The `this` pointer in C++ refers to a special pointer that points to the object for which a member function is called, allowing access to the object's members and methods.
Here's a simple code snippet to illustrate its use:
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
void showValue() {
cout << "Value: " << this->value << endl; // using 'this' pointer
}
};
int main() {
MyClass obj(10);
obj.showValue(); // Output: Value: 10
return 0;
}
Understanding Pointers in C++
Pointers are a fundamental concept in C++ that enable developers to efficiently manage memory and create dynamic data structures. A pointer is a variable whose value is the address of another variable. Understanding pointers is crucial because they allow for direct access to memory, which can speed up the operations of a program when used wisely.
Basic pointer syntax in C++ is fairly straightforward. Pointers are declared using the asterisk (`*`) before the pointer’s name, and they can point to any data type. Here’s an example of pointer declaration and initialization:
int a = 10;
int* ptr = &a; // ptr points to the address of a
In this example, `ptr` holds the memory address of variable `a`.
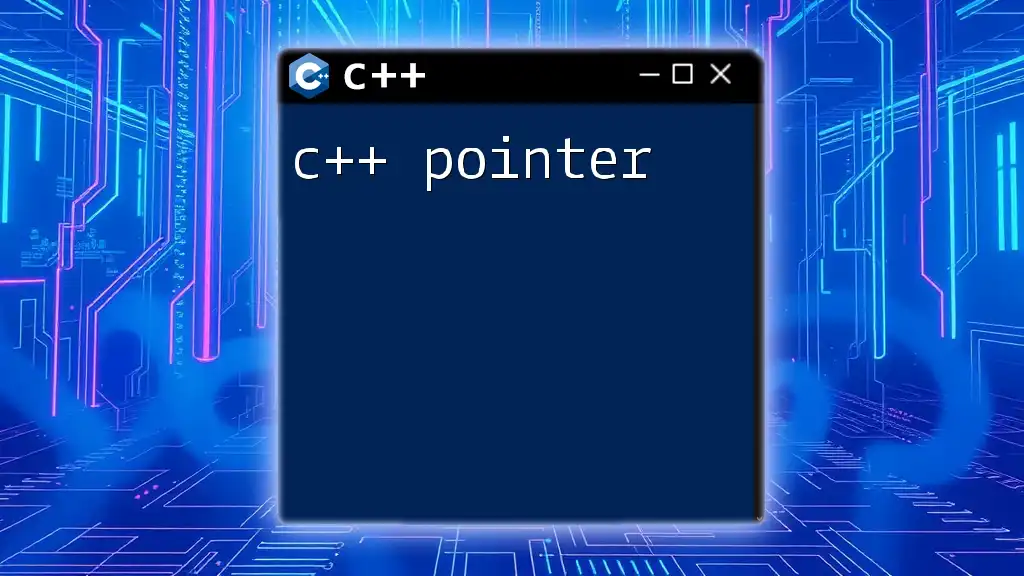
The Role of the this Pointer in C++
The `this` pointer is a special pointer in C++ that is automatically passed to non-static member functions. It serves as a reference to the object for which the member function is called. Understanding the `this` pointer is essential for manipulating object attributes and implementing class methods effectively.
Automatic Type Deduction
The `this` pointer provides a way to resolve ambiguity when member variables and parameters have the same name. It helps distinguish between the two. For example:
class Example {
public:
int value;
void setValue(int value) {
this->value = value; // Using 'this' to refer to the class member
}
};
In this snippet, `this->value` refers to the member variable of the class, while the unqualified `value` refers to the parameter passed to the function.
