The `++` operator in C++ is an increment operator that increases the value of a variable by 1.
int x = 5;
x++; // Now x is 6
Understanding the Increment Operator (++)
The increment operator in C++, represented by `++`, is a crucial arithmetic operator that increases the value of a variable by one. It ensures efficient and precise adjustments in various programming scenarios, making it pivotal in C++ coding practices.
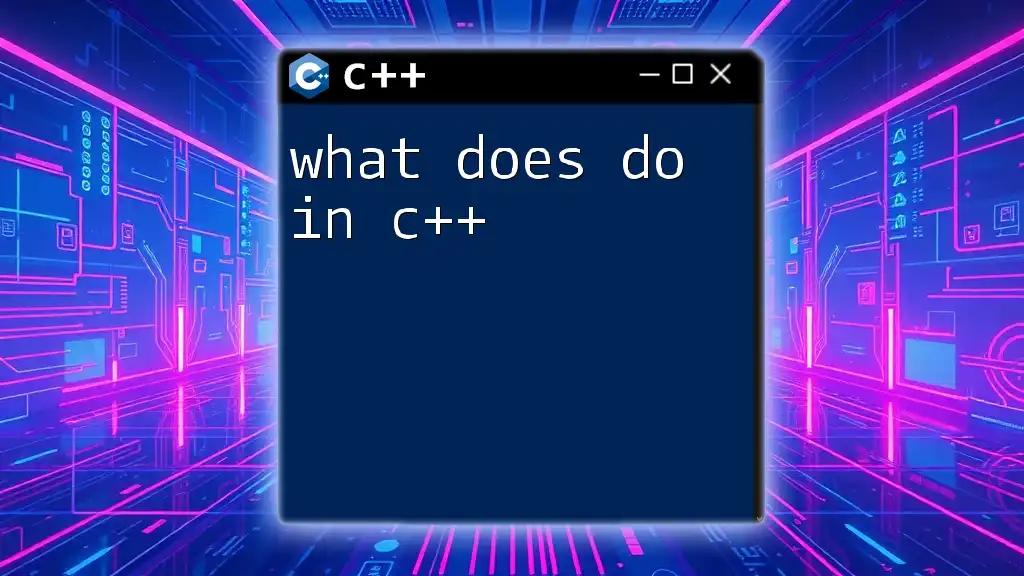
Types of Increment Operations
Prefix Increment Operator
The prefix increment operator is denoted as `++variable`. By using this operator, the variable's value is increased by one before the variable is used in any expression.
For example, consider the following snippet:
int x = 5;
int result = ++x; // x now is 6, result is 6
In this case, `x` is incremented before the assignment to `result`. As a result, both `x` and `result` hold the value `6`. This operator is particularly useful when you want to immediately utilize the incremented value in subsequent computations.
Postfix Increment Operator
Conversely, the postfix increment operator is represented as `variable++`. Here, the variable's value is increased by one after it has been used in an expression.
Consider this example:
int y = 5;
int result = y++; // y becomes 6, but result is 5
In this scenario, `y` is first assigned to `result` before being incremented, yielding `5` for `result` while `y` updates to `6`. This operator is essential when you need the original value before the increment for calculations or conditions.
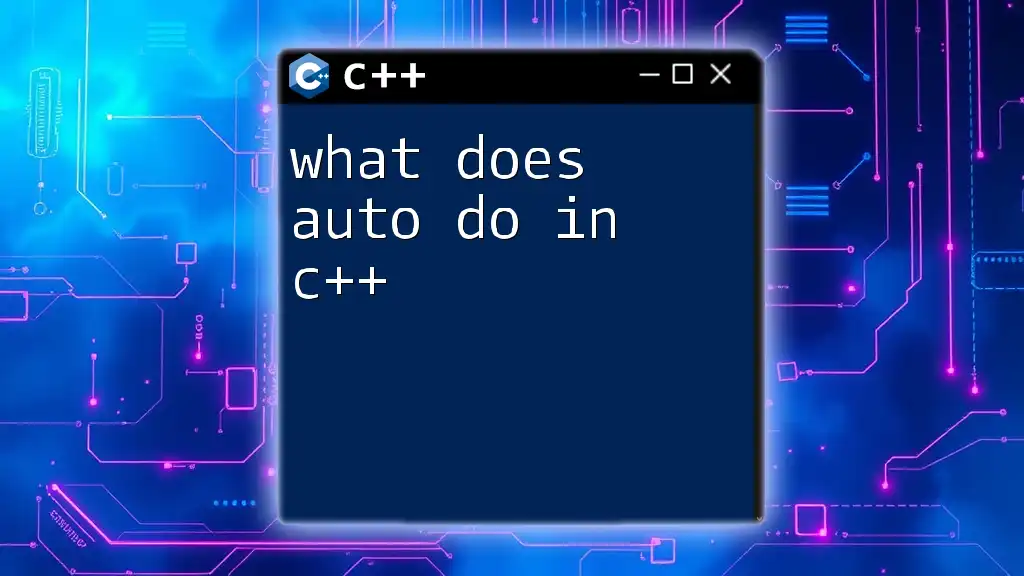
Practical Applications of the Increment Operator
Usage in Loops
The increment operator proves incredibly handy within loops, such as `for` loops, where it controls iteration.
