In C++, `stdin` is a standard input stream used to read input from the user or files, typically utilized with functions like `cin` in the input/output library.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number; // Reading input from stdin
cout << "You entered: " << number << endl;
return 0;
}
What is stdin?
stdin, or standard input, is an essential concept in C++ programming that allows developers to receive input directly from the user or another program. Understanding how stdin functions not only helps you interact with users but also enhances your ability to create dynamic applications where input is fundamental. Common use cases of stdin include collecting user data, receiving commands, and facilitating real-time program interaction.
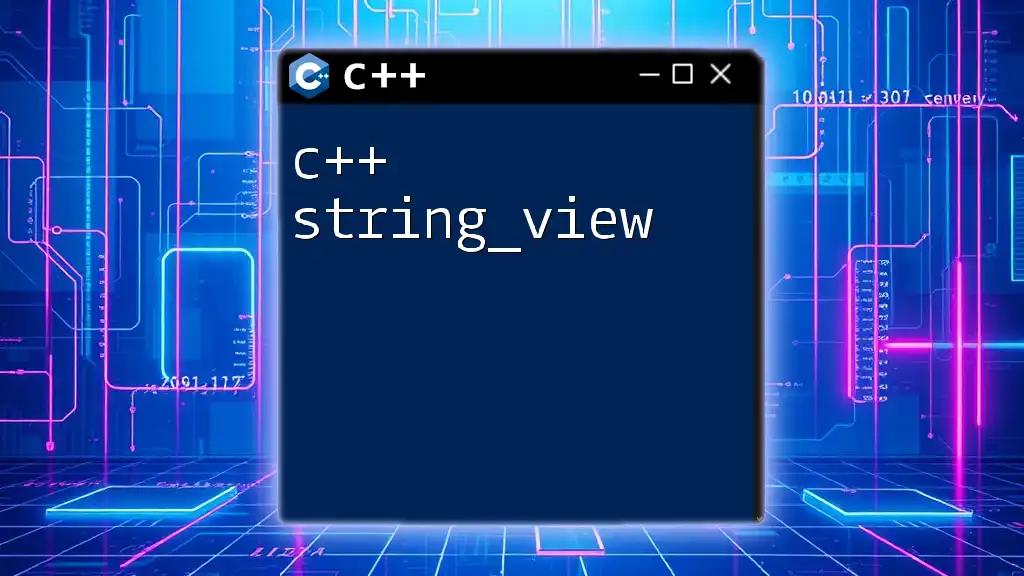
Understanding Standard Input
The Role of stdin in C++ Programs
In C++, standard input is managed through three primary streams: stdin, stdout (standard output), and stderr (standard error). stdin is specifically designed for input operations. When you use it, you expect data to be delivered to your program, typically from the keyboard or file redirection.
Differences Between stdin and Other Input Mechanisms
While stdin is widely used, it is crucial to differentiate it from other input methods, such as command-line arguments. Command-line arguments allow users to pass parameters to the program at execution time, whereas stdin can be utilized for ongoing input during a program's execution.
When to use stdin over command-line arguments depends on your application requirements. For example, if you need to continuously ask for user input, stdin is the clearer choice. In contrast, if your program only requires specific parameters at startup, command-line arguments may be sufficient.
