The C++ Snake Game is a simple console-based game where the player controls a snake that grows in length as it consumes food, while avoiding walls and itself.
#include <iostream>
#include <conio.h> // For getch()
#include <windows.h> // For Sleep()
using namespace std;
bool gameOver;
const int width = 20;
const int height = 20;
int x, y, fruitX, fruitY, score;
int tailX[100], tailY[100];
int nTail;
enum eDirection { STOP = 0, LEFT, RIGHT, UP, DOWN };
eDirection dir;
void Setup() {
gameOver = false;
dir = STOP;
x = width / 2;
y = height / 2;
fruitX = rand() % width;
fruitY = rand() % height;
score = 0;
}
void Draw() {
system("cls"); // Clear console
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (j == 0)
cout << "#"; // Walls
if (i == y && j == x)
cout << "O"; // Snake head
else if (i == fruitY && j == fruitX)
cout << "*"; // Fruit
else {
bool print = false;
for (int k = 0; k < nTail; k++) {
if (tailX[k] == j && tailY[k] == i) {
cout << "o"; // Snake body
print = true;
}
}
if (!print)
cout << " ";
}
if (j == width - 1)
cout << "#";
}
cout << endl;
}
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << "\nScore:" << score << endl;
}
void Input() {
if (_kbhit()) {
switch (_getch()) {
case 'a': dir = LEFT; break;
case 'd': dir = RIGHT; break;
case 'w': dir = UP; break;
case 's': dir = DOWN; break;
case 'x': gameOver = true; break;
}
}
}
void Logic() {
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x;
tailY[0] = y;
for (int i = 1; i < nTail; i++) {
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir) {
case LEFT: x--; break;
case RIGHT: x++; break;
case UP: y--; break;
case DOWN: y++; break;
default: break;
}
if (x >= width) x = 0; else if (x < 0) x = width - 1;
if (y >= height) y = 0; else if (y < 0) y = height - 1;
for (int i = 0; i < nTail; i++)
if (tailX[i] == x && tailY[i] == y)
gameOver = true;
if (x == fruitX && y == fruitY) {
score += 10;
fruitX = rand() % width;
fruitY = rand() % height;
nTail++;
}
}
int main() {
Setup();
while (!gameOver) {
Draw();
Input();
Logic();
Sleep(10); // Small delay
}
return 0;
}
Getting Started with C++
Requirements
Before diving into code, it's essential to set up the right environment for coding your C++ Snake Game. You will need:
- A C++ compiler (such as GCC or Clang).
- An Integrated Development Environment (IDE) like Visual Studio, Code::Blocks, or CLion.
- Basic knowledge of C++ syntax and structure.
Setting Up Your Environment
- Install an IDE: Choose and install an appropriate IDE if you haven’t done so. This will simplify the coding and debugging process.
- Set Up a Compiler: Ensure your IDE is configured to use a C++ compiler.
- Create a New Project: Start a new C++ project to structure your code effectively.
Basic C++ Concepts
Before we jump into the game development, here’s a quick refresher on some core C++ concepts that will be crucial throughout the process:
- Variables: You'll use variables to store the state of the game (e.g., the snake’s length and position).
- Loops: Essential for continuously running the game loop.
- Functions: To modularize your code into reusable components.
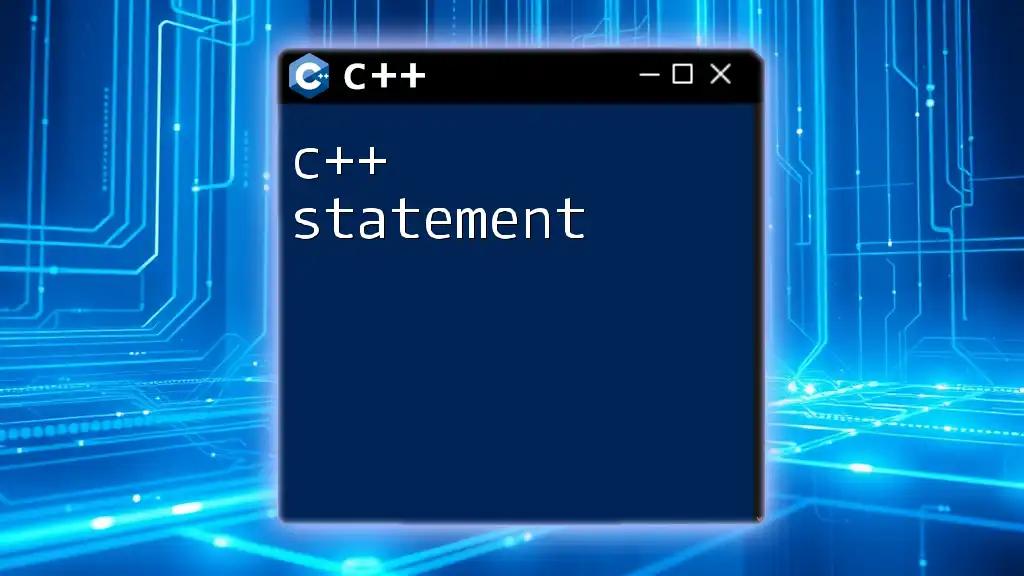
Game Design Basics
Game Mechanics
Understanding the mechanics of the C++ Snake Game is fundamental to creating an enjoyable gaming experience:
- Movement: The player controls the direction of the snake using keyboard inputs.