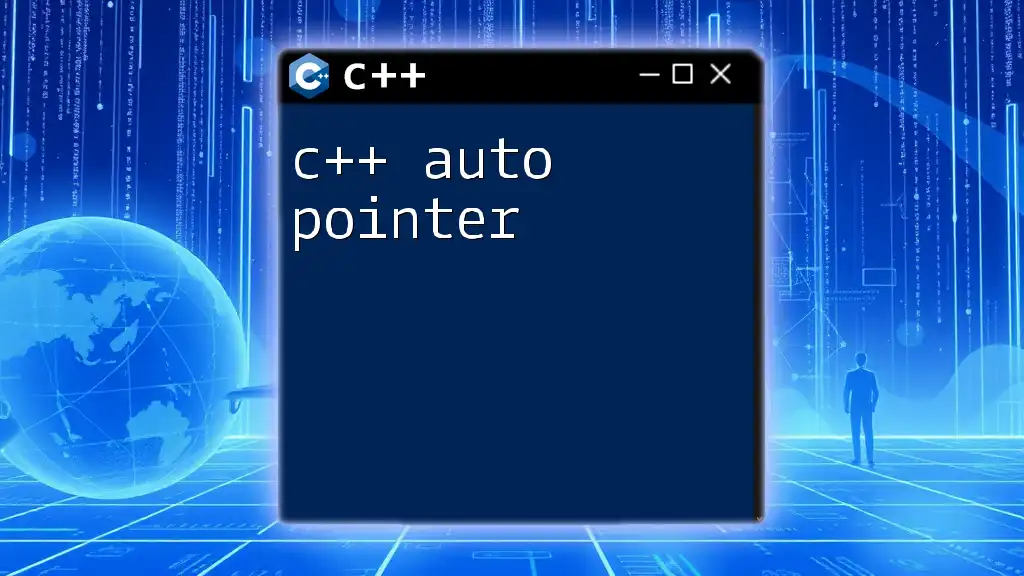
Using the this Pointer in Class Methods
Accessing Class Members
The `this` pointer can be employed to access and modify class members within member functions. This is particularly useful if the parameters of the functions shadow class member variables. Here’s a simple example:
class Counter {
private:
int count;
public:
Counter(int count) {
this->count = count; // Assigning the parameter value to the member variable
}
void increment() {
this->count++; // Using 'this' to explicitly refer to the member count
}
int getCount() {
return this->count; // Accessing the member variable using 'this'
}
};
In this example, the constructor and methods consistently use `this` to clarify which variable is being referenced.
Returning *this: Fluent Interfaces
The `this` pointer can also be essential for creating method chaining through fluent interfaces. When a member function returns `*this`, it allows other methods to be called on the same object within a single statement. Here’s how it works:
class Builder {
private:
int value;
public:
Builder& setValue(int val) {
this->value = val; // Assigning value
return *this; // Returning the current object
}
Builder& multiply(int factor) {
this->value *= factor; // Example operation
return *this; // Returning this for chaining
}
void display() {
std::cout << "Value: " << this->value << std::endl; // Displaying value
}
};
// Example usage
Builder builder;
builder.setValue(5).multiply(2).display(); // Chaining methods
In this demonstration, the `setValue` and `multiply` methods return the instance of `Builder`, allowing for concise method chaining.
