The `std::min` function in C++ returns the smaller of two values, providing a simple way to determine the minimum between them.
#include <iostream>
#include <algorithm> // To include std::min
int main() {
int a = 5, b = 10;
int minimum = std::min(a, b);
std::cout << "The minimum value is: " << minimum << std::endl; // Output: The minimum value is: 5
return 0;
}
Understanding std::min
What is std::min?
The `std::min` function is a fundamental component of the C++ Standard Library, primarily designed to return the smallest of two provided values. Its simplicity belies its utility, as it can be used in a variety of contexts, from simple comparisons of numerical values to more complex data types.
How std::min Works
`std::min` accepts two parameters, typically of the same type, and returns the value of the smaller of the two. The function is essential for making comparisons simpler and more readable in your code. Specifically, it offers multiple overloads and can work with different data types, enhancing its versatility.
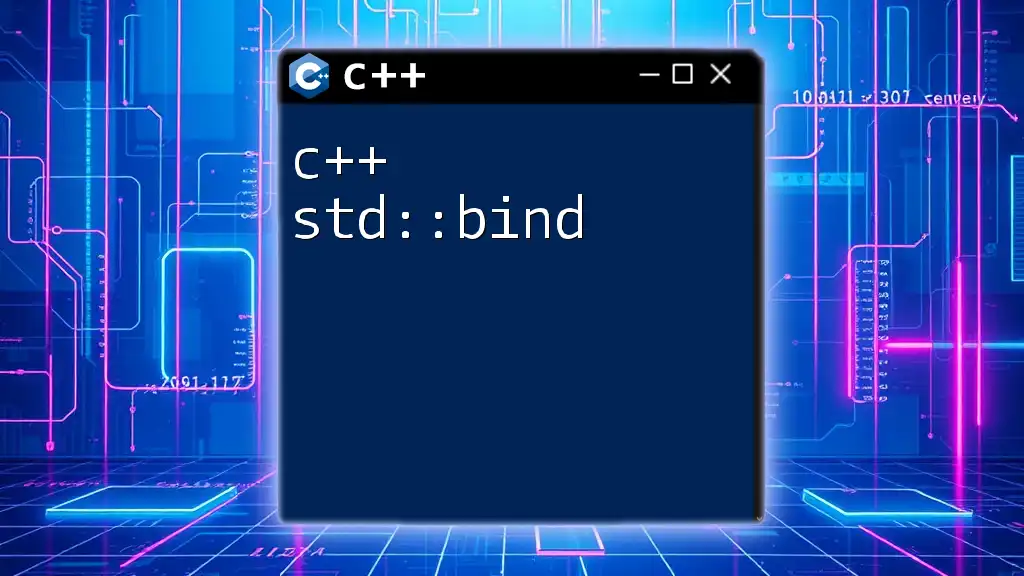
Syntax of std::min
Standard Syntax
The general syntax of the `std::min` function is as follows:
std::min(value1, value2);
This function requires the inclusion of the `<algorithm>` header for proper usage, which is a standard practice when utilizing functions from the C++ Standard Library.
Using std::min with Different Data Types
Integer Types
For basic integer comparisons, `std::min` is straightforward and effective. The following example demonstrates how to find the minimum of two integers:
#include <iostream>
#include <algorithm>
int main() {
int a = 10, b = 20;
std::cout << "The minimum is: " << std::min(a, b) << std::endl; // Output: 10
return 0;
}
In this example, the function evaluates the two integers, `a` and `b`, and returns `10`, clearly indicating that it is the smaller value.
Floating-Point Numbers
`std::min` can also be utilized with floating-point numbers. It behaves the same way as with integers:
float x = 2.5f, y = 3.6f;
std::cout << "The minimum of x and y is: " << std::min(x, y) << std::endl; // Output: 2.5
Here, the function compares the two float values and returns `2.5`, demonstrating its effectiveness across different data types.
Custom Data Types
One of the more powerful features of `std::min` is its compatibility with user-defined types. Here’s an example using a custom struct called `Point`:
struct Point {
int x, y;
bool operator<(const Point& p) const {
return (x < p.x && y < p.y);
}
};
Point p1{1, 2}, p2{3, 4};
Point minimum = std::min(p1, p2);
In this case, we define a comparison operator for the `Point` struct, allowing for a direct comparison using `std::min`.
