In C++, `std::fixed` is a manipulator that forces the output of floating-point numbers to be displayed in fixed-point notation, preventing scientific notation from being used.
Here's a code snippet demonstrating the use of `std::fixed`:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 123.46
return 0;
}
Understanding Floating-Point Representation
Floating-point numbers are essential in C++ and are used to represent real numbers that require fractional parts. The IEEE 754 standard defines how these numbers are stored and displayed, which can lead to precision issues if not managed correctly. This is where understanding the significance of formats like `fixed` becomes crucial.
When outputting floating-point numbers, precision matters. Fixed-point notation, provided by the `fixed` manipulator in C++, plays a significant role in ensuring that the displayed numbers retain the desired number of decimal places.
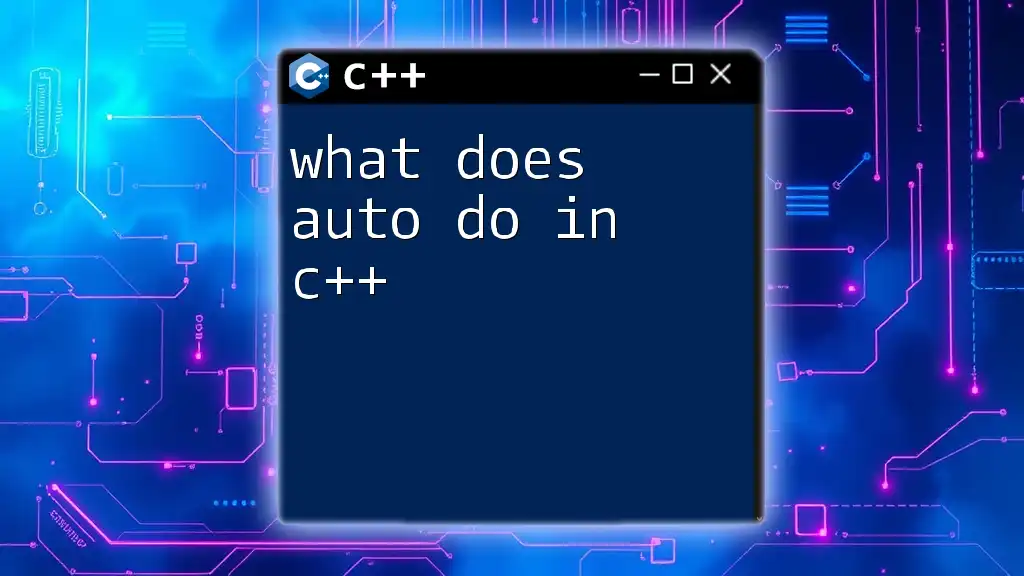
The Role of `fixed`
The `fixed` manipulator is designed to control the output format of floating-point numbers in C++. Its primary purpose is to enable fixed-point notation, which means that the values will be displayed with a consistent number of decimal places regardless of the numerical value. This is vital for clarity, particularly in applications requiring precise numerical presentations.
