C++ uses header files to declare the structure of classes, functions, and variables, enabling code organization and modularity for better management and reuse across multiple source files.
// example_header.h
#ifndef EXAMPLE_HEADER_H
#define EXAMPLE_HEADER_H
void exampleFunction();
#endif // EXAMPLE_HEADER_H
What Are Header Files?
Definition of Header Files
Header files are files that contain declarations of functions, classes, and other constructs that can be shared between multiple source files in a C++ program. They typically have the extensions `.h` or `.hpp` and serve as an important part of the C++ programming structure.
Typical Contents of Header Files
Header files commonly include the following elements:
- Function Declarations: These specify the function's name, return type, and parameters without providing the actual implementation.
- Class Definitions: Header files usually contain the definitions of classes, including their member variables and functions.
- Macro Definitions: Header files can define preprocessor macros that can be used throughout the code.
- Constants and Type Definitions: They often define constants or types through `typedef` or using `using` directives to streamline reference across multiple files.
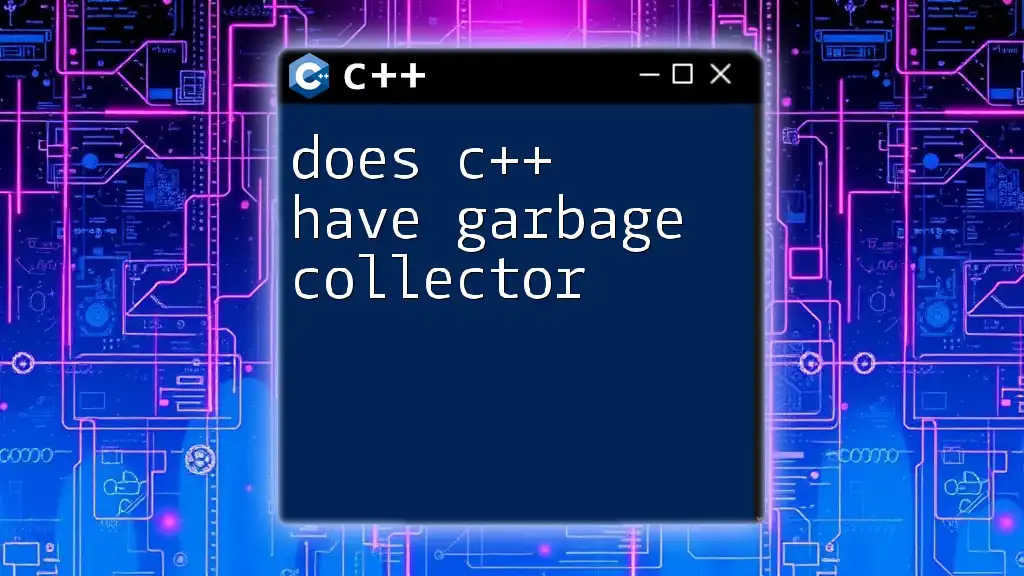
Purpose of Header Files
Code Organization and Structure
One of the fundamental reasons why C++ has header files is to improve code organization. In larger programs, keeping all code in a single file can lead to chaos and difficulty in maintenance. Header files allow developers to break up the code into smaller, manageable sections, making the codebase cleaner and easier to navigate.
For example, consider a small project involving user management. By separating user-related functions and classes into a dedicated header file, developers ensure that all user-related code is organized in one place, making it easier to locate and modify.
Reusability of Code
Header files also significantly enhance the reusability of code. By declaring functions and classes in header files, developers can easily include these files across various source files without needing to rewrite the code. This not only saves time but also ensures consistency across multiple parts of the application.
For instance, imagine creating a math library that provides several complex functions. By placing the declarations into a header file, developers can simply include this file in different projects or modules, thus promoting code reuse.
Separation of Interface and Implementation
Another crucial aspect of why C++ has header files is to establish a clear distinction between the interface and implementation. A header file typically contains declarations that define what functions and classes do, while the actual implementation resides in a separate `.cpp` file.
Consider the following code snippets:
// MathFunctions.h
double add(double a, double b);
// MathFunctions.cpp
#include "MathFunctions.h"
double add(double a, double b) {
return a + b;
}
In this case, the header file `MathFunctions.h` declares the `add` function while the implementation is provided in `MathFunctions.cpp`. This separation improves maintainability, as changes to the implementation won’t require changes to every file that uses the `add` function.
