The `break` statement in C++ is used to terminate the nearest enclosing loop or switch statement, allowing the program to control the flow more effectively.
Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Terminates the loop when i equals 5
}
cout << i << " ";
}
return 0;
}
This code prints the numbers 1 to 4, and then stops when `i` equals 5.
Understanding the Break Statement in C++
What is the Break Statement?
The break statement is a powerful control flow statement in C++. It serves as a way to interrupt the normal flow of execution in loops or switch statements. By using the break statement, programmers can exit loops or switch cases prematurely, rather than allowing them to run to completion.
Understanding how and when to implement the break statement is crucial for maintaining efficient and clear code.
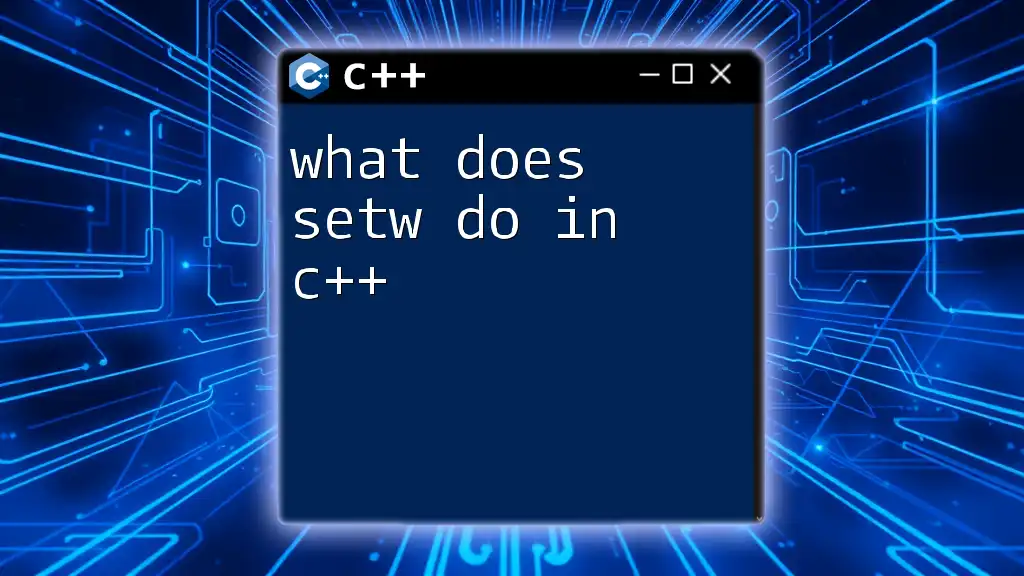
Usage of the Break Statement in C++
Breaking Out of Loops
Loops, such as `for`, `while`, and `do-while`, often use the break statement to provide more control over the iteration process.
For Loop Example
Consider the following code snippet that uses a break statement to exit a for loop when a certain condition is met:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
cout << i << " ";
}
return 0;
}
In this example, the output will be `0 1 2 3 4`. When `i` becomes equal to 5, the break statement is executed, causing the loop to terminate immediately and preventing any further iterations.
While Loop Example
Break statements can also be effectively used in a while loop. Here’s an example:
#include <iostream>
using namespace std;
int main() {
int count = 0;
while (true) { // Continuous loop
if (count == 3) {
break; // Exit when count equals 3
}
cout << count << " ";
count++;
}
return 0;
}
Here, the loop will output `0 1 2`. The while loop is set to run indefinitely, but the break statement ends the loop when the count reaches 3, showcasing how break can control execution even in what seems like an infinite loop.
Do-While Loop Example
Finally, consider the do-while loop:
#include <iostream>
using namespace std;
int main() {
int num = 0;
do {
if (num == 4) {
break;
}
cout << num << " ";
num++;
} while (num < 10);
return 0;
}
This example produces the output `0 1 2 3`. The break statement ensures that once `num` is equal to 4, the loop terminates, even though the condition in the while statement would allow it to continue.
Exiting Switch Statements
Understanding how to break out of switch statements is equally important.
Understanding the Switch Statement
A switch statement evaluates an expression and executes the corresponding case block. Without a break, execution continues to subsequent cases, which may not be the desired behavior.