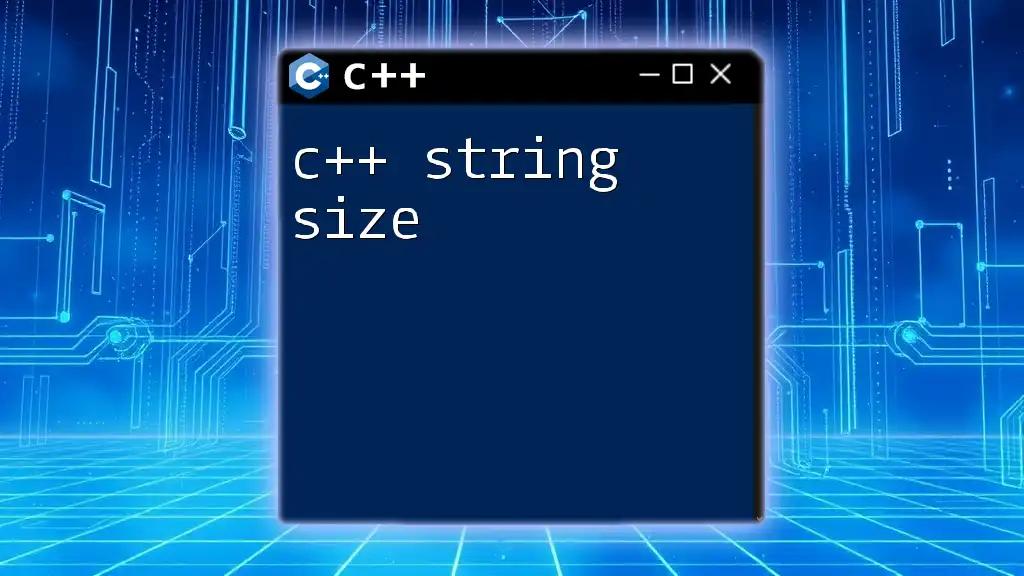
Using stdin in C++
Setting Up to Read from stdin
Before you can read from stdin in a C++ program, ensure you include the appropriate libraries. The `<iostream>` library handles stream operations and is essential for using stdin.
#include <iostream>
Basic Reading from stdin
The `std::cin` object is your primary tool for input through stdin. It allows users to input variables interactively. Here's a basic code snippet demonstrating how to use `std::cin` to collect a user's age.
#include <iostream>
int main() {
int age;
std::cout << "Enter your age: ";
std::cin >> age;
std::cout << "You are " << age << " years old." << std::endl;
return 0;
}
In this example, the program prompts the user for their age. The input is stored in the variable `age`, and the program then confirms the information back to the user.
Handling Different Data Types
C++ allows you to read various data types from stdin, including integers, floats, and strings. Here’s how you can handle each:
- Integer Input:
int num;
std::cin >> num;
- Float Input:
float decimal;
std::cin >> decimal;
- String Input:
std::string name;
std::cin >> name;
It is imperative to remember that while reading input, you must ensure type safety. If a user inputs a non-integer value while expecting an integer, the program will produce unexpected behavior.

Advanced stdin Techniques
Using std::getline for Strings
While `std::cin` works well for input, std::getline is preferred when reading strings, especially if the input might contain spaces. Here's when and how to use std::getline:
std::string fullName;
std::cout << "Enter your full name: ";
std::getline(std::cin, fullName);
Using `std::getline` allows you to capture the entire line of input, including spaces, ensuring that the entire name is read correctly.
Error Checking with stdin
Input validation is critical to ensuring your application runs smoothly without crashing. It is a best practice to implement error checking for stdin:
int choice;
while (true) {
std::cout << "Enter a valid number: ";
if (std::cin >> choice) {
break;
}
std::cin.clear(); // clear the error flag that prevents further input
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
}
This code will repeatedly prompt the user until they enter a valid integer, clearing any input errors along the way.
Redirecting stdin
You can redirect stdin to read from a file, which is particularly useful for testing purposes or when batch input is needed. For example, if you want to read from a file named "data.txt", you can set up your code like this:
#include <fstream>
std::ifstream inputFile("data.txt");
int number;
while (inputFile >> number) {
std::cout << number << std::endl;
}
This method can significantly simplify data handling in your applications.
