The `getline` function in C++ reads an entire line from an input stream, including spaces, until a newline character is encountered, effectively allowing for input of strings with spaces.
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a line of text: ";
getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding the Basics of getline
What is getline?
The `getline` function in C++ is designed for reading a line of text from an input stream, such as the standard input (`std::cin`) or a file. Unlike simple input functions that stop reading at whitespace, `getline` continues reading until it encounters a newline character (`\n`). This makes it particularly useful for capturing whole lines of text, including spaces.
Syntax of getline
The general syntax of the `getline` function is straightforward:
std::getline(istream, string, delimiter);
- istream: The input stream you are reading from (e.g., `std::cin` or a file stream).
- string: The string variable that will store the line of text read by `getline`.
- delimiter (optional): A character that specifies where to stop reading. By default, this is the newline character.
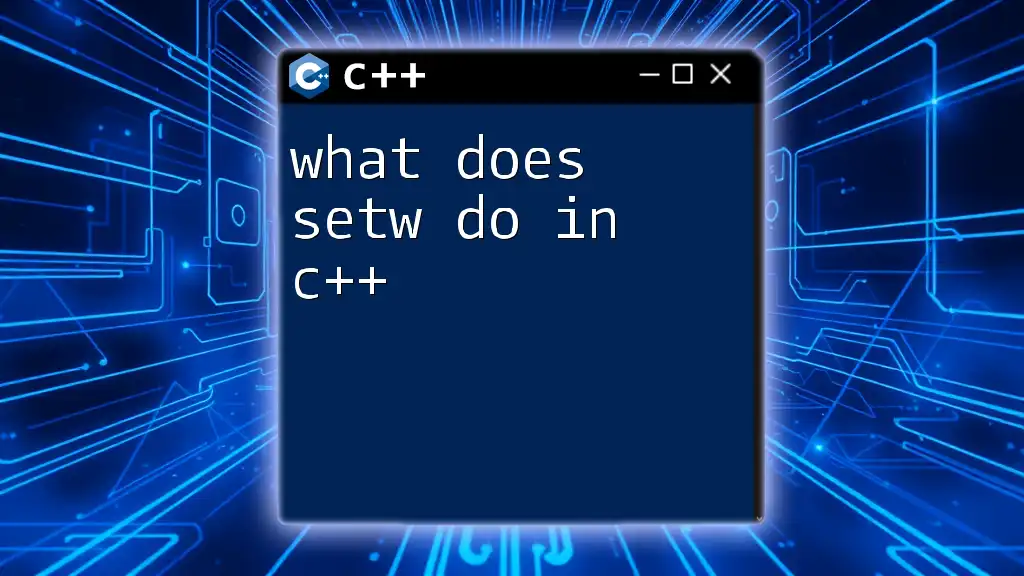
How getline Works in C++
Reading Input with getline
The behavior of `getline` is simple yet powerful. When invoked, it reads all characters from the specified input stream until it reaches a newline character. This feature allows it to capture user input exactly as it is provided.
Consider the following example:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
In this code, the program prompts the user to enter a line, which is stored in the `line` variable and then outputted. It effectively captures any spaces included in the input.
Handling Different Data Types
While `getline` is primarily used for strings, you can handle input of other data types by combining it with stringstreams. Here’s a quick example of how to read a line of text and then convert it to an integer:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a number: ";
std::getline(std::cin, input);
std::stringstream ss(input);
int number;
ss >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
This example showcases how `getline` can read a number in string form and then convert it to an integer.
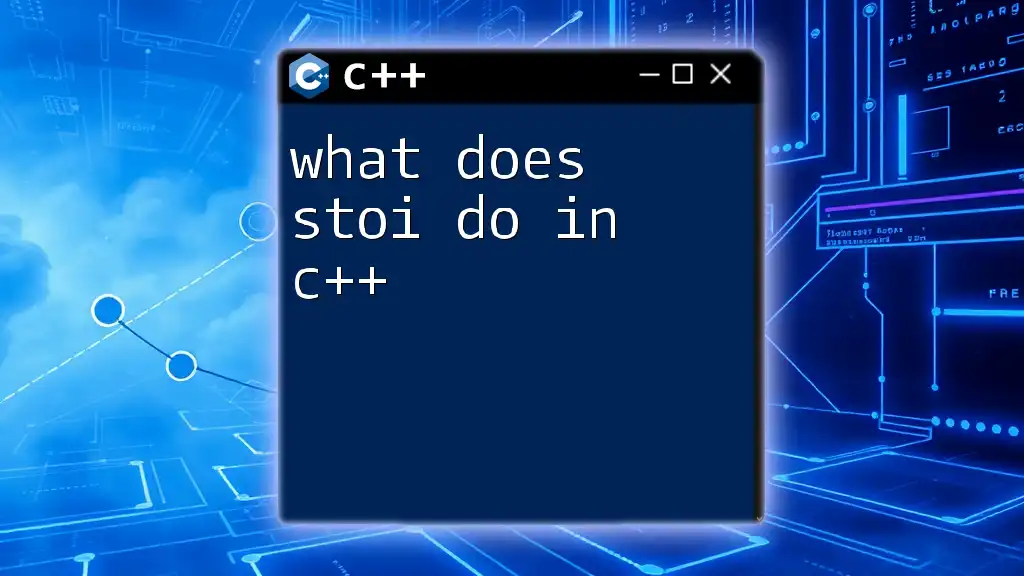
Advanced Features of getline
Specifying a Delimiter
One of the powerful features of `getline` is the ability to specify a different delimiter other than the newline character. This is particularly useful when you want to read data that is structured in a particular way (like CSV).