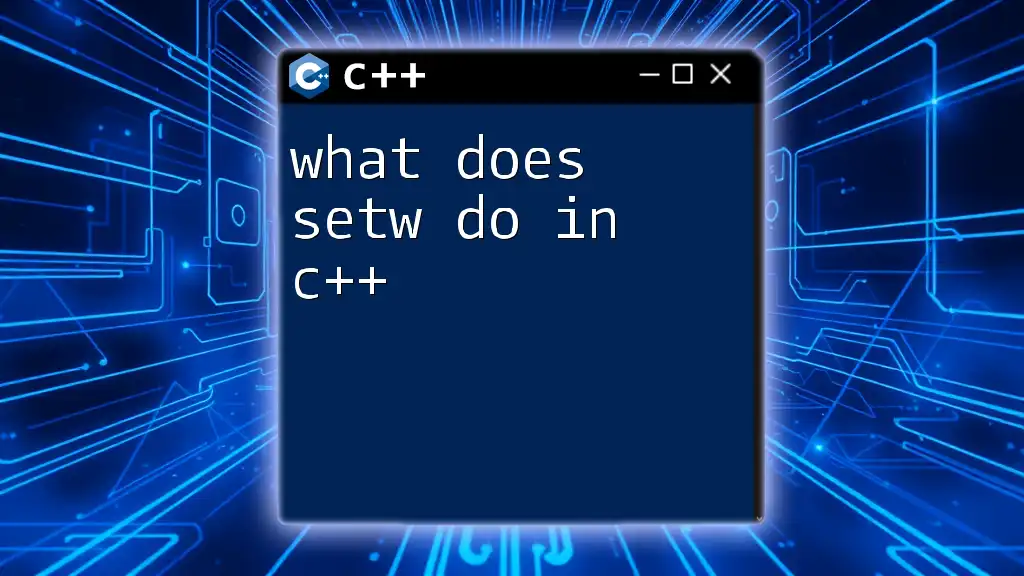
How to Use `fixed` in C++
Enabling Fixed-Point Notation
You can enable fixed-point notation by using the `fixed` manipulator from the `<iomanip>` library. This manipulator affects the output of floating-point numbers until it is explicitly changed back to a default or scientific representation. Here’s a simple example to demonstrate how to use `fixed`:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << num << std::endl;
return 0;
}
In this example, the number `123.456789` will be printed as-is with no adjustment to its appearance.
Combining Fixed with Precision
To take full advantage of the `fixed` manipulator, you can combine it with the `setprecision` manipulator, also available in `<iomanip>`. This combo allows you to specify how many decimal places will be displayed.
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Outputs: 123.46
return 0;
}
In this case, setting `setprecision(2)` ensures that the output is limited to two decimal places, producing the output `123.46`. This is particularly useful in applications where numerical precision is essential, such as financial calculations.
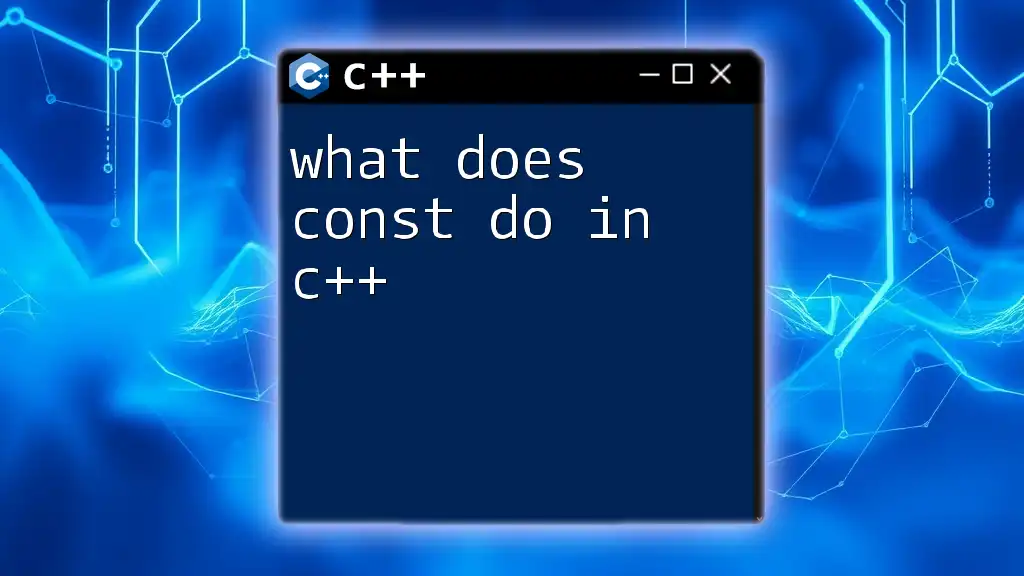
Practical Applications of `fixed`
Displaying Financial Data
In financial applications, accurate representation of monetary values is critical. The use of `fixed` ensures that amounts are consistently formatted, minimizing confusion for readers. For instance:
#include <iostream>
#include <iomanip>
int main() {
double price = 29.99;
std::cout << "Price: $" << std::fixed << std::setprecision(2) << price << std::endl;
return 0;
}
Here, the output will be `Price: $29.99`, making it easy for users to read and interpret the financial data clearly. This level of precision allows for accurate billing, account summaries, and financial analysis.
