In C++, the `const` keyword is used to define variables whose values cannot be modified after initialization, ensuring data integrity throughout the program.
Here’s a simple example:
#include <iostream>
int main() {
const int maxValue = 100; // maxValue cannot be changed
// maxValue = 200; // This line would cause a compilation error
std::cout << "The maximum value is: " << maxValue << std::endl;
return 0;
}
Understanding `const`
Definition of `const`
In C++, the `const` keyword is a qualifier used to indicate that a variable's value should not be changed after its initial definition. This means that once a variable is declared as `const`, any attempt to modify its value will result in a compilation error.
Purpose of `const`
The primary purpose of using `const` is to enhance code safety and improve readability. By explicitly stating that certain variables, parameters, or functions are not meant to change, developers can prevent unintended side effects, clarify their intentions, and create a more self-documenting codebase.
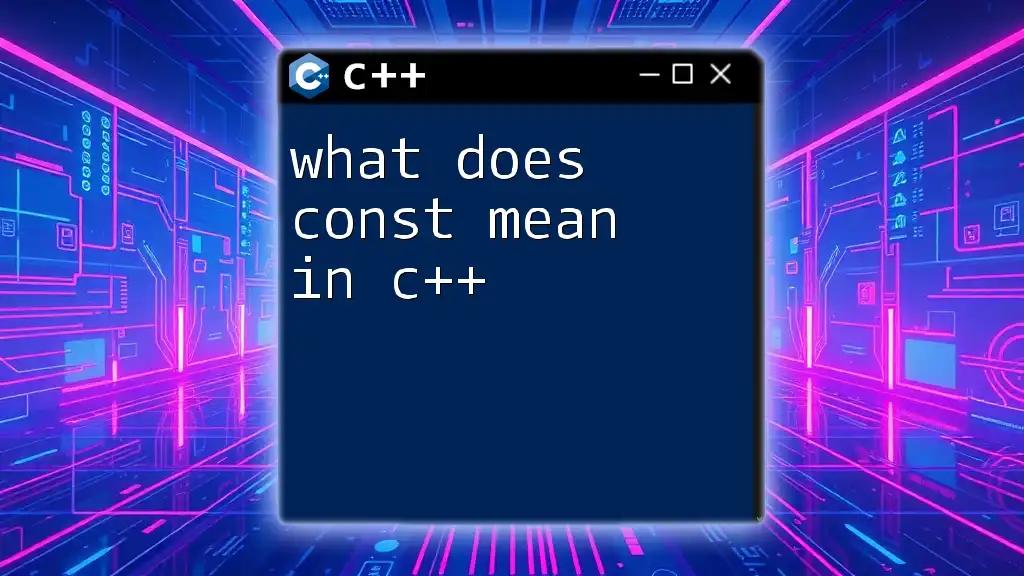
Different Contexts for Using `const`
Const in Variable Declarations
Declaring a variable as `const` is straightforward:
const int MAX_USERS = 100;
In this example, `MAX_USERS` is defined as a constant holding the value of 100. The value of `MAX_USERS` cannot be altered later in the program. This usage is critical in scenarios where the variable serves as a limit or fixed value, ensuring that it remains unchanged throughout the program's execution.
Const with Pointers
Using `const` with pointers can become complex, and understanding its various forms is essential. There are three primary contexts where `const` can be applied to pointers:
Pointer to a Constant
When you declare a pointer to a constant, you can use the pointer to access the value, but you cannot modify the value it points to:
const int* ptr = &someVariable;
This indicates that `ptr` is a pointer to an integer that should not be changed through this pointer.
Constant Pointer
Declaring a constant pointer means the pointer itself cannot be changed to point somewhere else, but you can modify the value it points to:
int* const ptr = &someVariable;
In this case, while the memory address stored in `ptr` cannot be changed (it's constant), the value at that memory location can be altered.
Constant Pointer to a Constant
A constant pointer to a constant means neither the pointer address nor the value pointed to can be changed:
const int* const ptr = &someVariable;
This is the strictest form, ensuring complete immutability.
Const with Function Parameters
Using `const` in function parameters, particularly with reference types, helps to prevent unintended modifications. Here's how you can declare a function using `const`:
void processData(const std::vector<int>& data);
In this case, `data` is a reference to a constant vector of integers. This not only prevents changes to the vector within the function but also enhances performance by avoiding unnecessary copies.
Const with Member Functions
When working with classes, marking member functions as `const` specifies that these functions will not modify the object they belong to. This is especially useful for getter functions, which typically don’t need to change the state of the object:
class Example {
public:
void display() const {
// code to display the object's state
}
};
By declaring `display()` as `const`, it signals to users of the class that invoking this function won’t alter the object, providing a clearer understanding of its functionality.

Benefits of Using `const`
Preventing Accidental Changes
The use of `const` plays a crucial role in preventing accidental modifications. For instance, by declaring critical variables as `const`, you reduce the risk of bugs that may arise from unintended writes.
Optimization by the Compiler
Compilers can optimize code more efficiently when they know certain variables are constant. With `const`, the compiler can make assumptions about values that remain unchanged, leading to improved performance.
Improved Readability and Maintainability
Using `const` enhances code readability. When other developers (or even future you) read the code, the usage of `const` clearly indicates which variables are intended to remain constant. This clarity eases maintenance and collaboration within the codebase.

Common Mistakes When Using `const`
Misunderstanding Pointers with `const`
One common mistake is misinterpreting how `const` applies to pointers. It's crucial to know whether you're applying `const` to the pointer or the data being pointed to. Remember that clarity in intentions matters.
Neglecting `const` in Function Overloading
Another frequent pitfall is forgetting to use `const` when overloading functions. Functions can be overloaded based on parameter types, including `const` versus non-`const`. A failure to account for this could lead to unexpected behavior:
void func(int& x);
void func(const int& x);
Here, the two `func` overloads handle `int` differently, with the second preventing modifications to its parameter.

Practical Examples of `const`
Example Project: Using `const` Effectively
Imagine you are developing a config manager for a software application. Using `const` throughout can safeguard configuration settings:
class Config {
const int maxAttempts;
public:
Config(int attempts) : maxAttempts(attempts) {}
int getMaxAttempts() const {
return maxAttempts;
}
};
In this example, `maxAttempts` is a constant member that cannot be changed, promoting reliability in your configuration management.
Summary of Best Practices
- Always use `const` when dealing with values that should remain unchanged.
- Prefer `const` references for function parameters to improve performance.
- Utilize `const` member functions in classes for improved clarity.

Conclusion
The `const` keyword in C++ is more than just a modifier; it is a powerful tool for improving code safety, readability, and performance. By leveraging `const` appropriately, developers can create more robust, error-resistant applications. Embracing `const` helps in maintaining the integrity of data, while simultaneously communicating intent, making it an essential concept for every C++ programmer.

Additional Resources
- Explore the [C++ Standard Library Documentation](https://en.cppreference.com/w/cpp) for in-depth knowledge.
- Recommended readings include “Effective C++” by Scott Meyers, which offers practical advice on using C++ effectively.
- Online resources such as [Codecademy](https://www.codecademy.com) and [LeetCode](https://leetcode.com) can help reinforce these concepts through practice.