In C++, `int` is a data type that represents integer values, allowing for the storage and manipulation of whole numbers.
int main() {
int number = 10; // Declare an integer variable named 'number' and assign it the value 10
return 0;
}
Understanding Data Types in C++
What are Data Types?
Data types are fundamental constructs in programming languages that specify the type of data a variable can hold. They define the operations that can be performed on the data and establish how much memory the data will occupy. In C++, every variable is associated with a data type, which is crucial for the compiler to understand the nature of the data being processed.
Categories of Data Types in C++
C++ supports various data types, generally classified into two categories:
-
Built-in Data Types: These are predefined by the language itself. They include:
- Integral Types: Such as `int`, `char`, `bool`.
- Floating-point Types: Such as `float`, `double`.
- Void Type: Denoted by `void`, which signifies no data.
-
User-defined Data Types: These are created by the programmer to enhance functionality and improve coding efficiency. Examples include `struct`, `union`, `enum`, and `class`.
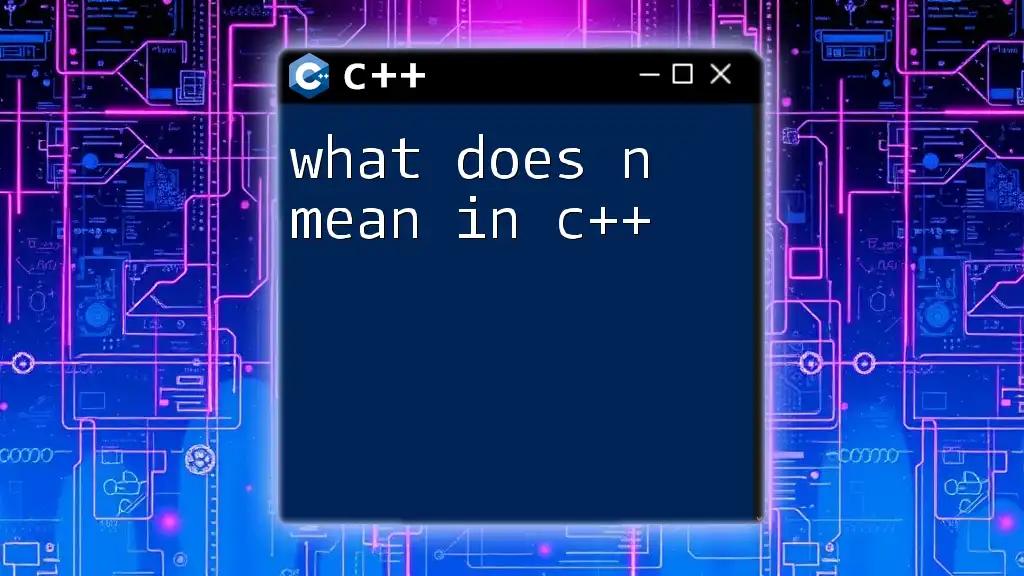
The `int` Data Type
Definition of `int`
In C++, the `int` keyword represents an integer, which is a data type used to store whole numbers (both positive and negative) without any decimal point. This makes `int` one of the most commonly used data types in programming, especially for counting, indexing, and calculations involving discrete values.
Characteristics of `int`
One of the significant characteristics of `int` is its size and range. Typically, an `int` occupies 4 bytes in memory, though this can vary based on the system and compiler being used. The valid range of values for a regular `int` is commonly:
- From -2,147,483,648 to 2,147,483,647 (which is the range for a 32-bit signed integer).
Here’s how that translates in your code:
int a = 2147483647; // Maximum value for a signed int
int b = -2147483648; // Minimum value for a signed int
Variants of `int`
signed int
By default, `int` is a signed integer, meaning it can represent both positive and negative numbers. When you simply declare an `int`, it is treated as signed:
int num = -10; // This is valid
unsigned int
In contrast, an unsigned int only accommodates positive numbers and 0. This variant effectively doubles the upper limit of the positive range, making it valuable when you know your number will never be negative. An example would be:
unsigned int positiveNum = 3000000000; // This is valid
When to Use `int`
Choosing when to use `int` involves considering several factors:
- Whole Numbers: Use `int` to store values that are whole numbers and require no fractional component. Examples include counts, indices, and identifiers.
- Performance: `int` is generally fast and efficient in operations compared to floating-point types, making it ideal for performance-critical applications.
- Memory Consideration: With its predictable size, `int` helps manage memory effectively, especially in large applications.
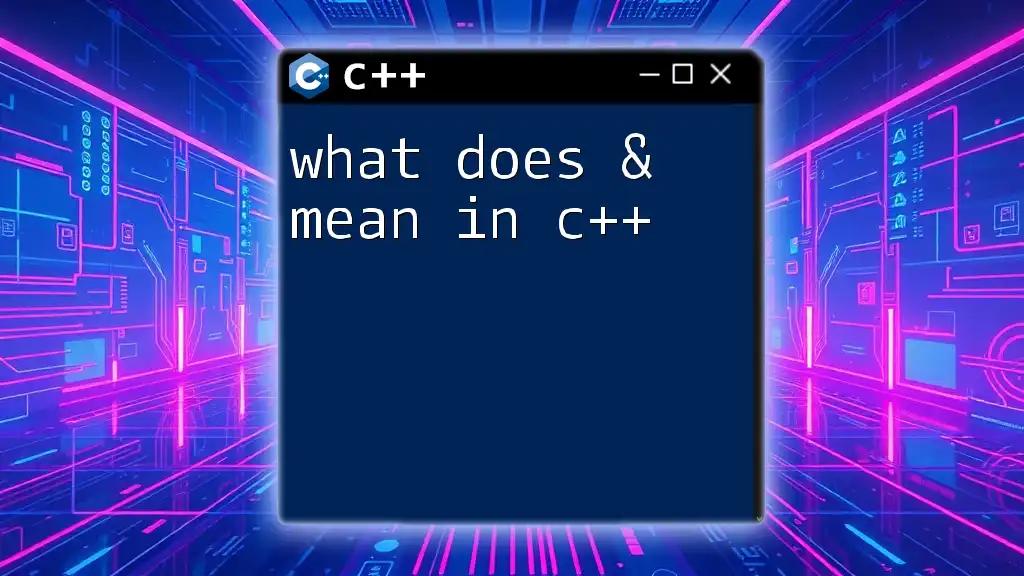
Declaring and Initializing `int`