Scientific Calculations
In scientific contexts, fixed-point notation can provide clear data representation. When conducting scientific measurements, precision is often key in ensuring data integrity. Consider this example:
#include <iostream>
#include <iomanip>
int main() {
double measurement = 0.003456789;
std::cout << "Measurement: " << std::fixed << std::setprecision(4) << measurement << " m" << std::endl;
return 0;
}
With this code, the output will display the measurement as `Measurement: 0.0035 m`. The `fixed` manipulator allows for a clear and concise presentation of data where precision is crucial.
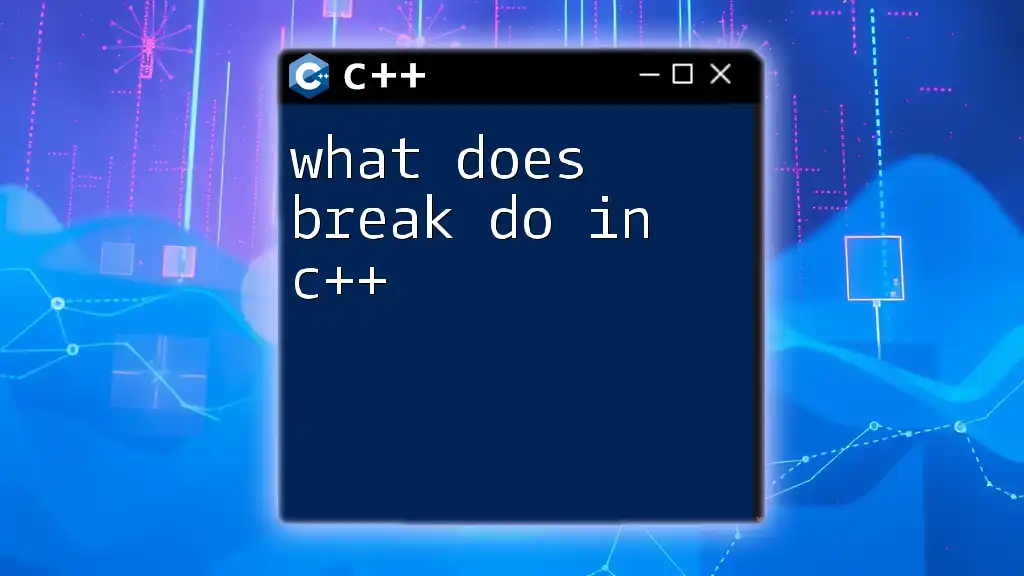
Comparisons: `fixed` vs. `scientific`
When to Use `fixed` Over `scientific`
Understanding when to use `fixed` in contrast to `scientific` is essential. While `scientific` notation presents numbers in exponential form, `fixed` provides a straightforward representation. This distinction is critical in contexts where users expect a specific format or clarity.
Consider the following code example that contrasts both formats:
#include <iostream>
#include <iomanip>
int main() {
double num = 0.00012345;
std::cout << "Fixed: " << std::fixed << num << std::endl;
std::cout << "Scientific: " << std::scientific << num << std::endl;
return 0;
}
The outputs here will show different formats: `Fixed: 0.000124` and `Scientific: 1.234500e-04`. The differentiation between the two ensures that data can be presented in a way that best suits the audience's needs.
