C++ is used for games due to its high performance and ability to directly manipulate hardware, allowing developers to create complex graphics and efficient game engines.
Here's a simple C++ code snippet that demonstrates the creation of a basic game loop:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
while (true) {
// Game logic update
std::cout << "Game is running..." << std::endl;
// Simulate frame rate
std::this_thread::sleep_for(std::chrono::milliseconds(16)); // ~60 FPS
}
return 0;
}
Understanding C++
What is C++?
C++ is a high-performance programming language that evolved from the C language, introducing object-oriented programming (OOP) paradigms. It combines the efficiency and power of low-level programming with the abstract capabilities of high-level languages, making it a versatile choice for various applications, particularly in game development.
Evolution of C++
C++ has a rich history, having been created in the early 1980s by Bjarne Stroustrup. Over decades, it has undergone various enhancements, with each new standard (like C++11, C++14, C++17, and C++20) adding features that support the evolving needs of the programming community. Its continued relevance is underscored by its adoption in the gaming industry, where performance and speed are paramount.
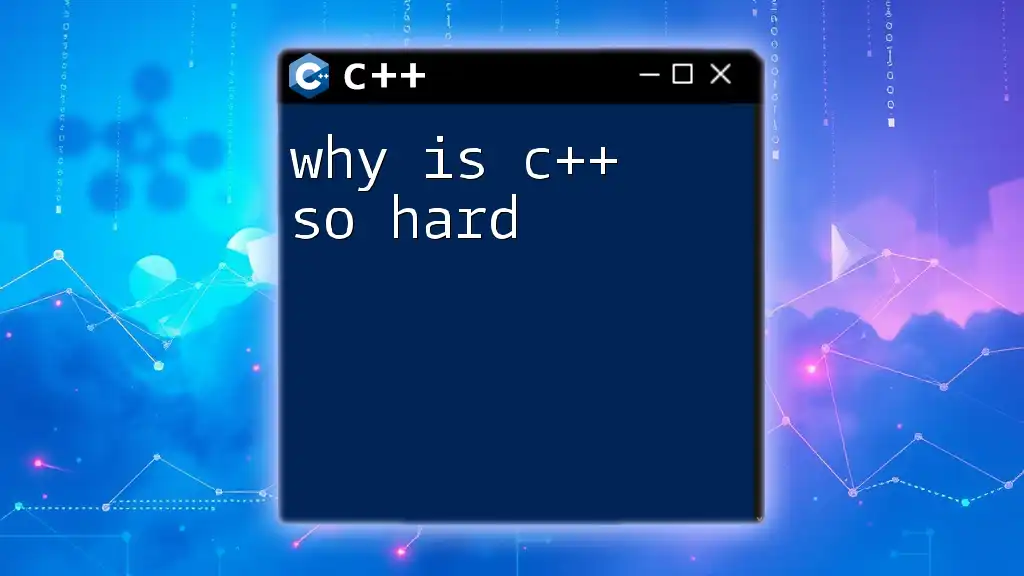
Performance and Efficiency
Speed and Memory Management
When it comes to gaming, performance often differentiates a great game from an outstanding one. C++ excels in speed due to its low-level programming capabilities, which allow developers to write code that makes optimum use of system resources. This performance edge is crucial for graphics-intensive applications like games.
C++ provides fine control over memory management. Developers can dynamically allocate memory when necessary, enhancing performance by preventing unnecessary copies. For instance:
int* myArray = new int[10]; // Creating an array dynamically
delete[] myArray; // Freeing memory
This ability allows game developers to manage resources efficiently, balancing speed and memory usage.
Resource Management
Control over system resources is another critical advantage of using C++ in game development. C++ allows developers to work directly with pointers and references. For example:
int score = 0;
int* scorePtr = &score; // Pointer to score
Here, the pointer provides direct access to the score variable, allowing developers to manipulate data efficiently and effectively manage resources during gameplay.