Syntax for Declaration
Declaring an `int` variable is straightforward:
int age;
This code declares a variable named `age`, though it remains uninitialized until you assign a value.
Initializing an `int`
Initialization can be done in several ways:
-
Direct Initialization:
int age = 30;
-
Copy Initialization:
int age(30);
-
Uniform Initialization (introduced in C++11):
int age{30};
These methods allow for flexibility in how you set initial values for your integers.
Modifying an `int`
Once declared, you can easily modify the value of an `int` variable. For example:
int age = 30;
age = age + 5; // Now age is 35
This example demonstrates how programming logic can update values based on computations or conditions.
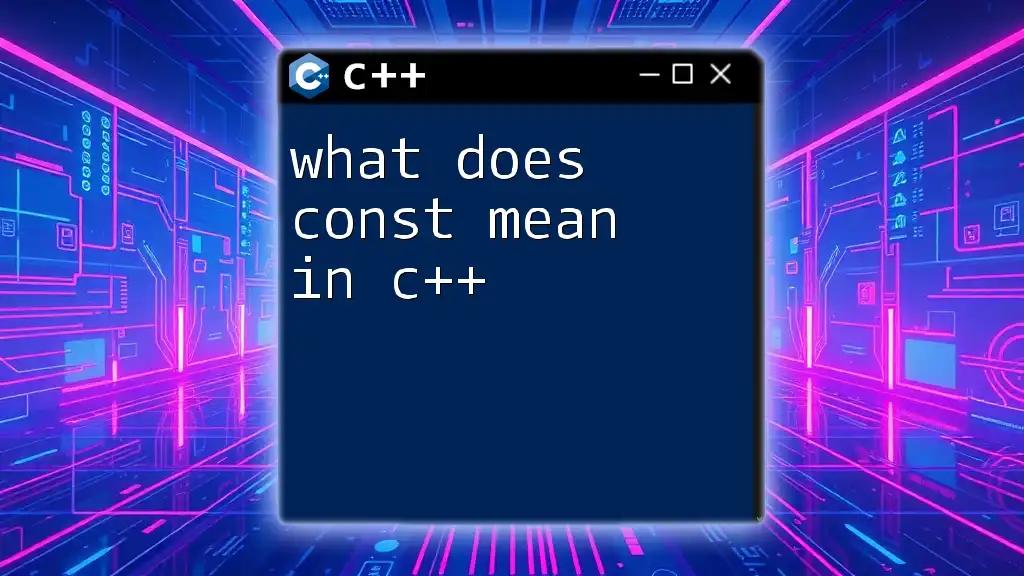
Operations with `int`
Arithmetic Operations
You can perform a variety of arithmetic operations with `int` variables. The fundamental operations include:
- Addition
- Subtraction
- Multiplication
- Division
Here’s a practical example:
int a = 10;
int b = 5;
int sum = a + b; // sum is 15
Increment and Decrement Operators
C++ also supports increment (`++`) and decrement (`--`) operators, which provide a concise way to increase or decrease the value of an integer. Both prefix and postfix forms are available:
int count = 5;
count++; // Now count is 6 (postfix increment)
++count; // Now count is 7 (prefix increment)
Comparison Operators
Using comparison operators with `int` values allows you to implement decision-making logic in your code. The relational operators include `==`, `!=`, `<`, `>`, `<=`, and `>=`. A simple condition can look like this:
if(a > b) {
// This block executes if a is greater than b
}

Common Pitfalls with `int`
Overflow and Underflow
One critical issue with using `int` is the possibility of overflow and underflow. Overflow occurs when you exceed the maximum representable value, while underflow happens when dipping below the minimum. For example:
int largeNumber = 2147483647;
largeNumber++; // This leads to overflow
Proper handling of these situations is essential for avoiding bugs or unexpected behavior.
Best Practices to Avoid Issues
To manage risks associated with overflow and underflow, consider the following tips:
- Validate input: Always check user inputs before assigning them to an `int`.
- Use larger types: If you anticipate larger values, consider using `long` or `long long`.
- Monitor computations: Be cautious with operations that deal with very high or low values.

Conclusion
In conclusion, understanding what does `int` mean in C++ is vital for anyone looking to code effectively with this powerful language. As a fundamental data type, `int` serves as a cornerstone for storing and manipulating whole numbers in various applications. By appreciating its characteristics, variants, and operations, you can harness the full potential of integers to improve your programming endeavors.

Additional Resources
For further learning on C++, several resources can enhance your understanding and coding skills:
- Books like "C++ Primer" and "Effective C++"
- Online coding platforms and tutorials
- Programming communities for discussions and guidance on `int` and data types in general.