In C++, a null pointer is a pointer that is not pointing to any object or function, and it can be initialized using the `nullptr` keyword or assigned the value of `0`.
Here’s a basic example:
#include <iostream>
int main() {
int* ptr = nullptr; // Initializing a null pointer
if (ptr == nullptr) {
std::cout << "Pointer is null." << std::endl;
}
return 0;
}
Understanding Null Pointers in C++
What is a Null Pointer in C++?
A null pointer is a special pointer that is guaranteed to point to no valid memory location. In C++, the concept of null pointers serves as an essential mechanism for managing pointer references and indicates that the pointer is not currently assigned to an object or memory. It is crucial to differentiate between a null pointer and an uninitialized pointer since the latter may point to an unpredictable memory location, leading to undefined behavior.
A null pointer can be defined using several forms, but starting with C++11, the use of `nullptr` is encouraged. This is preferable because `nullptr` provides a type-safe way to represent a pointer that does not point to any valid location.
How to Define a Null Pointer in C++
To define a null pointer in C++, you can simply initialize a pointer variable with `nullptr`. This approach enhances code clarity and safety. Here’s how it’s done:
int* ptr = nullptr; // Using nullptr in C++
Using `nullptr` is advisable over the older methods of representing a null pointer, such as using `NULL` or `0`, which can lead to ambiguity, especially in function overloads.
Why Null Pointers Matter
Understanding the importance of null pointers is critical in preventing common pitfalls that arise during pointer usage.
Avoiding Dangling Pointers
Dangling pointers occur when a pointer continues to reference a memory location after the memory has been deallocated. They can lead to catastrophic results including memory corruption or application crashes. By setting pointers to `nullptr` after they are deleted, you can avoid such issues, ensuring that pointers are explicitly marked as invalid when they should no longer be used.
Preventing Segmentation Faults
Null pointers play a fundamental role in preventing segmentation faults, which occur when a program attempts to access memory that it should not. By checking if a pointer is null before dereferencing it, you can significantly reduce the chance of encountering such runtime errors.
Best Practices for Using Null Pointers
Implementing best practices when dealing with null pointers can improve code robustness and prevent errors. One crucial practice is performing initial checks and validations before using pointers.
For example, use the following pattern to ensure that a pointer is safe to dereference:
if(ptr != nullptr) {
// Safe to dereference ptr
} else {
// Handle the case where ptr is null
}
This practice guarantees that any pointer operations only occur when there is a valid reference.
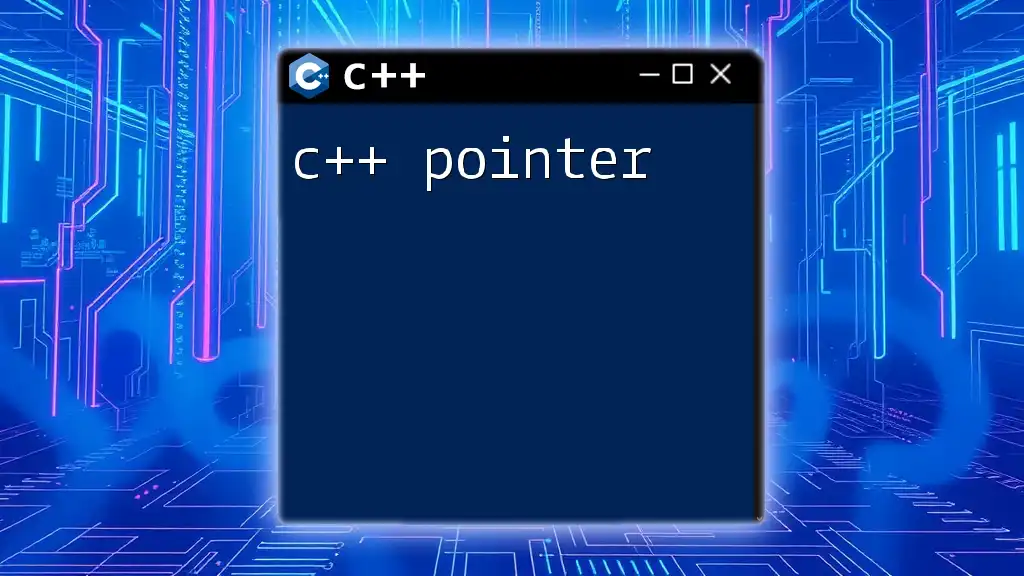
Common Use Cases of Null Pointers in C++
Managing Dynamic Memory
Dynamically allocated memory is a significant aspect of C++ programming, and null pointers are instrumental in avoiding memory management errors. When allocating memory using `new`, it’s vital to check whether the allocation was successful:
int* arr = new int[10];
if(arr == nullptr) {
// Handle memory allocation failure
}
If memory cannot be allocated, `arr` will be set to `nullptr`, allowing for safe failure handling instead of proceeding with invalid memory.
Null Pointers in Functions
Null pointers can also be beneficial when passing pointers to functions. This allows functions to accept an optional pointer, which can signify the absence of a value.
Consider the following function pattern:
void processPointer(int* ptr) {
if (ptr != nullptr) {
// Safely process ptr's value
} else {
// Handle the case where ptr is null
}
}
This ensures that the function will handle both scenarios—when a valid pointer is provided and when it is not.