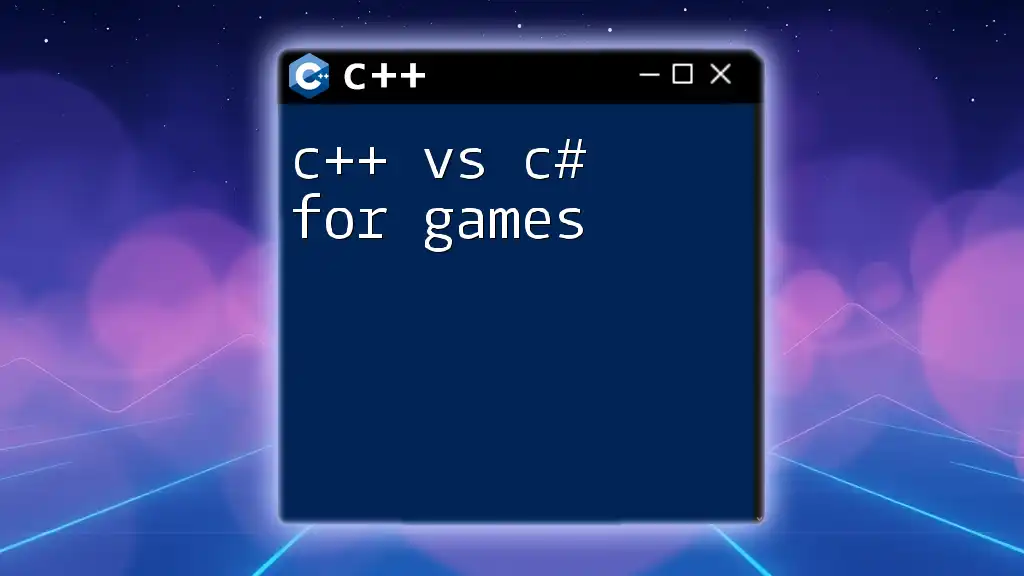
Object-Oriented Programming
Encapsulation
Encapsulation, one of the cornerstones of OOP, is vital for managing complexity in game development. It helps in creating self-contained modules that merge data and functions. For example, consider a simple `Player` class:
class Player {
private:
int health;
public:
void takeDamage(int damage) { health -= damage; }
};
This design ensures that the `health` variable is protected from unwanted access from outside the class, promoting a clean and maintainable codebase.
Inheritance and Polymorphism
C++ allows for the creation of a class hierarchy using inheritance, which helps organize game entities into an extensible structure. For instance, a `GameObject` base class can have specific types of game objects inherit from it, like an `Enemy` class:
class GameObject {
public:
virtual void update() = 0; // Pure virtual function
};
class Enemy : public GameObject {
public:
void update() override {
// Enemy-specific update logic
}
};
In this example, polymorphism allows different game entities to define their own versions of the `update` method, providing flexibility and code reuse across game projects.
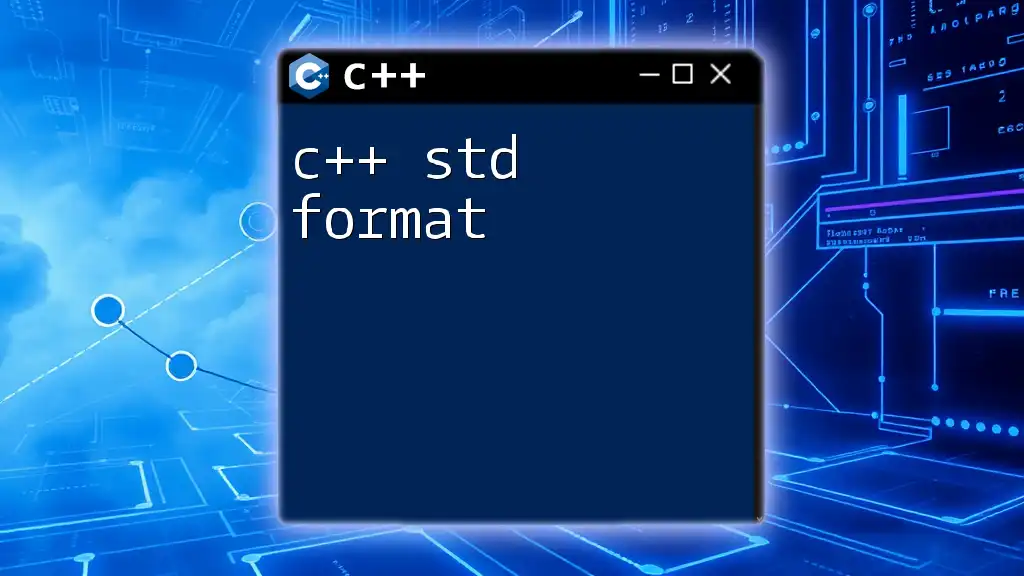
Flexibility and Control
Low-Level Access
C++ facilitates low-level access to hardware, enabling developers to fine-tune performance for demanding graphics and computational tasks. This level of control is essential in the gaming industry, where optimizing for various hardware configurations can significantly impact playability.
Cross-Platform Compatibility
C++ is inherently cross-platform, making it a popular choice among developers for targeting multiple platforms, including consoles like Xbox and PlayStation and PCs. This versatility is especially apparent in popular game engines, such as Unreal Engine, which relies heavily on C++ to offer robust performance across different gaming environments.
