A C++ statement is a single line of code that performs a specific action or completes a task in a program.
Here's an example of a simple C++ statement that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // This is a C++ statement
return 0;
}
What is a C++ Statement?
Definition of a Statement
A C++ statement is a single line of code that performs a specific action. Statements are the foundational building blocks of C++ programming as they express the logic and behavior of a program. Each statement must end with a semicolon (`;`), indicating the termination of that particular line. Understanding statements is crucial for writing effective and efficient C++ code.
Types of Statements in C++
C++ supports various types of statements, each serving a unique purpose. The major categories include:
- Expression Statements: Used for executing expressions.
- Declaration Statements: For declaring variables or functions.
- Control Flow Statements: Direct the flow of execution based on conditions or loops.
- Jump Statements: Alter the control flow in loops and functions.
- Exception Handling Statements: Manage errors in a controlled manner.
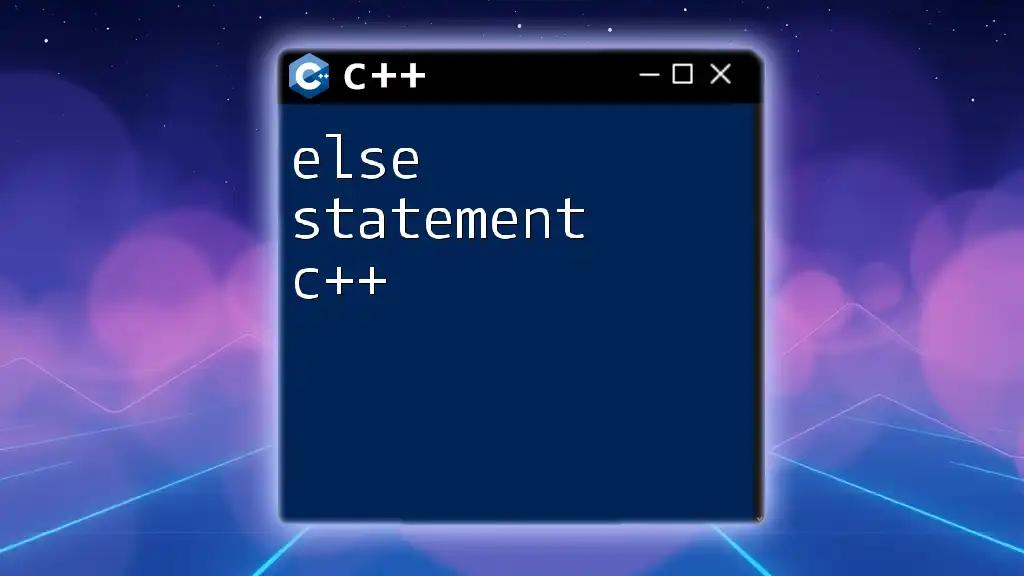
Basic Statements
Expression Statements
Expression statements are those that evaluate an expression. They can include variable declarations, assignments, and function calls. The result of an expression can be used or ignored based on the context.
Example:
int a = 5; // variable declaration
a = a + 10; // assignment expression
In this example, `int a = 5;` declares an integer variable `a` and initializes it with the value 5. The subsequent line modifies `a` by adding 10 to its current value.
Declaration Statements
Declaration statements are crucial for informing the compiler about the existence of a variable or function. They define the type and the name.
Example:
int age; // declaring an integer variable
void functionName(); // declaring a function
Here, `int age;` indicates that `age` will hold an integer, while `void functionName();` declares a function with no parameters or return value, allowing its definition to occur later in the code.
Compound Statements
A compound statement is a group of statements enclosed in braces (`{}`). These blocks are essential when multiple statements need to be executed together under a single control structure, such as loops or conditionals.
Example:
{
int x = 10;
x += 5; // compound statement
}
In this example, both declarations and expressions are encapsulated within braces, allowing them to be treated as a single statement.