- Growing: Each time the snake consumes food, it grows longer.
- Collision: The game ends if the snake collides with itself or the walls of the game area.
User Interface (UI)
Your game’s user interface is vital for player interaction. Use ASCII characters to represent both the snake and the food. Here’s a simple representation:
- The snake could be represented as `#`.
- The food could be represented as `*`.
Game Loop Structure
The game loop is the core of any game, controlling the flow of the game state. A basic structure can be outlined as follows:
- Input Handling: Capture user input.
- Game Logic: Update the snake position, check for collisions, and manage the score.
- Rendering: Display the current state of the game board.

Project Setup
Creating a New Project
Start a new project in your IDE, naming it something relevant, such as "SnakeGame". This will serve as the framework for your code.
Organizing Your Code
Organizing your code into separate files for different components (like game logic, the snake, and the food) will help maintain clarity and promote reusability.
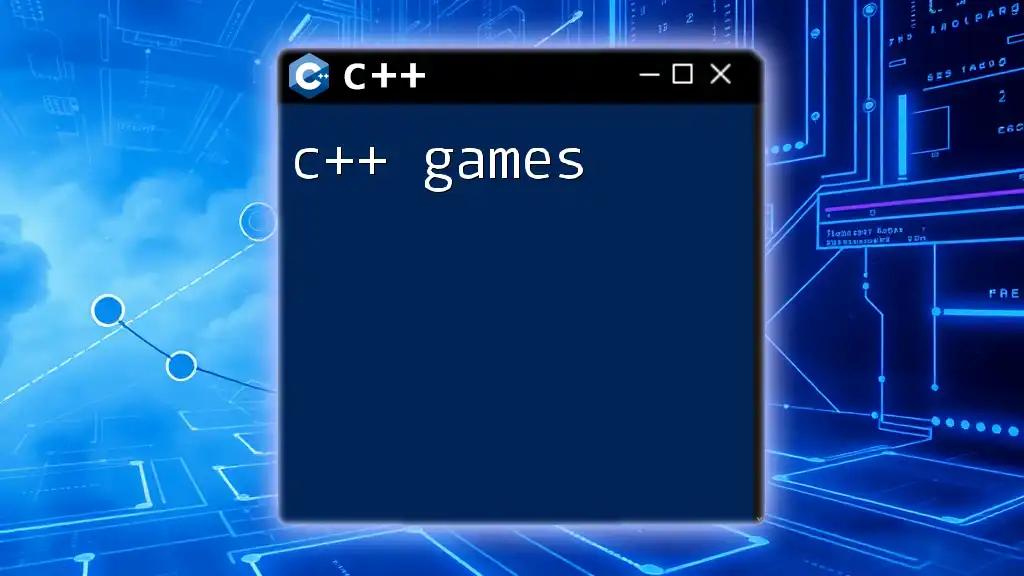
Implementing the Snake Game
Defining the Snake
Data Structures Overview
The snake can be represented using arrays or STL containers (like `std::vector`). For simplicity, we can use a vector to store the coordinates of the snake segments.
Creating the Snake Class
Here’s how to define a basic Snake class:
#include <vector>
class Snake {
public:
std::vector<std::pair<int, int>> body;
int direction; // 0: Up, 1: Right, 2: Down, 3: Left
Snake() {
// Initialize the snake at a specified starting position
body.push_back({5, 5});
direction = 1; // Start moving right
}
void move() {
// Add movement logic here
}
};
Game Board and Food
Designing the Game Board
Configure a simple text-based game board, perhaps using an 80x20 grid. A function can be developed to visualize it:
void drawBoard(const Snake& snake) {
system("clear"); // Use "cls" on Windows
for (int y = 0; y < 20; ++y) {
for (int x = 0; x < 80; ++x) {
if (std::find(snake.body.begin(), snake.body.end(), std::make_pair(x, y)) != snake.body.end()) {
std::cout << "#";
} else {
std::cout << ".";
}
}
std::cout << std::endl;
}
}
Food Generation
Randomly generate food on the board. Here’s a snippet that illustrates how to do this:
std::pair<int, int> generateFood() {
int x = rand() % 80;
int y = rand() % 20;
return {x, y};
}
Movement Mechanics
Handling User Input
To control the snake's movement, capture keyboard input. You could use libraries like `ncurses` for handling input without requiring the Enter key. Here's a pseudo-code example:
char input;
std::cin >> input;
if (input == 'w') direction = 0; // Up
if (input == 'd') direction = 1; // Right
if (input == 's') direction = 2; // Down
if (input == 'a') direction = 3; // Left
Updating Snake Position
Update the snake's position based on its current direction:
void Snake::move() {
std::pair<int, int> newHead = body.front();
switch (direction) {
case 0: newHead.second--; break; // Move up
case 1: newHead.first++; break; // Move right
case 2: newHead.second++; break; // Move down
case 3: newHead.first--; break; // Move left
}
body.insert(body.begin(), newHead);
// Logic for removing the tail or growing the snake
}
Game Logic
Collision Detection
Implement collision detection logic to check if the snake has collided with the walls or itself.