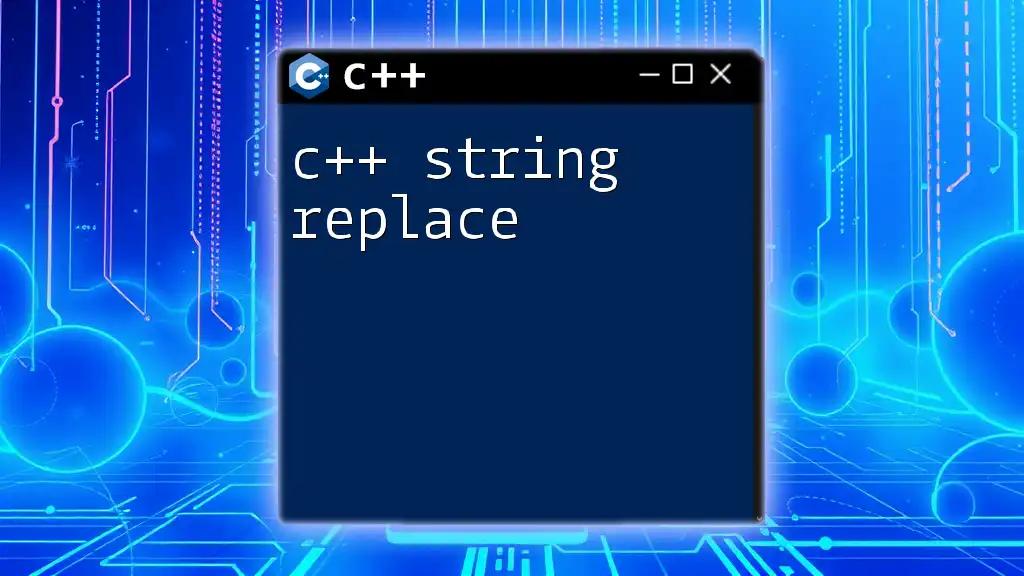
Common Pitfalls with stdin
Common Mistakes When Using stdin
One of the most frequent mistakes developers make with stdin is forgetting to clear the input buffer. If your program handles mixed inputs, it is crucial to manage the input stream carefully. Additionally, beware of mixing std::cin and std::getline; this can create seemingly inexplicable issues, especially when `std::cin` leaves a newline character in the buffer.
Best Practices
- Always Validate User Input: Never assume that user input will be in the correct format. Input validation ensures robustness.
- Use Clear and Informative Prompts: Clear prompts help prevent confusion and improve the user experience.

Conclusion
Understanding and effectively using c++ stdin is essential for interactive program development. Mastering its nuances, from basic input handling to advanced techniques like error checking and file redirection, prepares you for creating dynamic and responsive applications. Always remember to practice these techniques, as hands-on experience enhances comprehension and retention.
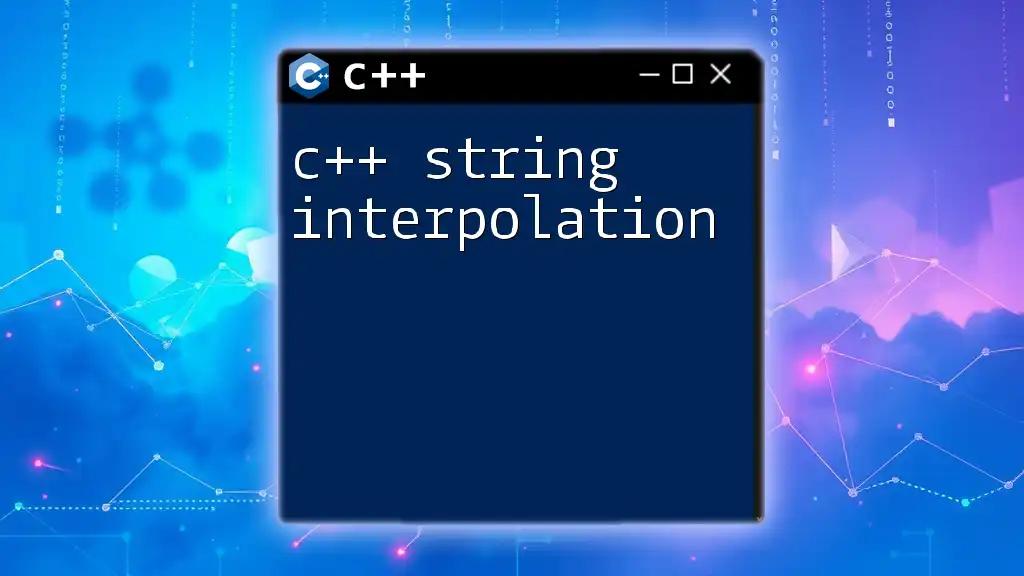
Additional Resources
To further your knowledge about c++ stdin and input handling in C++, consider exploring the following resources:
- Books on C++ programming fundamentals
- Online platforms offering coding tutorials and challenges
- Forums and community discussions on best practices

Call to Action
Join our community of learners today! Share your insights, ask questions, and collaborate with fellow enthusiasts as you dive deeper into the world of C++ programming.