Example of Break in a Switch Statement
#include <iostream>
using namespace std;
int main() {
int value = 2;
switch (value) {
case 1:
cout << "One";
break; // Exit after executing case 1
case 2:
cout << "Two";
break; // Exit after executing case 2
case 3:
cout << "Three";
break;
default:
cout << "Not one, two, or three";
}
return 0;
}
In this example, if `value` is equal to 2, only "Two" is printed. The break statement prevents any fall-through to subsequent case blocks, ensuring that the control flow is clean and predictable.
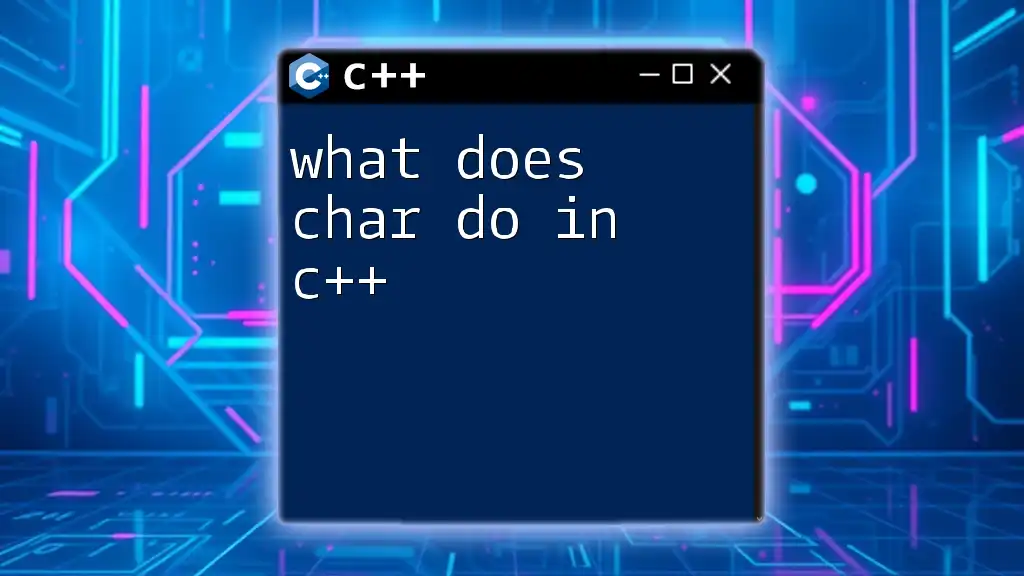
Best Practices for Using the Break Statement
When to Use the Break Statement
The break statement can be particularly useful in scenarios where early termination of loop execution is necessary. For example, if you’re processing input data and wish to stop the loop when a certain condition is met, using break can enhance performance by avoiding unnecessary computations.
Potential Drawbacks of Using Break
Despite its usefulness, over-reliance on break statements can lead to poor code readability. When multiple breaks are scattered throughout code, it can make understanding the execution flow more challenging.
Consider avoiding break statements in simple loops where conditions can be handled directly in the loop's logic. This can lead to cleaner and more understandable code.
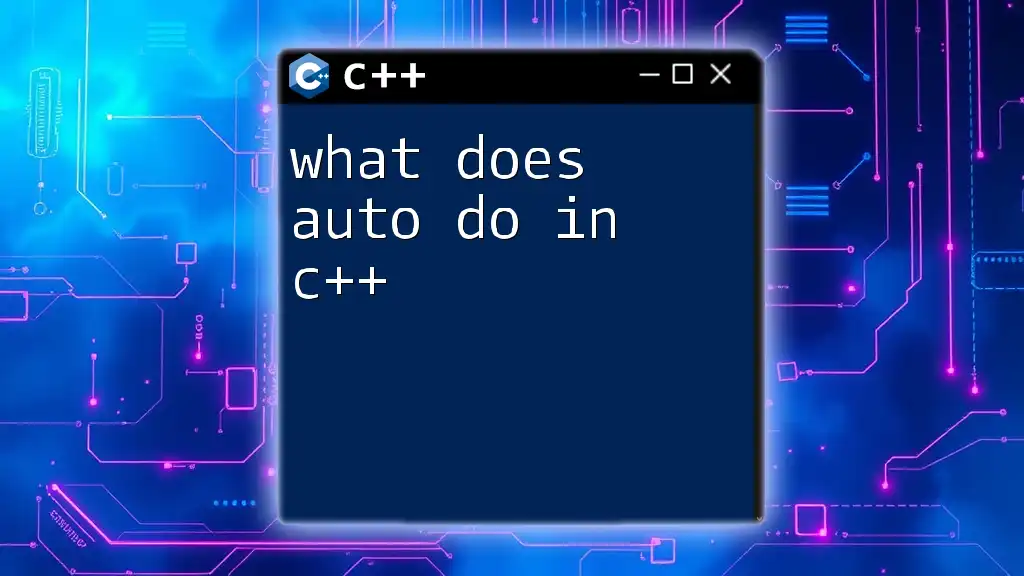
Advanced Concepts: Break Statement in Nested Structures
Breaking Out of Nested Loops
In scenarios where loops are nested, the break statement only exits the innermost loop. This is crucial for developers to understand to avoid unintended behavior.