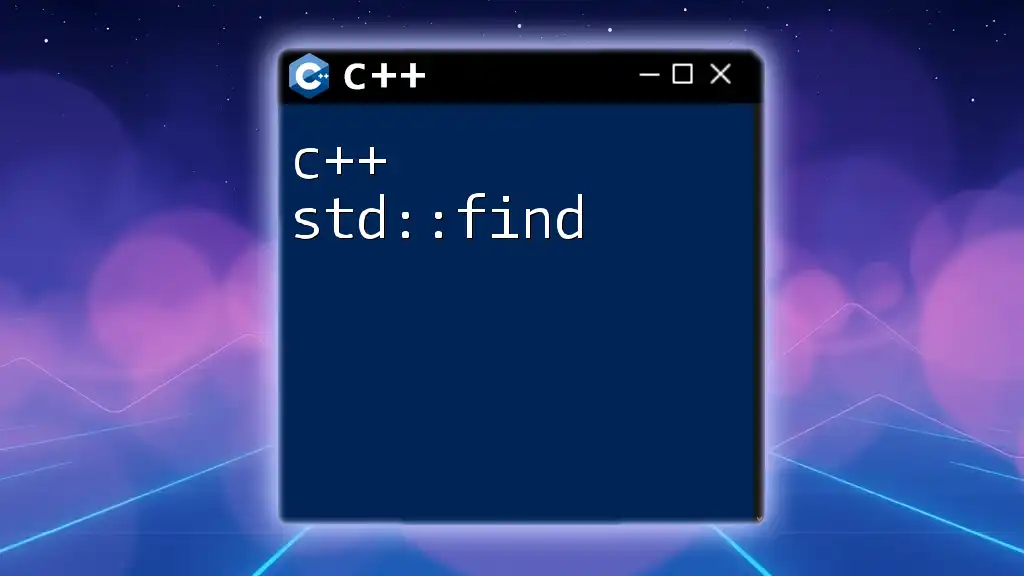
Important Features of std::min
Overloading std::min
The versatility of `std::min` is enhanced by its overloaded functions, which allow it to work seamlessly with various types and combinations of parameters. Understanding the overloads helps you to choose the right one based on your specific needs.
Using std::min with Containers
You can also utilize `std::min` in conjunction with C++ containers like vectors. To find the minimum value within a vector, the use of `std::min_element` is recommended:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> vec = {5, 3, 9, 1};
auto minVal = *std::min_element(vec.begin(), vec.end());
std::cout << "Minimum in the vector: " << minVal << std::endl; // Output: 1
return 0;
}
This example utilizes `std::min_element`, which allows for an efficient way to determine the smallest element in a collection of values.
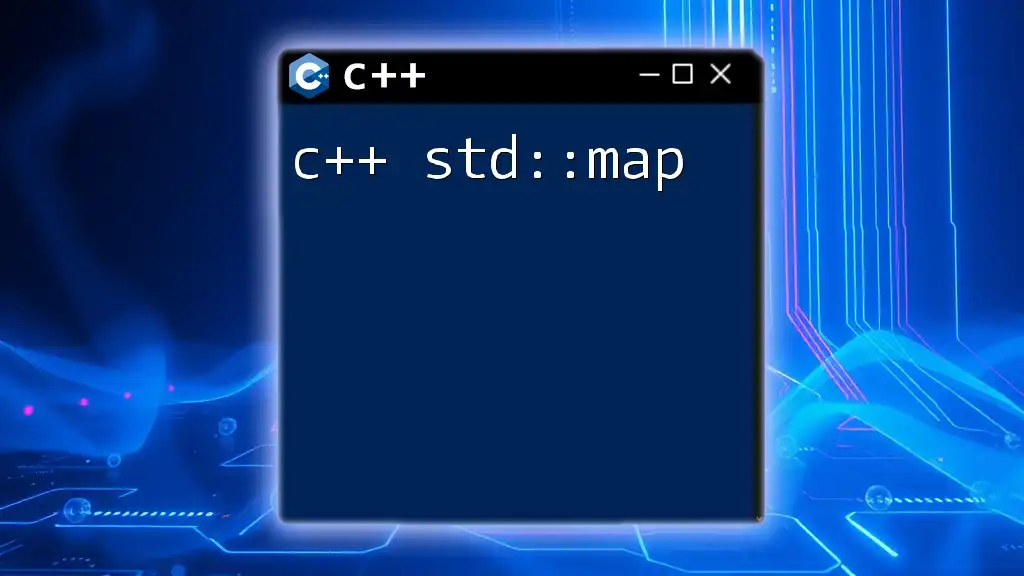
Differences Between std::min and Other Functions
Comparing std::min with std::max
`std::min` operates in tandem with `std::max`, which returns the largest of the two input values. The main difference lies in their functionality—one finds the minimum while the other identifies the maximum. Choosing which function to use depends entirely on the task at hand; you can leverage `std::min` when you need the smaller of two values and `std::max` when you need the larger.
std::min vs. Manual Comparison
Using `std::min` can significantly simplify your code compared to manual comparisons. Consider the manual approach:
int a = 5, b = 10;
int minimum;
if (a < b) {
minimum = a;
} else {
minimum = b;
}
While effective, this is more verbose and less clear than simply using:
int minimum = std::min(a, b);
The latter is not only cleaner but also reduces the potential for errors in your code.