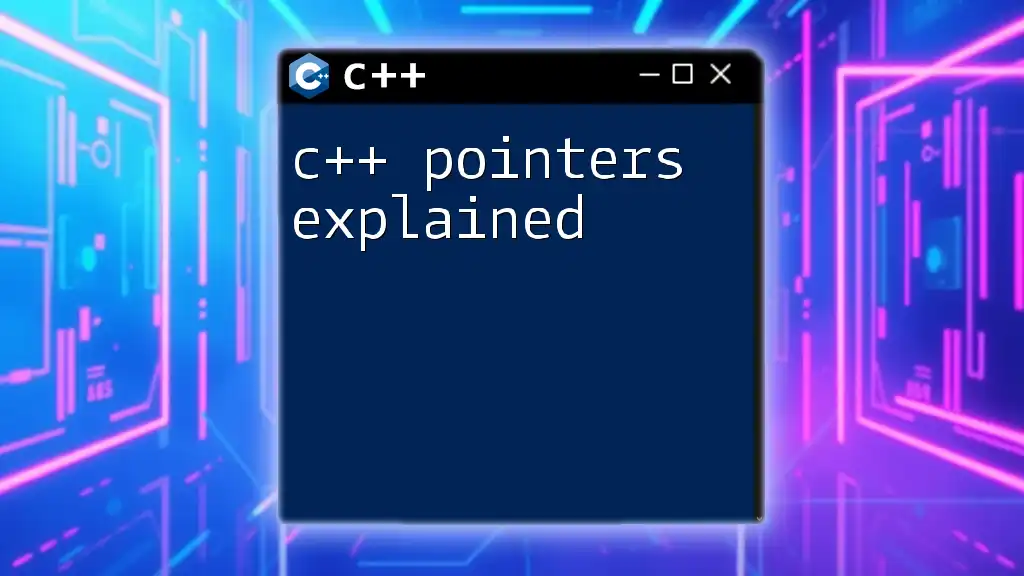
Debugging Issues with Null Pointers
Common Errors Involving Null Pointers
One of the most prevalent errors involving null pointers is the segmentation fault that occurs when attempting to dereference a null pointer. If the pointer points to `nullptr`, any attempt to access or modify the memory it supposedly points to will cause a crash.
Debugging Techniques
To effectively debug null pointer issues, assertions can be an invaluable tool. By including assertions in your code to ensure that pointers are not null before dereferencing, you bolster the safety of your program:
#include <cassert>
void safeDereference(int* ptr) {
assert(ptr != nullptr); // Ensures ptr is not null
// Proceed knowing ptr is safe to dereference
}
Using assertions can make debugging clearer and help catch errors during development rather than at runtime.
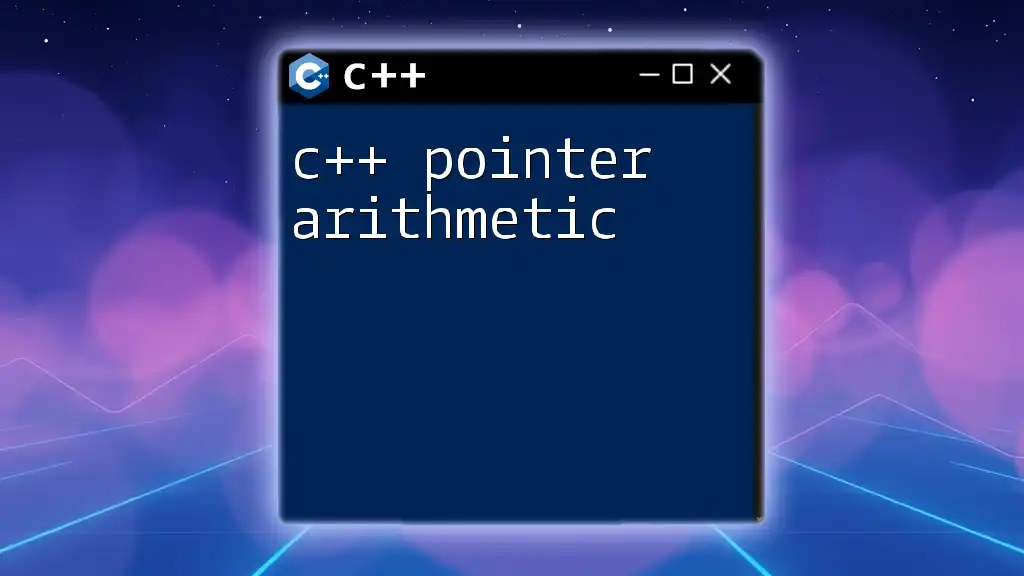
Conclusion
Recap of Key Points
Understanding and handling null pointers is a vital skill in C++ programming. By using `nullptr` and following best practices, you can prevent severe errors, maintain code clarity, and enhance the robustness of your applications.
Encouragement to Practice
For those looking to deepen their understanding, practice with varied pointer scenarios and memory management techniques will yield significant learning outcomes.
Additional Resources
Continuous learning about memory management, pointer arithmetic, and C++'s capabilities will further enrich your programming toolkit. Embrace the complexity of pointers in C++, as mastering them can lead to more powerful and efficient code.