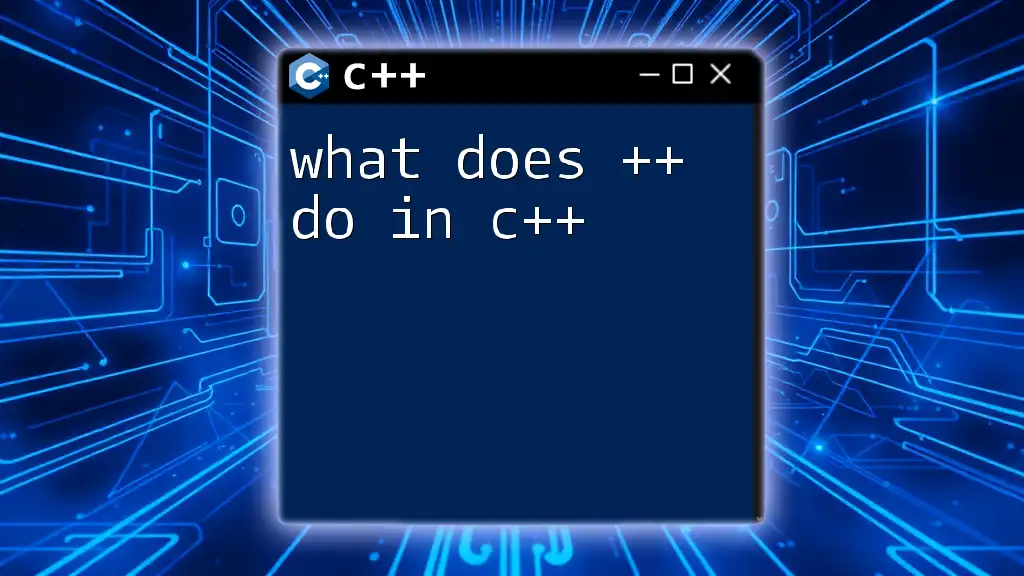
Common Pitfalls When Using `fixed`
Not Resetting Manipulators
One common mistake when using `fixed` is failing to reset the manipulator. If you do not reset it after use, subsequent output may continue in the same format unintentionally. Here's an example illustrating the consequences:
#include <iostream>
#include <iomanip>
int main() {
double num1 = 123.456789;
double num2 = 0.000123;
std::cout << std::fixed << num1 << std::endl;
std::cout << num2 << std::endl; // Will still be in fixed format
return 0;
}
In this case, `num2` will also be printed in the fixed format, which may lead to confusion or misinterpretation of the value. Always remember to reset manipulators as needed.
Confusing Results with Other Manipulators
Using `fixed` alongside other formatting manipulators can sometimes yield unexpected results. Here’s an example:
#include <iostream>
#include <iomanip>
int main() {
double num = 123456.789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl;
std::cout << std::scientific << num << std::endl;
return 0;
}
You might expect consistent output with the scientific notation, but the cumulative effect of manipulators can cause outputs to escalate in complexity if not managed well.