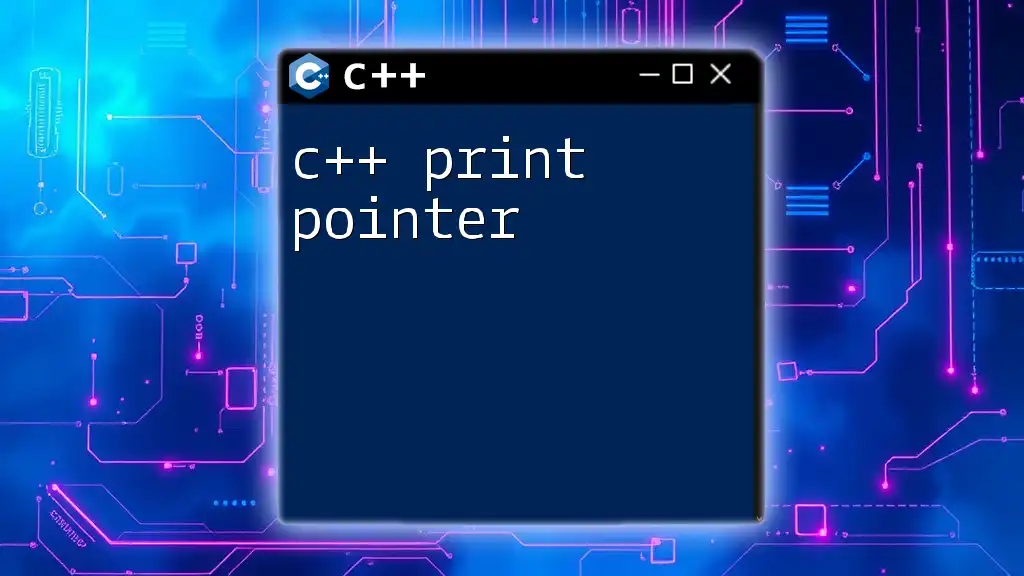
Common Use Cases for this Pointer
Constructor Initialization
When initializing member variables within a constructor, `this` simplifies assignments. It ensures that developers reference the correct variables, especially when parameters conflict with member names. This clarity is vital for maintaining readable and maintainable code.
Operator Overloading
The `this` pointer plays an important role in operator overloading, allowing operators to be defined for class objects. By using `this`, the class can naturally represent its current state when performing operations:
class Number {
public:
int value;
Number(int val) : value(val) {}
Number operator+(const Number& other) {
Number result(0);
result.value = this->value + other.value; // Using 'this' for current instance value
return result; // Returning a new Number object
}
};
// Example usage
Number num1(10);
Number num2(20);
Number num3 = num1 + num2; // Uses overloaded operator
This snippet shows how `this` is crucial for accessing member variables while defining custom behavior for the `+` operator.
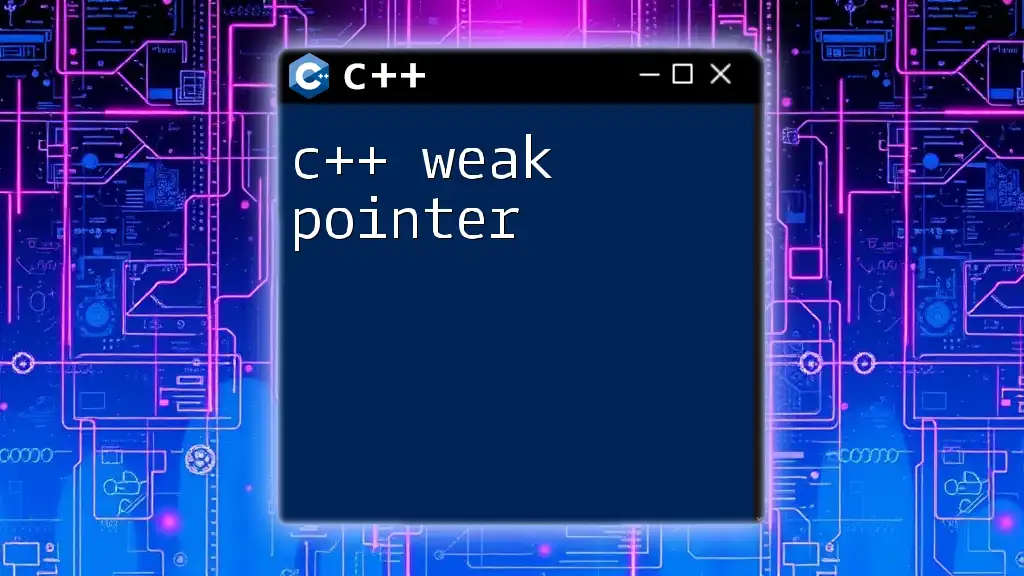
Limitations and Considerations
Const Member Functions
When defining `const` member functions, the usage of `this` is restricted to constant pointers. This means that within a `const` member function, `this` is of type `const ClassName*`. Here's an example illustrating this:
class ReadOnly {
private:
int value;
public:
ReadOnly(int val) : value(val) {}
int getValue() const {
return this->value; // Accessing value within a const function
}
void setValue(int val) { // Not allowed to modify using 'this'
this->value = val; // This would throw a compile error
}
};
Static Member Functions
Static member functions do not have access to `this` since they are not associated with a particular instance of a class. This prevents `static` functions from accessing instance variables or non-static methods directly. An example would clarify this limitation:
class MyClass {
public:
static void staticFunc() {
// this cannot be used here; example will throw an error
std::cout << "Static Function. No access to instance variables." << std::endl;
}
};
In `staticFunc`, attempting to use `this` results in a compile error, emphasizing the need to understand the context in which the `this` pointer can be utilized.