Forward Declarations
Header files also allow for forward declarations, a concept that enables developers to declare a class without providing the complete definition. This can help reduce dependencies and improve compilation time, as it limits the inclusion of unnecessary headers.
For example:
// Forward declaration
class Person;
// Function that takes a Person as a parameter
void printPersonName(Person* p);
Here, the `Person` class is declared but not fully defined. This technique is essential for breaking circular dependencies in code.
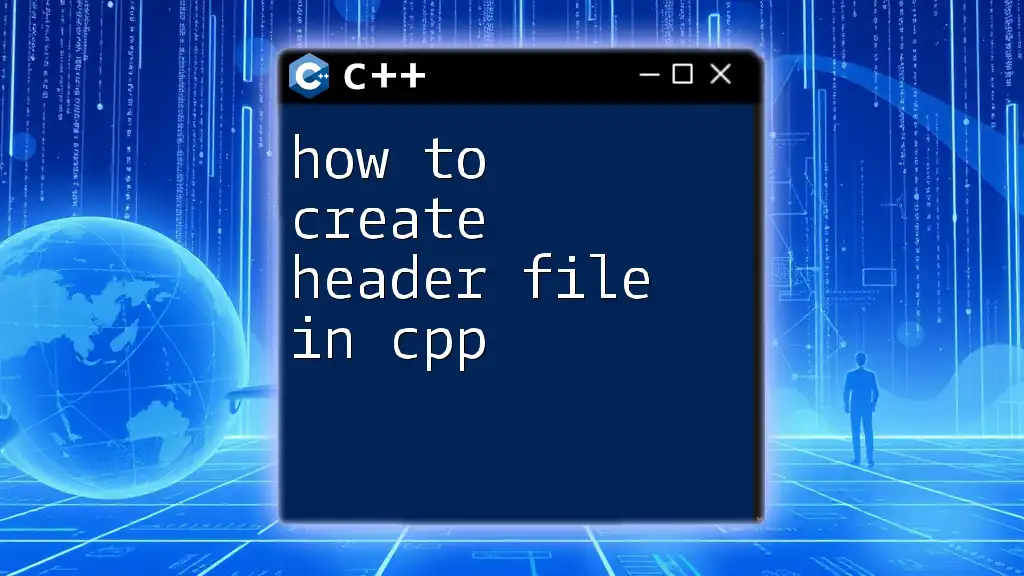
How to Use Header Files
Creating a Header File
Creating a header file involves crafting a structured format that includes necessary declarations. Below is a simple example of how to create a header file for a `Person` class:
// Person.h
#ifndef PERSON_H
#define PERSON_H
#include <string>
class Person {
public:
Person(const std::string& name);
void greet();
private:
std::string name;
};
#endif // PERSON_H
This code snippet clearly outlines a `Person` class, including a constructor and a member function called `greet`. The use of `#ifndef`, `#define`, and `#endif` is essential for including guards that prevent multiple inclusions.
Including Header Files
Incorporating header files into C++ source files is done using the `#include` directive. Below is an example of how to include the previously created `Person` header file and utilize its functionality:
#include "Person.h"
int main() {
Person p("Alice");
p.greet();
return 0;
}
In this scenario, the `Person` class is included in the main source file, providing access to its functionalities.
Guarding Against Multiple Inclusions
Header files must be protected against multiple inclusions to avoid redefinition errors. This is accomplished using include guards as demonstrated in the previous `Person.h` example. Implementing these precautions is critical to ensure that the compiler processes the header only once per translation unit.
