and pointers to const. Ensure clarity about what should be immutable in your design.
-
Ignoring const correctness: Forgetting to mark a member function as `const` when it does not modify the object can prevent its use on const instances, leading to compilation errors.
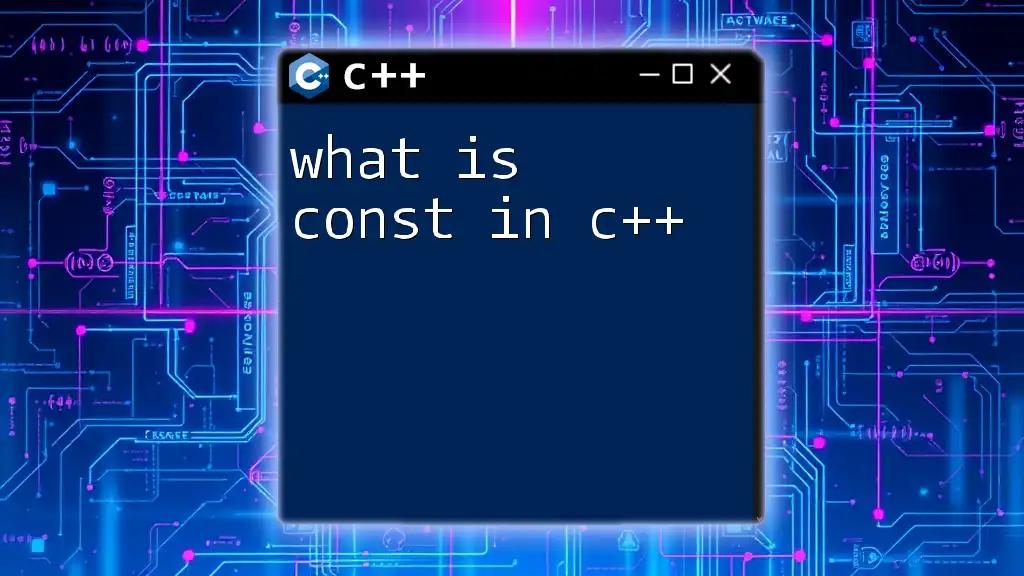
Conclusion
Understanding what `const` means in C++ is vital for writing robust, efficient, and readable code. By applying the `const` keyword appropriately, you can prevent accidental modifications, improve performance, and facilitate better interactions among developers regarding the intended usage of variables, pointers, and objects. Embrace `const` in your coding practices to enhance the integrity and clarity of your C++ programs.

Additional Resources
For further exploration of C++ concepts, consider diving into existing literature and online courses to broaden your knowledge and refine your skills in this powerful programming language!