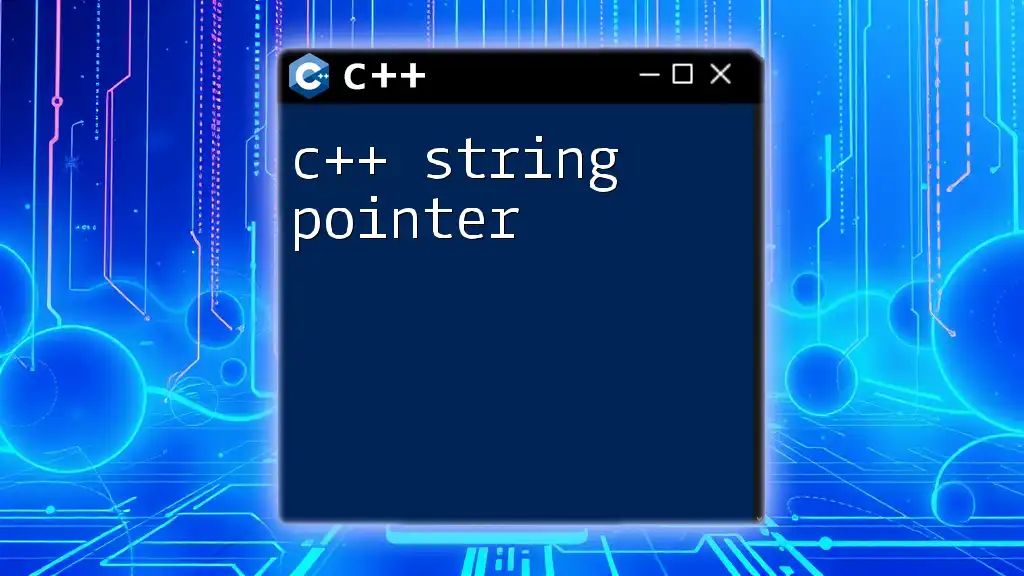
Debugging with this Pointer
Common Errors Related to this Pointer
Developers might encounter issues such as uninitialized pointers or incorrect memory access when misusing `this`. For example, using `this` within a static context will lead to compile-time errors, while dereferencing invalid pointers can cause runtime errors.
Debugging Techniques
When debugging code that involves the `this` pointer, consider using assertions to verify the memory addresses being accessed. Ensure that member functions are being called on properly instantiated objects. Additionally, clearly name your member variables so their uses are easily distinguishable even without `this`.