Here's how to utilize a custom delimiter:
std::string data;
std::cout << "Enter data separated by a comma: ";
std::getline(std::cin, data, ',');
std::cout << "You entered: " << data << std::endl;
In this case, the input will be captured up to the first comma. Everything following the comma will be left unread in the input buffer.
Dealing with Whitespaces
Another important aspect of `getline` is its treatment of whitespace. Unlike `cin`, which skips whitespace by default, `getline` captures everything, including leading and trailing spaces. This can be both an advantage and a consideration.
For instance:
std::string line;
std::cout << "Enter a line with spaces: ";
std::getline(std::cin, line);
std::cout << "Captured line: [" << line << "]" << std::endl;
If the input is " Hello, World! ", the output will reflect the exact input, including the spaces.
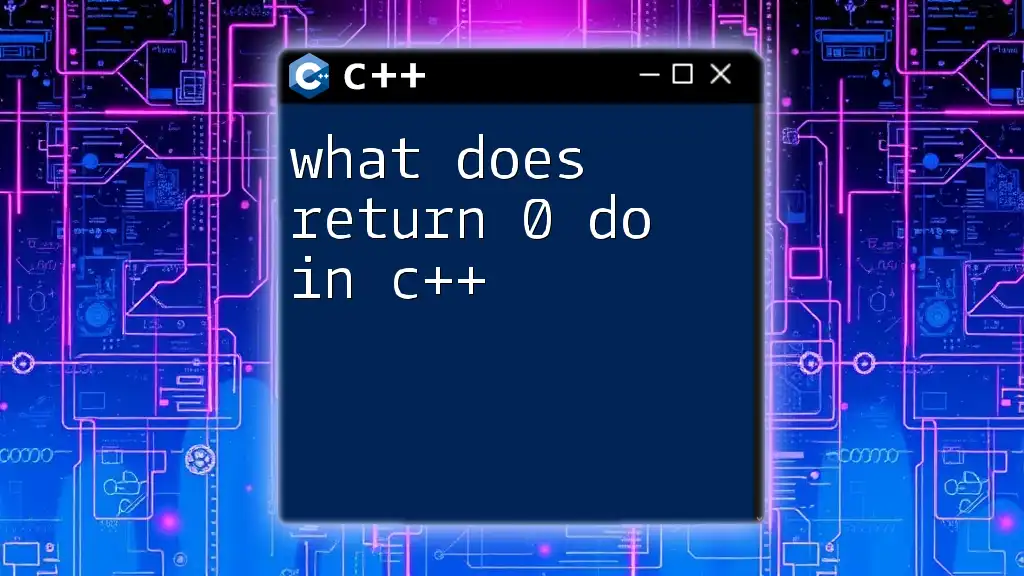
Common Pitfalls and Solutions
Using getline with cin
One common issue arises when mixing `getline` with `cin`. If `cin` was used previously to read data (especially if it’s a numeric type), the newline character left in the input buffer can cause `getline` to return immediately.
To avoid this, you can clear the input buffer before using `getline`:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
// Clear the newline left in the buffer
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
std::string line;
std::cout << "Now enter a line of text: ";
std::getline(std::cin, line);
Buffer Issues
Be aware of potential buffer issues when using `getline`, particularly when repeatedly reading inputs from the same stream. If a previous operation does not consume the entire input, you may end up reading unexpected values. The best practice is to always ensure you are handling your input correctly and flushing the buffer as necessary, especially in interactive programs.