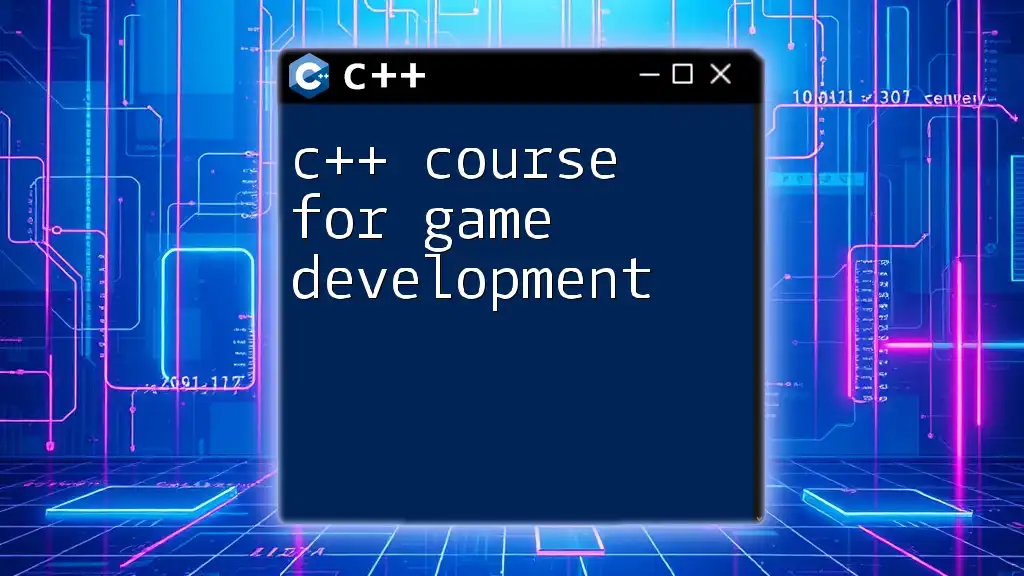
Rich Libraries and Frameworks
Game Development Frameworks
There are numerous frameworks built on C++ that simplify the game development process. For instance, libraries like SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer) provide essential tools for rendering graphics, handling input, and managing sound.
Here's a simple example using SFML to create a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
This demonstrates how easily developers can set up a graphics window, showcasing the language’s capability to streamline common gaming tasks.
Game Engines
Popular game engines like Unreal Engine leverage C++ to maximize performance and flexibility. The engine’s architecture allows developers to write performance-critical code in C++, while also providing a rich set of tools to facilitate game development. The performance benefits are tangible, particularly in rendering detailed graphics and managing game logic.
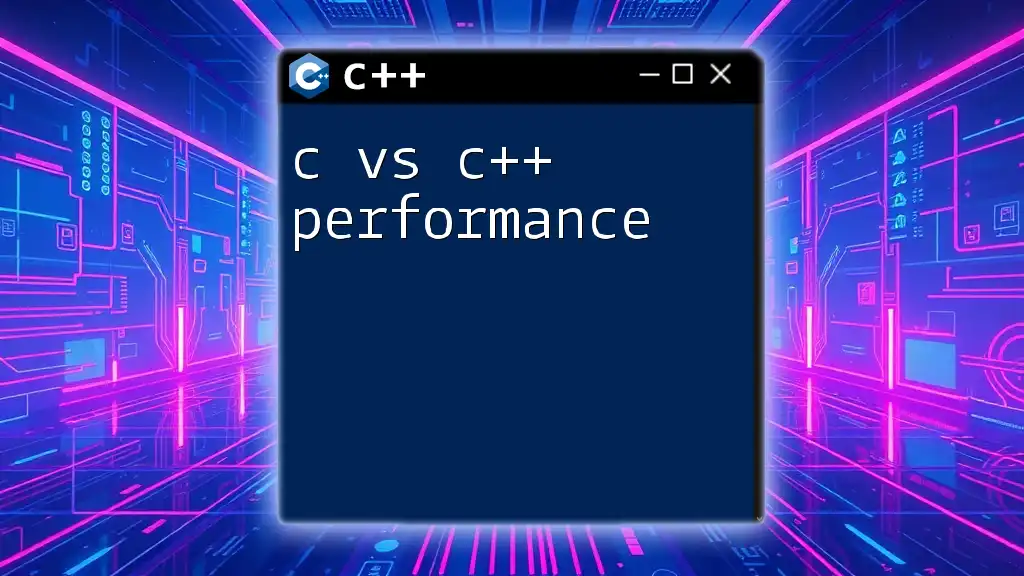
Community and Support
Large Developer Community
One of the significant advantages of using C++ is its extensive developer community. This community includes experts who actively contribute resources, tutorials, and libraries that new developers can utilize. Forums such as Stack Overflow or specific C++ communities are rich with shared knowledge and support for aspiring game developers.