For example:
for (int i = 0; i < 10; ++i) {
// Loop body
}
In this case, the loop begins with `i` at `0`, and on each iteration, the prefix increment operator increases `i` by one. While both `++i` and `i++` can be used here, the prefix increment can sometimes offer slight performance advantages in certain contexts, although for primitive types, the difference is negligible. It is always good to understand the context in which you are using these operators.
Applications in Collections
Understanding the increment operator is invaluable while working with iterators in collections, such as those found within the Standard Template Library (STL).
For instance:
std::vector<int> nums = {1, 2, 3, 4, 5};
auto it = nums.begin();
++it; // Move to the second element
Here, `++it` advances the iterator to point to the second element of the vector. Utilization of the increment operator not only allows for clearer iteration but also encapsulates more efficient control over dynamic collections.
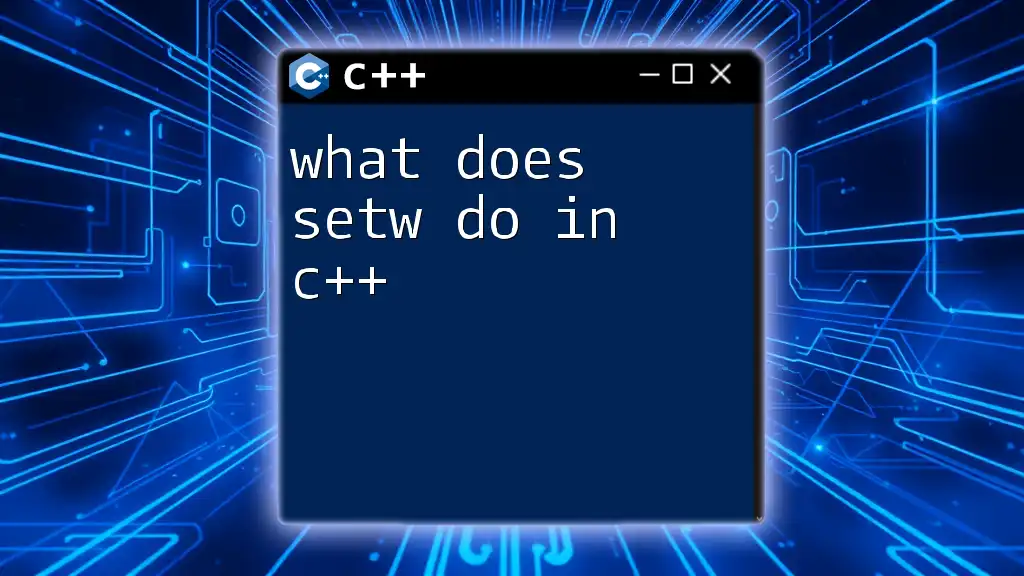
Increment vs. Decrement: Understanding the Differences
What is the Decrement Operator (--)?
In contrast to the increment operator, the decrement operator, represented by `--`, reduces the value of a variable by one. This operator can also be used as either a prefix or postfix, working similarly to how `++` functions.
Practical Examples of Decrement in Loops
Decrement operations can be seen in reverse loops as well. For example:
for (int i = 10; i > 0; --i) {
// Loop body
}
In this `for` loop, `--i` decrements the value of `i` before each iteration checks. Depending on your algorithm's requirements—whether you want to count down or count up—utilizing `++` or `--` appropriately is critical.