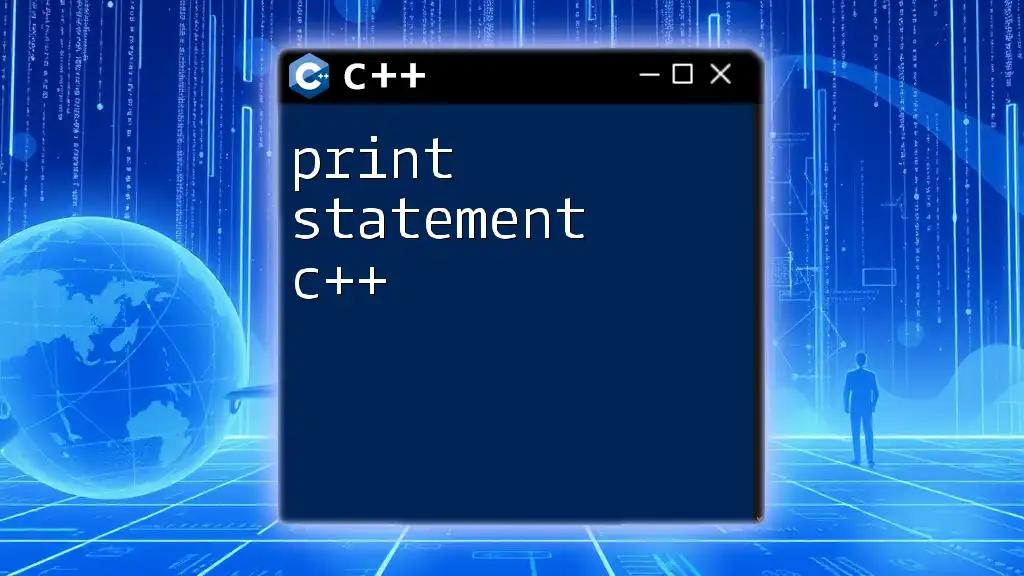
Control Flow Statements
Conditional Statements
Conditional statements allow the program to make decisions based on certain conditions.
if Statements
The most fundamental conditional statement is the `if` statement, which executes a block of code only if a specified condition evaluates to true.
Example:
if (a > 10) {
cout << "a is greater than 10";
}
In this instance, if `a` is indeed greater than 10, the message prints.
switch Statements
The `switch` statement offers a more efficient way to handle multiple conditional branches compared to several if-else statements.
Example:
switch (day) {
case 1: cout << "Monday"; break;
case 2: cout << "Tuesday"; break;
// more cases...
}
Here, the value of `day` determines which case block executes, based on predefined integer values. Each `break` statement exits the switch block after executing the corresponding case.
Looping Statements
Looping statements facilitate the execution of a block of code repeatedly, based on a condition.
for Loops
A `for` loop consists of three parts: initialization, condition, and iteration. It's a compact way to manage repetitive tasks.
Example:
for (int i = 0; i < 5; i++) {
cout << i;
}
This loop starts with `i` initialized to 0 and iterates until `i` is no longer less than 5, printing the value of `i` with each iteration.
while Loops
The `while` loop continues executing as long as the specified condition holds true. It's flexible when the number of iterations is uncertain beforehand.
Example:
int i = 0;
while (i < 5) {
cout << i;
i++;
}
In this scenario, the loop continues until `i` reaches 5, incrementing `i` on each iteration.
do-while Loops
A `do-while` loop ensures that the block of code executes at least once, as the condition is checked after the code execution.
Example:
int j = 0;
do {
cout << j;
j++;
} while (j < 5);
This loop behaves similarly to the `while` loop but guarantees at least one output of `j`, even if the condition is initially false.