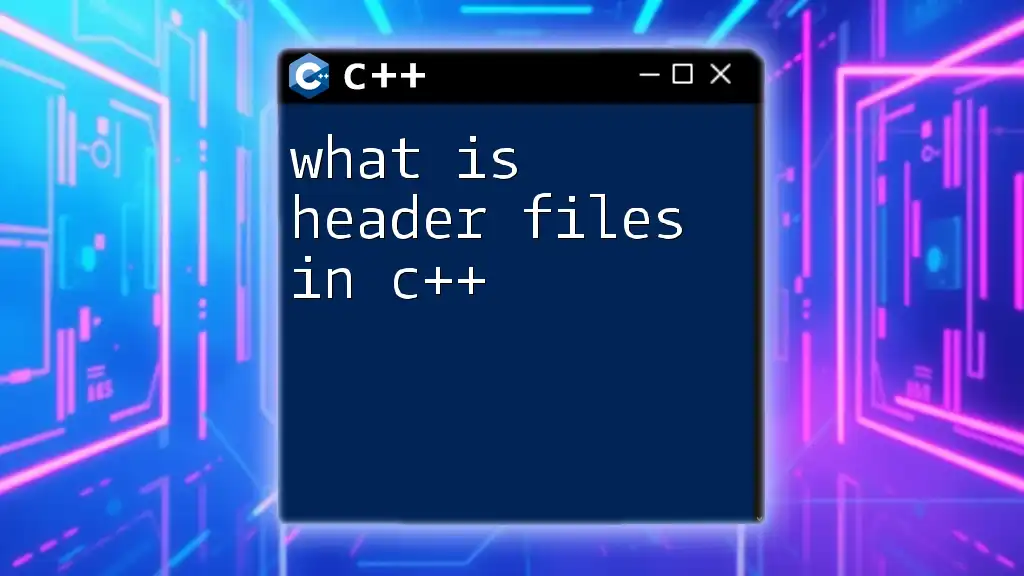
Best Practices for Using Header Files
Maintainability
When considering why C++ has header files, it's essential to highlight that well-structured header files significantly contribute to project maintainability. To achieve this, developers are advised to:
- Keep header files concise and focused.
- Avoid lengthy header files packed with unnecessary declarations.
Avoiding Circular Dependencies
Circular dependencies can lead to complex issues in C++ projects. It is a situation where two or more header files include each other, causing infinite loops of inclusion. Strategies to avoid such situations involve:
- Using forward declarations where possible.
- Rethinking the design to isolate dependent headers in a way that minimizes coupling.
Using Include Directives Responsibly
Minimizing unnecessary included headers within header files is critical for maintaining clean compilation units. By limiting dependencies, developers can prevent excessive compilation times and reduce potential errors due to interface changes. Whenever feasible, prefer forward declarations over full includes, especially in header files.
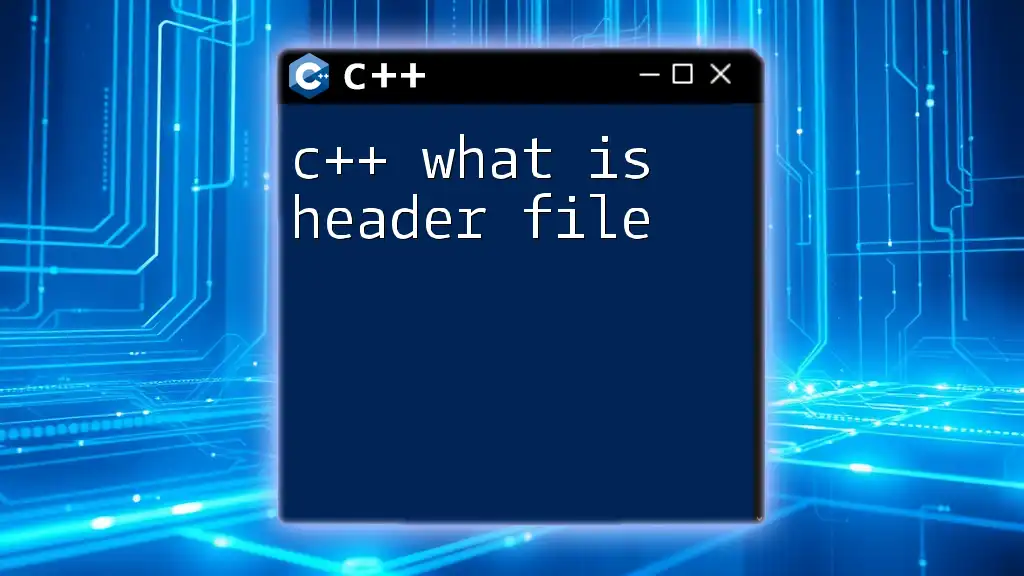
Conclusion
In summary, header files play a vital role in C++ programming, facilitating code organization, reusability, and clarity in separation between interfaces and implementations. Understanding why C++ has header files is key to developing cleaner, more maintainable code.
As you continue your journey in C++, consider incorporating header files to enhance your projects. Creating your own structured header files is an excellent way to practice these concepts and improve overall coding efficiency. Whether you’re a beginner or an experienced developer, embracing header files will significantly benefit your code structure and maintainability.