Example of Break in Nested Loops
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (j == 1) {
break; // Only exits the inner loop
}
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
This will output:
i: 0, j: 0
i: 1, j: 0
i: 2, j: 0
The inner loop is exited upon meeting the condition `j == 1`, while the outer loop continues to its next iteration.
Labeled Break Statements
C++ also allows the use of labeled break statements for more complex control flow management.
Understanding Labeled Breaks
A labeled break statement allows you to break out of not just the innermost loop but from any outer loop you specify by a label. This can enhance clarity in deeply nested structures.
Example of Labeled Break Statement
#include <iostream>
using namespace std;
int main() {
outerLoop: for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (i == 2) {
break outerLoop; // Exit both loops
}
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
In this example, the program exits both loops once `i` becomes equal to 2, stopping execution entirely when the outer loop condition is triggered.
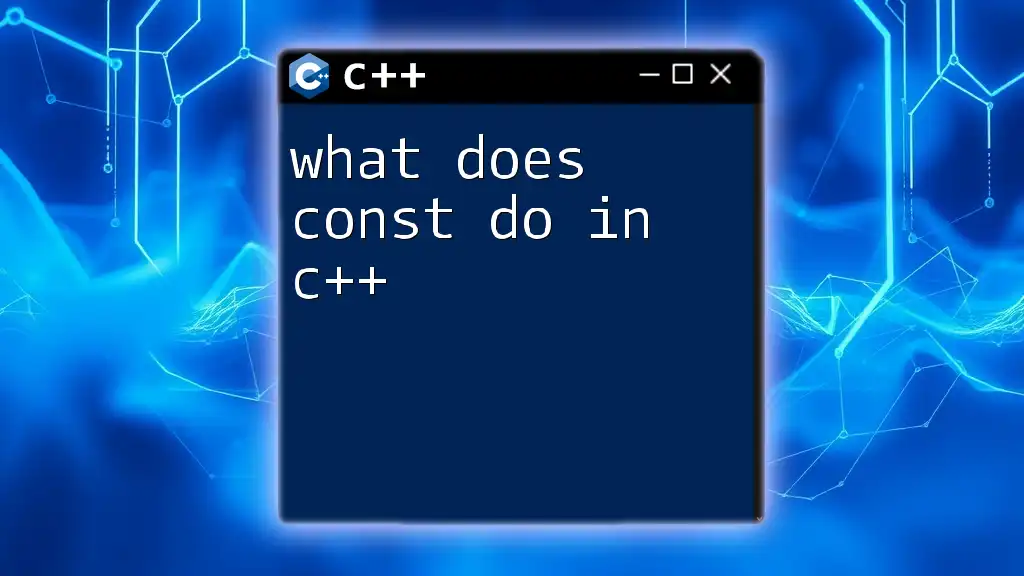
Conclusion
The break statement is a vital tool in C++ for controlling program flow within loops and switch statements. Proper understanding and application of the break statement can lead to clearer, more maintainable code while preventing unnecessary iterations and operations.
Final Thoughts on Effective Usage of Break in C++
Using the break statement efficiently enables you to write clean, concise code. As you continue to learn and apply C++, keep these principles in mind to promote best practices in your programming.