Extensive Documentation
C++ is well-documented, with a plethora of resources available for beginners and veterans alike. Websites like cppreference.com provide comprehensive information about C++ standard libraries and functionality, making it easier to learn and implement more advanced programming techniques.
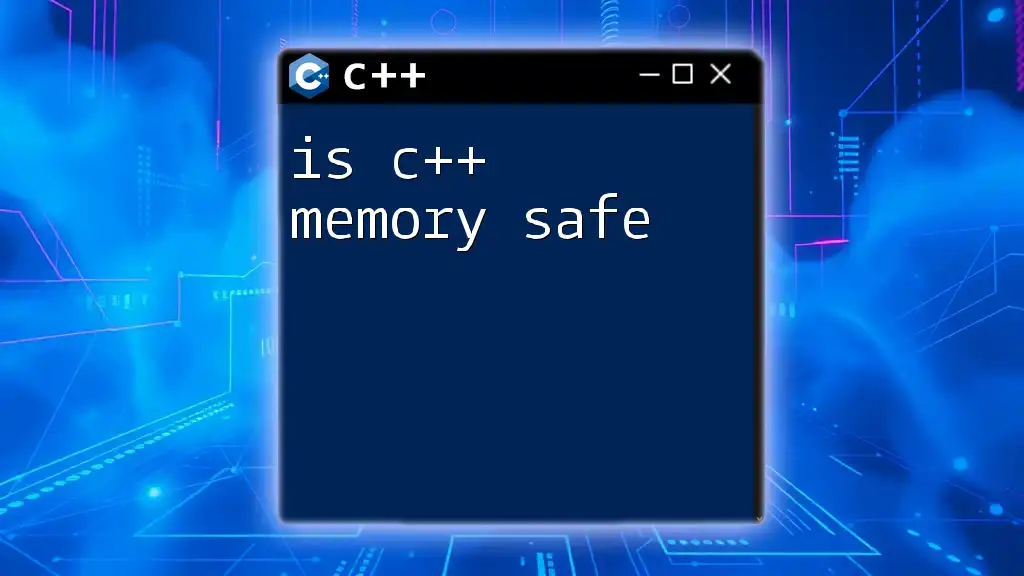
Conclusion
In summary, the question of why is C++ used for games boils down to its unparalleled performance, efficiency, robust support for object-oriented programming, flexibility, and extensive community resources. The combination of these attributes makes C++ an excellent choice for aspiring game developers. Whether you are looking to develop high-speed graphics engines or immersive game environments, mastering C++ will undoubtedly be beneficial in your journey through game development.
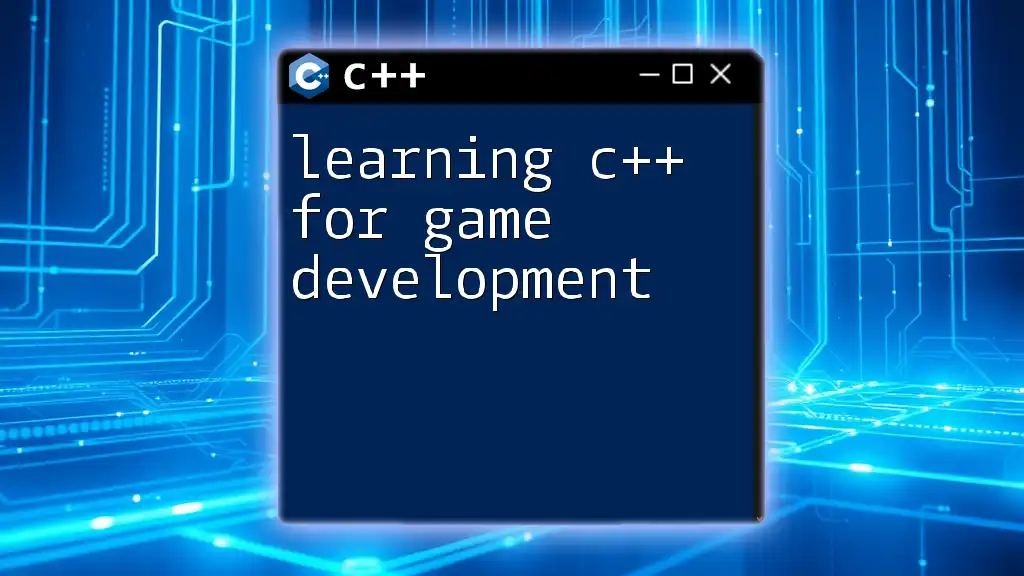
Call to Action
If you are passionate about game development and want to master C++, consider enrolling in tailored courses that focus on the essentials of C++ commands and programming practices. With the right resources and guidance, you can pave your way to becoming an expert in C++ and unleash your creativity in the gaming world.