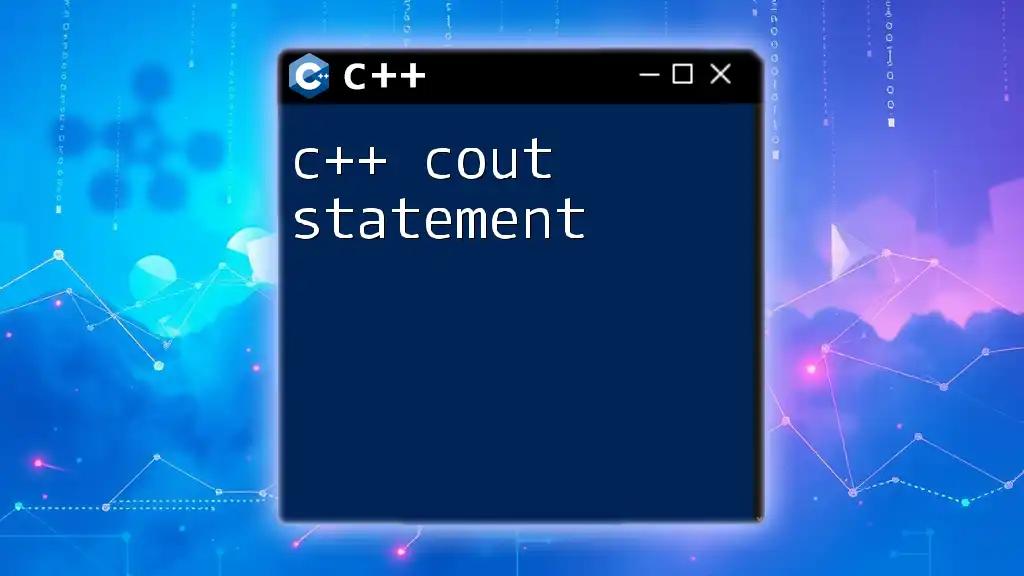
Jump Statements
break Statement
The `break` statement is used to exit a loop prematurely. It is particularly useful for terminating a loop based on some conditions.
Example:
for (int k = 0; k < 10; k++) {
if (k == 5) break; // exit loop when k is 5
}
In this example, the loop will stop when `k` equals 5, bypassing all subsequent iterations.
continue Statement
The `continue` statement is used to skip the current iteration of a loop and move to the next iteration.
Example:
for (int l = 0; l < 10; l++) {
if (l % 2 == 0) continue; // skip even numbers
cout << l;
}
This code will output only the odd numbers between 0 and 9, as it skips the even numbers.
return Statement
The `return` statement is essential in functions, signaling the end of function execution and optionally sending a value back to the calling function.
Example:
double add(double x, double y) {
return x + y; // return the sum
}
Here, the `add` function takes two parameters and returns their sum to the caller.
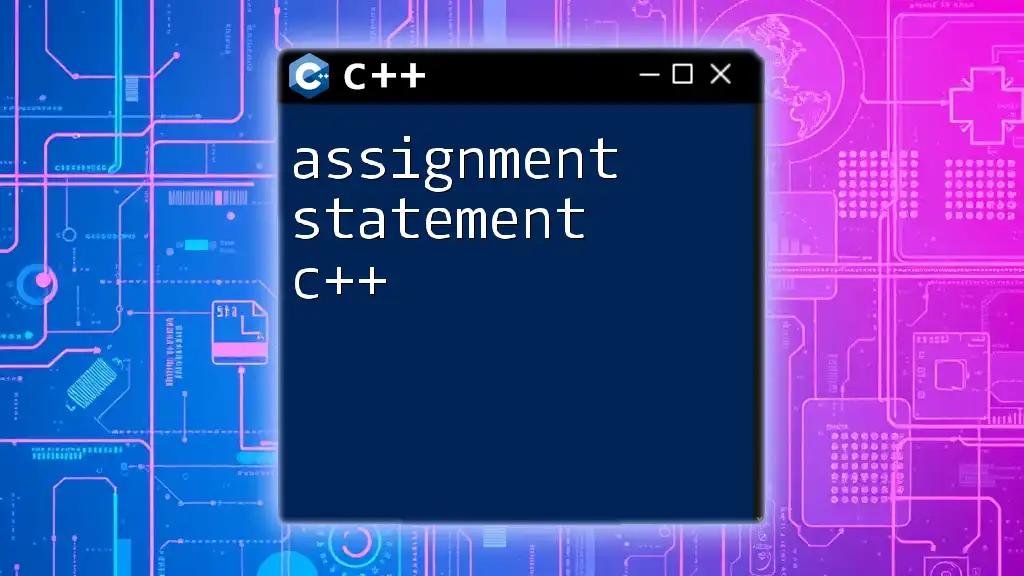
Exception Handling Statements
try, catch, throw
Exception handling statements enable developers to manage errors gracefully and provide a way to recover from them.
