In C++, the `do` keyword is used to create a do-while loop, which executes a block of code at least once and then repeatedly executes it as long as a specified condition is true.
Here's a simple example of a do-while loop in C++:
#include <iostream>
using namespace std;
int main() {
int count = 0;
do {
cout << "Count: " << count << endl;
count++;
} while (count < 5);
return 0;
}
What is the `do` Statement?
The `do` statement in C++ is a control flow statement designed for looping. It is used when you want a block of code to run repeatedly as long as a specified condition remains true. What sets the `do` statement apart from other loops, particularly the `while` loop, is that the `do` loop guarantees that the code block executes at least once, regardless of whether the condition evaluates to true or false on the first check.
Syntax of the `do` Statement
Understanding the syntax is crucial to utilizing the `do` statement effectively. Here is the general structure:
do {
// code block to be executed
} while (condition);
Components of the Syntax:
- do: This keyword signifies the beginning of the loop.
- Code Block: This is the section where the operations take place. It can include multiple statements, and all of them will be executed at least once.
- while (condition): This condition is evaluated after the code block is executed. If it returns true, the loop will continue; if it returns false, the loop will terminate.

When to Use the `do` Statement
In certain scenarios, using a `do` loop is preferable or even necessary. If you need to ensure that the loop's body executes at least once before any condition is checked, the `do` statement is the perfect choice.
Situations Favoring `do`
Examples of situations where a `do` loop is beneficial include:
- Input Validation: When you want to prompt users until they provide valid input.
- Menu-Driven Applications: When you want to display a menu to the user and allow them to choose an option, ensuring the menu is displayed at least once.
Real-World Applications
#include <iostream>
using namespace std;
int main() {
char choice;
do {
cout << "1. Option A\n";
cout << "2. Option B\n";
cout << "3. Exit\n";
cout << "Choose an option: ";
cin >> choice;
} while (choice != '3');
cout << "Exiting the program." << endl;
return 0;
}
In the example above, the menu will be displayed at least once, allowing the user to choose an option, which showcases a typical use case of the `do` statement.

How the `do` Statement Works
Flow of Execution
The execution flow of a `do` loop can be summarized in three key steps:
- The code block is executed first.
- The condition is evaluated after the block's execution.
- If the condition is true, the loop repeats; otherwise, it exits.
An essential characteristic of the `do` loop is that it checks the condition at the end, meaning that even if the condition is false initially, the code inside the loop will still execute once.
Example Code Snippet
To illustrate the flow further, consider the following example:
#include <iostream>
using namespace std;
int main() {
int num;
do {
cout << "Enter a positive number: ";
cin >> num;
} while (num <= 0);
cout << "You entered: " << num << endl;
return 0;
}
In this snippet, the user will be prompted for a positive number. Should they enter a negative number or zero, the loop will continue prompting until a valid number is provided – reflecting the practicality of the `do` statement.

Comparison with Other Looping Constructs
`do` vs. `while`
The primary difference between the `do` and `while` statements lies in when the condition is evaluated. In a `while` loop, the condition is checked before the loop's code block executes, which may lead to no execution if the condition is already false.
Example:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a positive number: ";
cin >> num;
while (num <= 0) {
cout << "Enter a positive number: ";
cin >> num; // Prompting occurs only if the user input initially was invalid.
}
cout << "You entered: " << num << endl;
return 0;
}
`do` vs. `for`
The `for` loop is generally preferred for situations where the number of iterations is known beforehand, while the `do` loop is ideal when the condition should be checked after the code has executed.
Example:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; ++i) {
cout << i << endl; // This will execute exactly five times based on a known count.
}
return 0;
}
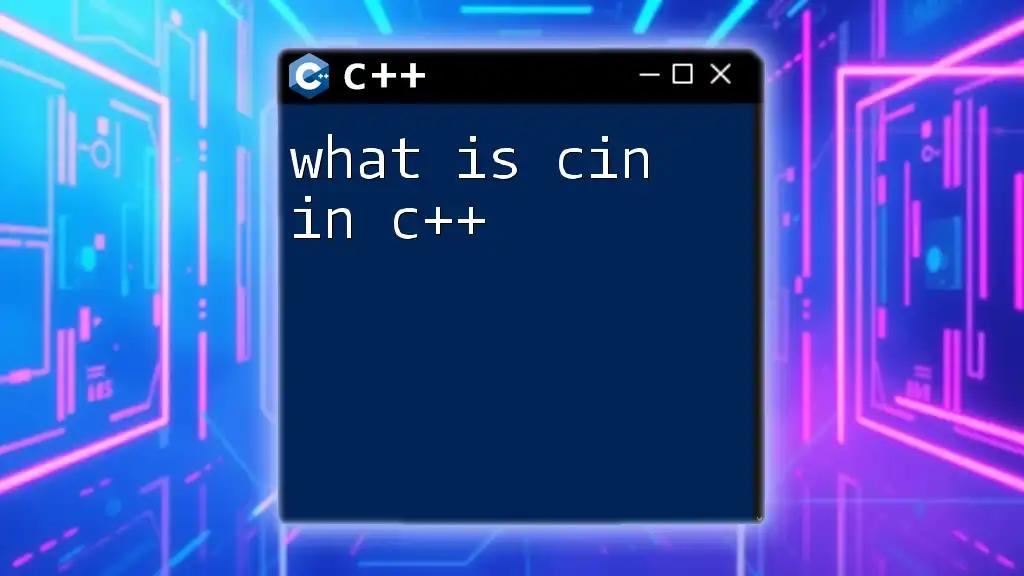
Common Mistakes with the `do` Statement
Misusing Condition
One common pitfall when using the `do` statement is an improper condition, which can lead to infinite loops. Careful crafting of conditions is essential to avoid scenarios where the loop never terminates.
Forgetting the Semicolon
Another frequent mistake is neglecting to include the semicolon after the `while` condition. This simple typo can generate compilation errors that might be confusing for beginners.