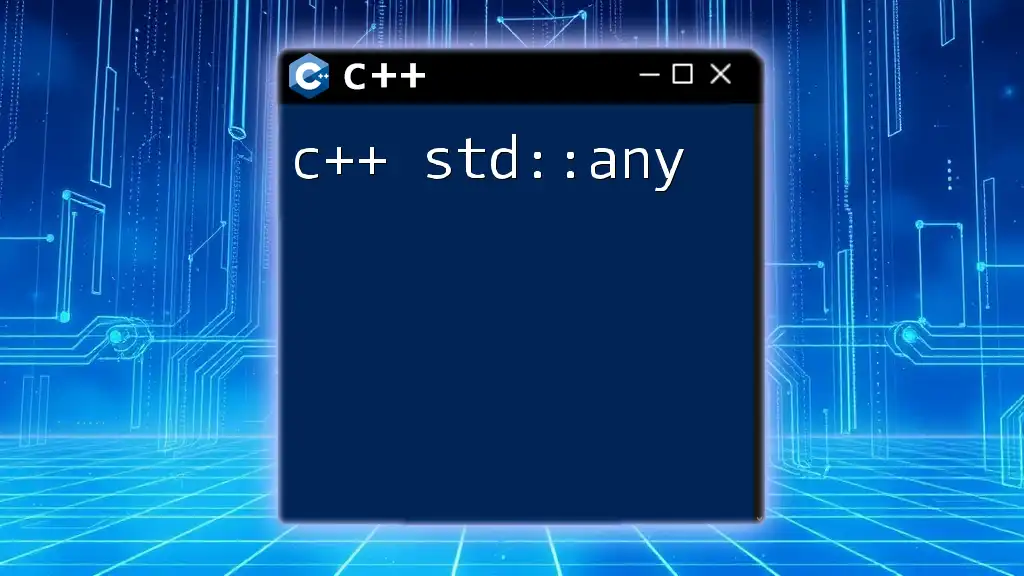
Error Handling and Edge Cases
Handling Equal Values
When both values passed to `std::min` are equal, the function returns that value:
int p = 5, q = 5;
std::cout << "Minimum is: " << std::min(p, q) << std::endl; // Output: 5
Understanding this behavior is crucial, especially in cases where you might expect an explicit indication of the lesser value.
Dealing with Special Data Types
With pointers or complex data types such as strings, it's important to consider how `std::min` will behave. For instance, comparing pointers requires caution, as the comparison will be based on the addresses rather than the values they point to. Always ensure that the types you compare are aligned, or C++ will generate compilation errors or logical missteps.
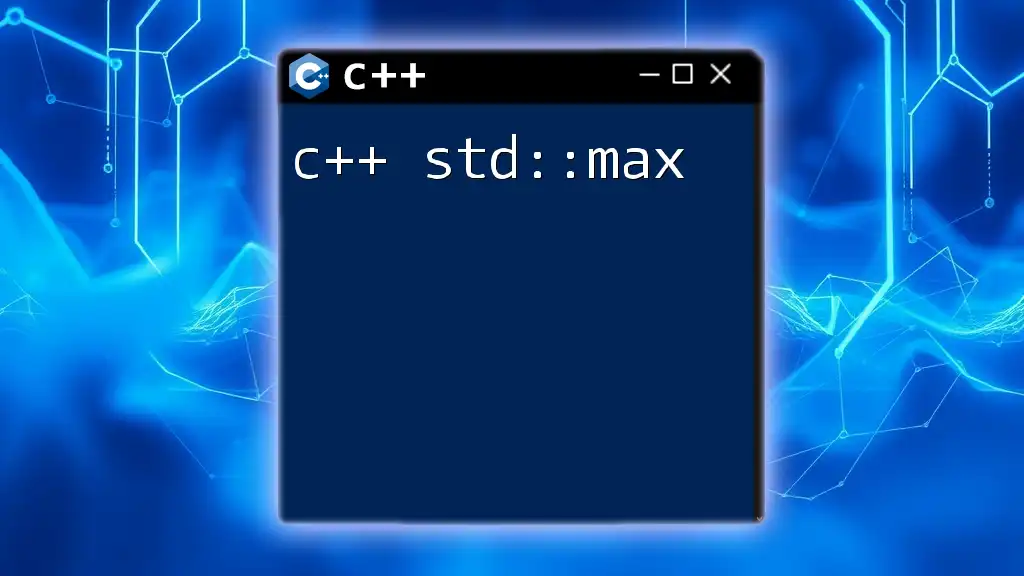
Performance Considerations
There are minimal performance implications when using `std::min`, as its underlying implementation is generally optimized. However, using `std::min` in cases where performance-critical code requires extreme efficiency may warrant manual comparisons for highly specialized applications. Always consider the context of your use case and ensure your choice aligns with your performance goals.