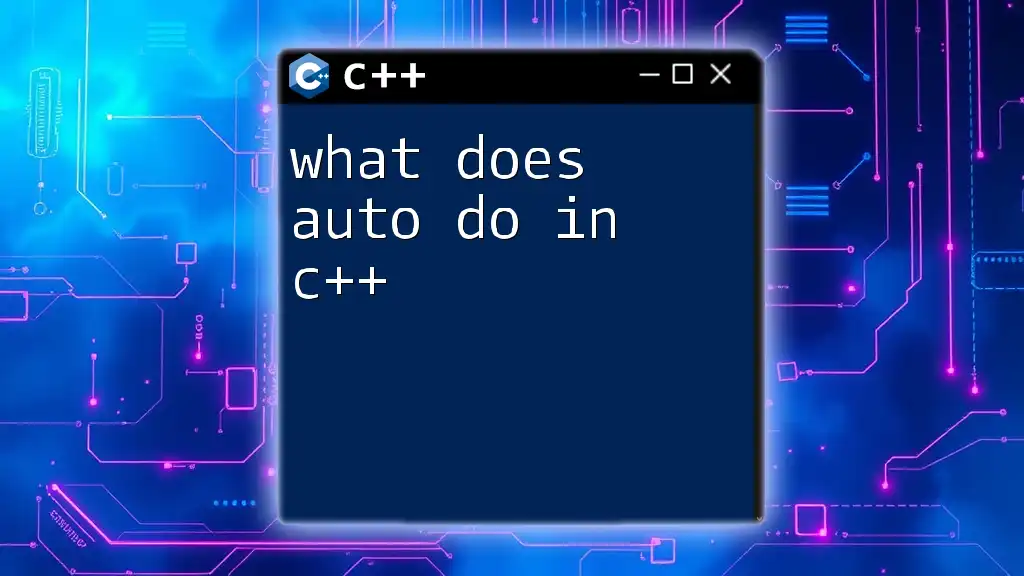
Practical Applications of getline
Reading Multiple Lines
`getline` can also be employed to read multiple lines until an end-of-file (EOF) is encountered. This is especially useful for applications that need to process a bulk of text data.
Here's an example of reading multiple lines:
std::string line;
std::cout << "Enter multiple lines of text (Ctrl+D to end):" << std::endl;
while (std::getline(std::cin, line)) {
std::cout << "You entered: " << line << std::endl;
}
This loop continues reading until the user signals EOF, allowing for continuous data entry.
File Input Handling
`getline` isn't limited to standard input; it can be used to read from files, making it an essential function for file handling in C++. The following example shows how to read lines from a file:
#include <fstream>
std::ifstream file("example.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
This demonstrates how easy it is to read data from a file line by line, making `getline` indispensable for text file processing.
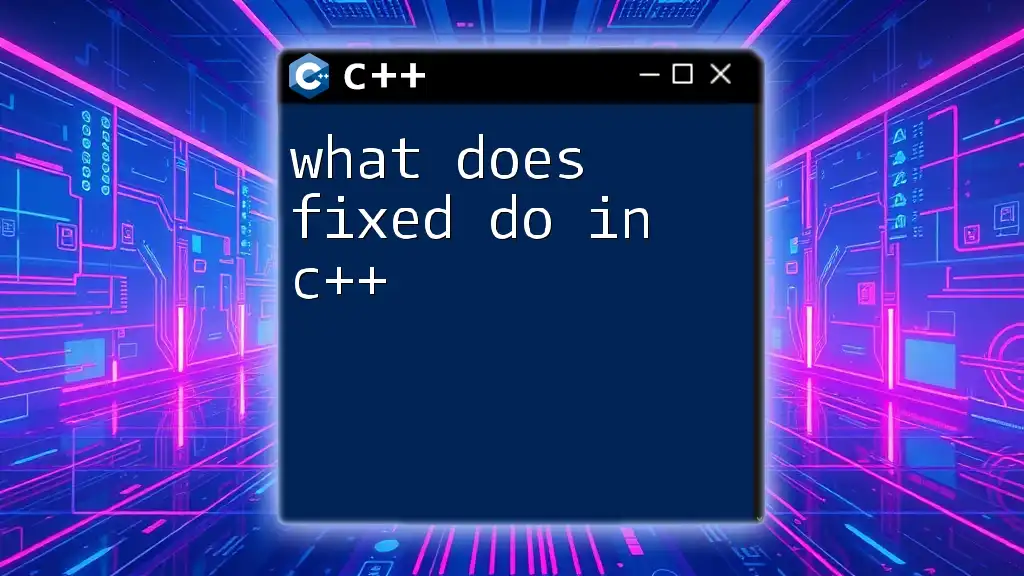
Conclusion
In conclusion, understanding what does getline do in C++ is fundamental for effective input handling in your programs. Its ability to read strings with spaces and specify delimiters sets it apart from other input functions. By keeping in mind common pitfalls and utilizing its advanced features, you can profoundly enhance your ability to manage text data programmatically.
As you move forward, practice using `getline` in your projects and explore its numerous functionalities. C++ offers many resources to deepen your understanding of input handling, and with `getline`, you have a powerful tool at your disposal.