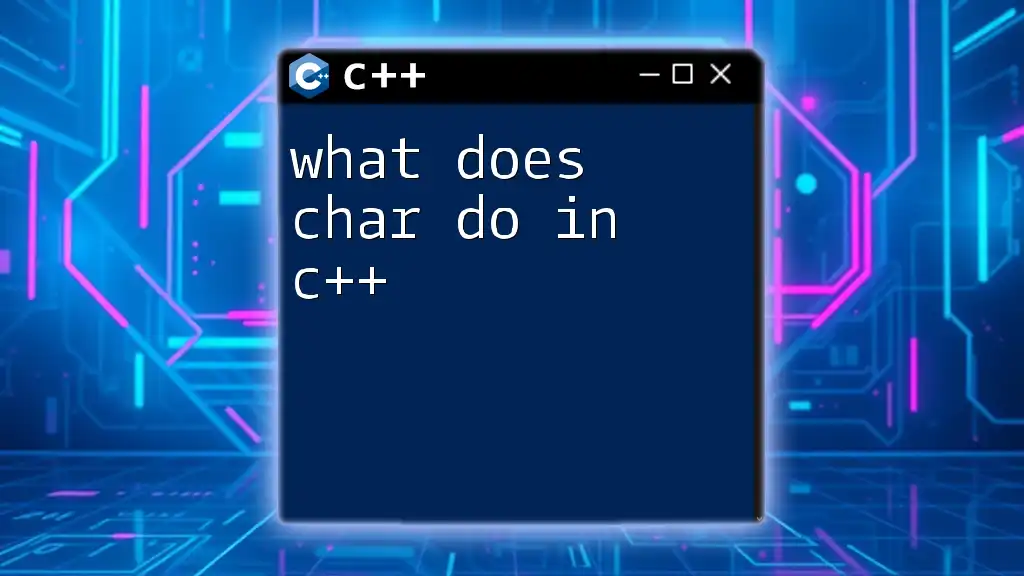
Common Mistakes and Misunderstandings
One common error involves misunderstanding the implications of prefix versus postfix notation, especially in complex expressions. For instance, consider the code:
int a = 3;
int b = a++; // Misunderstanding the outcome
In this situation, the programmer might mistakenly expect `b` to hold `4`. Instead, `b` is `3` because `a` was only incremented after the value was assigned. Recognizing whether your operator results in an lvalue or rvalue is essential to ensure accurate results and avoid bugs.
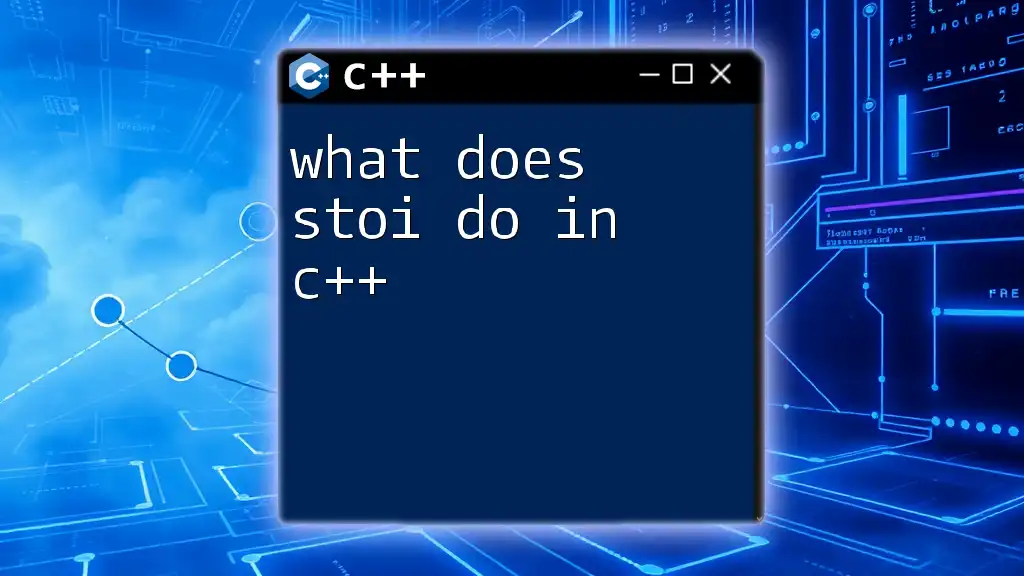
Conclusion
In summary, understanding what does `++` do in C++ is fundamental for any programmer venturing into C++. The increment operator, both in its prefix and postfix forms, plays a crucial role in arithmetic operations, especially in loops and STL manipulation. Mastering this operator can significantly enhance both the efficiency and readability of your code.