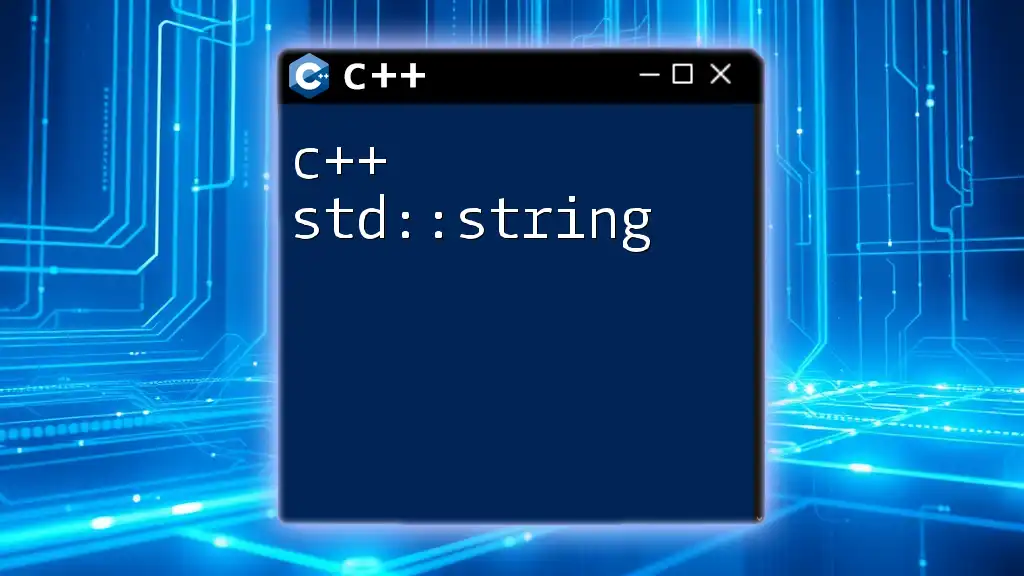
Conclusion
The `c++ std::min` function stands as a unique and versatile tool in the C++ Standard Library. Its ability to streamline comparisons across various data types makes it invaluable for any C++ programmer. As you venture deeper into the language, the benefits of utilizing the Standard Library will become increasingly apparent. If you're eager to elevate your C++ skills even further, consider joining our company's offerings for concise and dedicated learning experiences!
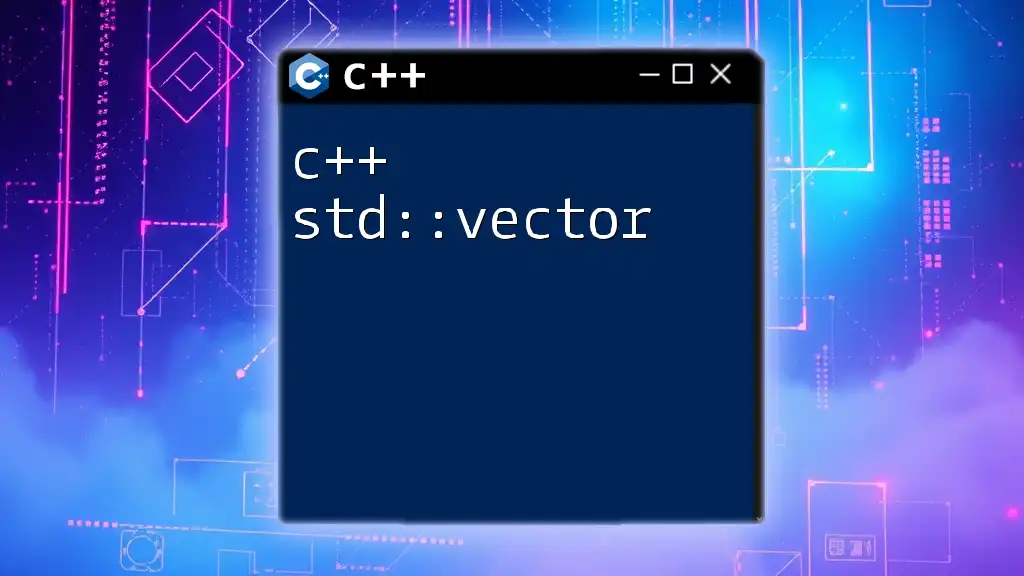
Additional Resources
Recommended Reading and References
To deepen your understanding of C++ and the Standard Library, consider exploring various books, articles, and online courses tailored to the language.
Join Us to Learn More!
We provide courses on a wide range of topics in C++, enabling you to master command-line commands in quick, concise formats perfect for every learning style.