A C++ string pointer is a variable that holds the memory address of a string object, allowing direct manipulation of the string's contents.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string* strPtr = &str; // Pointer to the string
std::cout << *strPtr << std::endl; // Dereference the pointer to access the string
return 0;
}
The Anatomy of a C++ String Pointer
Defining String Pointers
In C++, a string pointer is a variable that holds the memory address of a string. This can be either a pointer to a C-style string or a pointer to a C++ `std::string` object. The syntax for defining a pointer to a C-style string is straightforward. For instance, you can initialize a string pointer as follows:
char* strPtr = "Hello, World!";
In this example, `strPtr` points to the string literal `"Hello, World!"`. It's important to remember that string literals are stored in read-only memory, which means anything attempting to modify them can lead to undefined behavior. To define a mutable string, you can allocate memory dynamically:
char* dynamicStr = new char[20]; // Allocate memory for 20 characters
Memory Allocation
There are two primary ways to allocate memory for strings in C++: static and dynamic allocation. Static allocation occurs at compile time, while dynamic allocation is performed during program execution.
Dynamic memory allocation allows greater flexibility, especially if the size of the string is not known at compile time. For example, using `new` allocates memory for the specified number of characters, which can be particularly useful when handling user input or data retrieved from a database.
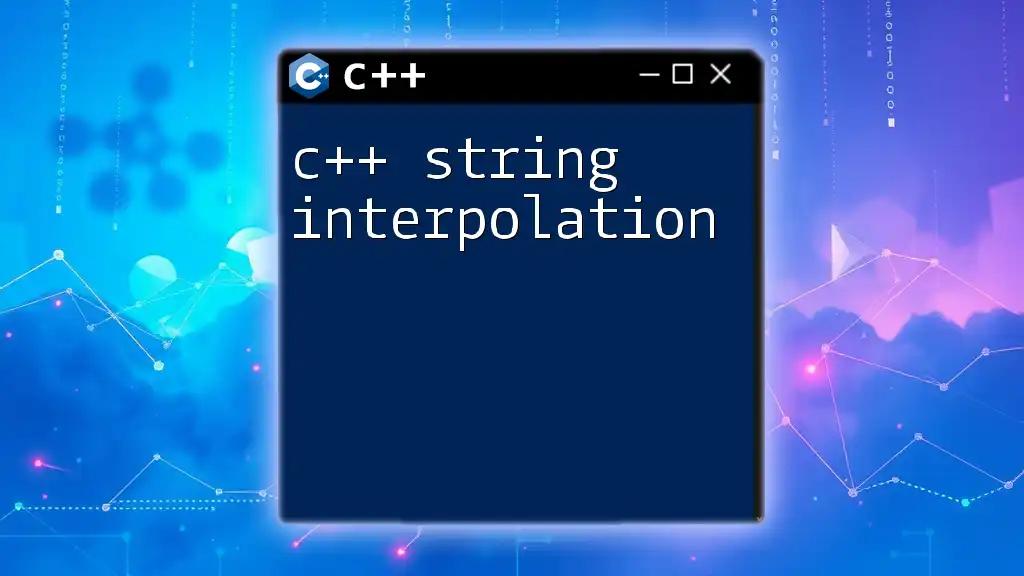
Working with String Pointers in C++
Accessing String Data
One of the primary uses of pointers in relation to strings is to access their data. You can easily dereference a string pointer to read its value. Here’s how you can print the string pointed to by `strPtr`:
std::cout << strPtr << std::endl; // Prints: Hello, World!
When using a pointer, you can also manipulate the characters directly, provided that the string is mutable.
Modifying Strings through Pointers
String pointers allow you to modify the contents of a string by manipulating the memory they point to. To change a character in a dynamically allocated string, you can do something like this:
dynamicStr[0] = 'H'; // Changing the first character
This directly modifies the content of `dynamicStr` without needing to create a copy.

Common Operations with C++ String Pointers
String Length Calculation
Knowing the length of a string is often important for various string manipulations. You can obtain the length of a C-style string using the `strlen()` function:
int length = strlen(strPtr); // Retrieves the length of the string
This function counts the number of characters until it encounters a null terminator (`\0`).
Concatenating Strings
Combining or concatenating strings is another common operation. Using the `strcat()` function, you can concatenate two strings together. Here’s a complete example that demonstrates this:
char destination[50] = "Hello, ";
char source[] = "World!";
strcat(destination, source); // Appends source to destination
std::cout << destination << std::endl; // Prints: Hello, World!
In this code snippet, `destination` is an array where the `source` string gets appended.

String Pointer Best Practices
Memory Management
One of the most critical aspects of working with C++ string pointers is proper memory management. When you allocate memory using `new`, it’s essential to free that memory once you're done with it. To do this, you can use the `delete[]` operator:
delete[] dynamicStr; // Free the allocated memory
This practice helps prevent memory leaks, which can lead to performance degradation over time.
Smart Pointers
To streamline memory management and reduce the risk of memory leaks, C++11 introduced smart pointers, such as `std::unique_ptr` and `std::shared_ptr`. These pointers automatically manage memory for you. For instance, using `std::unique_ptr`, you can simplify memory handling like this:
#include <memory>
std::unique_ptr<char[]> smartStr(new char[20]); // Unique pointer manages the memory
When `smartStr` goes out of scope, it will automatically release the allocated memory, greatly reducing the burden on the programmer.

Common Pitfalls with String Pointers
Dangling Pointers
A common pitfall in working with pointers is creating dangling pointers. A dangling pointer refers to a pointer that still points to a memory location that has been freed or deleted. For example:
char* danglingPtr = new char[10];
delete[] danglingPtr; // Memory is freed
// danglingPtr now points to deallocated memory
To avoid this, it's a good practice to set pointers to `nullptr` after freeing them.
Out of Bounds Access
When dealing with C-style strings and pointers, out-of-bounds access is a significant risk. If you try to access memory beyond the allocated array, it can lead to undefined behavior. For example:
char str[10] = "Hello";
char c = str[10]; // Out-of-bounds access; causes undefined behavior
Always ensure that your pointer operations respect the allocated size to avoid such errors.

Conclusion
In conclusion, understanding C++ string pointers is essential for effective memory management and string manipulation. String pointers allow you to efficiently handle and modify string data, whether through static or dynamic allocation. By following best practices regarding memory management and avoiding common pitfalls, you can harness the full potential of pointers in C++. For further study, consider seeking out additional resources to deepen your understanding of C++ strings and pointers.