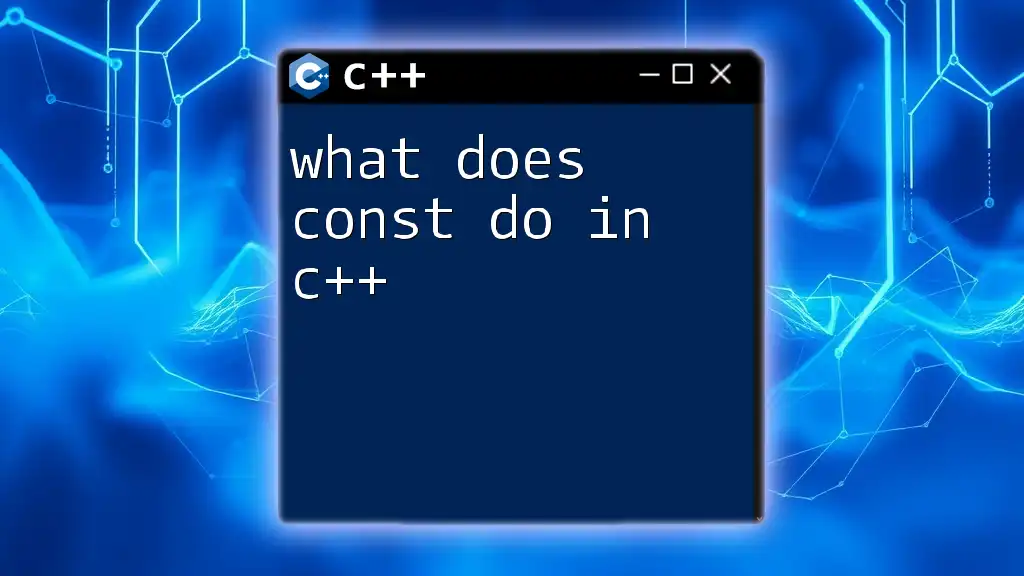
Call to Action
Explore more about C++ commands, and share your experiences or questions regarding increment and decrement operations. Embrace the opportunity to sharpen your skills and contribute to the thriving community of C++ developers!
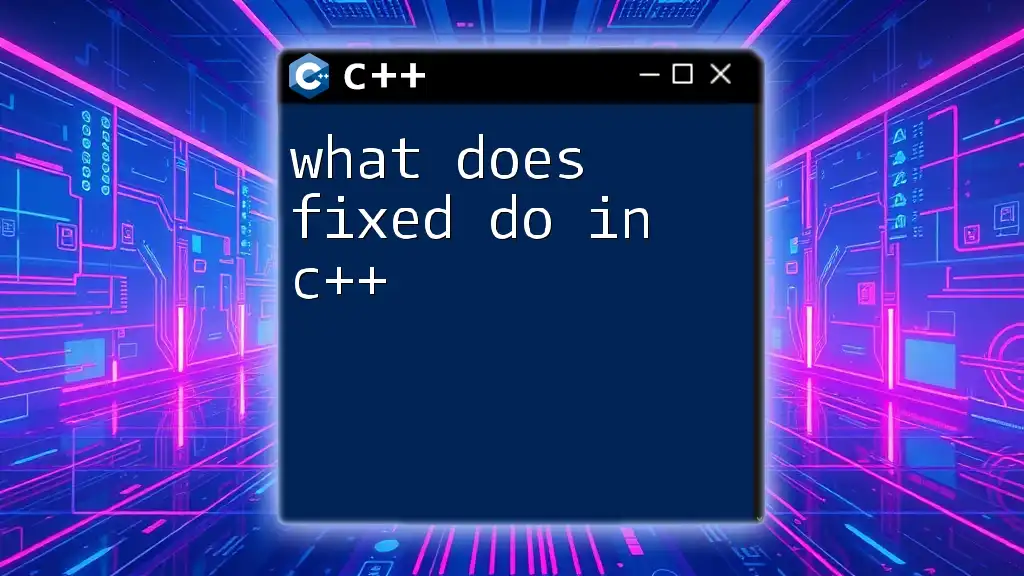
Additional Resources
To expand your knowledge further, consider reading additional tutorials about operators and basic programming constructs in C++. Keep practicing to strengthen your grasp of these essential concepts!