Overview: The `try` block is where code that may throw an exception is placed. The `catch` block handles the thrown exception, allowing for error management.
Example:
try {
throw 20; // throwing an exception
}
catch (int e) {
cout << "Caught exception: " << e;
}
In this example, the program throws an exception with the value 20, which is caught in the catch block and printed to the console, avoiding any program crashes.
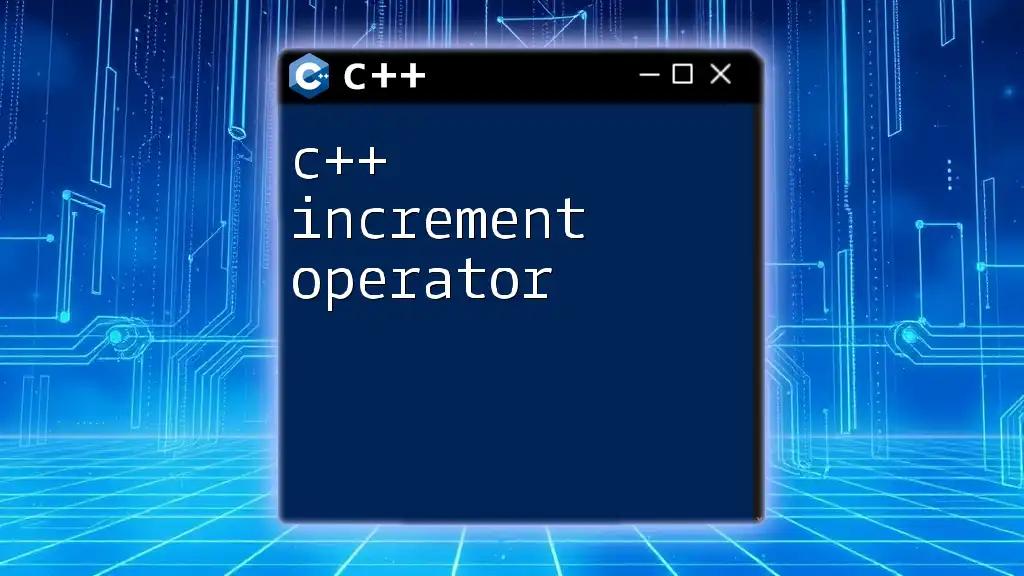
C++ Statements Best Practices
Structuring Your Code
For clarity and maintainability, it’s important to structure your code well. Use proper indentation, thoughtful naming conventions, and comments where necessary. These best practices enhance readability and allow other developers (and your future self) to understand the logic quickly.
Avoiding Common Mistakes
Frequent mistakes include missing semicolons, improper block scoping, and incorrect condition checks. To avoid pitfalls:
- Always end statements with a semicolon.
- Be cautious with the scope of your variables, particularly within conditional and loop constructs.
- Validate conditions with thorough testing to ensure expected flow.