bool checkCollision() {
auto head = body.front();
return head.first < 0 || head.first >= 80 || head.second < 0 || head.second >= 20 ||
std::find(body.begin() + 1, body.end(), head) != body.end(); // Check self-collision
}
Growing the Snake
Increase the length of the snake when it consumes food. This could be done by modifying the `move` function to not remove the tail if the snake has just consumed food.
Scoring System
Implementing Score Tracking
Create a simple score variable to keep track of how many pieces of food the snake has eaten.
int score = 0;
// Increment score when food is eaten
if (head == foodPosition) {
score++;
// Generate new food
}
Displaying the Score
Update your draw function to include the player score:
void drawBoard(const Snake& snake, int score) {
// Existing drawing logic...
std::cout << "Score: " << score << std::endl;
}
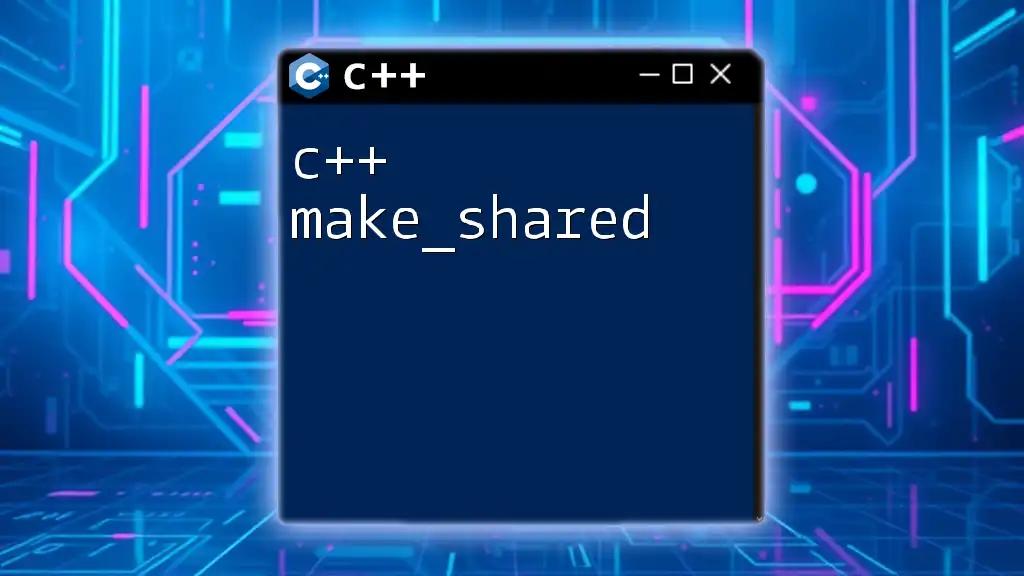
Enhancing Your Game
Adding Levels
Introduce levels by increasing the speed of the game or the snake's length with each level achieved.
Sound Effects and Music
For a better user experience, consider adding simple sound effects when the snake eats food, and background music when the game starts. Libraries like SDL can be helpful.
Using Graphics Libraries
To move beyond the terminal interface, utilize graphics libraries like SFML or SDL, which will enable you to draw shapes, move sprites, and capture input more intuitively.

Conclusion
Throughout this guide, we've covered the essential components required to create a C++ Snake Game: setting up the environment, implementing the game mechanics, and enhancing your game for additional fun. By following these guidelines, you've not just learned a fundamental programming concept but also gained practical experience that extends into the realm of game development.

Additional Resources
For further learning, check out books on C++ programming and game development, or online courses that delve into advanced topics. Engaging in communities and forums can also be extremely beneficial where you can ask questions and share your projects.

Code Repository
For a complete implementation of the C++ Snake Game, consider visiting a code repository that contains the entire source code. This will provide a tangible resource for you to study and modify.