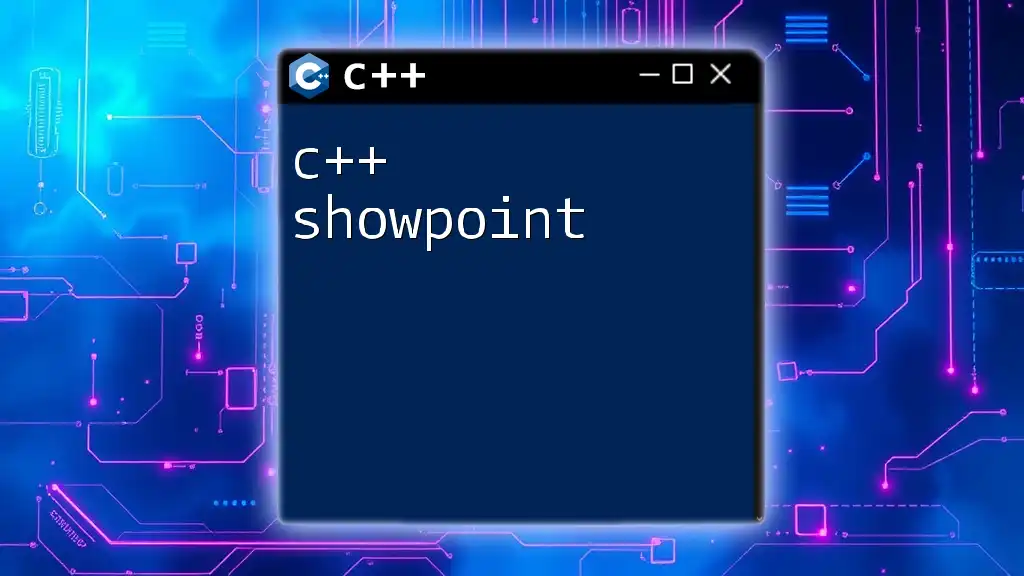
Conclusion
The `this` pointer in C++ is a powerful feature that enhances object-oriented programming by simplifying access to class members and enabling fluent interface design. Understanding how to effectively use `this` will result in clearer, more maintainable, and more efficient code.
As you continue to explore the intricacies of C++ and the capabilities of the `this` pointer, remember to practice using it in different scenarios. This will help solidify your understanding and establish best practices for your programming endeavors.
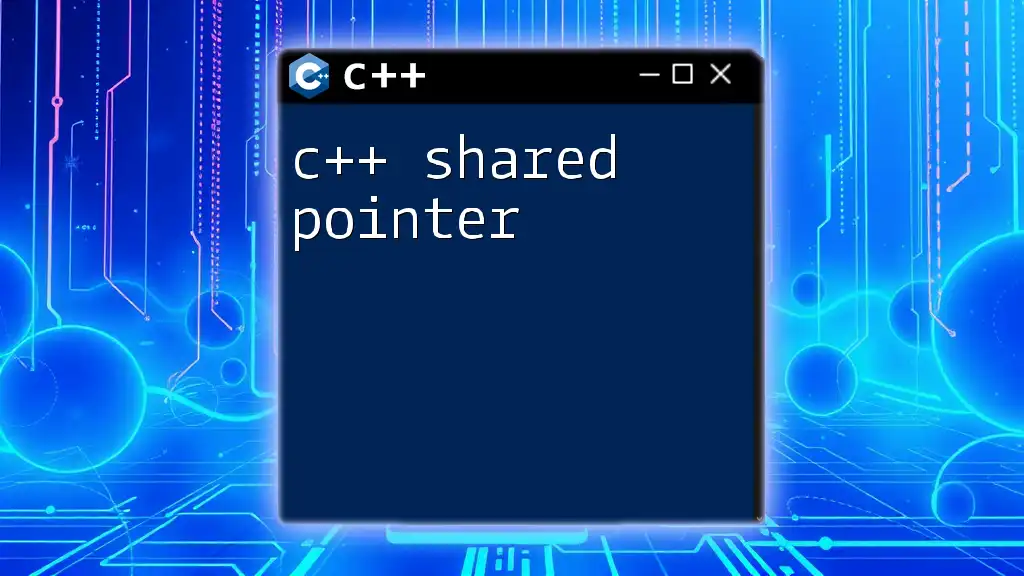
Additional Resources
To further your knowledge on pointers and classes in C++, consider checking out various books and online resources focused on these topics. Engaging with community forums and groups dedicated to C++ programming can also offer valuable insights and help with real-time questions.