Best Practices for Using the `do` Statement
Code Readability
Maintaining readability in your code is paramount. Proper spacing and clear comments can help yourself and others quickly grasp the logic of the `do` statement. For example, clearly indicating the loop's purpose within the code comments can save time for anyone examining your code later.
Proper Exit Conditions
Crafting effective exit conditions is vital. Make sure that the condition evaluated at the end of the loop is both meaningful and effective, ensuring that the loop doesn't run longer than intended.
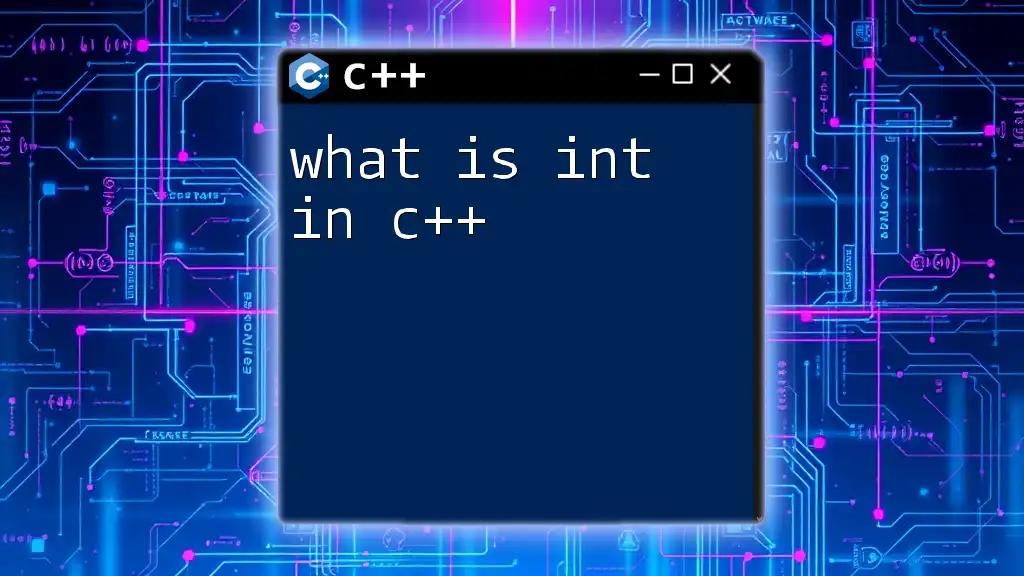
Conclusion
Understanding what does `do` in C++ do is essential for effective programming. The `do` statement provides a powerful tool for looping in situations where the body of the loop must execute at least once. By grasping the unique attributes of the `do` statement, as well as the potential pitfalls and best practices associated with it, programmers can significantly enhance their control flow and coding efficiency.

Bonus Section: Additional Resources
Recommended Readings
For those seeking to deepen their understanding of C++, numerous resources are available, such as classic programming books, online courses, and comprehensive coding websites that cover not just the `do` statement but also the full spectrum of C++ programming techniques.
Community and Support
Engaging with community forums, programming groups, and educational platforms can provide valuable support and answer queries as you navigate the complexities of C++ programming. These communities are often full of experienced developers who can share insights and clarifications on the language’s intricacies.