C++ is a powerful programming language known for its efficiency and flexibility, often structured with classes, functions, and variables, allowing for both procedural and object-oriented programming.
Here's a simple code snippet showcasing the basic syntax of a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
The Basics of C++ Syntax
What is C++?
C++ is a powerful programming language that evolved from C, adding object-oriented features alongside its procedural roots. It was created by Bjarne Stroustrup in 1979 and has since become a cornerstone in software development, particularly for systems programming, game development, and applications requiring real-time processing.
Understanding what C++ looks like hinges on grasping its syntax and structure, making it easier for programmers to write efficient and effective code. The language's versatility allows developers to manipulate system resources directly while offering abstractions that simplify complex tasks.
Structure of a C++ Program
At its core, every C++ program shares a fundamental structure, characterized by the following components:
Main Function: The execution of any C++ program begins in the `main()` function, which serves as the entry point. Every program must include a main function.
int main() {
return 0; // Indicates successful execution
}
Header Files: These files include predefined classes and functions that enhance functionality. By using the `#include` directive, developers can access these libraries. For example, including the iostream library allows the use of input/output streams.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Basic Elements of C++ Code
C++ code contains several basic elements that are critical for effective programming.
Comments: Comments are vital for code documentation and making it understandable for others (and yourself). Single-line comments use `//`, while multi-line comments are enclosed in `/` and `/`.
// This is a single-line comment
/* This is a
multi-line comment */
Variables and Data Types: Variables store data for processing. C++ supports various data types, including `int`, `float`, `char`, and `string`, each serving different purposes. For instance:
int age = 25; // Integer
float height = 5.9; // Floating-point number
char initial = 'J'; // Character
Operators in C++
Operators are essential tools for manipulating variables and data.
Arithmetic Operators include addition (+), subtraction (-), multiplication (*), and division (/). These are foundational for performing calculations within the program. For example, to add two numbers:
int sum = a + b; // where 'a' and 'b' are integers
Logical Operators (AND, OR, NOT) help in making decisions and controlling the flow of programs. The common logical operators are `&&`, `||`, and `!`.
Comparison Operators allow you to compare values. These include `==`, `!=`, `>`, `<`, `>=`, and `<=`. They are frequently used in conditional statements to determine the flow of execution.

Control Structures in C++
Conditional Statements
C++ provides various ways to implement decision-making structures.
If Statements allow you to execute code based on a condition. For example:
if (age >= 18) {
std::cout << "Adult" << std::endl;
}
Here, the message "Adult" will be printed only if the condition is true.
Switch Case is another form of control structure that is useful when you want to execute a block of code based on the value of a variable.
switch (grade) {
case 'A':
std::cout << "Excellent!" << std::endl;
break;
case 'B':
std::cout << "Well done!" << std::endl;
break;
default:
std::cout << "Invalid grade" << std::endl;
}
Loops
Loops allow you to execute code repeatedly based on conditions.
For Loops are used when the number of iterations is known. The syntax is clean and straightforward:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl; // Outputs 0 to 4
}
While Loops are used when the number of iterations is not predetermined. Here’s a simple example:
int i = 0;
while (i < 5) {
std::cout << i << std::endl; // Outputs 0 to 4
i++;
}
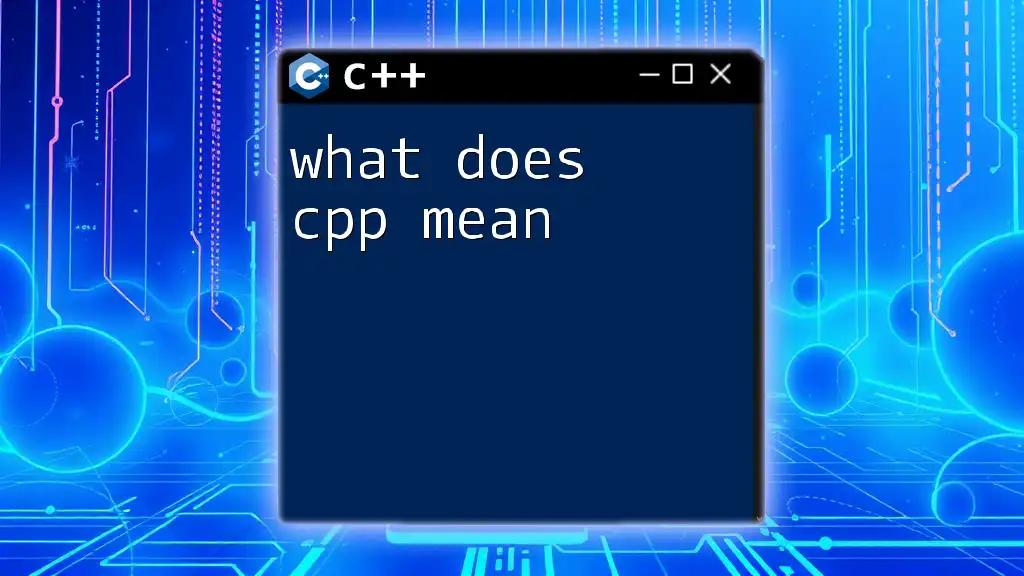
Functions in C++
Defining Functions
Functions are reusable blocks of code that perform specific tasks. They promote code organization and reduce redundancy.
Function Syntax is straightforward, comprising the return type, function name, and parameters. For example:
int add(int a, int b) {
return a + b; // Returns the sum of 'a' and 'b'
}
Function Overloading
In C++, the same function name can be reused with different parameters; this is known as function overloading. It allows you to define multiple behaviors for similar functionality.
int add(int a, int b); // Function for integers
float add(float a, float b); // Function for floating-point numbers

Object-Oriented Programming in C++
Classes and Objects
C++ is an object-oriented programming language, which means it uses classes to define data structures, encapsulating data and behavior together. For example:
class Car {
public:
std::string model;
int year;
void display() {
std::cout << model << " - " << year << std::endl;
}
};
This `Car` class contains characteristics (attributes) and behaviors (methods) related to a car.
Inheritance
Inheritance allows one class (derived class) to inherit attributes and methods from another class (base class). Here's a simple example demonstrating this concept:
class Vehicle { // Base class
public:
void drive() {
std::cout << "Driving" << std::endl;
}
};
class Car : public Vehicle { // Derived class inheriting from Vehicle
};

Advanced Topics
Templates
Templates enable code reusability and flexibility by allowing functions and classes to operate with any data type. For example, a simple function template can be defined as follows:
template <typename T>
T add(T a, T b) {
return a + b; // Adds two values of type T
}
Exception Handling
C++ provides robust mechanisms for error handling known as exception handling. It involves using `try`, `catch`, and `throw` keywords. An example of handling exceptions is outlined below:
try {
throw std::runtime_error("Error occurred!"); // Throwing an exception
} catch (const std::runtime_error &e) {
std::cout << e.what() << std::endl; // Catching and printing the exception
}

Conclusion
In summary, understanding what C++ looks like is fundamental for aspiring programmers. Mastering its syntax, control structures, functions, and object-oriented principles lays a strong foundation for effective programming. As you delve deeper into C++, keep practicing and exploring its extensive features to enhance your proficiency and skills in this versatile programming language.

Call to Action
Join our C++ training programs today to elevate your coding skills and explore the vast potential of C++. Whether you're just starting or looking to refine your expertise, our resources are designed to guide you through every step of your programming journey!