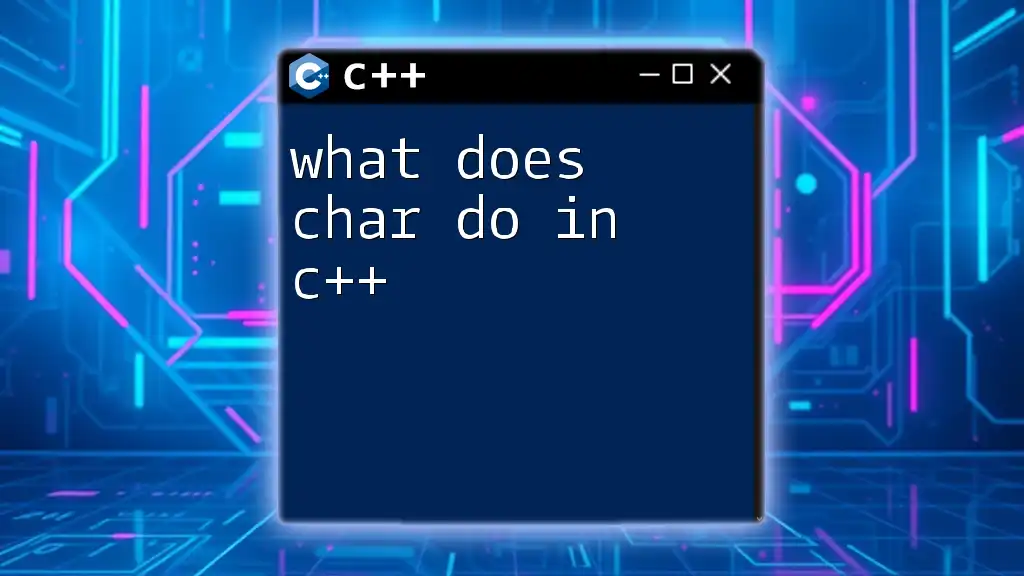
Conclusion: Embracing `fixed` in C++
Using the `fixed` manipulator in C++ can significantly improve the clarity and precision of numeric output. Whether displaying financial data or scientific measurements, it provides a straightforward method to convey information accurately.
Incorporating fixed-point notation ensures consistency in the appearance of your floating-point numbers, making your applications more user-friendly. As you experiment with `fixed`, consider how it can enhance the readability of your programs and presentations.
Remember that practice is essential in mastering any language feature. I encourage you to experiment with the `fixed` manipulator and explore its impact further to deepen your understanding of number formatting in C++.
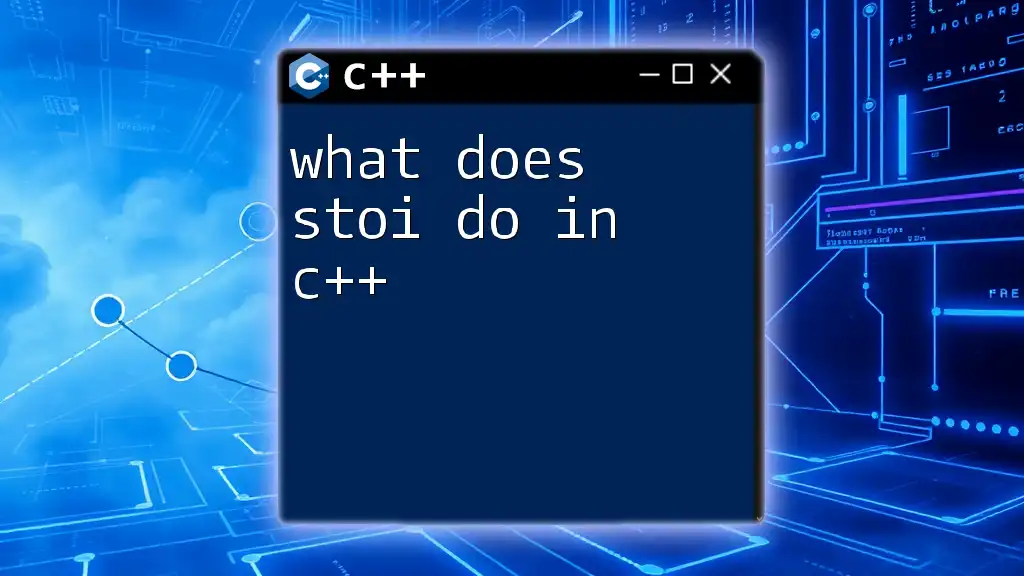
Recommended Resources
For those looking to expand their knowledge beyond this article, consider diving into literature focusing on C++ I/O, such as advanced programming textbooks or online tutorials dedicated to C++ formatting. Engaging with communities and discussion forums can also be an excellent avenue for learning, helping you connect with others who share your journey in mastering C++.