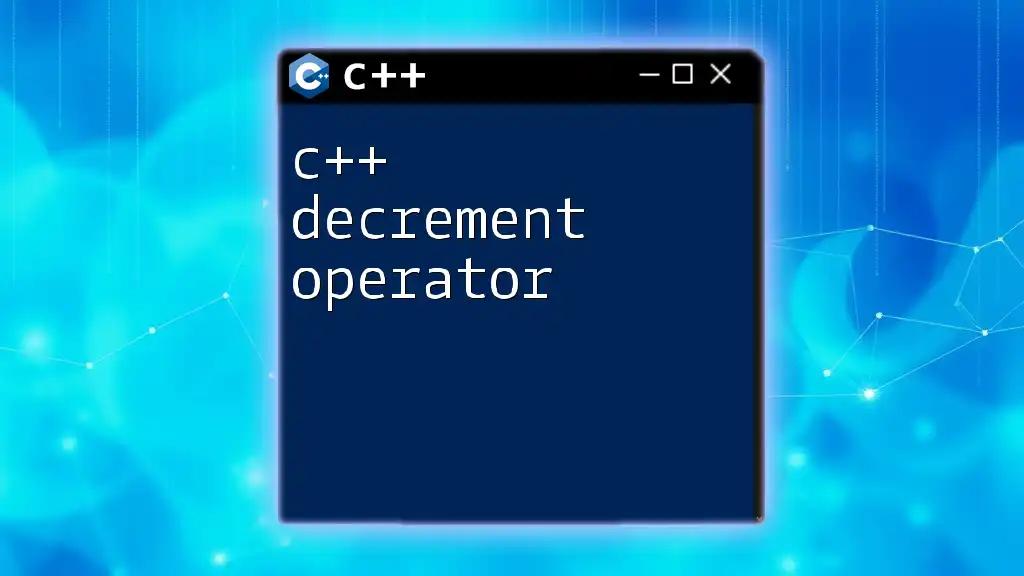
Conclusion
In summary, understanding C++ statements is fundamental to effective programming. They encompass a variety of forms, including expression, declaration, control flow, jump, and exception handling statements. Mastery of these concepts, alongside adherence to best practices, will significantly enhance your coding quality and efficiency.
Encouragement to Practice
To truly grasp C++ statements, hands-on practice is invaluable. Engaging with exercises that challenge your understanding will solidify your skills and prepare you for more complex programming tasks in the future. There are numerous resources and platforms available online to provide additional exercises and insights into C++.
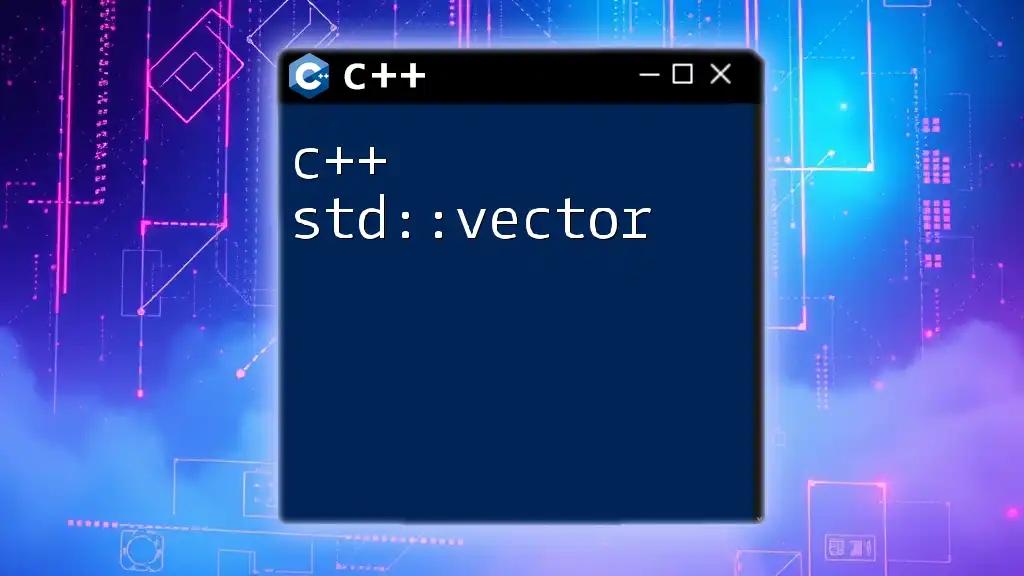
References and Further Reading
For those eager to deepen their understanding of C++ statements, consider exploring reputable sources, books, and online tutorials. These materials provide structured lessons and practical examples, allowing for a comprehensive grasp of C++ programming principles and advanced techniques.